**公司最近用WebService获取数据,数据格式是xml的,所以需要将xml转换成对象 下面是初步对XStream的一些学习使用!**
**程序需要两个jar包 分别是**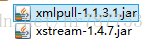
**运行Main类 有几个方法 根据方法名和注释你能很容易的找到你想要的**
package com.xstream.demo;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.List;
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.io.xml.DomDriver;
public class Demo {
public static void main(String[] args) throws Exception {
/*//对象转换成xml字符串
String xml = beanToXml();
//对象转换成xml文件
creatXMlFile(new File("1.xml"));
//xml字符串转换成对象
xmlToBean(xml);*/
//xml文件转换成对象
// xmlToBean(new File("1.xml"));
// List2Xml();
// creatXMlFile(new File("2.xml"));
xmlToList(new File("2.xml"));
}
/**
* 将xml字符串转换成对象 *@param xml */ public static void xmlToBean(String xml){ XStream xStream = new XStream(new DomDriver());//注解生效 xStream.processAnnotations(new Class[]{Student.class}); //将xml字符串转换成对象 Student student = (Student)xStream.fromXML(xml); System.out.println(student); } /** *将xml文件转换成对象 *@param xmlFile */ public static void xmlToBean(File xmlFile) {XStream xStream=new XStream(new DomDriver());xStream.processAnnotations(new Class[]{Student.class});Student student = (Student)xStream.fromXML(xmlFile);System.out.println(student);} /** *对象转换成xml字符串 *@return */ private static String beanToXml() {//定义bean Student student=new Student();student.setName("张三");student.setAge("18"); student.setSex("男"); List<StudentInfo> stuInfoList = new ArrayList<StudentInfo>(); stuInfoList.add(new StudentInfo("157","山西")); stuInfoList.add(new StudentInfo("158","河南")); student.setStuInfoList(stuInfoList); //创建XStream对象 XStream stream=new XStream();
// 注册使用了注解的Student类
stream.processAnnotations(new Class[]{Student.class});
//转换为xml(默认格式化)
String xml=stream.toXML(student);
return xml;
}
/**
* 对象转换成xml文件 *@param file *@throws IOException */ private static void creatXMlFile(File file) throws IOException {String xml=List2Xml();String xmlContent="<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n";if (!file.exists()) {file.getParentFile().mkdirs();
// file.createNewFile();
}
xmlContent+=xml;
//可以将xml字符串写入文件,要创建新文件,必须保证其父路径存在哦,单级目录自动创建文件
PrintWriter pw=new PrintWriter(file);
pw.write(xmlContent);
pw.close();
//如果要实现追加
// BufferedWriter bw=new BufferedWriter(new FileWriter(file ,true));
//那么此时必须保证xml头部申明只能一次
/* if (file.length()==0) {
xmlContent+=xml;
}else{
xmlContent=xml;
}*/
}
/**
* 将List转换成xml */ public static String List2Xml(){ List<Student> stuList = new ArrayList<Student>(); Student s1 = new Student(); s1.setName("何"); s1.setAge("18"); s1.setSex("男"); List<StudentInfo> stuInfoList = new ArrayList<StudentInfo>(); stuInfoList.add(new StudentInfo("1111","山西")); stuInfoList.add(new StudentInfo("2222","北京")); s1.setStuInfoList(stuInfoList);Student s2 = new Student(); s2.setName("王"); s2.setAge("18"); s2.setSex("女"); stuInfoList = new ArrayList<StudentInfo>(); stuInfoList.add(new StudentInfo("3333","太原")); stuInfoList.add(new StudentInfo("4444","运城")); s2.setStuInfoList(stuInfoList);Student s3 = new Student(); s3.setName("hah"); s3.setAge("18"); s3.setSex("女"); stuInfoList = new ArrayList<StudentInfo>(); stuInfoList.add(new StudentInfo("9999","aaaa")); stuInfoList.add(new StudentInfo("8888","bbbb")); s3.setStuInfoList(stuInfoList);stuList.add(s1); stuList.add(s2); stuList.add(s3);XStream stream = new XStream();
// 注册使用了注解的Student类
stream.processAnnotations(new Class[]{Student.class});
//转换为xml(默认格式化)
String xml=stream.toXML(stuList);
System.out.println(xml);
return xml;
}
/**
* 将xml文件转换成对象 *@param xmlFile */ public static void xmlToList(File xmlFile) {XStream xStream=new XStream(new DomDriver());xStream.processAnnotations(new Class[]{Student.class});List<Student> stuList = (List<Student>) xStream.fromXML(xmlFile); System.out.println(stuList); }
}
**实体类 Student**
package com.xstream.demo;
import java.util.ArrayList;
import java.util.List;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import com.thoughtworks.xstream.annotations.XStreamImplicit;
@XStreamAlias("student")
public class Student {
private String name; private String age;private String sex;@XStreamImplicit private List<StudentInfo> stuInfoList;public List<StudentInfo> getStuInfoList() { return stuInfoList; } public void setStuInfoList(List<StudentInfo> stuInfoList) { this.stuInfoList = stuInfoList; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAge() { return age; } public void setAge(String age) { this.age = age; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } @Override public String toString() { return "Student [name=" + name + ", age=" + age + ", sex=" + sex + ", stuInfoList=" + stuInfoList + "]"; }
}
**实体类Student的详细附加类 也是一个实体类StudentInfo**
package com.xstream.demo;
import com.thoughtworks.xstream.annotations.XStreamAlias;
@XStreamAlias("studentInfo")
public class StudentInfo {
private String phone; private String address;public StudentInfo(String phone,String address){ this.phone = phone; this.address = address; }public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } @Override public String toString() { return "StudentInfo [phone=" + phone + ", address=" + address + "]"; }
}
**紧接着 通过代码生成的两个xml文件**
1.<?xml version="1.0" encoding="UTF-8"?>
<student>
<name>张三</name>
<age>18</age>
<sex>男</sex>
<studentInfo>
<phone>157</phone>
<address>山西</address>
</studentInfo>
<studentInfo>
<phone>158</phone>
<address>河南</address>
</studentInfo>
<studentInfo>
<phone>000</phone>
<address>北京</address>
</studentInfo>
</student>
**第二个xml**<?xml version="1.0" encoding="UTF-8"?>
<list>
<student>
<name>何睿</name>
<age>18</age>
<sex>男</sex>
<studentInfo>
<phone>1111</phone>
<address>山西</address>
</studentInfo>
<studentInfo>
<phone>2222</phone>
<address>北京</address>
</studentInfo>
</student>
<student>
<name>王昱辉</name>
<age>18</age>
<sex>女</sex>
<studentInfo>
<phone>3333</phone>
<address>太原</address>
</studentInfo>
<studentInfo>
<phone>4444</phone>
<address>运城</address>
</studentInfo>
</student>
<student>
<name>hah</name>
<age>18</age>
<sex>女</sex>
<studentInfo>
<phone>9999</phone>
<address>aaaa</address>
</studentInfo>
<studentInfo>
<phone>8888</phone>
<address>bbbb</address>
</studentInfo>
</student>
</list>
XStream的基础学习使用
最新推荐文章于 2022-03-02 20:07:25 发布