作者主页:夜未央5788
简介:Java领域优质创作者、Java项目、学习资料、技术互助
文末获取源码
项目介绍
管理员角色包含以下功能:
管理员登录,修改密码,管理员管理,货号管理,仓库管理,入库管理,出库管理,查看入库信息等功能。
由于本程序规模不大,可供课程设计,毕业设计学习演示之用
环境需要
1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。
2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA;
3.tomcat环境:Tomcat 7.x,8.x,9.x版本均可
4.硬件环境:windows 7/8/10 1G内存以上;或者 Mac OS;
5.数据库:MySql 5.7版本;
技术栈
1. 后端:Spring+SpringMVC+Mybatis
2. 前端:HTML+CSS+JavaScript+jsp
使用说明
1. 使用Navicat或者其它工具,在mysql中创建对应名称的数据库,并导入项目的sql文件;
2. 使用IDEA/Eclipse/MyEclipse导入项目,Eclipse/MyEclipse导入时,若为maven项目请选择maven;若为maven项目,导入成功后请执行maven clean;maven install命令,然后运行;
3. 将项目中application.yml配置文件中的数据库配置改为自己的配置;
4. 运行项目,输入localhost:8080/ 登录
运行截图 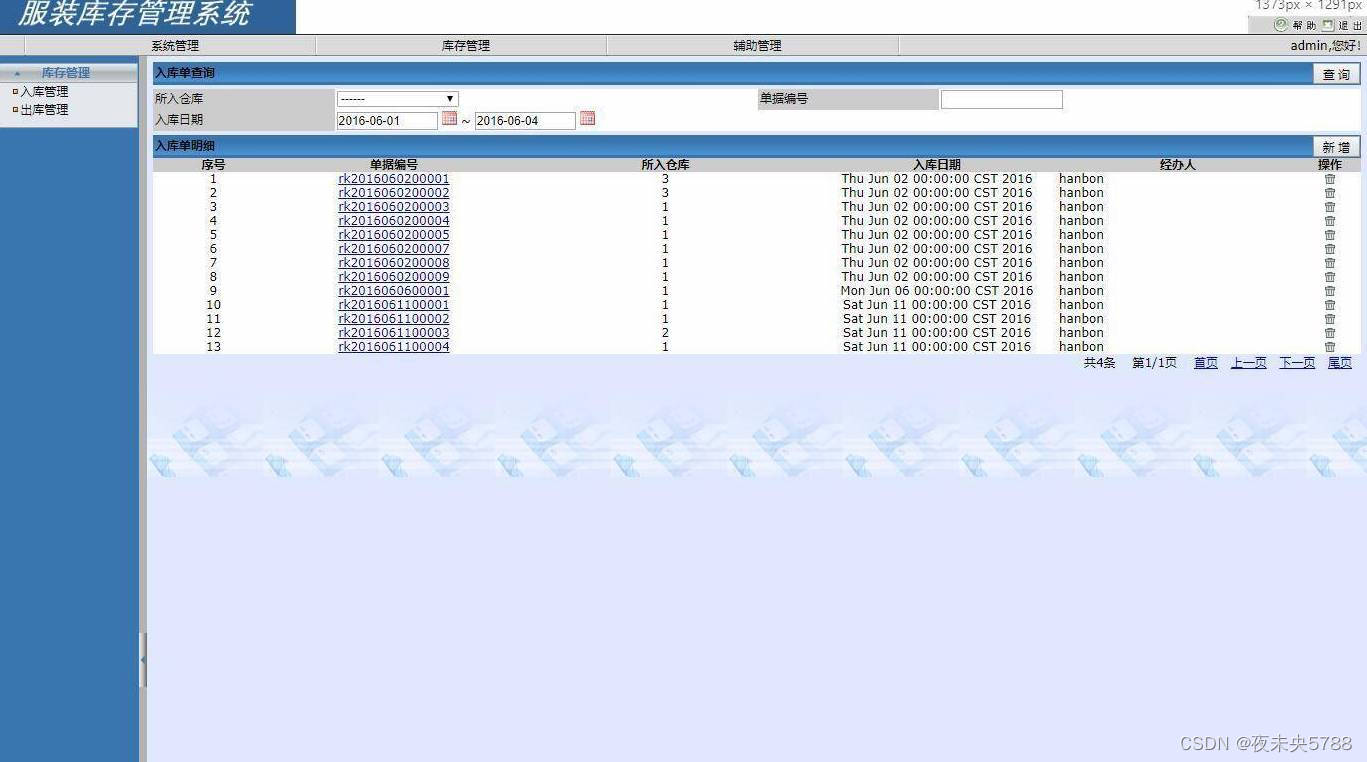
相关代码
通用管理控制器
public class BaseController {
@InitBinder
// 必须有一个参数WebDataBinder
public void initBinder(WebDataBinder binder) {
//System.out.println(binder.getFieldDefaultPrefix());
binder.registerCustomEditor(Date.class, new CustomDateEditor(
new SimpleDateFormat("yyyy-MM-dd"), false));
binder.registerCustomEditor(Integer.class, new PropertyEditorSupport() {
@Override
public String getAsText() {
return (getValue() == null) ? "" : getValue().toString();
}
@Override
public void setAsText(String text) {
Integer value = null;
if (null != text && !text.equals("")) {
try {
value = Integer.valueOf(text);
} catch(Exception ex) {
throw new UserException("数据格式输入不正确!");
}
}
setValue(value);
}
});
//binder.registerCustomEditor(Integer.class, null,new CustomNumberEditor(Integer.class, null, true));
binder.registerCustomEditor(Float.class, new PropertyEditorSupport() {
@Override
public String getAsText() {
return (getValue() == null)? "" : getValue().toString();
}
@Override
public void setAsText(String text) {
Float value = null;
if (null != text && !text.equals("")) {
try {
value = Float.valueOf(text);
} catch (Exception e) {
throw new UserException("数据格式输入不正确!");
}
}
setValue(value);
}
});
}
/**
* 处理图片文件上传,返回保存的文件名路径
* fileKeyName: 图片上传表单key
* @throws IOException
* @throws IllegalStateException
*/
public String handlePhotoUpload(HttpServletRequest request,String fileKeyName) throws IllegalStateException, IOException {
String fileName = "upload/NoImage.jpg";
MultipartHttpServletRequest multipartRequest = (MultipartHttpServletRequest) request;
/**构建图片保存的目录**/
String photoBookPathDir = "/upload";
/**得到图片保存目录的真实路径**/
String photoBookRealPathDir = request.getSession().getServletContext().getRealPath(photoBookPathDir);
/**根据真实路径创建目录**/
