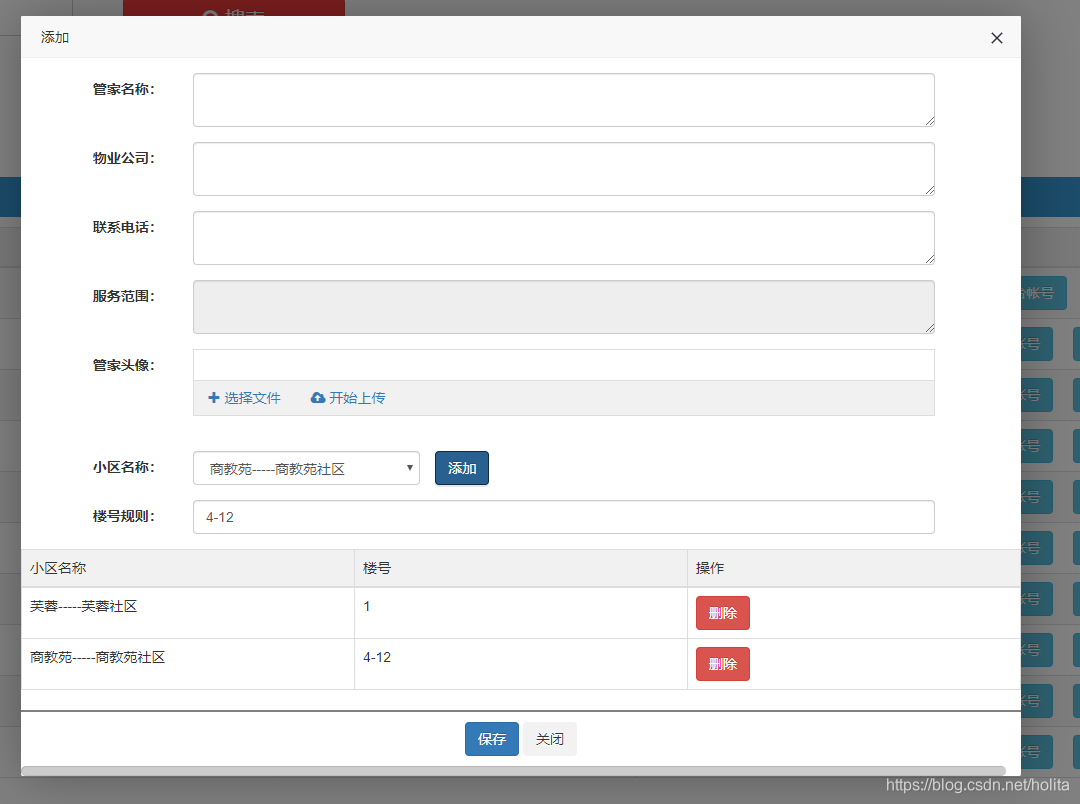
SdHouseKeeperController
package com.wise.SpringController.web.songduweb;
import com.wise.SpringController.dao.SdTCommunityMapper;
import com.wise.SpringController.domain.SdHouseKeeperBean;
import com.wise.SpringController.domain.TableDataInfo;
import com.wise.SpringController.domain.ToolBorrowingBean;
import com.wise.SpringController.mapper.DepartmentMapper;
import com.wise.SpringController.mapper.SUserMapper;
import com.wise.SpringController.mapper.TJuweiMapper;
import com.wise.SpringController.model.Department;
import com.wise.SpringController.model.SUser;
import com.wise.SpringController.model.T_Community;
import com.wise.SpringController.model.T_Juwei;
import com.wise.SpringController.service.SUserService;
import com.wise.SpringController.service.SdHouseKeeperService;
import com.wise.SpringController.tool.CustomResponse;
import com.wise.SpringController.tool.Md5Utils;
import com.wise.SpringController.view.SessionBean;
import com.wise.SpringController.vo.SdHouseKeeperVO;
import com.wise.SpringController.vo.ToolBorrowingVO;
import com.wise.SpringController.web.BaseController;
import org.hibernate.SQLQuery;
import org.hibernate.transform.Transformers;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import javax.persistence.Query;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/sdHouseKeeper")
public class SdHouseKeeperController extends BaseController {
@Autowired
private SdHouseKeeperService houseKeeperService;
@Autowired
private SdTCommunityMapper tCommunityMapper;
@Autowired
private TJuweiMapper tJuweiMapper;
@Autowired
private HttpSession httpsession;
@Autowired
SUserMapper suserMapper;
@Autowired
SUserService suserService;
@Autowired
DepartmentMapper departmentMapper;
@RequestMapping(value = "/index")
public String index(Model model) {
return "sdhousekeeper/index";
}
@RequestMapping("/formView")
public String formView(Model model, String action, HttpServletRequest request, SdHouseKeeperBean sdHouseKeeperBean) {
SdHouseKeeperVO caseOpenBean = null;
if (sdHouseKeeperBean.getId() != null) {
caseOpenBean = (SdHouseKeeperVO)houseKeeperService.selectHouseKeeperVOById(request,sdHouseKeeperBean);
} else {
caseOpenBean = new SdHouseKeeperVO();
}
model.addAttribute("sdHouseKeeperBean", caseOpenBean);
model.addAttribute("communityList", tCommunityMapper.selectTCommunityList(new T_Community()));
if("edit".equals(action) || "add".equals(action)){
return "sdhousekeeper/update";
}else {
return "sdhousekeeper/form";
}
}
@RequestMapping("selectHouseKeeperList")
@ResponseBody
public Object selectHouseKeeperList(HttpServletRequest request, SdHouseKeeperBean sdHouseKeeperBean){
startPage();
List<SdHouseKeeperVO> chartBean = houseKeeperService.selectHouseKeeperVO(request,sdHouseKeeperBean);
TableDataInfo dataTable = getDataTable(chartBean);
if(chartBean!=null){
return new CustomResponse(true,"获取成功",dataTable);
}else {
return new CustomResponse(false,"获取失败",dataTable);
}
}
@RequestMapping("insertHouseKeeper")
@ResponseBody
public Object insertHouseKeeper(HttpServletRequest request, SdHouseKeeperBean sdHouseKeeperBean,String attachmentId, String[] communityList, String[] floor){
SessionBean sessionBean = (SessionBean)httpsession.getAttribute("user");
sdHouseKeeperBean.setCreateUserId(sessionBean.getUserid());
Object chartBean = houseKeeperService.insertHouseKeeper(request,sdHouseKeeperBean,attachmentId,communityList,floor);
return chartBean;
}
@RequestMapping("updateHouseKeeper")
@ResponseBody
public Object updateHouseKeeper(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean,String attachmentId, String[] communityList, String[] floor){
Object chartBean = houseKeeperService.updateHouseKeeper(request,sdHouseKeeperBean,attachmentId,communityList,floor);
return chartBean;
}
@RequestMapping("deleteHouseKeeperById")
@ResponseBody
public Object deleteRotationChartById(String id){
Boolean aBoolean = houseKeeperService.deleteHouseKeeperById(id);
if(aBoolean){
return new CustomResponse(true,"success");
}else {
return new CustomResponse(false,"error");
}
}
@PostMapping(value = "deleteFile")
@ResponseBody
public Object deleteFile(String enclosureId, String attachmentId, HttpSession session) {
Map<String, Object> result = new HashMap<>();
try {
houseKeeperService.deleteFile(attachmentId,enclosureId);
result.put("status", true);
result.put("msg", "导入成功");
return result;
} catch (Exception e) {
result.put("status", false);
result.put("msg", "导入失败");
return result;
}
}
@RequestMapping("/function/add")
public String useradd(Model model,String id,HttpServletRequest request) {
SessionBean sessionbean = new SessionBean();
sessionbean = (SessionBean) httpsession.getAttribute("user");
SdHouseKeeperBean sdHouseKeeperBean = new SdHouseKeeperBean();
sdHouseKeeperBean.setId(id);
model.addAttribute("departmentlist", tJuweiMapper.findAll());
model.addAttribute("user", sessionbean);
model.addAttribute("sdHouseKeeperBean", houseKeeperService.selectHouseKeeperVOById(request,sdHouseKeeperBean));
return "sdhousekeeper/add";
}
@ResponseBody
@RequestMapping(value = "/saveUser", method = RequestMethod.POST)
public Object save(SUser suser, Model model, @RequestParam(value = "passwordss") String passwordss, String departmentidsss,String houseKeeperId,HttpServletRequest request) {
String aaa = Md5Utils.MD5Encode(passwordss, "utf-8", true);
System.out.println(aaa);
if (suser.getId() != null) {
SUser user =suserMapper.findAllById(suser.getId());
user.setUserName(suser.getUserName());
user.setFullName(suser.getFullName());
user.setLine(suser.getLine());
user.setMobile(suser.getMobile());
if (org.apache.commons.lang3.StringUtils.isNotEmpty(passwordss)) {
user.setPassword(aaa);
}
suser = user;
} else {
suser.setDelstate(0);
suser.setIs_lock(0);
suser.setIs_admin(0);
suser.setPassword(aaa);
}
Department dep= departmentMapper.findByCodeAndDelstate(departmentidsss, 0);
suser.setDepartmentid(dep.getId());
SUser save = suserService.save(suser);
SdHouseKeeperBean sdHouseKeeperBean = new SdHouseKeeperBean();
sdHouseKeeperBean.setId(houseKeeperId);
sdHouseKeeperBean.setUserId(save.getId());
houseKeeperService.addAccountNumber(sdHouseKeeperBean);
return "true";
}
}
SdHouseKeeperService
package com.wise.SpringController.service;
import com.wise.SpringController.domain.SdHouseKeeperBean;
import com.wise.SpringController.domain.SdHouserKeeperRange;
import com.wise.SpringController.vo.SdHouseKeeperVO;
import org.apache.ibatis.annotations.Param;
import javax.servlet.http.HttpServletRequest;
import java.util.List;
import java.util.Map;
public interface SdHouseKeeperService {
Object selectHouseKeeperVOById(HttpServletRequest request, SdHouseKeeperBean sdHouseKeeperBean);
