HTML,text,value
text:会将所有元素的内容连接成一个字符串。
$("div").text("a");
$("div:lt(3)").text("a");
var arr=["北京","上海","天津","武汉","广州"]
$("div").text(function(index,item){
return arr[index];
html:仅获取jQuery列表中第一个元素的html内容
console.log($(“div”).html());
var arr=[
// {site:"网易",url:"www.163.com"},
// {site:"腾讯",url:"www.qq.com"},
// {site:"淘宝",url:"www.taobao.com"},
// {site:"京东",url:"www.jd.com"},
// {site:"天猫",url:"www.tmall.com"}
// ];
// $("div").html(function(index){
// // return `<a href='http://${arr[index].url}'>${arr[index].site}</a>`;
// let {site,url}=arr[index];
// return `<a href='http://${url}'>${site}</a>`;
val:只适用于input
// 仅获取jQuery列表中第一个元素的val内容
// console.log($("input").val());
$("input").on("blur").function(){
console.log($(this).val());
} (获取输入的值)
attr和prop
arr:仅能获取jQuery列表中第一个元素的指定属性的内容
$("div").attr("toggle","a");//给所有标签增加相同的属性名
// 给所有标签增加同一个属性名,但是不同的属性值
// $("div").attr("toggle",function(index){
// return index+1;
// })
给所有标签增加多个属性,并且属性值相同
// $("div").attr({
// key:1,
// name:"xie",
// class:"div0"
// })
// 给所有标签增加多个属性,并且属性值不同
// var names=["a","b","c","d"]
// $("div").attr({
// key:function(index){
// return index;
// },
// name:function(index){
// return names[index];
// },
// class:function(index){
// return "div"+index;
// },
// a:function(index){
// // 返回null就是不添加该属性
// return index===0 ? null : index;
// }
// });
removeAttr:删除标签属性
prop:自定义的对象属性和DOM元素的对象属性(在对象属性添加或删除,原标签不改变)
data 自定义的对象属性
css,class
css:可以设置div的多个属性,不同的属性值
addClass:添加
removeClass:删除
toggclass:切换样式
宽高
width():获取第一个元素宽度
innerwidth:宽度+(2*padding)
outerwidth:宽度+padding+border
outerWidth(true):不能设置,只能获取 width+padding+border+margin
offset:相对页面顶端的距离
position:和定位中left和top相同,相对父元素,等同于DOM offsetLeft和offsetTop。(不可以设置,用于获取)
scrollTop:获取当前的滚动条位置。可以获取
DOM元素的使用
append 添加在子元素的尾部
// $("div").append(div);
appendTo()将什么元素放在某个选择器的子项尾部,返回这个被放入的jQuery元素
append appendTo 插入在父容器的尾部
prepend prependTo 插入在父容器的头部
同级兄弟元素的插入
before(目标在后) insertBefore(目标在前) 插入在指定元素的前面
after insertAfter 插入在指定元素的后面
包裹
wrap:在元素的外层添加一个标签包裹这个元素
unwrap:删除
wrapAll:将所有选择内容外面统一增加一个父容器
wrapInner:将元素的子元素或者内容包裹一层标签
删除
remove:将当前JQ对象的事件删除
detach:仅删除元素,不删除事件,
empty:将内容清除掉
替换
要替换的内容.replaceWith(替换后的目标);
/ 替换后的目标.replaceAll(要替换的内容)
事件
on:添加事件(可以增加多个事件)
off:删除事件
off(“click”):删除匿名函数;删除所有当前点击对象的点击事件
,事件类型用空格隔开
e.data:是事件传入的参数对象
侦听时传参 on(事件类型,传的参数,事件函数)
抛发时传参 on(事件类型,事件函数(e,收到的参数)) trigger(事件类型,[抛发传入参数])
多个参数,是传递参数数组的解构赋值
trigger和triggerHander都可以抛发
trigger:会影响所有,会冒泡
triggerHandler:不会触发事件的默认行为,只影响第一个匹配的元素。
one:运行一次事件
blur:当元素失去焦点时,
focus:获得焦点
keydown:键盘点击事件
keyup:键盘松开
mousedown:鼠标点击事件
mousemove:鼠标移动
动画
hide:隐藏
show:显示
hide(100,function()){:动画完成后执行函数
tooggle:切换
slideUp:向上
slideDown:向下
fadeIn;fadeOut:淡入淡出,透明显示
自动切换淡入淡出的方法:fade Toggle();
fadeTo(): 透明时间;透明度
animate:只能变化数值
JQ插件
静态插件模式
$.extend()
随机颜色
(function(){
$.extend({
randomColor:function(){
var col="rgb(";
for(var i=0;i<3;i++){
col+=Math.floor(Math.random()*256)+",";
}
col=col.slice(0,-1);
return col+")";
}
});
$.fn.bg=function(color){
this.css("backgroundColor",color);
return this;
}
})();
$("<div></div>").appendTo("body").width(50).height(50).bg($.randomColor())
.clone(false).bg($.randomColor()).appendTo("body"
);
拖拽
动态插件方法 这种方法里面使用到this,this就是jQuery对象,里面必须返回this,以便于连缀
$.fn.bg=function(color){
if(!color) return this.css("backgroundColor");
this.css("backgroundColor",color);
return this;
}
$.fn.drag=function(bool){
if(!bool){
this.off("mousedown.drag");
return this;
}
this.css("position","absolute");
this.on("mousedown.drag",function(e1){
e1.stopPropagation();
var elem=$(this);
$(document).mousemove(function(e2){
elem.css({
left:e2.clientX-e1.offsetX,
top:e2.clientY-e1.offsetY
});
}).mouseup(function(){
$(document).off("mousemove mouseup");
})
});
return this;
}
$("div").width(50).height(50).bg("red").drag(true).dblclick(function(){
this.bool=!this.bool;
$(this).drag(!this.bool);
});
JQ中的AJAX
$.ajax(); 最基础的ajax
中层
// $("div").load();
// $.get();
// $.post();
// 最高层
// $.getScript();
// $.getJSON();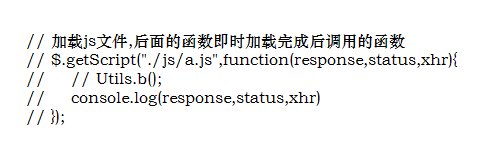
加载js文件,后面的函数即时加载完成后调用的函数
// $.getScript("./js/a.js",function(response,status,xhr){
// // Utils.b();
// console.log(response,status,xhr)
// });
// $.getJSON("./package-lock.json",function(response,status,xhr){
// console.log(response);//加载进来的json直接变成对象
// })
中层
// $.get("http://10.9.15.176:4001",{id:1001,name:"xietian"},function(response){
// console.log(response)
// })
// $.post("http://10.9.15.176:4002",{id:1001,name:"xietian"},function(response){
// console.log(response)
// })
加粗样式
// $("div").load("./a.xml");
// $("div").load("./package-lock.json");
// $(".div1").load("./菜单.html");
// $("div").load("http://10.9.15.176:4001?id=1001&name=xie");
最基础
// $.ajax({
// url:"http://10.9.15.176:4001",
// type:"get",
// data:{id:1001,name:"xietian"},
// success:function(res){
// console.log(res);
// }
// })
// $(":submit").click(function(e){
// e.preventDefault();
// $.ajax({
// url:"http://10.9.15.176:4001",
// type:"get",
// // 表单序列化的方式
// data:$("form").serialize(),
// success:function(res){
// console.log(res);
// }
// })
// })