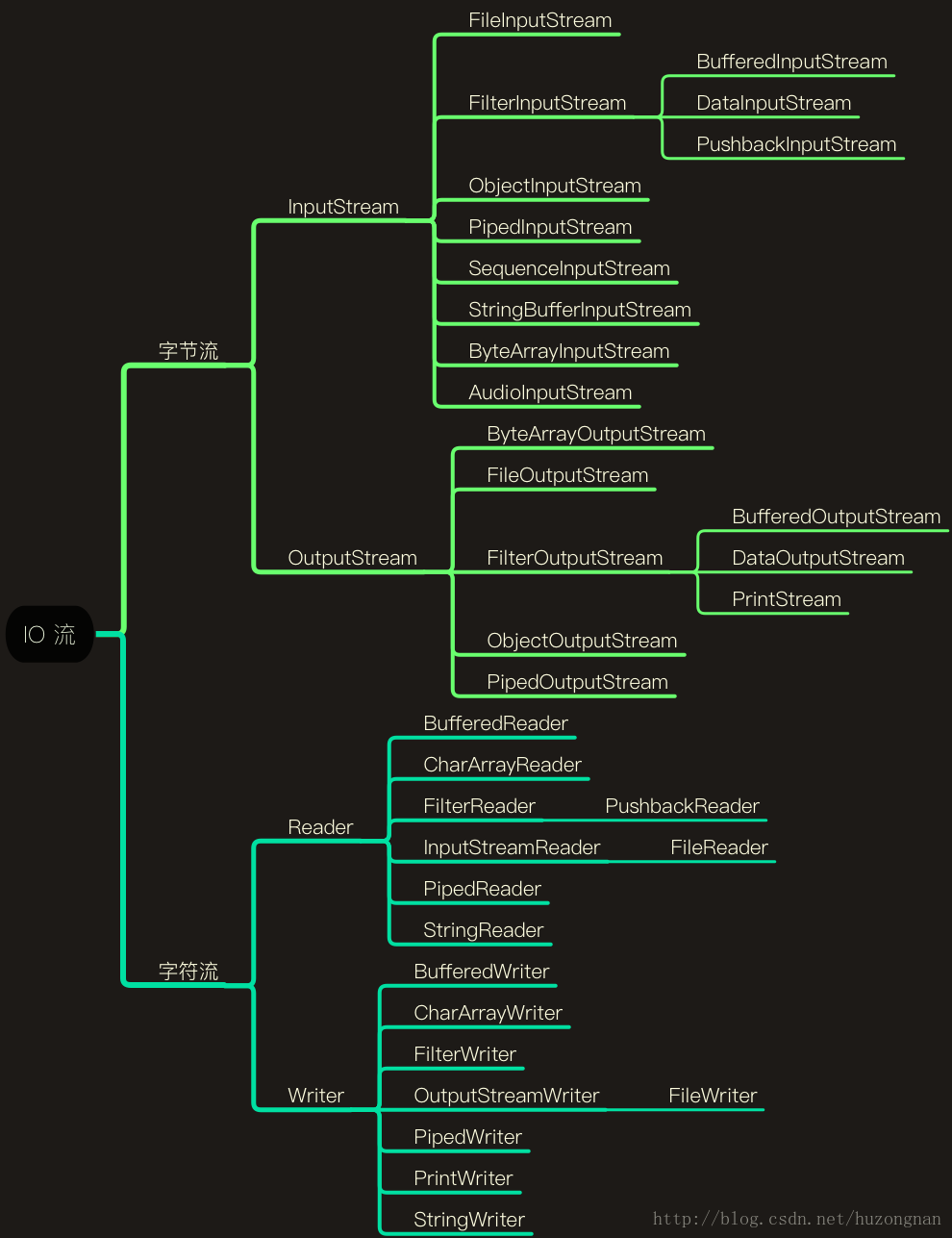
字符流
字符流
一个字符一个字符的读
注意:只能用来操作文本(不能写图片、音频、视频)
Writer(所有字符输出流的父类,是抽象类)
FileWirter
构造方法(绑定写入的路径):
文件
字符串
mac 系统下,一个中文字符占3个字节
默认使用 UTF-8的编码表(通用的编码表)
Windows 系统下,一个中文字符占2个字节
默认使用 GBK 编码表(简体中文)
FileWriter fw = new FileWriter("/Users/Desktop/Test/test.txt");
fw.write(100);
// 注意:字符输出流,在写入文件的时候
// 需要调用刷新方法
// 建议:每次写入,最好都刷新一次
fw.flush();
char[] c = {'l', 'o', 'n', 'g'};
fw.write(c);
fw.flush();
// 获取 char 类型中的字节
fw.write(c, 1, 3);
fw.flush();
// 使用字符串直接写入
fw.write("hello");
fw.flush();
fw.write("\n床前明月光\n");
fw.write("疑是地上霜\n");
fw.write("举头望明月\n");
fw.write("低头思故乡\n");
fw.flush();
// 输入字符串,获取其中的字符
fw.write("白日依山尽", 1, 2);
fw.flush();
// 关闭资源前,先刷新
fw.close();
字符输入流
字符输入流
Reader(所有字符输入流的父类,抽象类)
写的时候可以直接写入字符串
读的时候不能,字符串很难界定到哪里结束,不太容易判断一个字符串
单字节读取
FileReader fr = new FileReader("/Users/Desktop/Test/test.txt");
int len;
while ((len = fr.read()) != -1) {
System.out.println((char)len);
}
fr.close();
字符数组读取
FileReader fr = new FileReader("/Users/Desktop/Test/test.txt");
int len;
char[] c = new char[1024];
while ((len = fr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
fr.close();
/*
* 利用字符流,复制文件(带异常处理)
*/
public class Demo {
public static void main(String[] args) {
copyDir();
}
public static void copyDir() {
FileReader fr = null;
FileWriter fw = null;
try {
fr = new FileReader("/Users/Desktop/Test/test.txt");
fw = new FileWriter("/Users/Desktop/XTest/test.txt");
char[] c = new char[1024];
int len = 0;
while ((len = fr.read(c)) != -1) {
fw.write(c, 0, len);
fw.flush();
}
} catch (FileNotFoundException e) {
throw new RuntimeException("文件路径有误,无法找到该文件!");
} catch (IOException e) {
throw new RuntimeException("文件读取失败!");
} finally {
try {
if (fr != null) {
fr.close();
}
} catch (IOException e) {
throw new RuntimeException("资源关闭失败!");
} finally {
try {
fw.close();
} catch (Exception e2) {
throw new RuntimeException("资源关闭失败!");
}
}
}
}
}
转换流
转换流
OutputStreamWriter(字符流转向字节流)
作用:可以根据不同编码格式写入
需要使用到 FileOutputStream 类
InputStreamWriter(字节流转向字符流)
作用:可以读取不同编码格式的文件
需要使用到 FileInputStream 类
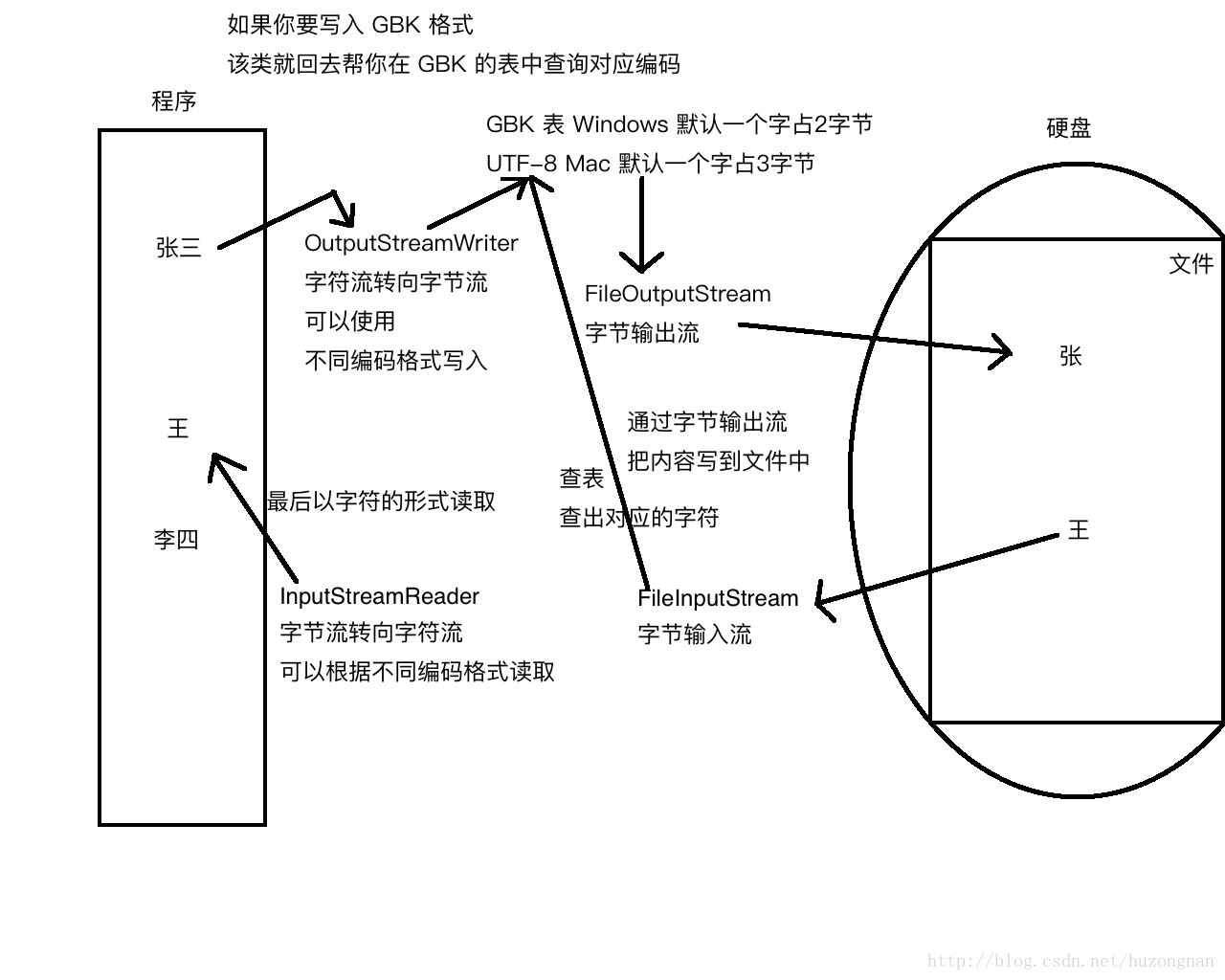
// 利用转换流写文件 OutputStreamWriter 默认UTF-8写的
public static void getUTF8() throws IOException{
FileOutputStream fos = new FileOutputStream("/Users/Desktop/Test/utf8.txt");
// 创建转换流,字符流转向字节流
OutputStreamWriter osw = new OutputStreamWriter(fos);
