1.什么是阻塞队列?
当队列是空的,从队列中获取元素的操作将会被阻塞
当队列是满的,从队列中添加元素的操作将会被阻寨
试图从空的队列中获取元素的线程将会被隆塞,直到其他线程往空的队列插入新的元素
试图向已满的队列中添加新元素的线程将会被阻塞,直到其他线程从队列中移除一个或多个元素或者完全清空,使队列变得空闲起来并后续新增
2.阻塞队列的用处?
在多线程领域:所谓阻塞,在某些情况下会挂起线程(即阻塞),一旦条件满足,被挂起的线程又会自动被唤起
为什么需要BlockingQueue?
好处是我们不需要关心什么时候需要阻塞线程,什么时候需要唤醒线程,因为这一切BlockingQueue都给你一手包办了
在concurrent包发布以前,在多线程环境下,我们每个程序员都必须去自己控制这些细节,尤其还要兼顾效率和线程安全,而这会给我们的程序带来不小的复杂度。
架构:
Blockingqueue的七个实现类:
3.核心方法:
抛出异常组:
add()方法:超过阻塞队列长度时会抛异常:
remove()方法:移除超过阻塞队列长度时会抛异常:
element()方法:检测元素是否存在
特殊值组:
offer()方法:添加元素超出长度会返回FALSE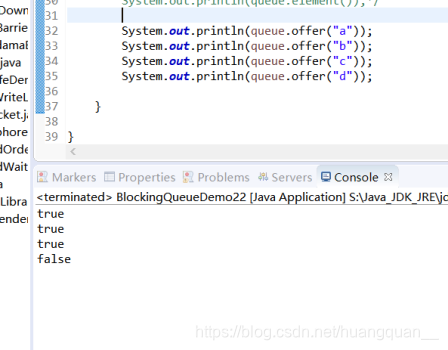
poll():取出元素超过队列长队的话返回null
阻塞组:
put():添加元素时,如果队列已满,则会一直阻塞:
:取元素时,如果队列为空,则会一直阻塞
有限期阻塞组:
offer(“d”,3L,TimeUnit.SECONDS):添加元素超过队列大小时,会进行有限时间阻塞(等待),时间一到,返回false
poll(3L, TimeUnit.SECONDS):取出元素如果队列为空,会进行有限时间阻塞(等待),时间一到,返回null
完整测试代码:
package JUCTest;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.TimeUnit;
public class BlockingQueueDemo22 {
public static void main(String[] args) throws Exception {
// List<Object> list = new ArrayList<>();
BlockingQueue<String> queue = new ArrayBlockingQueue<>(3);
/* System.out.println(queue.add("a"));
System.out.println(queue.add("b"));
System.out.println(queue.add("c"));
System.out.println(queue.add("d"));//添加超过阻塞队列长度时会抛异常:
System.out.println(queue.remove());
System.out.println(queue.remove());
System.out.println(queue.remove());
System.out.println(queue.remove());//移除超过阻塞队列长度时会抛异常:
*/
/*System.out.println(queue.add("a"));
System.out.println(queue.add("b"));
System.out.println(queue.element());*/
/*
System.out.println(queue.offer("a"));
System.out.println(queue.offer("b"));
System.out.println(queue.offer("c"));
// System.out.println(queue.offer("d"));//添加元素超出长度会返回FALSE
System.out.println(queue.poll());
System.out.println(queue.poll());
System.out.println(queue.poll());
System.out.println(queue.poll());//取出元素超过队列长队的话返回null
*/
// queue.put("a");
// queue.put("b");
// queue.put("c");
// queue.put("d");//添加元素时,如果队列已满,则会一直阻塞:
/* System.out.println(queue.take());
System.out.println(queue.take());
System.out.println(queue.take());
System.out.println(queue.take());//取元素时,如果队列为空,则会一直阻塞
*/
System.out.println(queue.offer("a"));
System.out.println(queue.offer("b"));
System.out.println(queue.offer("c"));
// System.out.println(queue.offer("d",3L,TimeUnit.SECONDS));//添加元素超过队列大小时,会进行有限时间阻塞(等待),
//时间一到,返回false
System.out.println(queue.poll());
System.out.println(queue.poll());
System.out.println(queue.poll());
System.out.println(queue.poll(3L, TimeUnit.SECONDS));//取出元素如果队列为空,会进行有限时间阻塞(等待),
//时间一到,返回null
}
}