SdHouseKeeperBean selectHouseKeeperById(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean);
List<SdHouseKeeperBean> selectHouseKeeperList(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean);
List<SdHouseKeeperVO> selectHouseKeeperVO(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean);
List<SdHouseKeeperVO> selectHouseKeeperVOForWeChat(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean);
Object insertHouseKeeper(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean, String attachmentId, String[] communityList, String[] floor);
Object updateHouseKeeper(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean, String attachmentId, String[] communityList, String[] floor);
Object addAccountNumber(SdHouseKeeperBean sdHouseKeeperBean);
Object updateHouseKeeperWeChat(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean, String attachmentId);
boolean deleteHouseKeeperById(String id);
Map<String,Object> selectBuildingNo(String id);
void deleteFile(String enclosureId, String attachmentId);
SdHouserKeeperRange selectRangeList(String communityName,String floor,String juweiCode);
}
SdHouseKeeperServiceImpl
package com.wise.SpringController.service.impl;
import com.wise.SpringController.dao.SAttachmentMapper;
import com.wise.SpringController.dao.SdHouseKeeperMapper;
import com.wise.SpringController.dao.SdHouserKeeperRangeMapper;
import com.wise.SpringController.domain.Attachment;
import com.wise.SpringController.domain.SdHouseKeeperBean;
import com.wise.SpringController.domain.SdHouserKeeperRange;
import com.wise.SpringController.mapper.TCommunityMapper;
import com.wise.SpringController.model.T_Community;
import com.wise.SpringController.service.SdHouseKeeperService;
import com.wise.SpringController.tool.CustomResponse;
import com.wise.SpringController.vo.SdHouseKeeperVO;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import javax.servlet.http.HttpServletRequest;
import java.util.*;
@Service
public class SdHouseKeeperServiceImpl implements SdHouseKeeperService {
@Autowired
private SdHouseKeeperMapper sdHouseKeeperMapper;
@Autowired
private SAttachmentMapper sAttachmentMapper;
@Autowired
private SdHouserKeeperRangeMapper rangeMapper;
@Autowired
private TCommunityMapper communityMapper;
@Override
public Object selectHouseKeeperVOById(HttpServletRequest request, SdHouseKeeperBean sdHouseKeeperBean) {
String requestUrl = request.getScheme()
+ "://" + request.getServerName()
+ ":" + request.getServerPort()
+ "/files/songdu";
SdHouseKeeperVO sdHouseKeeperVO = sdHouseKeeperMapper.selectHouseKeeperVOById(sdHouseKeeperBean.getId());
List<Attachment> attachments = sAttachmentMapper.getListByTableNameAndRelationId(sdHouseKeeperVO.getTitle().toLowerCase(), sdHouseKeeperVO.getId());
for (Attachment att : attachments
) {
att.setServerPath(requestUrl+"/SD_HOUSE_KEEPER".toLowerCase()+"/"+att.getFileName());
}
sdHouseKeeperVO.setAttachments(attachments);
return sdHouseKeeperVO;
}
@Override
public SdHouseKeeperBean selectHouseKeeperById(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean) {
return null;
}
@Override
public List<SdHouseKeeperBean> selectHouseKeeperList(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean) {
return null;
}
@Override
public List<SdHouseKeeperVO> selectHouseKeeperVO(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean) {
String requestUrl = request.getScheme()
+ "://" + request.getServerName()
+ ":" + request.getServerPort()
+ "/files/songdu";
List<SdHouseKeeperVO> keeperVOS = sdHouseKeeperMapper.selectHouseKeeperVO(sdHouseKeeperBean);
for(SdHouseKeeperVO rotationChartBean1 : keeperVOS){
List<Attachment> attachments = sAttachmentMapper.getListByTableNameAndRelationId(rotationChartBean1.getTitle().toLowerCase(), rotationChartBean1.getId());
for (Attachment att : attachments
) {
att.setServerPath(requestUrl+"/SD_HOUSE_KEEPER".toLowerCase()+"/"+att.getFileName());
}
rotationChartBean1.setAttachments(attachments);
}
return keeperVOS;
}
@Override
public List<SdHouseKeeperVO> selectHouseKeeperVOForWeChat(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean) {
String requestUrl = request.getScheme()
+ "://" + request.getServerName()
+ ":" + request.getServerPort()
+ "/files/songdu";
List<SdHouseKeeperVO> keeperVOS = sdHouseKeeperMapper.selectHouseKeeperVOForWeChat(sdHouseKeeperBean);
for(SdHouseKeeperVO rotationChartBean1 : keeperVOS){
List<Attachment> attachments = sAttachmentMapper.getListByTableNameAndRelationId(rotationChartBean1.getTitle().toLowerCase(), rotationChartBean1.getId());
for (Attachment att : attachments
) {
att.setServerPath(requestUrl+"/SD_HOUSE_KEEPER".toLowerCase()+"/"+att.getFileName());
}
rotationChartBean1.setAttachments(attachments);
}
return keeperVOS;
}
@Override
@Transactional
public Object insertHouseKeeper(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean, String attachmentId, String[] communityList, String[] floor) {
String s = UUID.randomUUID().toString().replace("-", "").toUpperCase();
sdHouseKeeperBean.setId(s);
sdHouseKeeperBean.setCreateTime(new Date());
sdHouseKeeperBean.setServiceStar("5");
String range="";
for(int i=0;i<communityList.length;i++){
T_Community tCommunity = communityMapper.getCommunityById(Integer.valueOf(communityList[i]));
if(i<communityList.length){
range+=tCommunity.getCommunityName() + floor[i] + "号,";
}
if(floor[i].contains("-")){
String[] split = floor[i].split("-");
int integer = Integer.valueOf(split[1]);
for(int j=1;j<=integer;j++){
SdHouserKeeperRange sdHouserKeeperRange = new SdHouserKeeperRange();
String uuid = UUID.randomUUID().toString().replace("-", "").toUpperCase();
sdHouserKeeperRange.setCommunityName(tCommunity.getCommunityName());
sdHouserKeeperRange.setCommunityId(String.valueOf(tCommunity.getId()));
sdHouserKeeperRange.setJuweiCode(String.valueOf(tCommunity.getJuweiCode()));
sdHouserKeeperRange.setFloor(j+"号");
sdHouserKeeperRange.setHousekeeperId(s);
sdHouserKeeperRange.setCreateTime(new Date());
sdHouserKeeperRange.setId(uuid);
rangeMapper.insertHouserKeeperRange(sdHouserKeeperRange);
}
}else {
SdHouserKeeperRange sdHouserKeeperRange = new SdHouserKeeperRange();
String uuid = UUID.randomUUID().toString().replace("-", "").toUpperCase();
sdHouserKeeperRange.setCommunityName(tCommunity.getCommunityName());
sdHouserKeeperRange.setCommunityId(String.valueOf(tCommunity.getId()));
sdHouserKeeperRange.setJuweiCode(String.valueOf(tCommunity.getJuweiCode()));
sdHouserKeeperRange.setFloor(floor[i]+"号");
sdHouserKeeperRange.setHousekeeperId(s);
sdHouserKeeperRange.setCreateTime(new Date());
sdHouserKeeperRange.setId(uuid);
rangeMapper.insertHouserKeeperRange(sdHouserKeeperRange);
}
sdHouseKeeperBean.setRange(range.substring(0,range.length()-1));
}
int chartBean = sdHouseKeeperMapper.insertHouseKeeper(sdHouseKeeperBean);
