8_2_array_vector.cpp
#include "hjcommon.hpp"
class A
{
public:
int m_num;
A(int num) : m_num(num) { cout << "one arg. " << m_num << endl; }
A(const A &a) : m_num(a.m_num) { cout << "copy. " << m_num << endl; }
~A() { cout << "destructor." << endl; }
};
int main_2_8_2(int argc, char *argv[])
{
// c++标准库包含STL标准模板库,STL模板库组成:容器、迭代器、算法(函数)、分配器、其他
// 容器分三大类:
// 顺序容器(sequence):array、vector、deque、list(双向链表)、forward_list(单向链表)
// 关联容器(Associative):键值对 set、multiset、map(元素key值各不相同)、multimap(key值可相同)、hash_set、hash_multiset、hash_map、hash_multimap
// 无序容器(c++11):也属于关联容器的一种, unordered_set、unordered_multiset、unordered_map、unordered_multimap
// array 数组,大小是固定的,内存地址连续
array<string, 5> arr = {"hj", "tyj", "ly"};
// vector 内存也是连续的,但是每次插入的时候可能都会重新构造vector,所有元素都会重新拷贝一次。。 删除的时候可能不会重新构造所有元素。
vector<A> vec;
// vec.reserve(10); // 预留尺寸大小
for(int i=0; i<5; ++i)
vec.push_back(A(i));
sleep(1min);
return 0;
}
8_3_deque_stack_queue_list_map_set.cpp
#include "hjcommon.hpp"
#include <queue>
#include <stack>
#include <list>
#include <forward_list>
#include <map>
#include <unordered_set>
#include <algorithm> // STL算法需要
class A
{
public:
int m_num;
A(int num) : m_num(num) { cout << "one arg. " << m_num << endl; }
A(const A &a) : m_num(a.m_num) { cout << "copy. " << m_num << endl; }
~A() { cout << "destructor." << endl; }
