作者主页:夜未央5788
简介:Java领域优质创作者、Java项目、学习资料、技术互助
文末获取源码
项目介绍
适合刚接触springboot的同学学习一下的,难度不大,前端使用layui框架,后台springboot+mybatis。代码量较少。 角色分为 学生、教师、管理员,学生可修改密码,先选择课程之后对对应课程的教师做出评价,最后计算出总分入库;
管理员角色包含以下功能:登录,管理员管理,教师管理增删改查,课程管理增删改查,教学指标增删改查,学生增删改查,查看评价等功能。
教师角色包含以下功能:登录,课程管理,教学指标管理,学生管理,收到的评价等功能。
学生角色包含以下功能:登录,修改个人信息,开始评分,选择课程进行评价,查看评分,打分等功能。
环境需要
1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。
2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA;
3.tomcat环境:Tomcat 7.x,8.x,9.x版本均可
4.硬件环境:windows 7/8/10 1G内存以上;或者 Mac OS;
5.是否Maven项目: 是;查看源码目录中是否包含pom.xml;若包含,则为maven项目,否则为非maven项目
6.数据库:MySql 5.7版本;
技术栈
1. 后端:SpringBoot+MyBatis
2. 前端:layui+html
使用说明
1. 使用Navicat或者其它工具,在mysql中创建对应名称的数据库,并导入项目的sql文件;
2. 将项目中application.properties配置文件中的数据库配置改为自己的配置
3. 使用IDEA/Eclipse/MyEclipse导入项目,Eclipse/MyEclipse导入时,若为maven项目请选择maven;
若为maven项目,导入成功后请执行maven clean;maven install命令,配置tomcat,然后运行;
4. 运行项目,输入localhost:8085 登录
5. 管理员账户:admin 密码:123456
教师账户:jiaoshi 密码:123456
学生账户:student 密码:123456
运行截图 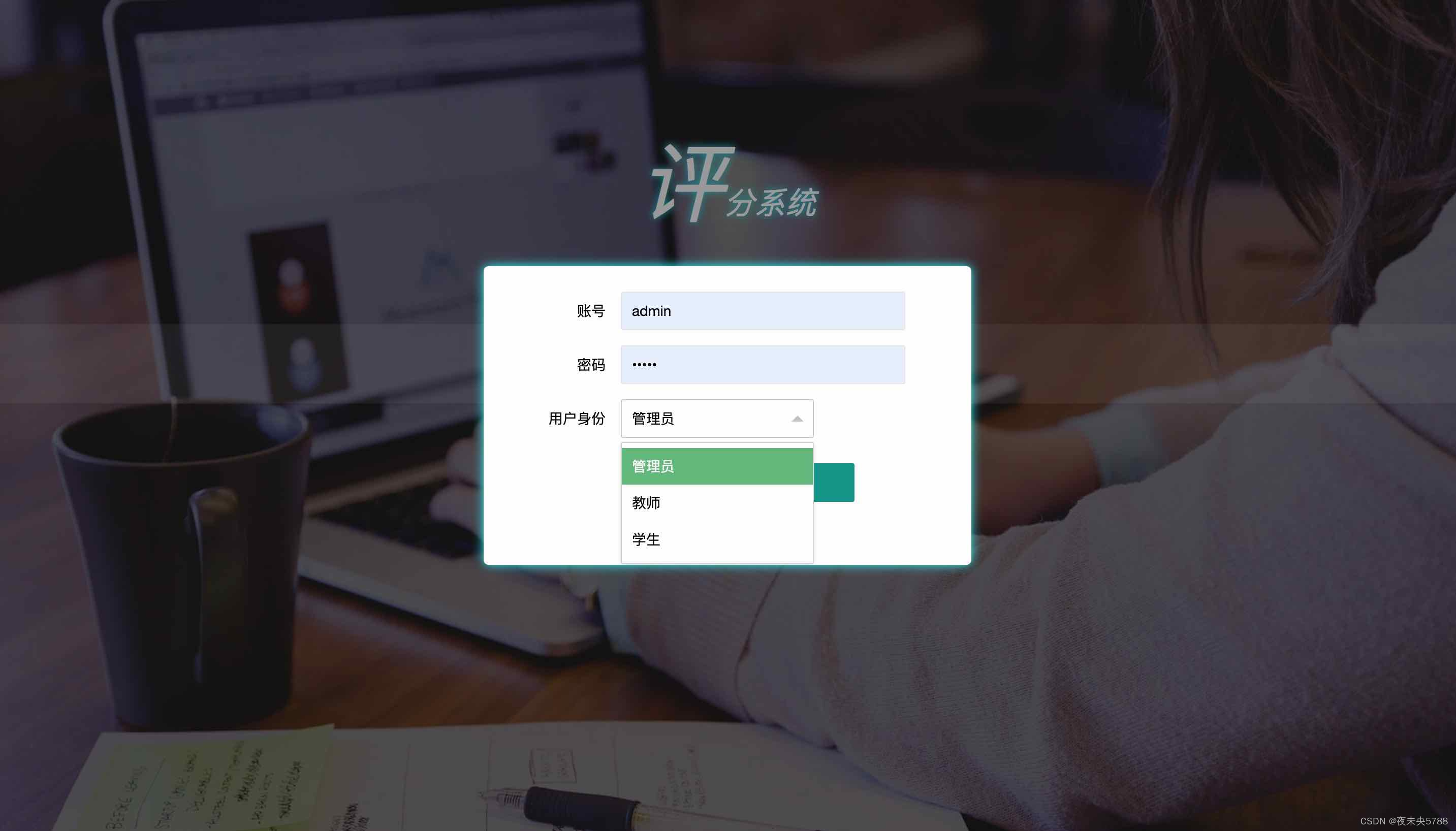
代码相关
管理端控制器
@RestController
@RequestMapping("/admin")
public class AdminController {
@Autowired
private AdminEntityMapper adminEntityMapper;
@PostMapping("/add")
public Integer add(@RequestBody AdminEntity entity) {
return adminEntityMapper.insert(entity);
}
@PostMapping(value = "/delete", consumes = "application/json")
public Integer delete(@RequestBody AdminEntity entity) {
return adminEntityMapper.deleteByPrimaryKey(entity.getUserid());
}
@PostMapping("/update")
public Integer update(@RequestBody AdminEntity entity) {
return adminEntityMapper.updateByPrimaryKey(entity);
}
@RequestMapping("/select")
public Layui select(@RequestParam(required = false) String username,@RequestParam(value = "page")Integer page,
@RequestParam(value = "limit")Integer limit) {
AdminEntityExample example = new AdminEntityExample();
if (!StringUtils.isEmpty(username)){
example.or().andUsernameLike("%"+username+"%");
}
example.getOrderByClause();
Long cou = adminEntityMapper.countByExample(example);
return Layui.data(cou.intValue(), adminEntityMapper.selectByExamplePaging(example, page-1, limit));
}
@RequestMapping("/getAdmin")
public AdminEntity getAdmin(@RequestParam(value="userid")Integer userid) {
return adminEntityMapper.selectByPrimaryKey(userid);
}
}
学生管理控制器
@RestController
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentEntityMapper studentEntityMapper;
@PostMapping("/add")
