配置类
package com.zz.config;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
@Configuration
public class WebAppConfig extends WebMvcConfigurerAdapter {
@Value("${imagesPath}")
private String mImagesPath;
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
if(mImagesPath.equals("") || mImagesPath.equals("${imagesPath}")){
String imagesPath = WebAppConfig.class.getClassLoader().getResource("").getPath();
if(imagesPath.indexOf(".jar")>0){
imagesPath = imagesPath.substring(0, imagesPath.indexOf(".jar"));
}else if(imagesPath.indexOf("classes")>0){
imagesPath = "file:"+imagesPath.substring(0, imagesPath.indexOf("classes"));
}
imagesPath = imagesPath.substring(0, imagesPath.lastIndexOf("/"))+"/images/";
mImagesPath = imagesPath;
}
//LoggerFactory.getLogger(WebAppConfig.class).info("imagesPath="+mImagesPath);
registry.addResourceHandler("/images/**").addResourceLocations(mImagesPath);
// TODO Auto-generated method stub
super.addResourceHandlers(registry);
}
}
配置文件设置外面路径地址
application.properties
## 图片上传真是地址
imagesPath=file:/C:/upload/
测试 访问图片路径: http://localhost:9001/core/images/2.jpg
图片实际存放路径:C:\upload
SpringBoot2.X
Springboot2 里面WebMvcConfigurerAdapter 已经过时。
推荐使用接口 WebMvcConfigurer 。
package com.cy.config;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebAppConfig implements WebMvcConfigurer {
@Value("${imagesPath}")
private String mImagesPath;
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
if(mImagesPath.equals("") || mImagesPath.equals("${imagesPath}")){
String imagesPath = WebAppConfig.class.getClassLoader().getResource("").getPath();
if(imagesPath.indexOf(".jar")>0){
imagesPath = imagesPath.substring(0, imagesPath.indexOf(".jar"));
}else if(imagesPath.indexOf("classes")>0){
imagesPath = "file:"+imagesPath.substring(0, imagesPath.indexOf("classes"));
}
imagesPath = imagesPath.substring(0, imagesPath.lastIndexOf("/"))+"/images/";
mImagesPath = imagesPath;
}
//LoggerFactory.getLogger(WebAppConfig.class).info("imagesPath="+mImagesPath);
registry.addResourceHandler("/images/**").addResourceLocations(mImagesPath);
// TODO Auto-generated method stub
}
}
跨域问题CORS 统一处理
package com.zz.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
/**
* @Description: java类作用描述
* @Author: Bsea
* @CreateDate: 2019/10/9$ 21:33$
*/
@Configuration
public class CorsConfig {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*");//允许域名访问,如果*,代表所有域名
}
};
}
}
错误日志:
:8080/#/pages/login/login:1 Access to XMLHttpRequest at ‘http://localhost:5557/xsz/api/user/login’ from origin ‘http://localhost:8080’ has been blocked by CORS policy: Request header field authorization is not allowed by Access-Control-Allow-Headers in preflight response.
解决办法
```java
package com.zz.util;
import org.springframework.context.annotation.Configuration;
import javax.servlet.*;
import javax.servlet.annotation.WebFilter;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebFilter(filterName = "cors", urlPatterns = "/*")
@Configuration
public class CORSFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) throws ServletException {
}
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest request = (HttpServletRequest) req;
response.setHeader("Access-Control-Allow-Origin", request.getHeader("Origin"));
response.setHeader("Access-Control-Allow-Methods", "POST, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Headers", "*");
response.setHeader("Access-Control-Allow-Headers", "Content-Type,Content-Length, Authorization, Accept,X-Requested-With,token");
response.setHeader("Access-Control-Allow-Credentials", "true");
if ("OPTIONS".equals(request.getMethod())) {
res.getWriter().println("ok");
response.setStatus( 200 );
return;
}
chain.doFilter(req, res);
}
@Override
public void destroy() {
}
}
## 关注公众号,可以获取学习资料和源码
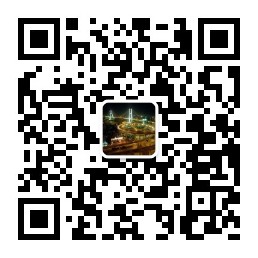