1、在Linux下安装ICTCLAS2015
•1) 环境
Eclipse、Linux、ICTCLAS2015、jna-platform-4.1.0.jar( JNA类库)
•2) 安装ICTCLAS2015
在Linux下的 Eclipse中新建MapReduce Project,假设工程名为RF;
下载并解压ICTCLAS2015,将ICTCLAS中lib目录下libNLPIR.so文件(对应Linux 32位)拷入到RF下的src文件夹下(注意该路径下,还应该有log4j文件);
将ICTCLAS2015目录下的Data文件夹整个复制到项目RF中,放在根目录下;
导入JNA类库 jna-platform-4.1.0.jar
项目结构图,如下:
2、使用JNA调用C++接口
CLibrary类:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
package
nlpir2015.rf.cs.swjtu;
import
com.sun.jna.Library;
//import com.sun.jna.Library;
public
interface
CLibrary
extends
Library{
//初始化
public
int
NLPIR_Init(String sDataPath,
int
encoding, String sLicenceCode);
//对字符串进行分词
public
String NLPIR_ParagraphProcess(String sSrc,
int
bPOSTagged);
//对TXT文件内容进行分词
public
double
NLPIR_FileProcess(String sSourceFilename,String sResultFilename,
int
bPOStagged);
//从字符串中提取关键词
public
String NLPIR_GetKeyWords(String sLine,
int
nMaxKeyLimit,
boolean
bWeightOut);
//从TXT文件中提取关键词
public
String NLPIR_GetFileKeyWords(String sLine,
int
nMaxKeyLimit,
boolean
bWeightOut);
//添加单条用户词典
public
int
NLPIR_AddUserWord(String sWord);
//删除单条用户词典
public
int
NLPIR_DelUsrWord(String sWord);
//从TXT文件中导入用户词典
public
int
NLPIR_ImportUserDict(String sFilename);
//将用户词典保存至硬盘
public
int
NLPIR_SaveTheUsrDic();
//从字符串中获取新词
public
String NLPIR_GetNewWords(String sLine,
int
nMaxKeyLimit,
boolean
bWeightOut);
//从TXT文件中获取新词
public
String NLPIR_GetFileNewWords(String sTextFile,
int
nMaxKeyLimit,
boolean
bWeightOut);
//获取一个字符串的指纹值
public
long
NLPIR_FingerPrint(String sLine);
//设置要使用的POS map
public
int
NLPIR_SetPOSmap(
int
nPOSmap);
//获取报错日志
public
String NLPIR_GetLastErrorMsg();
//退出
public
void
NLPIR_Exit();
}
3、分词类feici
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
package
nlpir2015.rf.cs.swjtu;
import
java.util.concurrent.ExecutorService;
import
java.util.concurrent.Executors;
import
com.sun.jna.Native;
public
class
fenci {
//Windows下的加载方式。如果需要支持Linux,需要修改这一行为libNLPIR.so的路径。
String Path=System.getProperty(
"user.dir"
).toString();
CLibrary Instance = (CLibrary)Native.loadLibrary(
"NLPIR"
, CLibrary.
class
);
private
boolean
initFlag =
false
;
public
boolean
init(){
String argu =
null
;
// String system_charset = "GBK";//GBK----0
int
charset_type =
1
;
int
init_flag = Instance.NLPIR_Init(argu, charset_type,
"0"
);
String nativeBytes =
null
;
if
(
0
== init_flag) {
nativeBytes = Instance.NLPIR_GetLastErrorMsg();
System.err.println(
"初始化失败!fail reason is "
+nativeBytes);
return
false
;
}
initFlag =
true
;
return
true
;
}
public
boolean
unInit(){
try
{
Instance.NLPIR_Exit();
}
catch
(Exception e) {
System.out.println(e);
return
false
;
}
initFlag =
false
;
return
true
;
}
public
String parseSen(String str){
String nativeBytes =
null
;
try
{
nativeBytes = Instance.NLPIR_ParagraphProcess(str,
0
);
}
catch
(Exception ex) {
// TODO Auto-generated catch block
ex.printStackTrace();
}
return
nativeBytes;
}
public
CLibrary getInstance() {
return
Instance;
}
public
boolean
isInitFlag() {
return
initFlag;
}
}
4、编写MapReduce函数
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
package
segwords.rf.cs.swjtu;
import
java.io.IOException;
import
nlpir2015.rf.cs.swjtu.fenci;
import
org.apache.hadoop.io.Text;
import
org.apache.hadoop.mapreduce.Mapper;
import
org.apache.hadoop.mapreduce.Reducer;
public
class
SegWords {
public
static
class
SegWordsMap
extends
Mapper<Object, Text, Text, Text> {
fenci tt =
new
fenci();
//
protected
void
setup(Context context)
throws
IOException, InterruptedException {
tt.init();
}
public
void
map(Object key, Text value,Context context)
throws
IOException {
String line = value.toString();
line = tt.parseSen(line.replaceAll(
"[\\pP‘’“”]"
,
""
));
try
{
context.write(
new
Text(
""
),
new
Text(line));
System.out.println(line);
}
catch
(InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
protected
void
cleanup(Context context){
tt.unInit();
}
}
public
static
class
SegWordsReduce
extends
Reducer<Text, Text, Text, Text> {
public
void
reduce(Text key, Text value, Context context)
throws
IOException, NumberFormatException, InterruptedException {
context.write(key, value);
//
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
package
segwords.rf.cs.swjtu;
import
java.io.IOException;
import
org.apache.hadoop.conf.Configuration;
import
org.apache.hadoop.fs.FileSystem;
import
org.apache.hadoop.fs.Path;
import
org.apache.hadoop.io.Text;
import
org.apache.hadoop.mapreduce.Job;
import
org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import
org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import
segwords.rf.cs.swjtu.SegWords.SegWordsMap;
import
segwords.rf.cs.swjtu.SegWords.SegWordsReduce;
public
class
tcMain {
/**
* @param args
* @throws IOException
* @throws InterruptedException
* @throws ClassNotFoundException
*/
public
static
void
main(String[] args)
throws
IOException, ClassNotFoundException, InterruptedException {
// TODO Auto-generated method stub
//JOB 1 TF
Path segIn =
new
Path(
"hdfs://localhost:9000/input/segIn"
);
Path segOut =
new
Path(
"hdfs://localhost:9000/output/segOut"
);
Configuration conf1=
new
Configuration();
Job job1=
new
Job(conf1,
"segWords"
);
FileSystem fs1 = FileSystem.get(conf1);
if
(fs1.exists(segOut)){
fs1.delete(segOut);
}
fs1.close();
job1.setJarByClass(SegWords.