File photoBookSaveFile = new File(photoBookRealPathDir);
if(!photoBookSaveFile.exists())
photoBookSaveFile.mkdirs();
/**页面控件的文件流**/
MultipartFile multipartFile_photoBook = multipartRequest.getFile(fileKeyName);
if(!multipartFile_photoBook.isEmpty()) {
/**获取文件的后缀**/
String suffix = multipartFile_photoBook.getOriginalFilename().substring
(multipartFile_photoBook.getOriginalFilename().lastIndexOf("."));
String smallSuffix = suffix.toLowerCase();
if(!smallSuffix.equals(".jpg") && !smallSuffix.equals(".gif") && !smallSuffix.equals(".png") )
throw new UserException("图片格式不正确!");
/**使用UUID生成文件名称**/
String photoBookFileName = UUID.randomUUID().toString()+ suffix;//构建文件名称
//String logImageName = multipartFile.getOriginalFilename();
/**拼成完整的文件保存路径加文件**/
String photoBookFilePath = photoBookRealPathDir + File.separator + photoBookFileName;
File photoBookFile = new File(photoBookFilePath);
multipartFile_photoBook.transferTo(photoBookFile);
fileName = "upload/" + photoBookFileName;
}
return fileName;
}
/**
* 处理图片文件上传,返回保存的文件名路径
* fileKeyName: 图片上传表单key
* @throws IOException
* @throws IllegalStateException
*/
public String handleFileUpload(HttpServletRequest request,String fileKeyName) throws IllegalStateException, IOException {
String fileName = "";
MultipartHttpServletRequest multipartRequest = (MultipartHttpServletRequest) request;
/**构建图片保存的目录**/
String photoBookPathDir = "/upload";
/**得到图片保存目录的真实路径**/
String photoBookRealPathDir = request.getSession().getServletContext().getRealPath(photoBookPathDir);
/**根据真实路径创建目录**/
File photoBookSaveFile = new File(photoBookRealPathDir);
if(!photoBookSaveFile.exists())
photoBookSaveFile.mkdirs();
/**页面控件的文件流**/
MultipartFile multipartFile_photoBook = multipartRequest.getFile(fileKeyName);
if(!multipartFile_photoBook.isEmpty()) {
/**获取文件的后缀**/
String suffix = multipartFile_photoBook.getOriginalFilename().substring
(multipartFile_photoBook.getOriginalFilename().lastIndexOf("."));
/**使用UUID生成文件名称**/
String photoBookFileName = UUID.randomUUID().toString()+ suffix;//构建文件名称
//String logImageName = multipartFile.getOriginalFilename();
/**拼成完整的文件保存路径加文件**/
String photoBookFilePath = photoBookRealPathDir + File.separator + photoBookFileName;
File photoBookFile = new File(photoBookFilePath);
multipartFile_photoBook.transferTo(photoBookFile);
fileName = "upload/" + photoBookFileName;
}
return fileName;
}
/*向客户端输出操作成功或失败信息*/
public void writeJsonResponse(HttpServletResponse response,boolean success,String message)
throws IOException, JSONException {