};
int main_2_8_3(int argc, char *argv[])
{
// deque(双端队列) 非完全连续内存,是分段连续内存
cout << "---------------deque-----------------" << endl;
deque<A> deq;
for(int i=0; i<6; ++i)
{
if (i<3) deq.push_back(A(i));
else deq.push_front(A(i));
}
// deq.insert()
// deq.front()
// deq.back()
// deq.pop_front() // back
// deq.erase()
// deq.clear()
// deq.at(1).m_num // 获取中间元素
// stack 非连续内存
cout << "---------------stack-----------------" << endl;
stack<A> sta;
for(int i=0; i<5; ++i)
sta.push(A(i));
// sta.top()
// sta.pop() // 没有 erase 和 clear
// queue 单向队列,非连续内存
cout << "---------------queue-----------------" << endl;
queue<A> que;
for(int i=0; i<5; ++i)
que.push(A(i));
// que.front()
// que.pop() // 没有 erase 和 clear
// list 双向链表,非连续内存
cout << "---------------list-----------------" << endl;
list<A> ls;
for(int i=0; i<6; ++i)
{
if (i<3) ls.push_back(A(i));
else ls.push_front(A(i));
}
// ls.insert()
// ls.front()
// ls.back()
// ls.pop_front() // back
// ls.remove(A(0));
// ls.erase()
// ls.clear()
// list<A>::iterator ite = std::find(ls.begin(), ls.end(), A(0)); // 查找某个元素,没有的话返回第二个参数 ls.end()
// forward_list 单向链表,c++11,非连续内存
cout << "---------------forward_list-----------------" << endl;
forward_list<A> fls;
for(int i=0; i<5; ++i)
fls.push_front(A(i));
// fls.insert_after()
// fls.pop_front()