class
);
job1.setMapperClass( SegWordsMap.
class
);
job1.setReducerClass( SegWordsReduce.
class
);
job1.setOutputKeyClass(Text.
class
);
job1.setOutputValueClass(Text.
class
);
FileInputFormat.addInputPath(job1, segIn);
FileOutputFormat.setOutputPath(job1, segOut);
//System.exit(job1.waitForCompletion(true)?0:1);
job1.waitForCompletion(
true
);
}
}
5、并行分词结果
1)输入文档(2个文档模拟2个split)
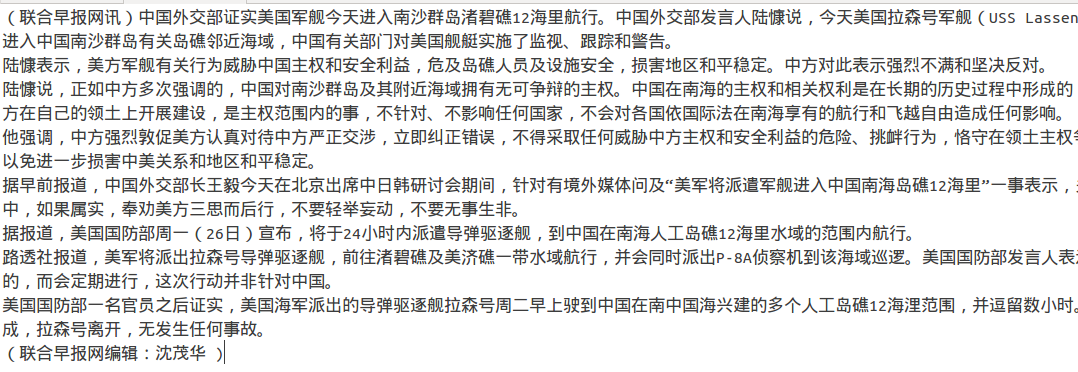
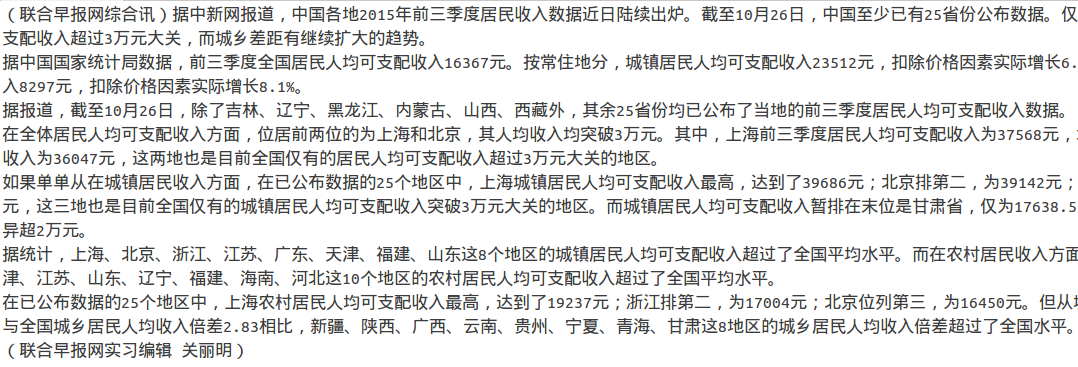
2)结果
15/10/28 01:07:12 WARN util.NativeCodeLoader: Unable to load native-hadoop library for your platform... using builtin-java classes where applicable
15/10/28 01:07:16 INFO Configuration.deprecation: session.id is deprecated. Instead, use dfs.metrics.session-id
15/10/28 01:07:16 INFO jvm.JvmMetrics: Initializing JVM Metrics with processName=JobTracker, sessionId=
15/10/28 01:07:16 WARN mapreduce.JobSubmitter: Hadoop command-line option parsing not performed. Implement the Tool interface and execute your application with ToolRunner to remedy this.
15/10/28 01:07:18 INFO input.FileInputFormat: Total input paths to process : 2
15/10/28 01:07:18 INFO mapreduce.JobSubmitter: number of splits:2
15/10/28 01:07:18 INFO mapreduce.JobSubmitter: Submitting tokens for job: job_local598241144_0001
15/10/28 01:07:19 INFO mapreduce.Job: The url to track the job: http://localhost:8080/
15/10/28 01:07:19 INFO mapreduce.Job: Running job: job_local598241144_0001
15/10/28 01:07:19 INFO mapred.LocalJobRunner: OutputCommitter set in config null
15/10/28 01:07:19 INFO mapred.LocalJobRunner: OutputCommitter is org.apache.hadoop.mapreduce.lib.output.FileOutputCommitter
15/10/28 01:07:19 INFO mapred.LocalJobRunner: Waiting for map tasks
15/10/28 01:07:19 INFO mapred.LocalJobRunner: Starting task: attempt_local598241144_0001_m_000000_0
15/10/28 01:07:19 INFO mapred.Task: Using ResourceCalculatorProcessTree : [ ]
15/10/28 01:07:20 INFO mapreduce.Job: Job job_local598241144_0001 running in uber mode : false
15/10/28 01:07:20 INFO mapreduce.Job: map 0% reduce 0%
15/10/28 01:07:20 INFO mapred.MapTask: Processing split: hdfs://localhost:9000/input/segIn/segtest01:0+2359
15/10/28 01:07:23 INFO mapred.MapTask: (EQUATOR) 0 kvi 26214396(104857584)
15/10/28 01:07:23 INFO mapred.MapTask: mapreduce.task.io.sort.mb: 100
15/10/28 01:07:23 INFO mapred.MapTask: soft limit at 83886080
15/10/28 01:07:23 INFO mapred.MapTask: bufstart = 0; bufvoid = 104857600
15/10/28 01:07:23 INFO mapred.MapTask: kvstart = 26214396; length = 6553600
15/10/28 01:07:23 INFO mapred.MapTask: Map output collector class = org.apache.hadoop.mapred.MapTask$MapOutputBuffer
15/10/28 01:07:40 INFO mapred.LocalJobRunner:
联合早报网 综合 讯 据 中新网 报道 中国 各地 2015年 前 三 季度 居民 收入 数据 近日 陆续 出炉 截至 10月 26日 中国 至少 已 有 25 省份 公布 数据 仅 上海 北京 全体 居民 人均 可 支配 收入 超过 3万 元 大关 而 城乡 差距 有 继续 扩大 的 趋势
据 中国 国家 统计局 数据 前 三 季度 全国 居民 人均 可 支配 收入 16367 元 按 常住 地 分 城镇 居民 人均 可 支配 收入 23512 元 扣除 价格 因素 实际 增长 68 农村 居民 人均 可 支配 收入 8297 元 扣除 价格 因素 实际 增长 81
据 报道 截至 10月 26日 除了 吉林 辽宁 黑龙江 内蒙古 山西 西藏 外 其余 25 省份 均 已 公布 了 当地 的 前 三 季度 居民 人均 可 支配 收入 数据
在 全体 居民 人均 可 支配 收入 方面 位居 前 两 位 的 为 上海 和 北京 其 人均收入 均 突破 3万 元 其中 上海 前 三 季度 居民 人均 可 支配 收入 为 37568 元 北京 前 三 季度 居民 人均 可 支配 收入 为 36047 元 这 两 地 也 是 目前 全国 仅 有 的 居民 人均 可 支配 收入 超过 3万 元 大关 的 地区
如果 单 单 从 在 城镇 居民 收入 方面 在 已 公布 数据 的 25 个 地区 中 上海 城镇 居民 人均 可 支配 收入 最高 达到 了 39686 元 北京 排 第二 为 39142 元 浙江 位列 第三 为 33464 元 这 三 地 也 是 目前 全国 仅 有 的 城镇 居民 人均 可 支配 收入 突破 3万 元 大关 的 地区 而 城镇 居民 人均 可 支配 收入 暂 排 在 末 位 是 甘肃省 仅 为 176385 元 收入 最高 和 最低 地区 的 差异 超 2万 元
据 统计 上海 北京 浙江 江苏 广东 天津 福建 山东 这 8 个 地区 的 城镇 居民 人均 可 支配 收入 超过 了 全国 平均 水平 而 在 农村 居民 收入 方面 上海 浙江 北京 天津 江苏 山东 辽宁 福建 海南 河北 这 10 个 地区 的 农村 居民 人均 可 支配 收入 超过 了 全国 平均 水平