if(chartBean>0){
if (org.apache.commons.lang3.StringUtils.isNotEmpty(attachmentId)){
List<Integer> attachmentids=new ArrayList<>();
String[] split = attachmentId.split(",");
for (String str:split
) {
if (org.apache.commons.lang3.StringUtils.isNotEmpty(str)){
attachmentids.add(Integer.valueOf(str));
sAttachmentMapper.updateBind(s,Integer.valueOf(str));
}
}
}
return new CustomResponse(true, "添加成功");
}else{
return new CustomResponse(false, "添加失败");
}
}
@Override
@Transactional
public Object updateHouseKeeper(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean, String attachmentId, String[] communityList, String[] floor) {
SdHouseKeeperBean bean = sdHouseKeeperMapper.selectHouseKeeperById(sdHouseKeeperBean.getId());
String id = bean.getId();
BeanUtils.copyProperties(sdHouseKeeperBean,bean);
sdHouseKeeperBean=bean;
String range="";
if(communityList!=null && communityList.length>0){
rangeMapper.deleteByHousekeeperId(id);
for(int i=0;i<communityList.length;i++){
T_Community tCommunity = communityMapper.getCommunityById(Integer.valueOf(communityList[i]));
if(i<communityList.length){
range+=tCommunity.getCommunityName() + floor[i] + "号,";
}
if(floor[i].contains("-")){
String[] split = floor[i].split("-");
int integer = Integer.valueOf(split[1]);
for(int j=1;j<=integer;j++){
SdHouserKeeperRange sdHouserKeeperRange = new SdHouserKeeperRange();
String uuid = UUID.randomUUID().toString().replace("-", "").toUpperCase();
sdHouserKeeperRange.setCommunityName(tCommunity.getCommunityName());
sdHouserKeeperRange.setCommunityId(String.valueOf(tCommunity.getId()));
sdHouserKeeperRange.setJuweiCode(String.valueOf(tCommunity.getJuweiCode()));
sdHouserKeeperRange.setFloor(j+"号");
sdHouserKeeperRange.setHousekeeperId(id);
sdHouserKeeperRange.setCreateTime(new Date());
sdHouserKeeperRange.setId(uuid);
rangeMapper.insertHouserKeeperRange(sdHouserKeeperRange);
}
}else {
SdHouserKeeperRange sdHouserKeeperRange = new SdHouserKeeperRange();
String uuid = UUID.randomUUID().toString().replace("-", "").toUpperCase();
sdHouserKeeperRange.setCommunityName(tCommunity.getCommunityName());
sdHouserKeeperRange.setCommunityId(String.valueOf(tCommunity.getId()));
sdHouserKeeperRange.setJuweiCode(String.valueOf(tCommunity.getJuweiCode()));
sdHouserKeeperRange.setFloor(floor[i]+"号");
sdHouserKeeperRange.setHousekeeperId(id);
sdHouserKeeperRange.setCreateTime(new Date());
sdHouserKeeperRange.setId(uuid);
rangeMapper.insertHouserKeeperRange(sdHouserKeeperRange);
}
}
sdHouseKeeperBean.setRange(range.substring(0,range.length()-1));
}
int chartBean = sdHouseKeeperMapper.updateHouseKeeper(sdHouseKeeperBean);
if(chartBean>0 ){
if (org.apache.commons.lang3.StringUtils.isNotEmpty(attachmentId)){
List<Integer> attachmentids=new ArrayList<>();
String[] split = attachmentId.split(",");
for (String str:split
) {
if (org.apache.commons.lang3.StringUtils.isNotEmpty(str)){
attachmentids.add(Integer.valueOf(str));
sAttachmentMapper.updateBind(sdHouseKeeperBean.getId(),Integer.valueOf(str));
}
}
}
return new CustomResponse(true, "更新成功");
}else{
return new CustomResponse(false, "更新失败");
}
}
@Override
@Transactional
public Object updateHouseKeeperWeChat(HttpServletRequest request,SdHouseKeeperBean sdHouseKeeperBean, String attachmentId) {
SdHouseKeeperBean bean = sdHouseKeeperMapper.selectHouseKeeperById(sdHouseKeeperBean.getId());
BeanUtils.copyProperties(sdHouseKeeperBean,bean);
sdHouseKeeperBean=bean;
int chartBean = sdHouseKeeperMapper.updateHouseKeeper(sdHouseKeeperBean);
if(chartBean>0 ){
sAttachmentMapper.updateBindByRelationId("SD_HOUSE_KEEPER",sdHouseKeeperBean.getId());
if (org.apache.commons.lang3.StringUtils.isNotEmpty(attachmentId)){
List<Integer> attachmentids=new ArrayList<>();
String[] split = attachmentId.split(",");
for (String str:split
) {
if (org.apache.commons.lang3.StringUtils.isNotEmpty(str)){
attachmentids.add(Integer.valueOf(str));
sAttachmentMapper.updateBind(sdHouseKeeperBean.getId(),Integer.valueOf(str));
}
}
}
return new CustomResponse(true, "更新成功");
}else{
return new CustomResponse(false, "更新失败");
}
}
@Override
@Transactional
public boolean deleteHouseKeeperById(String id) {
Boolean aBoolean = sdHouseKeeperMapper.deleteHouseKeeperById(id);
return aBoolean;
}
@Override
public Map<String, Object> selectBuildingNo(String id) {
return sdHouseKeeperMapper.selectBuildingNo(id);
}
@Override
@Transactional
public void deleteFile(String enclosureId, String attachmentId) {
sAttachmentMapper.deleteFile(enclosureId,attachmentId);
}
@Override
public SdHouserKeeperRange selectRangeList(String communityName,String floor,String juweiCode) {
return rangeMapper.selectRangeList(communityName,floor,juweiCode);
}
@Override
@Transactional
public Object addAccountNumber(SdHouseKeeperBean sdHouseKeeperBean) {
SdHouseKeeperBean bean = sdHouseKeeperMapper.selectHouseKeeperById(sdHouseKeeperBean.getId());
String id = bean.getId();
BeanUtils.copyProperties(sdHouseKeeperBean,bean);
sdHouseKeeperBean=bean;
int chartBean = sdHouseKeeperMapper.updateHouseKeeper(sdHouseKeeperBean);
if(chartBean>0 ){
return new CustomResponse(true, "更新成功");
}else{
return new CustomResponse(false, "更新失败");
}
}
}
SdHouseKeeperMapper
package com.wise.SpringController.dao;
import com.wise.SpringController.domain.SdHouseKeeperBean;
import com.wise.SpringController.vo.SdHouseKeeperVO;
import java.util.List;
import java.util.Map;
public interface SdHouseKeeperMapper {
SdHouseKeeperVO selectHouseKeeperVOById(String id);
SdHouseKeeperBean selectHouseKeeperById(String id);
List<SdHouseKeeperBean> selectHouseKeeperList(SdHouseKeeperBean sdHouseKeeperBean);
List<SdHouseKeeperVO> selectHouseKeeperVO(SdHouseKeeperBean sdHouseKeeperBean);
List<SdHouseKeeperVO> selectHouseKeeperVOForWeChat(SdHouseKeeperBean sdHouseKeeperBean);
int insertHouseKeeper(SdHouseKeeperBean sdHouseKeeperBean);
int updateHouseKeeper(SdHouseKeeperBean sdHouseKeeperBean);
boolean deleteHouseKeeperById(String id);
Map<String,Object> selectBuildingNo(String id);
}
SdHouseKeeperMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.wise.SpringController.dao.SdHouseKeeperMapper">
<resultMap type="com.wise.SpringController.domain.SdHouseKeeperBean" id="SdHouseKeeperResult">
<result property="id" column="id"/>
<result property="createTime" column="create_time"/>
<result property="isDel" column="is_del"/>
<result property="updateTime" column="update_time"/>
<result property="describe" column="describe"/>
<result property="title" column="title"/>
<result property="housekeeperName" column="housekeeper_name"/>
<result property="propertyCompany" column="property_company"/>
<result property="telephone" column="telephone"/>
<result property="range" column="range"/>
<result property="serviceStar" column="service_star"/>
