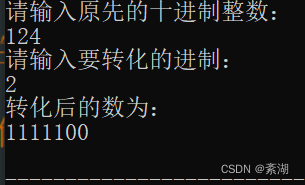
#include <stdio.h>
#include <stdlib.h>
#define Maxsize 100
struct SNode{
int Data[Maxsize];
int top;
};
typedef SNode* Stack;
Stack Create();
void Push(Stack,int);
void Pop(Stack);
int main()
{
Stack S;
int num1,num2,t;
S=Create();
printf("请输入原先的十进制整数:\n");
scanf("%d",&num1);
printf("请输入要转化的进制:\n");
scanf("%d",&t);
while(num1 !=0){
Push(S,num1%t);
num1/=t;
}
printf("转化后的数为:\n");
while(S->top != -1){
Pop(S);
}
putchar('\n');
return 0;
}
Stack Create()
{
Stack P = (Stack)malloc(sizeof(struct SNode));
if(P == NULL){
printf("空间不足!\n");
}
else{
P->top = -1;
}
return P;
}
void Push(Stack S,int item){
if(S->top == Maxsize-1){
printf("栈满!\n");
}
else{
S->Data[++S->top] = item;
}
}
void Pop(Stack S)
{
int n;
if(S->top == -1){
printf("栈空!\n");
}
else{
n = S->Data[S->top--];
printf("%d",n);
}
}