// fls.remove(A(0))
// fls.erase_after()
// fls.clear()
// map 内部实现多为红黑树,非连续内存
cout << "---------------map-----------------" << endl;
map<int, string> map1; // 不要将变量命名为map,可能会导致莫名其妙的编译错误
map1.insert(std::make_pair(0, "hj")); // 需要使用 pair 类型插入,可直接构造也可使用 make_pair 函数
map1.insert(pair<int, string>(1, "tyj"));
map1.insert(pair<int, string>(1, "ly")); // 插入时,如果有重复key值,那么不会插入
cout << map1.at(1) << endl; // tyj ,map.at(key) 获取对应key的value值,若key不存在会抛宕。。
auto iter = map1.find(3); // 根据key查找,若未找到返回 map.end()
if (iter!=map1.end()) cout << "key=" << iter->first << ", value=" << iter->second << endl; // first表示key值,second表示value值
else cout << "map.find == nullptr" << endl;
// 遍历map
for (auto it=map1.begin(), end=map1.end(); it!=end; ++it)
{
cout << "key=" << it->first << ", value=" << it->second << endl;
}
string str = map1[1]; // 由key值获取value, map1[1] 中的 1 不是下标。而是key值
cout << "str : " << str << endl;
map<int, A> mmp;
mmp.insert(make_pair(0, A(0)));
mmp.insert(make_pair(1, A(1)));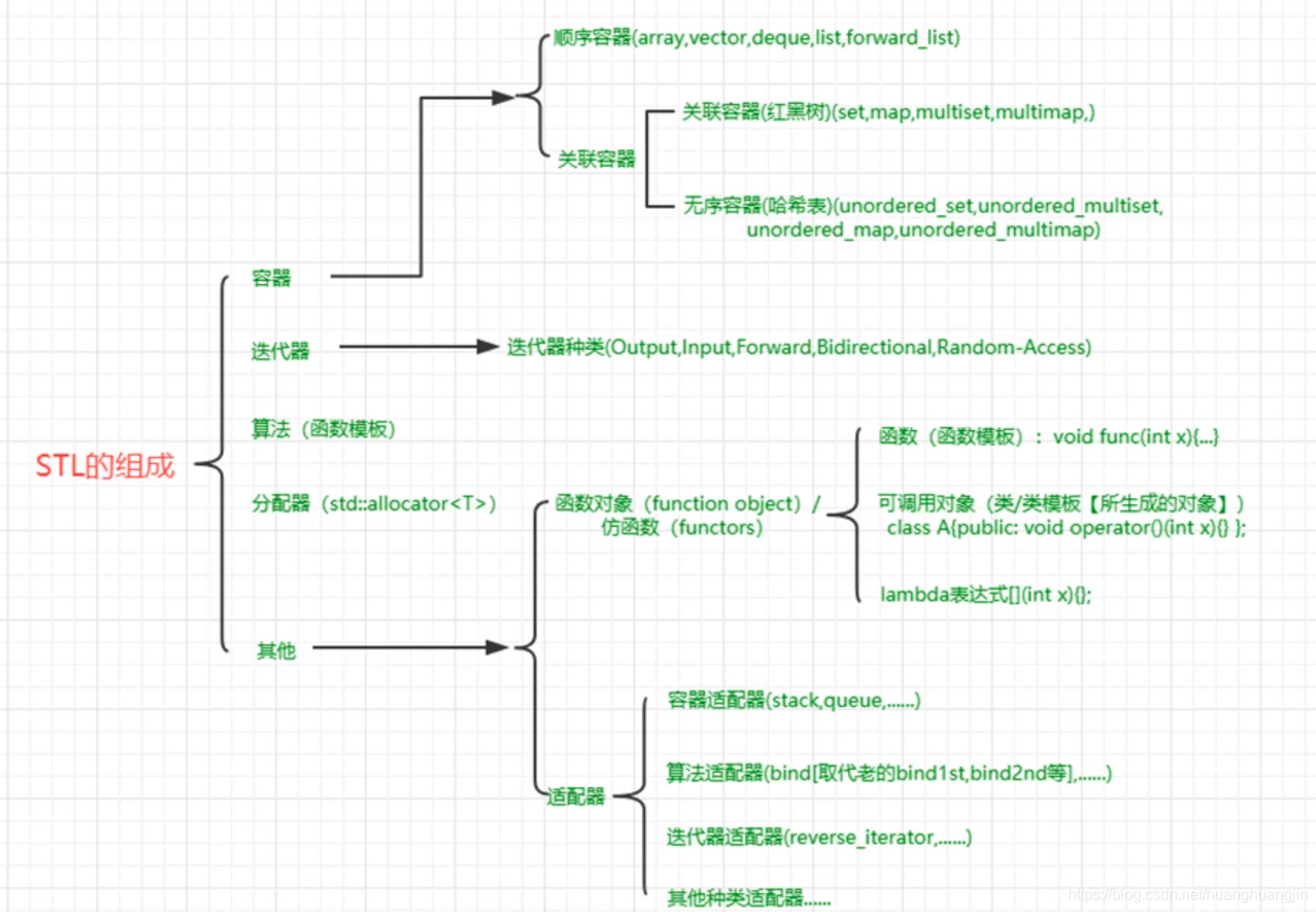
mmp.insert(pair<int, A>(2, A(2)));
// set 元素值不能重复
// unordered_set
cout << "---------------unordered_set-----------------" << endl;
unordered_set<int> us;
cout << us.bucket_count() << endl; // 篮子长度
us.insert(0);
sleep(1min);
return 0;
}
STL组成
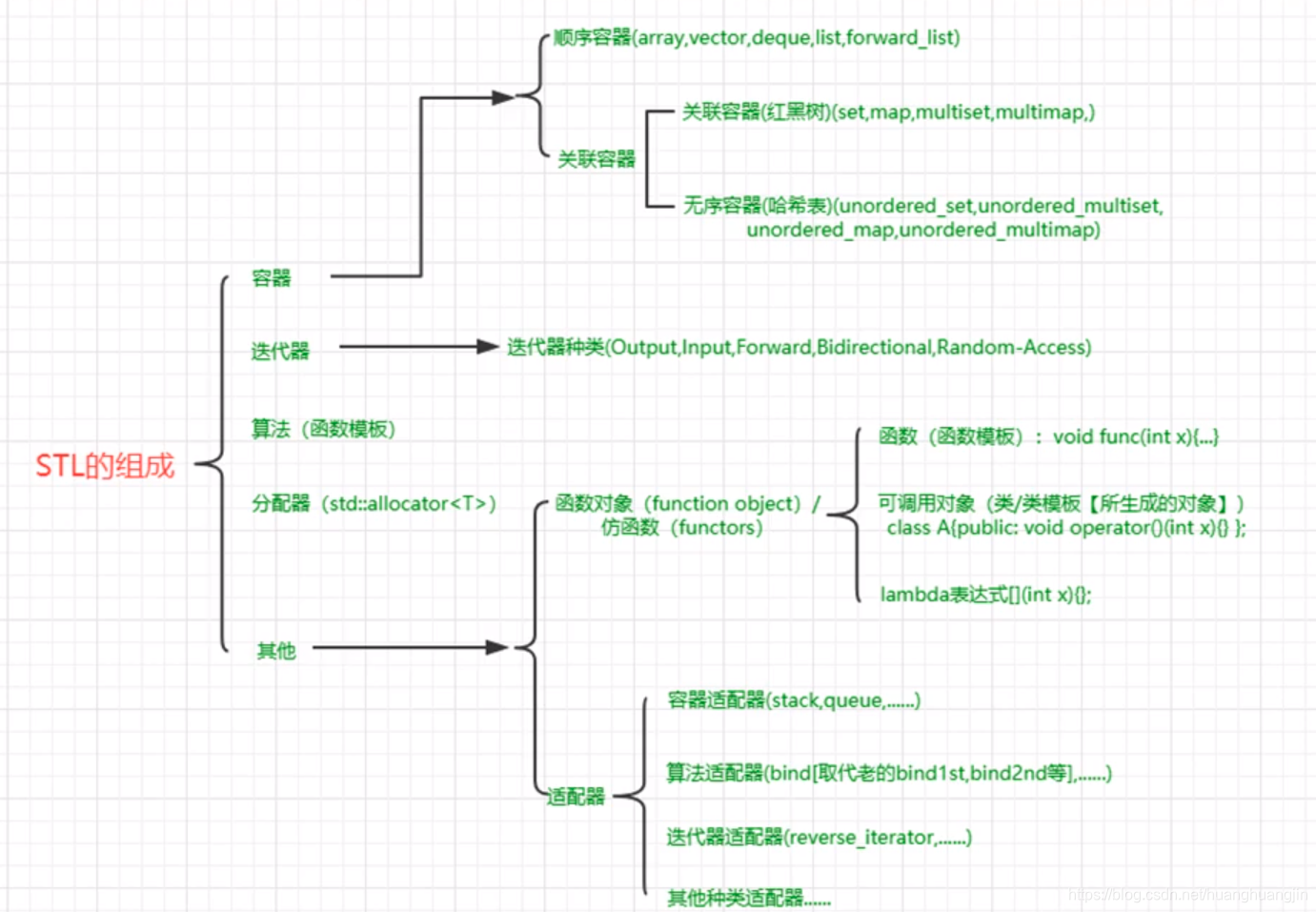