<result property="createUserId" column="createUserId"/>
<result property="juweiCode" column="juewi_code"/>
<result property="userId" column="user_id"/>
</resultMap>
<resultMap type="com.wise.SpringController.vo.SdHouseKeeperVO" id="SdHouseKeeperVO">
<result property="id" column="id"/>
<result property="createTime" column="create_time"/>
<result property="isDel" column="is_del"/>
<result property="updateTime" column="update_time"/>
<result property="describe" column="describe"/>
<result property="title" column="title"/>
<result property="housekeeperName" column="housekeeper_name"/>
<result property="propertyCompany" column="property_company"/>
<result property="telephone" column="telephone"/>
<result property="range" column="range"/>
<result property="serviceStar" column="service_star"/>
<result property="createUserId" column="createUserId"/>
<result property="juweiCode" column="juewi_code"/>
<result property="userId" column="user_id"/>
<result property="isAccountNumber" column="isAccountNumber"/>
</resultMap>
<select id="selectHouseKeeperVOById" resultMap="SdHouseKeeperVO">
select id,create_time,is_del,update_time,describe,title,housekeeper_name,property_company,telephone,range,
case when service_star=1 then '★' when service_star=2 then '★★' when service_star=3
then '★★★' when service_star=4 then '★★★★' else '★★★★★' end service_star,createUserId,juwei_code
from SD_HOUSE_KEEPER where id =#{id, jdbcType=VARCHAR}
</select>
<select id="selectHouseKeeperById" resultMap="SdHouseKeeperResult">
select id,create_time,is_del,update_time,describe,title,housekeeper_name,property_company,telephone,range,
service_star,createUserId,juwei_code
from SD_HOUSE_KEEPER where id =#{id, jdbcType=VARCHAR}
</select>
<select id="selectHouseKeeperList" parameterType="com.wise.SpringController.domain.SdHouseKeeperBean" resultMap="SdHouseKeeperResult">
select
id,create_time,is_del,update_time,describe,title,housekeeper_name,property_company,telephone,range,
service_star,createUserId,juwei_code
from SD_HOUSE_KEEPER where is_del=0
<if test="housekeeperName != null and housekeeperName != ''">
AND housekeeper_name like concat(concat('%',#{housekeeperName}),'%')
</if>
<if test="createUserId != null and createUserId != ''">
AND createUserId=#{createUserId}
</if>
order by create_time desc
</select>
<select id="selectHouseKeeperVO" parameterType="com.wise.SpringController.domain.SdHouseKeeperBean" resultMap="SdHouseKeeperVO">
select
id,create_time,is_del,update_time,describe,title,housekeeper_name,property_company,telephone,range,
service_star,createUserId,juwei_code,(select count(*) from s_user where id=user_id)isAccountNumber
from SD_HOUSE_KEEPER where is_del=0
<if test="housekeeperName != null and housekeeperName != ''">
AND housekeeper_name like concat(concat('%',#{housekeeperName}),'%')
</if>
<if test="range != null and range != ''">
AND range like concat(concat('%',#{range}),'%')
</if>
<if test="juweiCode != null and juweiCode != ''">
AND juwei_code =#{juweiCode}
</if>
<if test="createUserId != null and createUserId != ''">
AND createUserId=#{createUserId}
</if>
<if test="id != null and id != ''">
AND id=#{id}
</if>
order by create_time desc
</select>
<select id="selectHouseKeeperVOForWeChat" parameterType="com.wise.SpringController.domain.SdHouseKeeperBean" resultMap="SdHouseKeeperVO">
select
id,create_time,is_del,update_time,describe,title,housekeeper_name,property_company,telephone,range,
service_star,createUserId,juwei_code
from SD_HOUSE_KEEPER where is_del=0
<if test="housekeeperName != null and housekeeperName != ''">
AND housekeeper_name like concat(concat('%',#{housekeeperName}),'%')
</if>
<if test="range != null and range != ''">
AND range like concat(concat('%',#{range}),'%')
</if>
<if test="juweiCode != null and juweiCode != ''">
AND juwei_code =#{juweiCode}
</if>
<if test="createUserId != null and createUserId != ''">
AND createUserId=#{createUserId}
</if>
order by service_star desc
</select>
<insert id="insertHouseKeeper" parameterType="com.wise.SpringController.domain.SdHouseKeeperBean">
insert into SD_HOUSE_KEEPER
<trim prefix="(" suffix=")" suffixOverrides=",">
id,
<if test="createTime != null ">create_time,</if>
<if test="isDel != null ">is_del,</if>
<if test="updateTime != null ">update_time,</if>
<if test="describe != null and describe != ''">describe,</if>
<if test="housekeeperName != null and housekeeperName != ''">housekeeper_name,</if>
<if test="title != null and title != ''">title,</if>
<if test="propertyCompany != null and propertyCompany != ''">property_company,</if>
<if test="telephone != null and telephone != ''">telephone,</if>
<if test="range != null and range != ''">range,</if>
<if test="serviceStar != null and serviceStar != ''">service_star,</if>
<if test="juweiCode != null and juweiCode != ''">juwei_code,</if>
<if test="createUserId != null and createUserId != '' ">createUserId</if>
</trim>
<trim prefix="values (" suffix=")" suffixOverrides=",">
<if test="createTime != null ">#{id},</if>
<if test="createTime != null ">#{createTime},</if>
<if test="isDel != null ">#{isDel},</if>
<if test="updateTime != null ">#{updateTime},</if>
<if test="describe != null and describe != ''">#{describe},</if>
<if test="housekeeperName != null and housekeeperName != ''">#{housekeeperName},</if>
<if test="title != null and title != ''">#{title},</if>
<if test="propertyCompany != null and propertyCompany != ''">#{propertyCompany},</if>
<if test="telephone != null and telephone != ''">#{telephone},</if>
<if test="range != null and range != ''">#{range},</if>
<if test="serviceStar != null and serviceStar != ''">#{serviceStar},</if>
<if test="juweiCode != null and juweiCode != ''">#{juweiCode},</if>
<if test="createUserId != null and createUserId != '' ">#{createUserId}</if>
</trim>
</insert>
<update id="updateHouseKeeper" parameterType="com.wise.SpringController.domain.SdHouseKeeperBean">
update SD_HOUSE_KEEPER
<trim prefix="SET" suffixOverrides=",">
<if test="isDel != null ">is_del = #{isDel},</if>
<if test="updateTime != null ">update_time = #{updateTime},</if>
<if test="describe != null ">describe = #{describe},</if>
<if test="housekeeperName != null and housekeeperName != ''">housekeeper_name=#{housekeeperName},</if>
<if test="title != null and title != ''">title=#{title},</if>
<if test="propertyCompany != null and propertyCompany != ''">property_company=#{propertyCompany},</if>
<if test="telephone != null and telephone != ''">telephone=#{telephone},</if>
<if test="range != null and range != ''">range=#{range},</if>
<if test="serviceStar != null and serviceStar != ''">service_star=#{serviceStar},</if>
<if test="createUserId != null and createUserId != '' ">createUserId=#{createUserId},</if>
