前台主页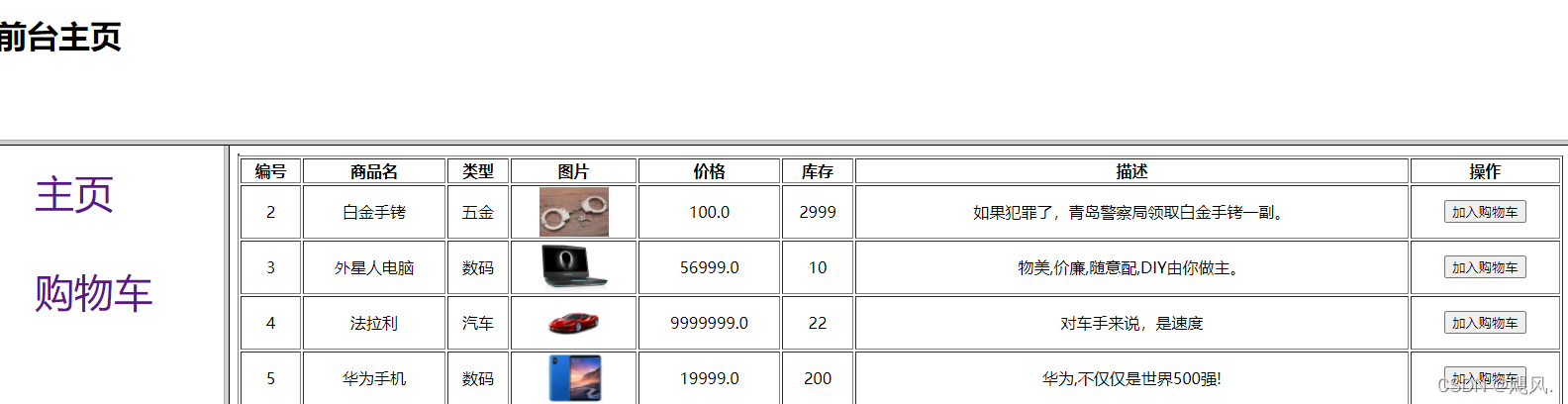
代码:
<%@page import="com.zking.carts.entity.Goods"%> <%@page import="java.util.List"%> <%@page import="com.zking.carts.biz.GoodsBiz"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="x" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <table border="1"> <table border="1" width="100%" style="text-align: center;"> <tr> <th>编号</th> <th>商品名</th> <th>类型</th> <th>图片</th> <th>价格</th> <th>库存</th> <th>描述</th> <th>操作</th> </tr> <x:if test="${empty listGoods}"> <jsp:forward page="doindex.jsp"></jsp:forward> </x:if> <x:forEach items="${listGoods }" var="goods" > <tr> <td>${goods.getGid() } </td> <td>${goods.getGname() }</td> <td>${goods.getGtype() }</td> <td><img style="width: 70px;height:50px" alt="" src="${goods.getGimage() }"></td> <td>${goods.getGprice() }</td> <td>${goods.getGkc() }</td> <td>${goods.getGinfo() }</td> <td><button onclick="addShooping(${goods.getGid() })">加入购物车</button ></td> </tr> </x:forEach> </table> <script type="text/javascript"> function addShooping(gid){ window.location.href="doshooping.jsp?gid="+gid+""; } </script> </body> </html>
购物车
<%@page import="com.zking.carts.entity.Shooping"%> <%@page import="java.util.List"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <table id="oTab" border="1" width="100%" style="text-align: center;"> <tr> <th>商品编号</th> <th>商品名</th> <th>类型</th> <th>图片</th> <th>价格</th> <th>数量</th> <th>总价</th> <th>操作</th> </tr> <c:if test="${not empty list}"> <c:forEach items="${list }" var="ls" begin="${start }" end="${end }"> <tr> <td>${ls.getGoods().getGid() }</td> <td>${ls.getGoods().getGname()}</td> <td>${ls.getGoods().getGtype() }</td> <td><img style="width: 70px; height: 50px" src="${ls.getGoods().getGimage() }"></td> <td>${ls.getGoods().getGprice() }</td> <td><button onclick="setCount('minus',${ls.getGoods().getGid() })">-</button> <input style="width: 50px" type="text" value="${ls.getGcount()}" /> <button onclick="setCount('add',${ls.getGoods().getGid() })">+</button></td> <td>${ls.getGtotal() }</td> <td><button onclick="del(${ls.getGoods().getGid() })">删除</button> <button onclick="update(${ls.getGoods().getGid() })">修改</button></td> </tr> </c:forEach> </c:if> <%-- <% List<Shooping> list=(List<Shooping>)session.getAttribute("list"); System.out.println(list); int pageIndex=1; int pageSize=3; String index=request.getParameter("pageIndex"); if(index!=null){ pageIndex=Integer.valueOf(index); } //统计购物车一共有多少件商品 int max=0; //设置开始值 int start=0; //设置结束 int end=0; //最大页码 int pageMax=0; //获取pageMax String pMax=request.getParameter("pageMax"); if(pMax!