// 写文件
osw.write("张三");
// 关闭资源(之关闭外层流即可,内层流在外层流关闭时,外层流会帮你内部实现关闭)
osw.close();
}
// 利用转换流,使用 GBK 的编码写入文件
public static void getGBK() throws IOException{
FileOutputStream fos = new FileOutputStream("/Users/Desktop/Test/gbk.txt");
// 构建转换流,传入编码格式(编码格式的字符串,忽略大小写)
OutputStreamWriter osw = new OutputStreamWriter(fos, "GBK");
osw.write("李四");
osw.close();
}
// 利用转换流 InputStreamWriter 读文件,默认 UTF-8
public static void readUTF8() throws IOException {
FileInputStream fis = new FileInputStream("/Users/Desktop/Test/utf8.txt");
InputStreamReader isr = new InputStreamReader(fis);
char[] c = new char[1024];
int len = 0;
while ((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
// 利用转换流,读取 GBK 编码的文件
public static void readGBK() throws IOException {
FileInputStream fis = new FileInputStream("/Users/Desktop/Test/gbk.txt");
InputStreamReader isr = new InputStreamReader(fis, "GBK");
char[] c = new char[1024];
int len = 0;
while ((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
/*
* "Success is the constant experience of failure and always keeping the initial enthusiasm"
* 把上列字符串以下列形式写入文件
* Success=1
* is=1
* the=2
* .....
*/
public class Demo {
public static void main(String[] args) throws IOException {
mapPutIntoTxt();
readGBK();
}
/*
* 定义一个 String string = "Success is the constant experience of failure and always keeping the initial enthusiasm";
* 用空格分割成一个一个字符串
* 将获取到的字符串存入 map 中为key 值,比对有无字符串,有value + 1,没有 value = 1;
*/
public static HashMap<String, Integer> stringPutIntoMap() {
HashMap<String, Integer> map = new HashMap<>();
String string = "Success is the constant experience of failure and always keeping the initial enthusiasm";
String[] split = string.split(" ");
for (String key : split) {
if(map.containsKey(key)) {
Integer value = map.get(key);
map.put(key, value + 1);
} else {
map.put(key, 1);
}
}
return map;
}
/*
* 获取 key - value 键值对
* 将键值对输入文件中
*/
public static void mapPutIntoTxt() throws IOException {
FileOutputStream fos = new FileOutputStream("/Users/Desktop/Test/utf8.txt");
HashMap<String, Integer> map = stringPutIntoMap();
OutputStreamWriter osw = null;
for (Entry<String, Integer> entry : map.entrySet()) {
String string = entry.toString();
string = string +"\n";
osw = new OutputStreamWriter(fos);
osw.write(string);
osw.flush();