在 已 公布 数据 的 25 个 地区 中 上海 农村 居民 人均 可 支配 收入 最高 达到 了 19237 元 浙江 排 第二 为 17004 元 北京 位 列 第三 为 16450 元 但 从 城乡 居民 人均收入 倍 差 来 看 与 全国 城乡 居民 人均收入 倍 差 283 相比 新疆 陕西 广西 云南 贵州 宁夏 青海 甘肃 这 8 地区 的 城乡 居民 人均收入 倍 差 超过 了 全国 水平
联合早报网 实习 编辑 关丽明
15/10/28 01:07:41 INFO mapred.MapTask: Starting flush of map output
15/10/28 01:07:41 INFO mapred.MapTask: Spilling map output
15/10/28 01:07:41 INFO mapred.MapTask: bufstart = 0; bufend = 2552; bufvoid = 104857600
15/10/28 01:07:41 INFO mapred.MapTask: kvstart = 26214396(104857584); kvend = 26214368(104857472); length = 29/6553600
15/10/28 01:07:41 INFO mapred.MapTask: Finished spill 0
15/10/28 01:07:41 INFO mapred.Task: Task:attempt_local598241144_0001_m_000000_0 is done. And is in the process of committing
15/10/28 01:07:42 INFO mapred.LocalJobRunner: map
15/10/28 01:07:42 INFO mapred.Task: Task 'attempt_local598241144_0001_m_000000_0' done.
15/10/28 01:07:42 INFO mapred.LocalJobRunner: Finishing task: attempt_local598241144_0001_m_000000_0
15/10/28 01:07:42 INFO mapred.LocalJobRunner: Starting task: attempt_local598241144_0001_m_000001_0
15/10/28 01:07:42 INFO mapred.Task: Using ResourceCalculatorProcessTree : [ ]
15/10/28 01:07:42 INFO mapreduce.Job: map 100% reduce 0%
15/10/28 01:07:42 INFO mapred.MapTask: Processing split: hdfs://localhost:9000/input/segIn/segtest00:0+2314
15/10/28 01:07:42 INFO mapred.MapTask: (EQUATOR) 0 kvi 26214396(104857584)
15/10/28 01:07:42 INFO mapred.MapTask: mapreduce.task.io.sort.mb: 100
15/10/28 01:07:42 INFO mapred.MapTask: soft limit at 83886080
15/10/28 01:07:42 INFO mapred.MapTask: bufstart = 0; bufvoid = 104857600
15/10/28 01:07:42 INFO mapred.MapTask: kvstart = 26214396; length = 6553600
15/10/28 01:07:42 INFO mapred.MapTask: Map output collector class = org.apache.hadoop.mapred.MapTask$MapOutputBuffer
联合早报网 讯 中国 外交部 证实 美国 军舰 今天 进入 南沙 群岛 渚 碧 礁 12 海里 航行 中 国 外交部 发言人 陆 慷 说 今天 美国 拉森 号 军舰 USS Lassen 未经 中国政府 允许 非法 进入 中国 南沙 群岛 有关 岛礁 邻近 海域 中国 有关 部门 对 美国 舰艇 实施 了 监视 跟踪 和 警告
陆 慷 表示 美方 军舰 有关 行为 威胁 中国 主权 和 安全 利益 危及 岛礁 人员 及 设施 安全 损害 地区 和平 稳定 中方 对 此 表示 强烈 不满 和 坚决 反对
陆 慷 说 正 如 中方 多次 强调 的 中国 对 南沙 群岛 及其 附近 海域 拥有 无可争辩 的 主权 中国 在 南海 的 主权 和 相关 权利 是 在 长期 的 历史 过程 中 形成 的 为 历代 中国政府 所 坚持 中方 在 自己 的 领土 上 开展 建设 是 主权 范围 内 的 事 不 针对 不 影响 任何 国家 不 会 对 各国 依 国际法 在 南海 享有 的 航行 和 飞越 自由 造成 任何 影响
他 强调 中方 强烈 敦促 美方 认真 对待 中方 严正 交涉 立即 纠正 错误 不得 采取 任何 威胁 中方 主权 和 安全 利益 的 危险 挑衅 行为 恪守 在 领土 主权 争议 问题 上 不 持 立场 的 承诺 以免 进一步 损害 中 美 关系 和 地区 和平 稳定
据 早 前 报道 中国 外交部长 王毅 今天 在 北京 出席 中 日 韩 研讨会 期间 针对 有 境外 媒体 问及 美军 将 派遣 军舰 进入 中国 南海 岛礁 12 海里 一 事 表示 关于 此事 中方 正在 核实 当中 如果 属实 奉劝 美方 三思而后行 不要 轻举妄动 不要 无事生非
据 报道 美国 国防部 周一 26日 宣布 将 于 24 小时 内 派遣 导弹 驱逐舰 到 中国 在 南海 人工岛 礁 12 海里 水域 的 范围 内 航行
路透社 报道 美军 将 派出 拉森 号 导弹 驱逐舰 前往 渚 碧 礁 及 美 济 礁 一带 水域 航行 并 会同 时 派出 P8A 侦察机 到 该 海域 巡逻 美国 国防部 发言人 表示 美军 的 行动 不 会 是 一次性 的 而 会 定期 进行 这次 行动 并非 针对 中国
美国 国防部 一 名 官员 之后 证实 美国 海军 派出 的 导弹 驱逐舰 拉森 号 周二 早上 驶 到 中国 在 南中国海 兴建 的 多 个 人工 岛礁 12 海 浬 范围 并 逗留 数 小时 美军 之后 表示 行动 已经 完成 拉森 号 离开 无 发生 任何 事故
联合早报网 编辑 沈茂华
15/10/28 01:07:51 INFO mapred.LocalJobRunner:
15/10/28 01:07:51 INFO mapred.MapTask: Starting flush of map output
15/10/28 01:07:51 INFO mapred.MapTask: Spilling map output
15/10/28 01:07:51 INFO mapred.MapTask: bufstart = 0; bufend = 2534; bufvoid = 104857600
15/10/28 01:07:51 INFO mapred.MapTask: kvstart = 26214396(104857584); kvend = 26214364(104857456); length = 33/6553600
15/10/28 01:07:51 INFO mapred.MapTask: Finished spill 0
15/10/28 01:07:51 INFO mapred.Task: Task:attempt_local598241144_0001_m_000001_0 is done. And is in the process of committing
15/10/28 01:07:51 INFO mapred.LocalJobRunner: map
15/10/28 01:07:51 INFO mapred.Task: Task 'attempt_local598241144_0001_m_000001_0' done.