<if test="juweiCode != null and juweiCode != '' ">juwei_code=#{juweiCode},</if>
<if test="userId != null ">user_id=#{userId}</if>
</trim>
where id = #{id}
</update>
<delete id="deleteHouseKeeperById">
update SD_HOUSE_KEEPER set is_del=1 where id = #{id}
</delete>
<select id="selectBuildingNo" resultType="map">
select c.id,c.juwei_code,c.community_name,b.address from sd_wechat_user u left join t_community c on u.community_id=c.id left join t_building_live b on u.building_number=b.id where u.community_id is not null and u.id=#{id}
</select>
</mapper>
index.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org" lang="zh_cn">
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<meta name="format-detection" content="telephone=no">
<meta name="format-detection" content="email=no">
<title>Title</title>
<link rel="stylesheet" th:href="@{/iframe/zui/css/zui.min.css}">
<link rel="stylesheet" th:href="@{/iframe/css/page1.css}">
<link rel="stylesheet" th:href="@{/css/zui.uploader.min.css}">
<link rel="stylesheet" th:href="@{/css/bootstrap.min.css}">
<link rel="stylesheet" th:href="@{/css/bootstrap-datetimepicker.min.css}">
<script th:src="@{/iframe/zui/lib/jquery/jquery.js}"></script>
<script type="text/javascript" th:src="@{/js/jquery-3.3.1.min.js}"></script>
<script type="text/javascript" th:src="@{/js/jquery.form.js}"></script>
<script type="text/javascript" th:src="@{/js/jquery.page.js}"></script>
<script type="text/javascript" th:src="@{/js/zui.min.js}"></script>
<script type="text/javascript" th:src="@{/js/eventcommon.js}"></script>
<script type="text/javascript" th:src="@{/js/bootstrap-multiselect.js}"></script>
<script type="text/javascript" th:src="@{/js/bootstrap-datetimepicker.min.js}"></script>
<script type="text/javascript" th:src="@{/js/bootstrap-datetimepicker.zh-CN.js}"></script>
<script type="text/javascript" th:src="@{/js/common.js}"></script>
<script type="text/javascript" th:src="@{/js/zui.uploader.min.js}"></script>
<script type="text/javascript" th:src="@{/layer/layer.js}"></script>
<script type="text/javascript" th:src="@{/js/wise.openlayer.js}"></script>
<script type="text/javascript" th:src="@{/js/laydate.js}"></script>
</head>
<body>
<div class="wrapper">
<div class="search row">
<div class="search-item col-sm-3">
<label class="search-label">名称</label>
<input type="text" id="housekeeperName" name="housekeeperName" class="form-control">
</div>
<div class="search-item col-sm-2">
<button class="btn btn-danger search-btn" type="button" onclick='loadEventList(1)'>
<i class="icon icon-search"></i>
<span class="text">搜索</span>
</button>
</div>
</div>
<div class="result">
<div class="result-option">
<a class="btn btn-danger result-btn"
th:onclick="'openlayer.openWindows(\'添加\',\''+@{/sdHouseKeeper/formView(action=add)}+'\')'" data-position="60px"
aria-hidden="true">
<i class="icon icon-plus"></i>
<span class="text">添加</span>
</a>
</div>
<input type="hidden" id="dqpage">
<h2 class="result-table-title">金牌管家</h2>
<div class="result-table">
<table class="table table-striped table-bordered table-hover">
<thead>
<tr>
<th>管家名称</th>
<th>物业公司</th>
<th>电话</th>
<th>服务星级</th>
<th>操 作</th>
</tr>
</thead>
<tbody class="tbody" id="tablist">
</tbody>
</table>
</div>
<div class="result-footer">
<ul class="pager" data-ride="pager"
data-elements="prev, nav, next"
data-max-nav-count="6"
data-page="1" data-rec-total="100" data-rec-per-page="10">
</ul>
</div>
</div>
</div>
</body>
<script type="text/javascript" th:inline="javascript">
var basePath =;
$(function () {
loadEventList(1);
});
function reflush() {
var pagenum = $("#dqpage").val();
loadEventList(pagenum);
}
$('.pager').pager({
page: 1,
onPageChange: function (state, oldState) {
if (typeof (oldState.page) !== "undefined") {
if (state.page !== oldState.page) {
loadEventList(state.page);
$("#dqpage").val(state.page);
}
}
}
});
function loadEventList(page) {
$.ajax({
type: "POST",
url: basePath + "sdHouseKeeper/selectHouseKeeperList",
async: false,
data: "pageNum=" + page +"&pageSize=10&housekeeperName=" + $("#housekeeperName").val(),
success: function (obj) {
console.log(obj);
console.log(obj.data.rows);
$("#tablist tr").remove();
$(obj.data.rows).each(function () {
var html = "<tr>";
if(this.housekeeperName !=null){
html += "<td>" + this.housekeeperName + "</td>";
}else{
html += "<td></td>";
}
if(this.propertyCompany !=null){
html += "<td>" + this.propertyCompany + "</td>";
}else{
html += "<td></td>";
}
if(this.telephone !=null){
html += "<td>" + this.telephone + "</td>";
}else{
html += "<td></td>";
}
if(this.serviceStar !=null){
if(this.serviceStar =="1"){
html += "<td>★</td>";
}
if(this.serviceStar =="2"){
html += "<td>★★</td>";
}
if(this.serviceStar =="3"){
html += "<td>★★★</td>";
}
if(this.serviceStar =="4"){
html += "<td>★★★★</td>";
}
if(this.serviceStar =="5"){
html += "<td>★★★★★</td>";
}
}else{
html += "<td></td>";
}
html += "<td>";
if(this.isAccountNumber>0){
html += "<a class='btn btn-info'>已有帐号</a>";
}else{
html += "<a class='btn btn-info' οnclick='openlayer.openWindows('添加后台帐号','function/add?id=" + this.id+ "')'>添加后台帐号</a>";
}
html += "<a class='btn btn-info' οnclick='openlayer.openWindows('详情','formView?id=" + this.id + "&action=show')'>查看</a>";
html += "<a class='btn btn-info' οnclick='openlayer.openWindows('编辑','formView?id=" + this.id + "&action=edit')'>编辑</a>";
html+="<button class='btn btn-danger' οnclick='delbyid(\"" + this.id + "\")' >删除</button>";
html += "</td>";
html += "</tr>";
$("#tablist").append(html);
});
refreshPager(obj.data,page);
}
});
}
function giveBack(id) {
if (confirm("确定需要归还工具吗?")) {
$.ajax({
type: "POST",
url: basePath + "toolBorrowing/giveBack?id=" + id,
async: false,
success: function (obj) {
if (obj.status) {
layer.msg("归还成功");
loadEventList(1);
} else {
layer.msg("归还失败");
}
}
});
}
}
function delbyid(id) {
if (confirm("确定需要删除吗?")) {
$.ajax({
type: "POST",
url: basePath + "sdHouseKeeper/deleteHouseKeeperById?id=" + id,
async: false,
success: function (obj) {
if (obj.status) {
layer.msg("删除成功");
loadEventList(1);
} else {
layer.msg("删除失败");
}
}
});
}
}
function refreshPager(obj,page) {
var pager = $('.pager').data('zui.pager');
pager.set(page, obj.total, 10);
}
</script>
</html>
update.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org" lang="en">
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"/>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<meta name="format-detection" content="telephone=no"/>
<meta name="format-detection" content="email=no"/>
</head>
<body style="background-color:white;overflow-x:hidden;overflow-y:auto">
<div style="margin-top: 15px;">
<form method="post" class="form-horizontal" id="saveForm">
<input type="hidden" class="form-control" name="id" th:value="${sdHouseKeeperBean.id}"/>