}
osw.close();
}
// 利用转换流,读取 GBK 编码的文件
public static void readGBK() throws IOException {
FileInputStream fis = new FileInputStream("/Users/Desktop/Test/utf8.txt");
InputStreamReader isr = new InputStreamReader(fis);
char[] c = new char[1024];
int len = 0;
while ((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
}
/*
* 将一个文件夹下的所有txt文件 复制 到另一个文件夹下 并且把txt改成java
*/
public class Demo {
public static void main(String[] args) throws IOException {
test();
}
public static void test() throws IOException {
File src = new File("/Users/Desktop/Test");
File dest = new File("/Users/Desktop/XTest");
copyDirTXTFIle(src, dest);
}
public static void copyDirTXTFIle(File src, File dest) throws IOException {
File[] listFiles = src.listFiles(new MyFilterByTxt());
InputStreamReader isr = null;
OutputStreamWriter osw = null;
for (File subFile : listFiles) {
// 判断是否是文件
if (subFile.isFile()) {
// 是文件,通过过滤器,得到的一定是 TXT 文件,进行读写
FileInputStream fis = new FileInputStream(subFile);
// 拼接
String[] split = subFile.getName().split("\\.");
File tempFile = new File(dest,split[0] + ".java");
isr = new InputStreamReader(fis);
FileOutputStream fos = new FileOutputStream(tempFile);
osw = new OutputStreamWriter(fos);
char[] c = new char[1024];
int len = 0;
while((len = isr.read(c)) != -1) {
osw.write(new String(c, 0, len));
}
osw.flush();
} else {
copyDirTXTFIle(subFile, dest);
}
}
isr.close();
osw.close();
}
}
class MyFilterByTxt implements FileFilter{
@Override
public boolean accept(File pathname) {
// 判断是否是文件夹,是直接返回 true, 确保文件不被过滤
if (pathname.isDirectory()) {
return true;
}
// 直接返回 TXT 文件
return pathname.getName().endsWith("txt");
}
}
/*
* 计算
* 字节流的两种读取文件方式(单个字节读/字符数组读)
* 字符流的两种读取文件方式(单个字节读/字符数组读)
* 复制文件的时间(使用模板设计模式)
*/
public class Demo {
public static void main(String[] args) throws IOException {
Test();
}
public static void Test() throws IOException {
System.out.println("字节流单字节读取文件:");
ByteStreamRead byteStreamReadSingleByte = new ByteStreamReadSingleByte();
byteStreamReadSingleByte.countSpeedTimes();
System.out.println("字节流字符数组读取文件:");
ByteStreamRead byteStreamReadCharacterArray = new ByteStreamReadCharacterArray();
byteStreamReadCharacterArray.countSpeedTimes();
System.out.println("字符流单字节读取文件:");
CharacterStream characterStreamSingleByte = new CharacterStreamSingleByte();
