一,分块功能代码展示
1.三元运算符实现点赞、收藏状态转换
this.setData({
imgUrl: souchang ? "../../images/shoucang-no.png" : "../../images/shoucang-yes.png"
})
souchang = !souchang
2.主界面携带数据跳转至详情页
<view class="itemRoot" bindtap="goDetail" data-item="{{item}}">
- 主界面接收到前端传来的数据,将数据加在页面跳转参数中将数据传输到详情页
goDetail(event) {
console.log("点击获取的数据", event.currentTarget.dataset.item._id)
wx.navigateTo({
url: '/pages/detail/detail?id=' + event.currentTarget.dataset.item._id,
})
}
- 在详情页中,通过访问event的属性来获取主界面传来的数据,从而进行后续详情页操作
onLoad(options) {
ID = options.id
console.log("详情接受的id", ID)
wx.cloud.database().collection("homelist")
.doc(ID)
.get()
.then(res =>{})
.catch(err =>{})
二,整体代码
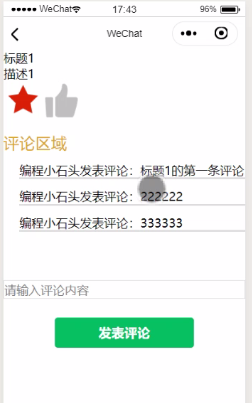
1.复合云函数代码
- 微信小程序限制一个小程序最多只能拥有25个云函数,所以在进行一些共性的操作时,尽量使用if-else来进行函数复用,以此来减少云函数数量的消耗。
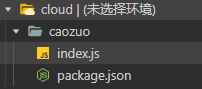
const cloud = require('wx-server-sdk')
cloud.init({
env: cloud.DYNAMIC_CURRENT_ENV
})
exports.main = async(event, context) => {
if (event.action == 'souchang') {
return await cloud.database().collection("homelist").doc(event.id)
.update({
data: {
souchang: event.souchang
}
})
.then(res => {
console.log("改变收藏状态成功", res)
return res
})
.catch(res => {
console.log("改变收藏状态失败", res)
return res
})
} else if (event.action == 'fabiao') {
return await cloud.database().collection("homelist").doc(event.id)
.update({
data: {
pinglun: event.pinglun
}
})
.then(res => {
console.log("评论成功", res)
return res
})
.catch(res => {
console.log("评论失败", res)
return res
})
} else {
return await cloud.database().collection("homelist").doc(event.id)
.update({
data: {
dianzan: event.dianzan
}
})
.then(res => {
console.log("改变点赞状态成功", res)
return res
})
.catch(res => {
console.log("改变点赞状态失败", res)
return res
})
}
}
2.主界面代码
Page({
data: {
datalist: []
},
onLoad() {
wx.cloud.database().collection('homelist')
.get()
.then(res => {
console.log("获取成功", res)
this.setData({
datalist: res.data
})
})
.catch(res => {
console.log("获取失败", res)
})
},
goDetail(event) {
console.log("点击获取的数据", event.currentTarget.dataset.item._id)
wx.navigateTo({
url: '/pages/detail/detail?id=' + event.currentTarget.dataset.item._id,
})
}
})
<block wx:for="{{datalist}}" wx:key="index">
<view class="itemRoot" bindtap="goDetail" data-item="{{item}}">
<text>{{item.title}}</text>
<text>{{item.desc}}</text>
</view>
</block>
.itemRoot{
border-bottom: 1px solid gainsboro;
margin: 15rpx;
}
3.详情页代码
let ID = ''
let souchang = false
let dianzan = false
Page({
data: {
detail: '',
imgUrl: "../../images/shoucang-no.png",
dianzanUrl: "../../images/dianzan-no.png",
pinglun: [],
content: ''
},
onLoad(options) {
ID = options.id
console.log("详情接受的id", ID)
wx.cloud.database().collection("homelist")
.doc(ID)
.get()
.then(res => {
console.log("详情页成功", res)
souchang = res.data.souchang
dianzan = res.data.dianzan
this.setData({
detail: res.data,
imgUrl: souchang ? "../../images/shoucang-yes.png" : "../../images/shoucang-no.png",
dianzanUrl: dianzan ? "../../images/dianzan-yes.png" : "../../images/dianzan-no.png",
pinglun: res.data.pinglun
})
})
.catch(res => {
console.log("详情页失败", res)
})
},
clickMe() {
this.setData({
imgUrl: souchang ? "../../images/shoucang-no.png" : "../../images/shoucang-yes.png"
})
souchang = !souchang
wx.cloud.callFunction({
name: "caozuo",
data: {
action: "souchang",
id: ID,
souchang: souchang
}
}).then(res => {
console.log("改变收藏状态成功", res)
})
.catch(res => {
console.log("改变收藏状态成功", res)
})
},
clickMe2() {
this.setData({
dianzanUrl: dianzan ? "../../images/dianzan-no.png" : "../../images/dianzan-yes.png"
})
dianzan = !dianzan
wx.cloud.callFunction({
name: "caozuo",
data: {
action: "dianzan",
id: ID,
dianzan: dianzan
}
}).then(res => {
console.log("小程序改变点赞状态成功", res)
})
.catch(res => {
console.log("小程序改变点赞状态成功", res)
})
},
getContent(event) {
this.setData({
content: event.detail.value
})
},
fabiao() {
let content = this.data.content
if (content.length < 4) {
wx.showToast({
icon: "none",
title: '评论太短了',
})
return
}
let pinglunItem = {}
pinglunItem.name = '编程小石头'
pinglunItem.content = content
let pinglunArr = this.data.pinglun
pinglunArr.push(pinglunItem)
console.log("添加后的评论数组", pinglunArr)
wx.showLoading({
title: '发表中。。。',
})
wx.cloud.callFunction({
name: "caozuo",
data: {
action: "fabiao",
id: ID,
pinglun: pinglunArr
}
}).then(res => {
console.log("发表成功", res)
this.setData({
pinglun: pinglunArr,
content: ''
})
wx.hideLoading()
}).catch(res => {
console.log("发表失败", res)
wx.hideLoading()
})
}
})
<!-- 标题和描述 -->
<view>
<view>{{detail.title}}</view>
<view>{{detail.desc}}</view>
</view>
<!-- 点赞和收藏 -->
<image class="image" src="{{imgUrl}}" bindtap="clickMe"></image>
<image class="image" src="{{dianzanUrl}}" bindtap="clickMe2"></image>
<!-- 评论 -->
<view class="tip">评论区域</view>
<block wx:for="{{pinglun}}" wx:key="index">
<view class="pinglunItem">
<text>{{item.name}}发表评论:</text>
<text>{{item.content}}</text>
</view>
</block>
<!-- 发表评论 -->
<input class="input" bindinput="getContent" placeholder="请输入评论内容" value="{{content}}"></input>
<button type="primary" bindtap="fabiao">发表评论</button>
.image {
width: 120rpx;
height: 120rpx;
}
.tip {
margin-top: 30rpx;
font-size: 50rpx;
color: goldenrod;
}
.pinglunItem {
border-bottom: 2px solid gainsboro;
margin-left: 50rpx;
margin-top: 30rpx;
}
.input {
border: 1px solid gainsboro;
margin-top: 150rpx;
margin-bottom: 60rpx
}