public Integer add(@RequestBody StudentEntity entity) {
return studentEntityMapper.insert(entity);
}
@PostMapping("/delete")
public Integer delete(@RequestBody StudentEntity entity) {
return studentEntityMapper.deleteByPrimaryKey(entity.getStuId());
}
@PostMapping("/update")
public Integer update(@RequestBody StudentEntity entity) {
return studentEntityMapper.updateByPrimaryKey(entity);
}
@RequestMapping("/select")
public Layui select(@RequestParam(required = false) String stuRealname, @RequestParam(value = "page") Integer page,
@RequestParam(value = "limit") Integer limit) {
StudentEntityExample example = new StudentEntityExample();
if (!StringUtils.isEmpty(stuRealname)){
example.or().andStuRealnameLike("%"+stuRealname+"%");
}
Long cou = studentEntityMapper.countByExample(example);
return Layui.data(cou.intValue(), studentEntityMapper.selectByExamplePaging(example, page - 1, limit));
}
@RequestMapping("/getStudent")
public StudentEntity getAdmin(@RequestParam(value="stuId")Integer stuId) {
return studentEntityMapper.selectByPrimaryKey(stuId);
}
}
教师管理控制器
@RestController
@RequestMapping("/teacher")
public class TeacherController {
@Autowired
TeacherEntityMapper teacherEntityMapper;
@Autowired
PingjiaxinxiEntityMapper pingjiaxinxiEntityMapper;
@Autowired
CourseTeaEntityMapper courseTeaEntityMapper;
@PostMapping("/add")
public Integer add(@RequestBody TeacherDTO entity) {
List<CourseTeaEntity> teaEntities = new ArrayList<>();
for (int i = 0; i < entity.getCourseIds().length; i++) {
CourseTeaEntity courseTeaEntity = new CourseTeaEntity();
courseTeaEntity.setCourseId(entity.getCourseIds()[i]);
courseTeaEntity.setTeacherId(entity.getTeaId());
teaEntities.add(courseTeaEntity);
}
if (teaEntities.size() > 0) {
courseTeaEntityMapper.insertBatch(teaEntities);
}
return teacherEntityMapper.insert(entity);
}
@PostMapping("/delete")
public Integer delete(@RequestBody TeacherEntity entity) {
return teacherEntityMapper.deleteByPrimaryKey(entity.getTeaId());
}
@PostMapping("/update")
public Integer update(@RequestBody TeacherDTO entity) {
CourseTeaEntityExample example = new CourseTeaEntityExample();
example.or().andTeacherIdEqualTo(entity.getTeaId());
//先清空后插入
courseTeaEntityMapper.deleteByExample(example);
List<CourseTeaEntity> teaEntities = new ArrayList<>();
for (int i = 0; i < entity.getCourseIds().length; i++) {
CourseTeaEntity courseTeaEntity = new CourseTeaEntity();
courseTeaEntity.setCourseId(entity.getCourseIds()[i]);