characterStreamSingleByte.countSpeedTimes();
System.out.println("字符流字符数字读取文件:");
CharacterStream characterStreamCharacterArray = new CharacterStreamCharacterArray();
characterStreamCharacterArray.countSpeedTimes();
}
}
// 模板设计模式
abstract class Count{
// 统计程序所用时间
public void countSpeedTimes() throws IOException {
// 获取开始时间
long start = System.currentTimeMillis();
// 调用抽象方法
readFile();
// 获取结束时间
long end = System.currentTimeMillis();
// 打印所用时间
System.out.println("花费:" + (end - start) + " ms");
}
// 声明一个抽象方法
public abstract void readFile() throws IOException;
}
// 字节流的读取方法
abstract class ByteStreamRead extends Count{
// 重新抽象方法
@Override
public void readFile() throws IOException {
FileInputStream fis = null;
try {
fis = new FileInputStream("/Users/Desktop/Test/utf8.txt");
int len = 0;
run(len, fis);
} catch (FileNotFoundException e) {
throw new RuntimeException("文件路径有误,无法找到该文件!");
} catch (IOException e) {
throw new RuntimeException("文件读取失败!");
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
throw new RuntimeException("资源关闭失败!");
}
}
}
public abstract void run(int len, FileInputStream fis) throws IOException;
}
class ByteStreamReadSingleByte extends ByteStreamRead{
@Override
public void run(int len, FileInputStream fis) throws IOException {
while ((len = fis.read()) !=-1) {
System.out.println((char)len);
}
}
}
class ByteStreamReadCharacterArray extends ByteStreamRead{
@Override
public void run(int len, FileInputStream fis) throws IOException {
byte[] b = new byte[1024];
while((len = fis.read(b)) != -1) {
System.out.println(new String(b, 0, len));
}
}
}
abstract class CharacterStream extends Count{
@Override
public void readFile() throws IOException {
FileReader fr = new FileReader("/Users/Desktop/Test/utf8.txt");
run(fr);
fr.close();
}
public abstract void run(FileReader fr) throws IOException;
}
class CharacterStreamSingleByte extends CharacterStream{
@Override
public void run(FileReader fr) throws IOException {
int len;
while ((len = fr.read()) != -1) {
System.out.println((char)len);
}
}
}
class CharacterStreamCharacterArray extends CharacterStream{
@Override
public void run(FileReader fr) throws IOException {
int len;
char[] c = new char[1024];
while ((len = fr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
}
}
http://blog.csdn.net/huzongnan/article/list