15/10/28 01:07:51 INFO mapred.LocalJobRunner: Finishing task: attempt_local598241144_0001_m_000001_0
15/10/28 01:07:51 INFO mapred.LocalJobRunner: map task executor complete.
15/10/28 01:07:52 INFO mapred.LocalJobRunner: Waiting for reduce tasks
15/10/28 01:07:52 INFO mapred.LocalJobRunner: Starting task: attempt_local598241144_0001_r_000000_0
15/10/28 01:07:52 INFO mapred.Task: Using ResourceCalculatorProcessTree : [ ]
15/10/28 01:07:52 INFO mapred.ReduceTask: Using ShuffleConsumerPlugin: org.apache.hadoop.mapreduce.task.reduce.Shuffle@1f0dbfd
15/10/28 01:07:53 INFO reduce.MergeManagerImpl: MergerManager: memoryLimit=178821520, maxSingleShuffleLimit=44705380, mergeThreshold=118022208, ioSortFactor=10, memToMemMergeOutputsThreshold=10
15/10/28 01:07:53 INFO reduce.EventFetcher: attempt_local598241144_0001_r_000000_0 Thread started: EventFetcher for fetching Map Completion Events
15/10/28 01:07:54 INFO reduce.LocalFetcher: localfetcher#1 about to shuffle output of map attempt_local598241144_0001_m_000001_0 decomp: 2568 len: 2572 to MEMORY
15/10/28 01:07:54 INFO reduce.InMemoryMapOutput: Read 2568 bytes from map-output for attempt_local598241144_0001_m_000001_0
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: closeInMemoryFile -> map-output of size: 2568, inMemoryMapOutputs.size() -> 1, commitMemory -> 0, usedMemory ->2568
15/10/28 01:07:54 INFO reduce.LocalFetcher: localfetcher#1 about to shuffle output of map attempt_local598241144_0001_m_000000_0 decomp: 2583 len: 2587 to MEMORY
15/10/28 01:07:54 INFO reduce.InMemoryMapOutput: Read 2583 bytes from map-output for attempt_local598241144_0001_m_000000_0
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: closeInMemoryFile -> map-output of size: 2583, inMemoryMapOutputs.size() -> 2, commitMemory -> 2568, usedMemory ->5151
15/10/28 01:07:54 INFO reduce.EventFetcher: EventFetcher is interrupted.. Returning
15/10/28 01:07:54 INFO mapred.LocalJobRunner: 2 / 2 copied.
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: finalMerge called with 2 in-memory map-outputs and 0 on-disk map-outputs
15/10/28 01:07:54 INFO mapred.Merger: Merging 2 sorted segments
15/10/28 01:07:54 INFO mapred.Merger: Down to the last merge-pass, with 2 segments left of total size: 5145 bytes
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: Merged 2 segments, 5151 bytes to disk to satisfy reduce memory limit
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: Merging 1 files, 5153 bytes from disk
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: Merging 0 segments, 0 bytes from memory into reduce
15/10/28 01:07:54 INFO mapred.Merger: Merging 1 sorted segments
15/10/28 01:07:54 INFO mapred.Merger: Down to the last merge-pass, with 1 segments left of total size: 5146 bytes
15/10/28 01:07:55 INFO mapred.LocalJobRunner: 2 / 2 copied.
15/10/28 01:07:57 INFO Configuration.deprecation: mapred.skip.on is deprecated. Instead, use mapreduce.job.skiprecords
15/10/28 01:07:58 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:07:59 INFO mapreduce.Job: map 100% reduce 100%
15/10/28 01:08:01 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:08:05 INFO mapred.Task: Task:attempt_local598241144_0001_r_000000_0 is done. And is in the process of committing
15/10/28 01:08:05 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:08:05 INFO mapred.Task: Task attempt_local598241144_0001_r_000000_0 is allowed to commit now
15/10/28 01:08:05 INFO output.FileOutputCommitter: Saved output of task 'attempt_local598241144_0001_r_000000_0' to hdfs://localhost:9000/output/segOut/_temporary/0/task_local598241144_0001_r_000000
15/10/28 01:08:05 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:08:05 INFO mapred.Task: Task 'attempt_local598241144_0001_r_000000_0' done.
15/10/28 01:08:05 INFO mapred.LocalJobRunner: Finishing task: attempt_local598241144_0001_r_000000_0
15/10/28 01:08:05 INFO mapred.LocalJobRunner: reduce task executor complete.