response.setContentType("text/json;charset=UTF-8");
PrintWriter out = response.getWriter();
//将要被返回到客户端的对象
JSONObject json=new JSONObject();
json.accumulate("success", success);
json.accumulate("message", message);
out.println(json.toString());
out.flush();
out.close();
}
}
系统管理控制器
@Controller
@SessionAttributes("username")
public class SystemController {
@Resource AdminService adminService;
@RequestMapping(value="/login",method=RequestMethod.GET)
public String login(Model model) {
model.addAttribute(new Admin());
return "login";
}
@RequestMapping(value="/login",method=RequestMethod.POST)
public void login(@Validated Admin admin,BindingResult br,Model model,HttpServletRequest request,HttpServletResponse response,HttpSession session) throws Exception {
boolean success = true;
String msg = "";
if(br.hasErrors()) {
msg = br.getAllErrors().toString();
success = false;
}
if (!adminService.checkLogin(admin)) {
msg = adminService.getErrMessage();
success = false;
}
if(success) {
session.setAttribute("username", admin.getUsername());
}
response.setContentType("text/json;charset=UTF-8");
PrintWriter out = response.getWriter();
//将要被返回到客户端的对象
JSONObject json=new JSONObject();
json.accumulate("success", success);
json.accumulate("msg", msg);
out.println(json.toString());
out.flush();
out.close();
}
@RequestMapping("/logout")
public String logout(Model model,HttpSession session) {
model.asMap().remove("username");
session.invalidate();
return "redirect:/login";
}
@RequestMapping(value="/changePassword",method=RequestMethod.POST)
public String ChangePassword(String oldPassword,String newPassword,String newPassword2,HttpServletRequest request,HttpSession session) throws Exception {
if(oldPassword.equals("")) throw new UserException("请输入旧密码!");
if(newPassword.equals("")) throw new UserException("请输入新密码!");
if(!newPassword.equals(newPassword2)) throw new UserException("两次新密码输入不一致");
String username = (String)session.getAttribute("username");
if(username == null) throw new UserException("session会话超时,请重新登录系统!");
Admin admin = adminService.findAdminByUserName(username);
if(!admin.getPassword().equals(oldPassword)) throw new UserException("输入的旧密码不正确!");
try {
adminService.changePassword(username,newPassword);
request.setAttribute("message", java.net.URLEncoder.encode(
"密码修改成功!", "GBK"));
return "message";
} catch (Exception e) {
e.printStackTrace();
request.setAttribute("error", java.net.URLEncoder
.encode("密码修改失败!","GBK"));
return "error";
}
}
}
如果也想学习本系统,下面领取。关注并回复:160ssm