<div class="form-group">
<label class="col-sm-2">管家名称:</label>
<div class="col-xs-9">
<textarea class="form-control" name="housekeeperName" id="housekeeperName1" th:text="${sdHouseKeeperBean.housekeeperName}"></textarea>
</div>
</div>
<!--<div class="form-group">-->
<!--<label class="col-sm-2">物品分类:<span style="color:red">*</span></label>-->
<!--<div class="col-xs-3">-->
<!--<select class="form-control" id="articleClassify" name="articleClassify">-->
<!--<option value="">===请选择===</option>-->
<!--<option th:each="qt:${articleClassify}" th:value="${qt.dictkey}"-->
<!--th:text="${qt.dictvalue}"></option>-->
<!--</select>-->
<!--</div>-->
<!--<label class="col-sm-2">物品名称:</label>-->
<!--<div class="col-xs-3">-->
<!--<select class="form-control" name="articleName" id="articleName">-->
<!--<option value="">===请选择===</option>-->
<!--</select>-->
<!--</div>-->
<!--</div>-->
<div class="form-group">
<label class="col-sm-2">物业公司:</label>
<div class="col-xs-9">
<textarea class="form-control" name="propertyCompany" id="propertyCompany" th:text="${sdHouseKeeperBean.propertyCompany}"></textarea>
</div>
</div>
<div class="form-group">
<label class="col-sm-2">联系电话:</label>
<div class="col-xs-9">
<textarea class="form-control" name="telephone" id="telephone" th:text="${sdHouseKeeperBean.telephone}"></textarea>
</div>
</div>
<div class="form-group">
<label class="col-sm-2">服务范围:</label>
<div class="col-xs-9">
<textarea class="form-control" name="range" id="range" th:text="${sdHouseKeeperBean.range}" readonly></textarea>
</div>
</div>
<div class="form-group" style="margin-top:10px;" id="uploadM2">
<label class="col-sm-2">管家头像:</label>
<div class="col-xs-9">
<div id="uploader2" class="uploader" data-ride="uploader">
<div class="uploader-message text-center">
<div class="content"></div>
<button type="button" class="close">×</button>
</div>
<div class="uploader-files file-list file-list-lg" data-drag-placeholder=""></div>
<div class="uploader-actions">
<div class="uploader-status pull-right text-muted"></div>
<button type="button" class="btn btn-link uploader-btn-browse">
<i class="icon icon-plus"></i> 选择文件
</button>
<button type="button" class="btn btn-link uploader-btn-start">
<i class="icon icon-cloud-upload"></i> 开始上传
</button>
</div>
</div>
</div>
</div>
<div class="form-group">
<label class="col-sm-2">小区名称:</label>
<div class="col-sm-3">
<select class="form-control" id="type1" >
<option th:each="cl :${communityList}" th:value="${cl.id}"
th:text="${cl.communityName+'-----'+cl.juwei}"></option>
</select>
</div>
<a id="savebtn2" class=" btn btn-primary" href="javascript:void(0)" onclick="addlou()">添加</a>
</div>
<div class="form-group">
<label class="col-sm-2">楼号规则:</span></label>
<div class="col-xs-9">
<input class="form-control" id="range1"
placeholder="楼号规则:楼号配置1、楼号连续,如11-20号,请填写11-20 2 、单个楼号配置,如1号,请填写1"></input>
</div>
</div>
<table class="table table-hover table-bordered" style="table-layout:fixed;">
<thead>
<tr>
<th>小区名称</th>
<th>楼号</th>
<th>操作</th>
</tr>
</thead>
<tbody class="tbody" id="jutablist2">
</tbody>
</table>
<div style="border:1px solid gray"></div>
<div style="text-align:center;margin-top:10px">
<a class="btn btn-primary" href="javascript:submitForm('saveForm')" id="saveBtn">保存</a>
<a class="btn" data-dismiss="modal" onclick="goBack()">关闭</a>
</div>
</form>
</div>
</body>
<script type="text/javascript" th:inline="javascript">
var saveForm = $("#saveForm");
var action = [[${#httpServletRequest.getParameter("action")}]];
var basePath =;
var attachmentIds =[];
var id = [[${#request.getParameter('id')}]];
$(function () {
initDefaultSettings(action);
loadAttachmentList2();
});
function initDefaultSettings(action) {
if (action == "" || action == "show") {
disableTag("saveForm");
}
if (action == "edit" || action == "add") {
$(".form-date").datetimepicker(
{
language: "zh-CN",
weekStart: 1,
todayBtn: 1,
autoclose: 1,
todayHighlight: 1,
startView: 2,
minView: 0,
minuteStep: 1,
forceParse: 0,
format: 'yyyy-mm-dd hh:ii:ss',
});
}
}
$("#articleClassify").on("change", function () {
var jdbm = $("#articleClassify").val();
$("#articleName").children().not(':eq(0)').remove();
if (jdbm != 0) {
$.ajax({
type: "POST",
url: basePath + "/toolBorrowing/change",
data: {dictKey: jdbm},
datetype: "json",
success: function (data) {
for (var i = 0; i < data.toolBeans.length; i++) {
console.log(data);
$("#articleName").append("<option value='" + data.toolBeans[i].id + "'>" + data.toolBeans[i].name+"(剩余"+data.toolBeans[i].toolNum+")" + "</option>");
}
}
})
}
})
function addlou() {
var type = $("#type1").val();
var typeName = $("#type1").find("option:selected").text();
var subject = $("#range1").val();
if(subject ==''){
layer.msg("请填写楼号");
return false;
}
var data = type + "," + subject;
var html = "";
html += "<tr>";
html += "<td>" + typeName + "<input type='hidden' value='" + type + "' name='communityList'/></td>";
html += "<td>" + $("#range1").val() + "<input type='hidden' value='" + subject + "' name='floor' /></td>";
html += "<td> <a class='btn btn-danger' οnclick='javascript:removetr(this)'>删除</a></td>";
html += "</tr>";
$("#jutablist2").append(html);
}
function removetr(obj) {
$(obj).parents("tr").remove();
}
function disableTag(formId) {
var form = document.getElementById(formId)
var inputTags = form.getElementsByTagName("input");
for (i = 0; i < inputTags.length; i++) {
if (inputTags[i].type == "radio" || inputTags[i].type == "text" || inputTags[i].type == "password" || inputTags[i].type == "file") {
inputTags[i].readOnly = true;
}
if (inputTags[i].type == "checkbox") {
inputTags[i].disabled = true;
}
}
var selectTags = form.getElementsByTagName("select");
for (i = 0; i < selectTags.length; i++) {
selectTags[i].disabled = true;
}
var buttonTags = form.getElementsByTagName("button");
for (i = 0; i < buttonTags.length; i++) {
buttonTags[i].remove();
}
var textareaTags = form.getElementsByTagName("textarea");
for (i = 0; i < textareaTags.length; i++) {
textareaTags[i].readOnly = true;
}
$("#saveBtn").remove();
}
function preSubmitCheck() {
var errorMsg = [];
if ($("#housekeeperName1").val()=="") {
errorMsg.push("请输入管家名称");
}
if ($("#propertyCompany").val()=="") {
errorMsg.push("请输入物业公司");
}
if ($("#telephone").val()=="") {
errorMsg.push("请输入电话");
}
if ($("#serviceStar").val()=="") {
errorMsg.push("请输入服务星级");
}
var showMsg = errorMsg.join("<br/><br/>");
if (showMsg != "") {
layer.msg(showMsg)
}
return errorMsg.length == 0 ? true : false;
}
function goBack() {
var href = [[${#httpServletRequest.getHeader("referer")}]];
if (href == null || href.indexOf("formView") != -1 || href.indexOf("case/track") != -1) {
href = window.location.origin + window.location.pathname + "/../"
}
window.location.href = href;
}