=null){ pageMax=Integer.valueOf(pMax); } if(list!=null){ max=list.size(); start=(pageIndex-1)*pageSize; end=pageIndex*pageSize>max?max:pageIndex*pageSize; pageMax=max%pageSize==0?max/pageSize:max/pageSize+1; list=list.subList(start, end); for (Shooping shooping : list) { %> <tr id="<%=shooping.getGoods().getGid()%>"> <td><%=shooping.getGoods().getGname()%></td> <td><%=shooping.getGoods().getGtype()%></td> <td><img style="width: 70px; height: 50px" alt="" src="<%=shooping.getGoods().getGimage()%>"></td> <td><%=shooping.getGoods().getGprice() %></td> <td><button onclick="setCount('minus',<%=shooping.getGoods().getGid()%>)">-</button> <input style="width: 50px" type="text" value="<%=shooping.getGcount() %>" /> <button onclick="setCount('add',<%=shooping.getGoods().getGid()%>)">+</button></td> <td><%=shooping.getGtotal() %></td> <td><button onclick="del(<%=shooping.getGoods().getGid()%>)">删除</button> <button onclick="update(<%=shooping.getGoods().getGid()%>)">修改</button></td> </tr> <% } } %> --%> </table> <div style="text-align: right;"> 《${pageIndex }/${pageMax }》 <a href="doShooping_fy.jsp?pageIndex=${pageIndex-1<1?1:pageIndex-1 }">上一页</a> <a href="doShooping_fy.jsp?pageIndex=${pageIndex+1>pageMax?pageMax:pageIndex+1 }">下一页</a> </div> <%-- <div style="text-align: right;"> 《<%=pageIndex %>/<%=pageMax %>》 <a href="Shooping.jsp?pageIndex=<%=pageIndex-1<1?1:pageIndex-1%>&pageMax=<%=pageMax%>">上一页</a> <a href="Shooping.jsp?pageIndex=<%=pageIndex+1>pageMax?pageMax:pageIndex+1%>&pageMax=<%=pageMax%>">下一页</a> </div> --%> <script type="text/javascript"> function del(gid){ window.location.href="dodel.jsp?gid="+gid+""; } /* function update(gid){ window.location.href="doshooping.jsp?gid="+gid+""; } */ window.onload=function(){ } function setCount(type,id){ var count=document.getElementById(id).cells[4].children[1].value; if(type==="minus"){ if(count<=1){ }else{ count--; } } if(type=="add"){ count++; } document.getElementById(id).cells[4].children[1].value=count; setTotal(); } function setTotal(){ var tb=document.getElementById("oTab"); var trs=tb.rows; for(var i=1;i<trs.length;i++){ //价格 var price=trs[i].cells[3].innerHTML; //数量 var count=trs[i].cells[4].children[1].value; trs[i].cells[5].innerHTML=price*count; } } function update(gid,count){ var count=document.getElementById(gid).cells[4].children[1].value; window.location.href="doupdate.jsp?gid="+gid+"&count="+count+""; } </script> </body> </html>