一、基础语法体系
1.1 模板语法
<template>
<!-- 文本插值 -->
<div>{{ message }}</div>
<!-- 原始HTML -->
<div v-html="rawHtml"></div>
<!-- 属性绑定 -->
<img :src="imageSrc" :alt="imgAlt">
<!-- 条件渲染 -->
<div v-if="isVisible">可见内容</div>
<div v-else-if="otherCondition">其他条件</div>
<div v-else>默认内容</div>
<!-- 列表渲染 -->
<ul>
<li v-for="(item, index) in items" :key="item.id">
{{ index }}. {{ item.name }}
</li>
</ul>
</template>
1.2 指令系统
指令 | 说明 | 示例 |
---|
v-model | 双向绑定 | <input v-model="text"> |
v-show | 显示/隐藏 | <div v-show="isShow"> |
v-on | 事件监听 | <button @click="handleClick"> |
v-bind | 属性绑定 | <div :class="{ active: isActive }"> |
v-slot | 插槽定义 | <template v-slot:header> |
v-memo | 性能优化 | <div v-memo="[valueA, valueB]"> |
二、响应式系统深度解析
2.1 响应式API对比
import { ref, reactive, computed, watch } from 'vue'
// 基本类型响应式
const count = ref(0)
// 对象类型响应式
const state = reactive({
user: {
name: 'John',
age: 30
}
})
// 计算属性
const doubleCount = computed(() => count.value * 2)
// 侦听器
watch(
() => state.user.age,
(newVal, oldVal) => {
console.log(`年龄变化: ${oldVal} → ${newVal}`)
},
{ deep: true }
)
2.2 响应式工具函数
方法 | 作用 | 示例 |
---|
toRef | 保持响应式引用 | const nameRef = toRef(state.user, 'name') |
toRefs | 解构响应式对象 | const { user } = toRefs(state) |
isRef | 检查ref对象 | isRef(count) // true |
unref | 获取ref值 | const raw = unref(count) |
三、组件生命周期全解
3.1 生命周期图示
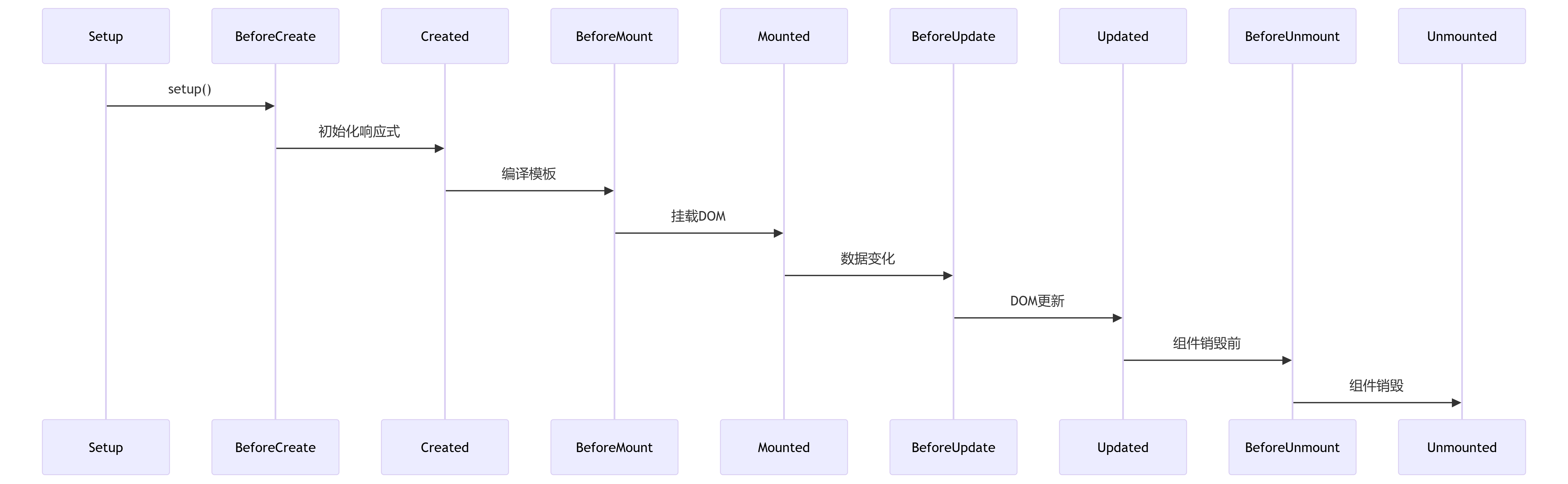
3.2 Composition API生命周期
<script setup>
import {
onBeforeMount,
onMounted,
onBeforeUpdate,
onUpdated,
onBeforeUnmount,
onUnmounted
} from 'vue'
onBeforeMount(() => {
console.log('挂载前')
})
onMounted(() => {
console.log('挂载完成')
})
onBeforeUpdate(() => {
console.log('更新前')
})
onUpdated(() => {
console.log('更新完成')
})
onBeforeUnmount(() => {
console.log('卸载前')
})
onUnmounted(() => {
console.log('卸载完成')
})
</script>
四、组合式API进阶
4.1 自定义Hook示例
// useFetch.js
import { ref, onMounted } from 'vue'
export function useFetch(url) {
const data = ref(null)
const error = ref(null)
const loading = ref(false)
const fetchData = async () => {
try {
loading.value = true
const response = await fetch(url)