function submitForm() {
if(action=='add'){
if (preSubmitCheck()) {
$.ajax({
type: "POST",
url: "/ljzqksg/sdHouseKeeper/insertHouseKeeper",
data: $('#saveForm').serialize() + "&attachmentId=" + attachmentIds,
success: function (result) {
alert("保存成功");
$("#saveBtn").hide();
goBack();
},
error: function () {
alert("保存失败");
}
});
}
}else {
if (preSubmitCheck()) {
$.ajax({
type: "POST",
url: "/ljzqksg/sdHouseKeeper/updateHouseKeeper",
data: $('#saveForm').serialize() + "&attachmentId=" + attachmentIds,
success: function (result) {
alert("保存成功");
$("#saveBtn").hide();
goBack();
},
error: function () {
alert("保存失败");
}
});
}
}
}
function loadAttachmentList2() {
var tablename = 'sd_house_keeper';
var downloadPath = "/files/songdu/" + tablename;
if (action == 'view') {
$.ajax({
type: "POST",
url: basePath + 'api/attachment/list',
data: "id=" + id + "&tablename=" + tablename.toLowerCase(),
async: false,
success: function (msg) {
console.log(msg);
var files = [];
if (msg.status) {
$.each(msg.data, function (key, val) {
var obj = new Object();
obj.name = val.originalName;
obj.size = val.fileSize;
obj.url = downloadPath + "/" + val.fileName;
obj.id = val.id;
files.push(obj);
});
}
var uploaderOptions;
uploaderOptions = {
lang: 'zh_cn',
deleteConfirm: true,
repeat: false,
rename: false,
renameByClick: false,
staticFiles: files,
};
$('#uploader2').uploader(uploaderOptions);
}
});
} else if (action == 'add') {
$('#uploader2').uploader({
autoUpload: false,
chunk_size: 0,
lang: 'zh_cn',
repeat: false,
renameByClick: false,
rename: false,
filters:{
mime_types: [
{title: '图片', extensions: 'jpg,gif,png'},
{title: '图标', extensions: 'ico'}
]
},
url: basePath + 'api/attachment/upload?tablename='+ tablename.toLowerCase(),
responseHandler: function (responseObject, file) {
var obj = eval("(" + responseObject.response + ")");
var msg;
if (obj.status) {
msg = "成功上传";
attachmentIds.push(obj.data.id);
} else {
msg = "上传失败";
}
layer.msg(msg);
}
});
} else {
$.ajax({
type: "POST",
url: basePath + 'api/attachment/list',
data: "id=" + id + "&tablename=" + tablename.toLowerCase(),
async: false,
success: function (msg) {
var files = [];
if (msg.status) {
$.each(msg.data, function (key, val) {
var obj = new Object();
obj.name = val.originalName;
obj.size = val.fileSize;
obj.url = downloadPath + "/" + val.fileName;
obj.id = val.id;
obj.attachmentId=val.id;
files.push(obj);
});
}
var uploaderOptions;
uploaderOptions = {
lang: 'zh_cn',
deleteConfirm: true,
repeat: false,
staticFiles: files,
renameByClick: false,
rename: false,
autoUpload: false,
chunk_size: 0,
filters:{
mime_types: [
{title: '图片', extensions: 'jpg,gif,png'},
{title: '图标', extensions: 'ico'}
]
},
url: basePath + 'api/attachment/upload?tablename='+ tablename.toLowerCase(),
responseHandler: function (responseObject, file) {
var obj = eval("(" + responseObject.response + ")");
var msg;
if (obj.status) {
msg = "成功上传";
attachmentIds.push(obj.data.id);
file.attachmentId = obj.data.id;
} else {
msg = "上传失败";
}
layer.msg(msg);
},
deleteActionOnDone: function (file, doRemoveFile) {
$.ajax({
type: "POST",
url: basePath + 'sdHouseKeeper/deleteFile',
data: "enclosureId=" + id + "&attachmentId=" + file.attachmentId,
async: false,
success: function (msg) {
if (msg.status) {
doRemoveFile();
} else {
layer.msg("删除失败,请重试");
}
}
});
},
};
$('#uploader2').uploader(uploaderOptions);
}
});
}
}
</script>
</html>
form.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org" lang="en">
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"/>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<meta name="format-detection" content="telephone=no"/>
<meta name="format-detection" content="email=no"/>
</head>
<body style="background-color:white;overflow-x:hidden;overflow-y:auto">
<div style="margin-top: 15px;">
<form method="post" class="form-horizontal" id="saveForm">
<input type="hidden" class="form-control" name="id" th:value="${sdHouseKeeperBean.id}"/>
<div class="form-group">
<label class="col-sm-2">管家名称:</label>
<div class="col-xs-9">
<textarea class="form-control" name="housekeeperName" id="housekeeperName1" th:text="${sdHouseKeeperBean.housekeeperName}"></textarea>
</div>
</div>
<!--<div class="form-group">-->
<!--<label class="col-sm-2">物品分类:<span style="color:red">*</span></label>-->
<!--<div class="col-xs-3">-->
<!--<select class="form-control" id="articleClassify" name="articleClassify">-->
<!--<option value="">===请选择===</option>-->
<!--<option th:each="qt:${articleClassify}" th:value="${qt.dictkey}"-->
<!--th:text="${qt.dictvalue}"></option>-->
<!--</select>-->
<!--</div>-->
<!--<label class="col-sm-2">物品名称:</label>-->
<!--<div class="col-xs-3">-->
<!--<select class="form-control" name="articleName" id="articleName">-->
<!--<option value="">===请选择===</option>-->
<!--</select>-->
<!--</div>-->
<!--</div>-->
<div class="form-group">
<label class="col-sm-2">物业公司:</label>
<div class="col-xs-9">
<textarea class="form-control" name="propertyCompany" id="propertyCompany" th:text="${sdHouseKeeperBean.propertyCompany}"></textarea>
</div>
</div>
<div class="form-group">
<label class="col-sm-2">联系电话:</label>
<div class="col-xs-9">
<textarea class="form-control" name="telephone" id="telephone" th:text="${sdHouseKeeperBean.telephone}"></textarea>
</div>
</div>
<div class="form-group">
<label class="col-sm-2">服务星级:</label>
<div class="col-xs-3">
<textarea class="form-control" name="serviceStar" id="serviceStar" th:text="${sdHouseKeeperBean.serviceStar}"></textarea>
</div>
</div>
<div class="form-group">
<label class="col-sm-2">服务范围:</label>
<div class="col-xs-3">
<textarea class="form-control" name="range" id="range" th:text="${sdHouseKeeperBean.range}"></textarea>
</div>
</div>
<div class="form-group" style="margin-top:10px;" id="uploadM2">
<label class="col-sm-2">管家头像:</label>
<div class="col-xs-9">
<div id="uploader2" class="uploader" data-ride="uploader">
<div class="uploader-message text-center">
<div class="content"></div>
<button type="button" class="close">×</button>
</div>
<div class="uploader-files file-list file-list-lg" data-drag-placeholder=""></div>
<div class="uploader-actions">
<div class="uploader-status pull-right text-muted"></div>
<!--<button type="button" class="btn btn-link uploader-btn-browse">-->
<!--<i class="icon icon-plus"></i> 选择文件-->
<!--</button>-->
<!--<button type="button" class="btn btn-link uploader-btn-start">-->
<!--<i class="icon icon-cloud-upload"></i> 开始上传-->
<!--</button>-->
</div>
</div>
</div>
</div>
<div style="border:1px solid gray"></div>
<div style="text-align:center;margin-top:10px">
<a class="btn btn-primary" href="javascript:submitForm('saveForm')" id="saveBtn">保存</a>
<a class="btn" data-dismiss="modal" onclick="goBack()">关闭</a>
</div>
</form>
