#include "stdio.h" #include "stdlib.h" #define ResMax 8 //资源的最大数目 #define ProMax 10 //进程的最大数目 int Available[ResMax];//可利用资源向量 int Max[ProMax][ResMax];//最大需求矩阵 int Allocation[ProMax][ResMax];//分配矩阵 int Need[ProMax][ResMax];//需求矩阵 int m;//用户输入的资源数目 int n;//用户输入的进程数目 int Request[ResMax];//进程的请求向量 int Work[ResMax];//安全性检查中的工作向量 int Finish[ProMax];//表示系统是否有足够的资源分配给进程 void show(void); void ToAllocate(int i); void nonAllocate(int i); int condition(void); int isSafe(void); int BankerAlgorithm(int i); void main(void) { int i,j,k,t; int safe=1; printf("Please input the number of resources(0~%d):",ResMax); scanf("%d",&m); printf("/nPlease input the number of processes(0~%d):",ProMax); scanf("%d",&n); printf("/nPlease input how many resources available:"); for(j=0;j<m;j++) { printf("/nResource %d:",j); scanf("%d",&Available[j]); } printf("/nPlease input the processes' max need of resource!"); for(i=0;i<n;i++) for(j=0;j<m;j++) { printf("/nProcess %d max need of Resource %d:",i,j); scanf("%d",&Max[i][j]); } do{ if(safe==0) { printf("The system is unsafe,please re-enter the allocation!"); safe=1; } printf("/nPlease input allocation:"); for(i=0;i<n;i++) for(j=0;j<m;j++) { printf("/nProcess %d has Resource %d :",i,j); scanf("%d",&Allocation[i][j]); } for(i=0;i<n;i++) for(j=0;j<m;j++) Need[i][j]=Max[i][j]-Allocation[i][j]; show(); }while((safe=isSafe())==0); do{ printf("/nPlease input request:"); printf("/nplease input request Process(0~%d):",n); scanf("%d",&i); printf("/nPlease input requested Resource number:"); for(j=0;j<m;j++) { printf("/nProcess %d request Reource %d:",i,j); scanf("%d",&Request[j]); } t=BankerAlgorithm(i); if(t==-1) printf("/nError:Requested Resource number> available,please wait!"); else if(t==-2) printf("/nError:Requested Resource number> process need!"); else if(t==1) { printf("/nAllocation Finished!"); show(); } else if(t==0) { printf("/nSystem is unsafe,request denied!"); show(); } printf("/nenter 0 to exit,other number to continue!"); scanf("%d",&k); if(k==0) exit(0); }while(1); } void show(void) {//显示资源分配情况 int i,j; printf("/n********Max******Allocation***Need******"); printf("/nProcess"); for(i=0;i<3;i++) for(j=0;j<m;j++) printf(" R%d",j); for(i=0;i<n;i++) { printf("/n P%d: ",i); for(j=0;j<m;j++) printf("%-3d",Max[i][j]); for(j=0;j<m;j++) printf("%-3d",Allocation[i][j]); for(j=0;j<m;j++) printf("%-3d",Need[i][j]); } printf("/nAvailable:"); for(j=0;j<m;j++) printf("R%d:%d ",j,Available[j]); } void ToAllocate(int i) {//试探分配 int j; for(j=0;j<m;j++) { Available[j]=Available[j]-Request[j]; Allocation[i][j]=Allocation[i][j]+Request[j]; Need[i][j]=Need[i][j]-Request[j]; } } void nonAllocate(int i) {//上次试探分配作废 int j; for(j=0;j<m;j++) { Available[j]=Available[j]+Request[j]; Allocation[i][j]=Allocation[i][j]-Request[j]; Need[i][j]=Need[i][j]+Request[j]; } } int BankerAlgorithm(int i) {//银行家算法 int j; for(j=0;j<m;j++) { if(Request[j]>Need[i][j]) return -2; if(Request[j]>Available[j]) return -1; } ToAllocate(i); if(isSafe()==0) { nonAllocate(i); return 0; } return 1; } int isSafe(void) {//安全性检查 int i,j; for(j=0;j<m;j++) Work[j]=Available[j]; for(i=0;i<n;i++) Finish[i]=0; printf("/nSecurity sequence:"); while(1) { if((i=condition())!=-1) { for(j=0;j<m;j++) Work[j]=Work[j]+Allocation[i][j]; Finish[i]=1; printf("P%d ",i); } else { for(i=0;i<n;i++) if(Finish[i]==0) return 0; return 1; } } } int condition(void) {//进程集合中查找的条件 int i,t,j; for(i=0;i<n;i++) { if(Finish[i]==0) { t=0; for(j=0;jWork[j]) {t=1;break;} if(t==0) return i; } } return -1; }
银行家算法
最新推荐文章于 2023-11-02 09:04:46 发布
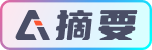