courseTeaEntity.setTeacherId(entity.getTeaId());
teaEntities.add(courseTeaEntity);
}
if (teaEntities.size() > 0) {
courseTeaEntityMapper.insertBatch(teaEntities);
}
return teacherEntityMapper.updateByPrimaryKey(entity);
}
@RequestMapping("/select")
public Layui select(@RequestParam(required = false) String teaRealname, @RequestParam(value = "page") Integer page,
@RequestParam(value = "limit") Integer limit) {
TeacherEntityExample example = new TeacherEntityExample();
if (!StringUtils.isEmpty(teaRealname)) {
example.or().andTeaRealnameLike("%"+teaRealname+"%");
}
Long cou = teacherEntityMapper.countByExample(example);
return Layui.data(cou.intValue(), teacherEntityMapper.selectByExamplePaging(example, page - 1, limit));
}
@RequestMapping("/getTeacher")
public TeacherDTO getAdmin(@RequestParam(value = "teaId") Integer teaId) {
TeacherEntity entity = teacherEntityMapper.selectByPrimaryKey(teaId);
CourseTeaEntityExample example = new CourseTeaEntityExample();
example.or().andTeacherIdEqualTo(entity.getTeaId());
List<CourseTeaEntity> courseTeaEntities = courseTeaEntityMapper.selectByExample(example);
Integer[] arr = new Integer[courseTeaEntities.size()];
for (int i = 0; i < courseTeaEntities.size(); i++) {
arr[i] = courseTeaEntities.get(i).getCourseId();
}
TeacherDTO dto = new TeacherDTO();
dto.setCourseIds(arr);
dto.setLoginName(entity.getLoginName());
dto.setLoginPw(entity.getLoginPw());
dto.setTeaId(entity.getTeaId());
dto.setDel(entity.getDel());
dto.setTeaAge(entity.getTeaAge());
dto.setTeaBianhao(entity.getTeaBianhao());
dto.setTeaRealname(entity.getTeaRealname());
dto.setTeaSex(entity.getTeaSex());
return dto;
}
/**
* 若存在--提示已经评论过
*
* @param teaId
* @return
*/
@RequestMapping("/exit")
public Boolean exit(@RequestParam(value = "teaId") Integer teaId) {
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.getRequestAttributes()).getRequest();
HttpSession session = request.getSession();
//学生id
Integer stuId = Integer.parseInt(session.getAttribute("loginUserId").toString());
String loginType = session.getAttribute("loginUserType").toString();
if (!StringUtils.endsWithIgnoreCase(UserTypeEnum.STUDENT.getCode(), loginType)) {
return true;
}
PingjiaxinxiEntityExample example = new PingjiaxinxiEntityExample();
example.or().andStuIdEqualTo(stuId).andTeaIdEqualTo(teaId);
return pingjiaxinxiEntityMapper.selectByExample(example).size() > 0;
}
}
如果也想学习本系统,下面领取。回复:041springboot