data.value = await response.json()
} catch (err) {
error.value = err
} finally {
loading.value = false
}
}
onMounted(fetchData)
return { data, error, loading, retry: fetchData }
}
4.2 依赖注入系统
// 提供者组件
import { provide, ref } from 'vue'
const theme = ref('dark')
provide('theme', theme)
// 消费者组件
import { inject } from 'vue'
const theme = inject('theme', 'light') // 默认值
五、高级组件开发
5.1 动态组件与异步组件
<template>
<component :is="currentComponent" />
<Suspense>
<template #default>
<AsyncComponent />
</template>
<template #fallback>
<div>Loading...</div>
</template>
</Suspense>
</template>
<script setup>
import { defineAsyncComponent } from 'vue'
const AsyncComponent = defineAsyncComponent(() =>
import('./components/AsyncComponent.vue')
)
</script>
5.2 Render函数与JSX
import { h } from 'vue'
export default {
render() {
return h(
'button',
{
onClick: this.handleClick,
class: 'btn-primary'
},
this.$slots.default()
)
}
}
// JSX版本
export default {
setup() {
return () => (
<button class="btn-primary" onClick={handleClick}>
{ctx.slots.default()}
</button>
)
}
}
六、TypeScript集成
6.1 类型定义示例
// 组件Props类型
interface Props {
title: string
count?: number
items: string[]
}
const props = defineProps<Props>()
// 自定义事件类型
const emit = defineEmits<{
(e: 'update', id: number): void
(e: 'delete'): void
}>()
// 模板Ref类型
const inputRef = ref<HTMLInputElement | null>(null)
七、性能优化策略
7.1 优化技巧对比
技术 | 应用场景 | 实现方式 |
---|
v-once | 静态内容 | <div v-once>{{ staticText }}</div> |
v-memo | 复杂计算 | <div v-memo="[valueA, valueB]"> |
懒加载 | 路由/组件 | () => import('./HeavyComponent.vue') |
虚拟列表 | 大数据量 | 使用vue-virtual-scroller库 |
八、常见问题解决方案
8.1 响应式丢失问题
// 错误示例
const state = reactive({ ... })
const { foo } = state // 失去响应式
// 正确解法
const { foo } = toRefs(state)
8.2 循环引用处理
// 使用shallowRef处理大对象
const bigData = shallowRef(largeObject)
// 使用markRaw跳过代理
const rawObj = markRaw(nonReactiveObject)
九、生态系统整合
9.1 官方工具链
工具 | 用途 | 命令示例 |
---|
Vite | 构建工具 | npm create vite@latest |
Pinia | 状态管理 | npm install pinia |
Vue Router | 路由管理 | npm install vue-router@4 |
Vue Test Utils | 单元测试 | npm install @vue/test-utils@next |
十、学习路线图