15/10/28 01:08:07 INFO mapreduce.Job: Job job_local598241144_0001 completed successfully
15/10/28 01:08:07 INFO mapreduce.Job: Counters: 38
File System Counters
FILE: Number of bytes read=33097684
FILE: Number of bytes written=34155860
FILE: Number of read operations=0
FILE: Number of large read operations=0
FILE: Number of write operations=0
HDFS: Number of bytes read=11705
HDFS: Number of bytes written=5059
HDFS: Number of read operations=28
HDFS: Number of large read operations=0
HDFS: Number of write operations=8
Map-Reduce Framework
Map input records=17
Map output records=17
Map output bytes=5086
Map output materialized bytes=5159
Input split bytes=216
Combine input records=0
Combine output records=0
Reduce input groups=1
Reduce shuffle bytes=5159
Reduce input records=17
Reduce output records=17
Spilled Records=34
Shuffled Maps =2
Failed Shuffles=0
Merged Map outputs=2
GC time elapsed (ms)=179
CPU time spent (ms)=0
Physical memory (bytes) snapshot=0
Virtual memory (bytes) snapshot=0
Total committed heap usage (bytes)=457125888
Shuffle Errors
BAD_ID=0
CONNECTION=0
IO_ERROR=0
WRONG_LENGTH=0
WRONG_MAP=0
WRONG_REDUCE=0
File Input Format Counters
Bytes Read=4673
File Output Format Counters
Bytes Written=5059
http://blog.csdn.net/luoyhang003/article/details/44586731
http://jingyan.baidu.com/article/9158e0004251b4a2541228e5.html
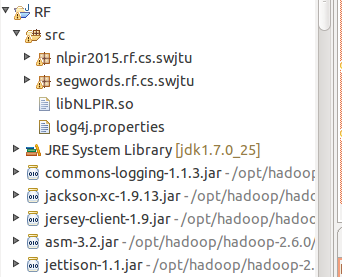
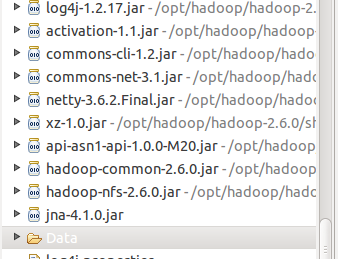
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
package
nlpir2015.rf.cs.swjtu;
import
com.sun.jna.Library;
//import com.sun.jna.Library;
public
interface
CLibrary
extends
Library{
//初始化
public
int
NLPIR_Init(String sDataPath,
int
encoding, String sLicenceCode);
//对字符串进行分词
public
String NLPIR_ParagraphProcess(String sSrc,
int
bPOSTagged);
//对TXT文件内容进行分词
public
double
NLPIR_FileProcess(String sSourceFilename,String sResultFilename,
int
bPOStagged);
//从字符串中提取关键词
public
String NLPIR_GetKeyWords(String sLine,
int
nMaxKeyLimit,
boolean
bWeightOut);
//从TXT文件中提取关键词
public
String NLPIR_GetFileKeyWords(String sLine,
int
nMaxKeyLimit,
boolean
bWeightOut);
//添加单条用户词典
public
int
NLPIR_AddUserWord(String sWord);
//删除单条用户词典
public
int
NLPIR_DelUsrWord(String sWord);
//从TXT文件中导入用户词典
public
int
NLPIR_ImportUserDict(String sFilename);
//将用户词典保存至硬盘
public
int
NLPIR_SaveTheUsrDic();
//从字符串中获取新词
public
String NLPIR_GetNewWords(String sLine,
int
nMaxKeyLimit,
boolean
bWeightOut);
//从TXT文件中获取新词
public
String NLPIR_GetFileNewWords(String sTextFile,
int
nMaxKeyLimit,
boolean
bWeightOut);
//获取一个字符串的指纹值
public
long
NLPIR_FingerPrint(String sLine);
//设置要使用的POS map
public
int
NLPIR_SetPOSmap(
int
nPOSmap);
//获取报错日志
public
String NLPIR_GetLastErrorMsg();
//退出
public
void
NLPIR_Exit();
}
|
3、分词类feici
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
|
package
nlpir2015.rf.cs.swjtu;
import
java.util.concurrent.ExecutorService;
import
java.util.concurrent.Executors;
import
com.sun.jna.Native;
public
class
fenci {
//Windows下的加载方式。如果需要支持Linux,需要修改这一行为libNLPIR.so的路径。
String Path=System.getProperty(
"user.dir"
).toString();
CLibrary Instance = (CLibrary)Native.loadLibrary(
"NLPIR"
, CLibrary.
class
);
private
boolean
initFlag =
false
;
public
boolean
init(){
String argu =
null
;
// String system_charset = "GBK";//GBK----0
int
charset_type =
1
;
int
init_flag = Instance.NLPIR_Init(argu, charset_type,
"0"
);
String nativeBytes =
null
;
if
(
0
== init_flag) {
nativeBytes = Instance.NLPIR_GetLastErrorMsg();
System.err.println(
"初始化失败!fail reason is "
+nativeBytes);
return
false
;
}
initFlag =
true
;
return
true
;
}
public
boolean
unInit(){
try
{
Instance.NLPIR_Exit();
}
catch
(Exception e) {
System.out.println(e);
return
false
;
}
initFlag =
false
;
return
true
;
}
public
String parseSen(String str){
String nativeBytes =
null
;
try
{
nativeBytes = Instance.NLPIR_ParagraphProcess(str,
0
);
}
catch
(Exception ex) {
// TODO Auto-generated catch block
ex.printStackTrace();
}
return
nativeBytes;
}
public
CLibrary getInstance() {
return
Instance;
}
public
boolean
isInitFlag() {
return
initFlag;
}
}
|
4、编写MapReduce函数
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
package
segwords.rf.cs.swjtu;
import
java.io.IOException;
import
nlpir2015.rf.cs.swjtu.fenci;
import
org.apache.hadoop.io.Text;
import
org.apache.hadoop.mapreduce.Mapper;
import
org.apache.hadoop.mapreduce.Reducer;
public
class
SegWords {
public
static
class
SegWordsMap
extends
Mapper<Object, Text, Text, Text> {
fenci tt =
new
fenci();
//
protected
void
setup(Context context)
throws
IOException, InterruptedException {
tt.init();
}
public
void
map(Object key, Text value,Context context)
throws
IOException {
String line = value.toString();
line = tt.parseSen(line.replaceAll(
"[\\pP‘’“”]"
,
""
));
try
{
context.write(
new
Text(
""
),
new
Text(line));
System.out.println(line);
}
catch
(InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
protected
void
cleanup(Context context){
tt.unInit();
}
}
public
static
class
SegWordsReduce
extends
Reducer<Text, Text, Text, Text> {
public
void
reduce(Text key, Text value, Context context)
throws
IOException, NumberFormatException, InterruptedException {
context.write(key, value);
//
}
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
|
package
segwords.rf.cs.swjtu;
import
java.io.IOException;
import
org.apache.hadoop.conf.Configuration;
import
org.apache.hadoop.fs.FileSystem;
import
org.apache.hadoop.fs.Path;
import
org.apache.hadoop.io.Text;
import
org.apache.hadoop.mapreduce.Job;
import
org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import
org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import
segwords.rf.cs.swjtu.SegWords.SegWordsMap;
import
segwords.rf.cs.swjtu.SegWords.SegWordsReduce;
public
class
tcMain {
/**
* @param args
* @throws IOException
* @throws InterruptedException
* @throws ClassNotFoundException
*/
public
static
void
main(String[] args)
throws
IOException, ClassNotFoundException, InterruptedException {
// TODO Auto-generated method stub
//JOB 1 TF
Path segIn =
new
Path(
"hdfs://localhost:9000/input/segIn"
);
Path segOut =
new
Path(
"hdfs://localhost:9000/output/segOut"
);
Configuration conf1=
new
Configuration();
Job job1=
new
Job(conf1,
"segWords"
);
FileSystem fs1 = FileSystem.get(conf1);
if
(fs1.exists(segOut)){
fs1.delete(segOut);
}
fs1.close();
job1.setJarByClass(SegWords.