</div>
</body>
<script type="text/javascript" th:inline="javascript">
var saveForm = $("#saveForm");
var action = [[${#httpServletRequest.getParameter("action")}]];
var basePath =;
var attachmentIds =[];
var id = [[${#request.getParameter('id')}]];
$(function () {
initDefaultSettings(action);
loadAttachmentList2();
});
function initDefaultSettings(action) {
if (action == "" || action == "show") {
disableTag("saveForm");
}
if (action == "edit" || action == "add") {
$(".form-date").datetimepicker(
{
language: "zh-CN",
weekStart: 1,
todayBtn: 1,
autoclose: 1,
todayHighlight: 1,
startView: 2,
minView: 0,
minuteStep: 1,
forceParse: 0,
format: 'yyyy-mm-dd hh:ii:ss',
});
}
}
$("#articleClassify").on("change", function () {
var jdbm = $("#articleClassify").val();
$("#articleName").children().not(':eq(0)').remove();
if (jdbm != 0) {
$.ajax({
type: "POST",
url: basePath + "/toolBorrowing/change",
data: {dictKey: jdbm},
datetype: "json",
success: function (data) {
for (var i = 0; i < data.toolBeans.length; i++) {
console.log(data);
$("#articleName").append("<option value='" + data.toolBeans[i].id + "'>" + data.toolBeans[i].name+"(剩余"+data.toolBeans[i].toolNum+")" + "</option>");
}
}
})
}
})
function disableTag(formId) {
var form = document.getElementById(formId)
var inputTags = form.getElementsByTagName("input");
for (i = 0; i < inputTags.length; i++) {
if (inputTags[i].type == "radio" || inputTags[i].type == "text" || inputTags[i].type == "password" || inputTags[i].type == "file") {
inputTags[i].readOnly = true;
}
if (inputTags[i].type == "checkbox") {
inputTags[i].disabled = true;
}
}
var selectTags = form.getElementsByTagName("select");
for (i = 0; i < selectTags.length; i++) {
selectTags[i].disabled = true;
}
var buttonTags = form.getElementsByTagName("button");
for (i = 0; i < buttonTags.length; i++) {
buttonTags[i].remove();
}
var textareaTags = form.getElementsByTagName("textarea");
for (i = 0; i < textareaTags.length; i++) {
textareaTags[i].readOnly = true;
}
$("#saveBtn").remove();
}
function submitForm(formId) {
if (preSubmitCheck()) {
$("#" + formId).ajaxSubmit({
async: false,
success: function (data) {
var msg;
if (data.status) {
$("#saveBtn").hide();
msg = "保存成功";
} else {
msg = "保存失败";
}
layer.msg(msg);
}
});
}
}
function preSubmitCheck() {
var errorMsg = [];
if (saveForm.find("input[name='articleClassify']").val()!=0) {
errorMsg.push("请选择物品分类");
}
if (saveForm.find("input[name='articleName']").val()!=0) {
errorMsg.push("请选择物品");
}
if (saveForm.find("input[name='borrowNum']").val() != "") {
var borrowNum = saveForm.find("input[name='borrowNum']").val();
if(!(/^1[3456789]\d{9}$/.test(borrowNum))){
errorMsg.push("借用数量只能填写整数");
}
}
if (saveForm.find("input[name='startDate']").val() == "") {
errorMsg.push("请输入开始时间");
}
if (saveForm.find("input[name='endDate']").val()=="") {
errorMsg.push("请输入结束时间");
}
if (saveForm.find("input[name='name']").val()=="") {
errorMsg.push("请输入称呼");
}
var showMsg = errorMsg.join("<br/><br/>");
if (showMsg != "") {
layer.msg(showMsg)
}
return errorMsg.length == 0 ? true : false;
}
function goBack() {
var href = [[${#httpServletRequest.getHeader("referer")}]];
if (href == null || href.indexOf("formView") != -1 || href.indexOf("case/track") != -1) {
href = window.location.origin + window.location.pathname + "/../"
}
window.location.href = href;
}
function submitForm() {
if(action=='add'){
if (preSubmitCheck()) {
$.ajax({
type: "POST",
url: "/ljzqksg/toolBorrowing/insertToolBorrowing",
data: $('#saveForm').serialize() + "&attachmentId=" + attachmentIds,
success: function (result) {
alert("保存成功");
$("#saveBtn").hide();
goBack();
},
error: function () {
alert("保存失败");
}
});
}
}else {
if (preSubmitCheck()) {
$.ajax({
type: "POST",
url: "/ljzqksg/toolBorrowing/updateToolBorrowing",
data: $('#saveForm').serialize() + "&attachmentId=" + attachmentIds,
success: function (result) {
alert("保存成功");
$("#saveBtn").hide();
goBack();
},
error: function () {
alert("保存失败");
}
});
}
}
}
function loadAttachmentList2() {
var tablename = 'sd_house_keeper';
var downloadPath = "/files/songdu/" + tablename;
if (action == 'show') {
$.ajax({
type: "POST",
url: basePath + 'api/attachment/list',
data: "id=" + id + "&tablename=" + tablename.toLowerCase(),
async: false,
success: function (msg) {
console.log(msg);
var files = [];
if (msg.status) {
$.each(msg.data, function (key, val) {
var obj = new Object();
obj.name = val.originalName;
obj.size = val.fileSize;
obj.url = downloadPath + "/" + val.fileName;
obj.id = val.id;
files.push(obj);
});
}
var uploaderOptions;
uploaderOptions = {
lang: 'zh_cn',
deleteConfirm: false,
repeat: false,
rename: false,
renameByClick: false,
staticFiles: files,
};
$('#uploader2').uploader(uploaderOptions);
}
});
} else if (action == 'add') {
$('#uploader2').uploader({
autoUpload: false,
chunk_size: 0,
lang: 'zh_cn',
repeat: false,
renameByClick: false,
rename: false,
filters:{
mime_types: [
{title: '图片', extensions: 'jpg,gif,png'},
{title: '图标', extensions: 'ico'}
]
},
url: basePath + 'api/attachment/upload?tablename='+ tablename.toLowerCase(),
responseHandler: function (responseObject, file) {
var obj = eval("(" + responseObject.response + ")");
var msg;
if (obj.status) {
msg = "成功上传";
attachmentIds.push(obj.data.id);
} else {
msg = "上传失败";
}
layer.msg(msg);
}
});
} else {
$.ajax({
type: "POST",
url: basePath + 'api/attachment/list',
data: "id=" + id + "&tablename=" + tablename.toLowerCase(),
async: false,
success: function (msg) {
var files = [];
if (msg.status) {
$.each(msg.data, function (key, val) {
var obj = new Object();
obj.name = val.originalName;
obj.size = val.fileSize;
obj.url = downloadPath + "/" + val.fileName;
obj.id = val.id;
obj.attachmentId=val.id;
files.push(obj);
});
}
var uploaderOptions;
uploaderOptions = {
lang: 'zh_cn',
deleteConfirm: true,
repeat: false,
staticFiles: files,
renameByClick: false,
rename: false,
autoUpload: false,
chunk_size: 0,
filters:{
mime_types: [
{title: '图片', extensions: 'jpg,gif,png'},
{title: '图标', extensions: 'ico'}
]
},
url: basePath + 'api/attachment/upload?tablename='+ tablename.toLowerCase(),
responseHandler: function (responseObject, file) {
var obj = eval("(" + responseObject.response + ")");
var msg;
if (obj.status) {
msg = "成功上传";
attachmentIds.push(obj.data.id);
file.attachmentId = obj.data.id;
} else {
msg = "上传失败";
}
layer.msg(msg);
},
deleteActionOnDone: function (file, doRemoveFile) {
$.ajax({
type: "POST",
url: basePath + 'sdRotationChart/deleteFile',
data: "enclosureId=" + id + "&attachmentId=" + file.attachmentid,
async: false,
success: function (msg) {
if (msg.status) {
doRemoveFile();
} else {
layer.msg("删除失败,请重试");
}
}
});
},
};
$('#uploader2').uploader(uploaderOptions);
}
});
}
}
</script>
</html>