class
);
job1.setMapperClass( SegWordsMap.
class
);
job1.setReducerClass( SegWordsReduce.
class
);
job1.setOutputKeyClass(Text.
class
);
job1.setOutputValueClass(Text.
class
);
FileInputFormat.addInputPath(job1, segIn);
FileOutputFormat.setOutputPath(job1, segOut);
//System.exit(job1.waitForCompletion(true)?0:1);
job1.waitForCompletion(
true
);
}
}
|
5、并行分词结果
1)输入文档(2个文档模拟2个split)
2)结果
15/10/28 01:07:12 WARN util.NativeCodeLoader: Unable to load native-hadoop library for your platform... using builtin-java classes where applicable
15/10/28 01:07:16 INFO Configuration.deprecation: session.id is deprecated. Instead, use dfs.metrics.session-id
15/10/28 01:07:16 INFO jvm.JvmMetrics: Initializing JVM Metrics with processName=JobTracker, sessionId=
15/10/28 01:07:16 WARN mapreduce.JobSubmitter: Hadoop command-line option parsing not performed. Implement the Tool interface and execute your application with ToolRunner to remedy this.
15/10/28 01:07:18 INFO input.FileInputFormat: Total input paths to process : 2
15/10/28 01:07:18 INFO mapreduce.JobSubmitter: number of splits:2
15/10/28 01:07:18 INFO mapreduce.JobSubmitter: Submitting tokens for job: job_local598241144_0001
15/10/28 01:07:19 INFO mapreduce.Job: The url to track the job: http://localhost:8080/
15/10/28 01:07:19 INFO mapreduce.Job: Running job: job_local598241144_0001
15/10/28 01:07:19 INFO mapred.LocalJobRunner: OutputCommitter set in config null
15/10/28 01:07:19 INFO mapred.LocalJobRunner: OutputCommitter is org.apache.hadoop.mapreduce.lib.output.FileOutputCommitter
15/10/28 01:07:19 INFO mapred.LocalJobRunner: Waiting for map tasks
15/10/28 01:07:19 INFO mapred.LocalJobRunner: Starting task: attempt_local598241144_0001_m_000000_0
15/10/28 01:07:19 INFO mapred.Task: Using ResourceCalculatorProcessTree : [ ]
15/10/28 01:07:20 INFO mapreduce.Job: Job job_local598241144_0001 running in uber mode : false
15/10/28 01:07:20 INFO mapreduce.Job: map 0% reduce 0%
15/10/28 01:07:20 INFO mapred.MapTask: Processing split: hdfs://localhost:9000/input/segIn/segtest01:0+2359
15/10/28 01:07:23 INFO mapred.MapTask: (EQUATOR) 0 kvi 26214396(104857584)
15/10/28 01:07:23 INFO mapred.MapTask: mapreduce.task.io.sort.mb: 100
15/10/28 01:07:23 INFO mapred.MapTask: soft limit at 83886080
15/10/28 01:07:23 INFO mapred.MapTask: bufstart = 0; bufvoid = 104857600
15/10/28 01:07:23 INFO mapred.MapTask: kvstart = 26214396; length = 6553600
15/10/28 01:07:23 INFO mapred.MapTask: Map output collector class = org.apache.hadoop.mapred.MapTask$MapOutputBuffer
15/10/28 01:07:40 INFO mapred.LocalJobRunner:
联合早报网 综合 讯 据 中新网 报道 中国 各地 2015年 前 三 季度 居民 收入 数据 近日 陆续 出炉 截至 10月 26日 中国 至少 已 有 25 省份 公布 数据 仅 上海 北京 全体 居民 人均 可 支配 收入 超过 3万 元 大关 而 城乡 差距 有 继续 扩大 的 趋势
据 中国 国家 统计局 数据 前 三 季度 全国 居民 人均 可 支配 收入 16367 元 按 常住 地 分 城镇 居民 人均 可 支配 收入 23512 元 扣除 价格 因素 实际 增长 68 农村 居民 人均 可 支配 收入 8297 元 扣除 价格 因素 实际 增长 81
据 报道 截至 10月 26日 除了 吉林 辽宁 黑龙江 内蒙古 山西 西藏 外 其余 25 省份 均 已 公布 了 当地 的 前 三 季度 居民 人均 可 支配 收入 数据
在 全体 居民 人均 可 支配 收入 方面 位居 前 两 位 的 为 上海 和 北京 其 人均收入 均 突破 3万 元 其中 上海 前 三 季度 居民 人均 可 支配 收入 为 37568 元 北京 前 三 季度 居民 人均 可 支配 收入 为 36047 元 这 两 地 也 是 目前 全国 仅 有 的 居民 人均 可 支配 收入 超过 3万 元 大关 的 地区
如果 单 单 从 在 城镇 居民 收入 方面 在 已 公布 数据 的 25 个 地区 中 上海 城镇 居民 人均 可 支配 收入 最高 达到 了 39686 元 北京 排 第二 为 39142 元 浙江 位列 第三 为 33464 元 这 三 地 也 是 目前 全国 仅 有 的 城镇 居民 人均 可 支配 收入 突破 3万 元 大关 的 地区 而 城镇 居民 人均 可 支配 收入 暂 排 在 末 位 是 甘肃省 仅 为 176385 元 收入 最高 和 最低 地区 的 差异 超 2万 元
据 统计 上海 北京 浙江 江苏 广东 天津 福建 山东 这 8 个 地区 的 城镇 居民 人均 可 支配 收入 超过 了 全国 平均 水平 而 在 农村 居民 收入 方面 上海 浙江 北京 天津 江苏 山东 辽宁 福建 海南 河北 这 10 个 地区 的 农村 居民 人均 可 支配 收入 超过 了 全国 平均 水平
在 已 公布 数据 的 25 个 地区 中 上海 农村 居民 人均 可 支配 收入 最高 达到 了 19237 元 浙江 排 第二 为 17004 元 北京 位 列 第三 为 16450 元 但 从 城乡 居民 人均收入 倍 差 来 看 与 全国 城乡 居民 人均收入 倍 差 283 相比 新疆 陕西 广西 云南 贵州 宁夏 青海 甘肃 这 8 地区 的 城乡 居民 人均收入 倍 差 超过 了 全国 水平
联合早报网 实习 编辑 关丽明
15/10/28 01:07:41 INFO mapred.MapTask: Starting flush of map output
15/10/28 01:07:41 INFO mapred.MapTask: Spilling map output
15/10/28 01:07:41 INFO mapred.MapTask: bufstart = 0; bufend = 2552; bufvoid = 104857600
15/10/28 01:07:41 INFO mapred.MapTask: kvstart = 26214396(104857584); kvend = 26214368(104857472); length = 29/6553600
15/10/28 01:07:41 INFO mapred.MapTask: Finished spill 0
15/10/28 01:07:41 INFO mapred.Task: Task:attempt_local598241144_0001_m_000000_0 is done. And is in the process of committing
15/10/28 01:07:42 INFO mapred.LocalJobRunner: map
15/10/28 01:07:42 INFO mapred.Task: Task 'attempt_local598241144_0001_m_000000_0' done.
15/10/28 01:07:42 INFO mapred.LocalJobRunner: Finishing task: attempt_local598241144_0001_m_000000_0
15/10/28 01:07:42 INFO mapred.LocalJobRunner: Starting task: attempt_local598241144_0001_m_000001_0
15/10/28 01:07:42 INFO mapred.Task: Using ResourceCalculatorProcessTree : [ ]
15/10/28 01:07:42 INFO mapreduce.Job: map 100% reduce 0%
15/10/28 01:07:42 INFO mapred.MapTask: Processing split: hdfs://localhost:9000/input/segIn/segtest00:0+2314
15/10/28 01:07:42 INFO mapred.MapTask: (EQUATOR) 0 kvi 26214396(104857584)
15/10/28 01:07:42 INFO mapred.MapTask: mapreduce.task.io.sort.mb: 100
15/10/28 01:07:42 INFO mapred.MapTask: soft limit at 83886080
15/10/28 01:07:42 INFO mapred.MapTask: bufstart = 0; bufvoid = 104857600
15/10/28 01:07:42 INFO mapred.MapTask: kvstart = 26214396; length = 6553600
15/10/28 01:07:42 INFO mapred.MapTask: Map output collector class = org.apache.hadoop.mapred.MapTask$MapOutputBuffer
联合早报网 讯 中国 外交部 证实 美国 军舰 今天 进入 南沙 群岛 渚 碧 礁 12 海里 航行 中 国 外交部 发言人 陆 慷 说 今天 美国 拉森 号 军舰 USS Lassen 未经 中国政府 允许 非法 进入 中国 南沙 群岛 有关 岛礁 邻近 海域 中国 有关 部门 对 美国 舰艇 实施 了 监视 跟踪 和 警告
陆 慷 表示 美方 军舰 有关 行为 威胁 中国 主权 和 安全 利益 危及 岛礁 人员 及 设施 安全 损害 地区 和平 稳定 中方 对 此 表示 强烈 不满 和 坚决 反对
陆 慷 说 正 如 中方 多次 强调 的 中国 对 南沙 群岛 及其 附近 海域 拥有 无可争辩 的 主权 中国 在 南海 的 主权 和 相关 权利 是 在 长期 的 历史 过程 中 形成 的 为 历代 中国政府 所 坚持 中方 在 自己 的 领土 上 开展 建设 是 主权 范围 内 的 事 不 针对 不 影响 任何 国家 不 会 对 各国 依 国际法 在 南海 享有 的 航行 和 飞越 自由 造成 任何 影响
他 强调 中方 强烈 敦促 美方 认真 对待 中方 严正 交涉 立即 纠正 错误 不得 采取 任何 威胁 中方 主权 和 安全 利益 的 危险 挑衅 行为 恪守 在 领土 主权 争议 问题 上 不 持 立场 的 承诺 以免 进一步 损害 中 美 关系 和 地区 和平 稳定
据 早 前 报道 中国 外交部长 王毅 今天 在 北京 出席 中 日 韩 研讨会 期间 针对 有 境外 媒体 问及 美军 将 派遣 军舰 进入 中国 南海 岛礁 12 海里 一 事 表示 关于 此事 中方 正在 核实 当中 如果 属实 奉劝 美方 三思而后行 不要 轻举妄动 不要 无事生非
据 报道 美国 国防部 周一 26日 宣布 将 于 24 小时 内 派遣 导弹 驱逐舰 到 中国 在 南海 人工岛 礁 12 海里 水域 的 范围 内 航行
路透社 报道 美军 将 派出 拉森 号 导弹 驱逐舰 前往 渚 碧 礁 及 美 济 礁 一带 水域 航行 并 会同 时 派出 P8A 侦察机 到 该 海域 巡逻 美国 国防部 发言人 表示 美军 的 行动 不 会 是 一次性 的 而 会 定期 进行 这次 行动 并非 针对 中国
美国 国防部 一 名 官员 之后 证实 美国 海军 派出 的 导弹 驱逐舰 拉森 号 周二 早上 驶 到 中国 在 南中国海 兴建 的 多 个 人工 岛礁 12 海 浬 范围 并 逗留 数 小时 美军 之后 表示 行动 已经 完成 拉森 号 离开 无 发生 任何 事故
联合早报网 编辑 沈茂华
15/10/28 01:07:51 INFO mapred.LocalJobRunner:
15/10/28 01:07:51 INFO mapred.MapTask: Starting flush of map output
15/10/28 01:07:51 INFO mapred.MapTask: Spilling map output
15/10/28 01:07:51 INFO mapred.MapTask: bufstart = 0; bufend = 2534; bufvoid = 104857600
15/10/28 01:07:51 INFO mapred.MapTask: kvstart = 26214396(104857584); kvend = 26214364(104857456); length = 33/6553600
15/10/28 01:07:51 INFO mapred.MapTask: Finished spill 0
15/10/28 01:07:51 INFO mapred.Task: Task:attempt_local598241144_0001_m_000001_0 is done. And is in the process of committing
15/10/28 01:07:51 INFO mapred.LocalJobRunner: map
15/10/28 01:07:51 INFO mapred.Task: Task 'attempt_local598241144_0001_m_000001_0' done.
15/10/28 01:07:51 INFO mapred.LocalJobRunner: Finishing task: attempt_local598241144_0001_m_000001_0
15/10/28 01:07:51 INFO mapred.LocalJobRunner: map task executor complete.
15/10/28 01:07:52 INFO mapred.LocalJobRunner: Waiting for reduce tasks
15/10/28 01:07:52 INFO mapred.LocalJobRunner: Starting task: attempt_local598241144_0001_r_000000_0
15/10/28 01:07:52 INFO mapred.Task: Using ResourceCalculatorProcessTree : [ ]
15/10/28 01:07:52 INFO mapred.ReduceTask: Using ShuffleConsumerPlugin: org.apache.hadoop.mapreduce.task.reduce.Shuffle@1f0dbfd
15/10/28 01:07:53 INFO reduce.MergeManagerImpl: MergerManager: memoryLimit=178821520, maxSingleShuffleLimit=44705380, mergeThreshold=118022208, ioSortFactor=10, memToMemMergeOutputsThreshold=10
15/10/28 01:07:53 INFO reduce.EventFetcher: attempt_local598241144_0001_r_000000_0 Thread started: EventFetcher for fetching Map Completion Events
15/10/28 01:07:54 INFO reduce.LocalFetcher: localfetcher#1 about to shuffle output of map attempt_local598241144_0001_m_000001_0 decomp: 2568 len: 2572 to MEMORY
15/10/28 01:07:54 INFO reduce.InMemoryMapOutput: Read 2568 bytes from map-output for attempt_local598241144_0001_m_000001_0
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: closeInMemoryFile -> map-output of size: 2568, inMemoryMapOutputs.size() -> 1, commitMemory -> 0, usedMemory ->2568
15/10/28 01:07:54 INFO reduce.LocalFetcher: localfetcher#1 about to shuffle output of map attempt_local598241144_0001_m_000000_0 decomp: 2583 len: 2587 to MEMORY
15/10/28 01:07:54 INFO reduce.InMemoryMapOutput: Read 2583 bytes from map-output for attempt_local598241144_0001_m_000000_0
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: closeInMemoryFile -> map-output of size: 2583, inMemoryMapOutputs.size() -> 2, commitMemory -> 2568, usedMemory ->5151
15/10/28 01:07:54 INFO reduce.EventFetcher: EventFetcher is interrupted.. Returning
15/10/28 01:07:54 INFO mapred.LocalJobRunner: 2 / 2 copied.
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: finalMerge called with 2 in-memory map-outputs and 0 on-disk map-outputs
15/10/28 01:07:54 INFO mapred.Merger: Merging 2 sorted segments
15/10/28 01:07:54 INFO mapred.Merger: Down to the last merge-pass, with 2 segments left of total size: 5145 bytes
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: Merged 2 segments, 5151 bytes to disk to satisfy reduce memory limit
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: Merging 1 files, 5153 bytes from disk
15/10/28 01:07:54 INFO reduce.MergeManagerImpl: Merging 0 segments, 0 bytes from memory into reduce
15/10/28 01:07:54 INFO mapred.Merger: Merging 1 sorted segments
15/10/28 01:07:54 INFO mapred.Merger: Down to the last merge-pass, with 1 segments left of total size: 5146 bytes
15/10/28 01:07:55 INFO mapred.LocalJobRunner: 2 / 2 copied.
15/10/28 01:07:57 INFO Configuration.deprecation: mapred.skip.on is deprecated. Instead, use mapreduce.job.skiprecords
15/10/28 01:07:58 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:07:59 INFO mapreduce.Job: map 100% reduce 100%
15/10/28 01:08:01 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:08:05 INFO mapred.Task: Task:attempt_local598241144_0001_r_000000_0 is done. And is in the process of committing
15/10/28 01:08:05 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:08:05 INFO mapred.Task: Task attempt_local598241144_0001_r_000000_0 is allowed to commit now
15/10/28 01:08:05 INFO output.FileOutputCommitter: Saved output of task 'attempt_local598241144_0001_r_000000_0' to hdfs://localhost:9000/output/segOut/_temporary/0/task_local598241144_0001_r_000000
15/10/28 01:08:05 INFO mapred.LocalJobRunner: reduce > reduce
15/10/28 01:08:05 INFO mapred.Task: Task 'attempt_local598241144_0001_r_000000_0' done.
15/10/28 01:08:05 INFO mapred.LocalJobRunner: Finishing task: attempt_local598241144_0001_r_000000_0
15/10/28 01:08:05 INFO mapred.LocalJobRunner: reduce task executor complete.
15/10/28 01:08:07 INFO mapreduce.Job: Job job_local598241144_0001 completed successfully
15/10/28 01:08:07 INFO mapreduce.Job: Counters: 38
File System Counters
FILE: Number of bytes read=33097684
FILE: Number of bytes written=34155860
FILE: Number of read operations=0
FILE: Number of large read operations=0
FILE: Number of write operations=0
HDFS: Number of bytes read=11705
HDFS: Number of bytes written=5059
HDFS: Number of read operations=28
HDFS: Number of large read operations=0
HDFS: Number of write operations=8
Map-Reduce Framework
Map input records=17
Map output records=17
Map output bytes=5086
Map output materialized bytes=5159
Input split bytes=216
Combine input records=0
Combine output records=0
Reduce input groups=1
Reduce shuffle bytes=5159
Reduce input records=17
Reduce output records=17
Spilled Records=34
Shuffled Maps =2
Failed Shuffles=0
Merged Map outputs=2
GC time elapsed (ms)=179
CPU time spent (ms)=0
Physical memory (bytes) snapshot=0
Virtual memory (bytes) snapshot=0
Total committed heap usage (bytes)=457125888
Shuffle Errors
BAD_ID=0
CONNECTION=0
IO_ERROR=0
WRONG_LENGTH=0
WRONG_MAP=0
WRONG_REDUCE=0
File Input Format Counters
Bytes Read=4673
File Output Format Counters
Bytes Written=5059
http://blog.csdn.net/luoyhang003/article/details/44586731
http://jingyan.baidu.com/article/9158e0004251b4a2541228e5.html