8.1 池化技术
线程池特点:线程复用,控制最大并发数,管理线程。
池化技术简单点来说,就是提前保存大量的资源,以备不时之需。在机器资源有限的情况下,使用池化技术可以大大的提高资源的利用率,提升性能等。在编程领域,比较典型的池化技术有:线程池、连接池、内存池、对象池等。
8.2 线程池实现原理
通过创建一个线程对象,并且实现Runnable接口就可以实现一个简单的线程。可以利用上多核CPU。当一个任务结束,当前线程就接收。但很多时候,我们不止会执行一个任务。如果每次都是如此的创建线程->执行任务->销毁线程,会造成很大的性能开销那能否一个线程创建后,执行完一个任务后,又去执行另一个任务,而不是销毁。这就是线程池。
8.3 使用线程池好处
- 降低资源消耗,通过重复利用已创建的线程降低线程创建和销毁造成的消耗。
- 提高响应速度。当任务到达时,任务可以不需要等待线程创建就能立即执行。
- 提高线程的可管理性,线程是稀缺资源,如果无限制的创建,不仅会消耗系统资源,还会降低系统的稳定性,使用线程池可以进行统一分配,调优和监控。
8.4 三大方法
代码示例
package cn.guardwhy.BlockingQueue;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
// Executors 工具类、3大方法
public class ThreadPoolDemo06 {
public static void main(String[] args) {
// ExecutorService threadPool = Executors.newSingleThreadExecutor(); // 单个线程
// ExecutorService threadPool = Executors.newFixedThreadPool(5); // 创建一个固定的线程池的大小
ExecutorService threadPool = Executors.newCachedThreadPool();// 无限容器
try {
for (int i = 0; i < 100; i++) {
// 使用线程池来创建线程
threadPool.execute(()->{
System.out.println(Thread.currentThread().getName() + " 创建成功..");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭线程池,程序结束。
threadPool.shutdown();
}
}
}
8.5 七大参数
8.5.1 源码分析
查看三大方法的底层源码,都调用了new ThreadPoolExecutor( )方法
public ThreadPoolExecutor(int corePoolSize, // 核心线程池大小
int maximumPoolSize, // 最大核心线程池大小
long keepAliveTime, // 空闲的线程保留的时间
TimeUnit unit, // 超时单位
BlockingQueue<Runnable> workQueue, // 阻塞队列
ThreadFactory threadFactory, // 线程工厂,创建线程的
RejectedExecutionHandler handler) { // 拒绝策略
if (corePoolSize < 0 ||
maximumPoolSize <= 0 ||
maximumPoolSize < corePoolSize ||
keepAliveTime < 0)
throw new IllegalArgumentException();
if (workQueue == null || threadFactory == null || handler == null)
throw new NullPointerException();
this.acc = System.getSecurityManager() == null ?
null :
AccessController.getContext();
this.corePoolSize = corePoolSize;
this.maximumPoolSize = maximumPoolSize;
this.workQueue = workQueue;
this.keepAliveTime = unit.toNanos(keepAliveTime);
this.threadFactory = threadFactory;
this.handler = handler;
}
8.5.2 注意点
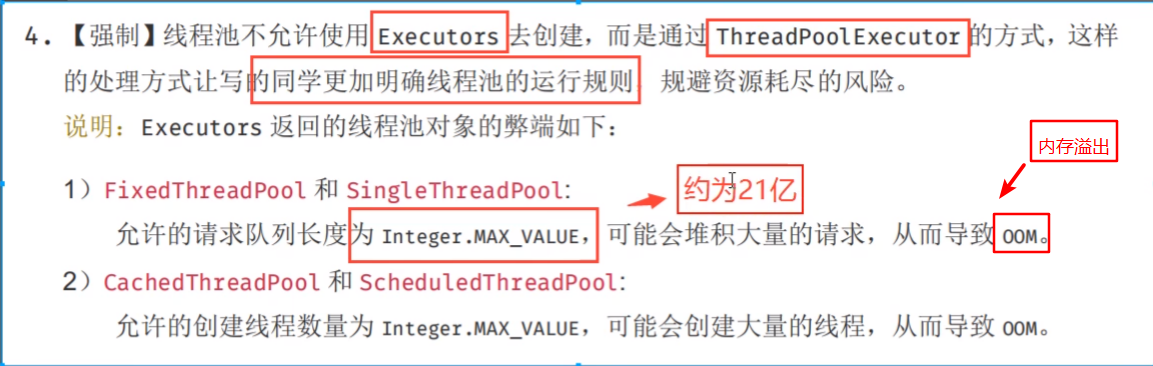
8.5.3 ThreadPoolExecutor 底层原理
8.6 四种拒绝策略
8.6.1 银行办理业务
- 1~2人被受理(核心区大小core)
- 3~5人进入队列(Queue)
- 6~8人到最大线程池(扩容大小max)
- 再有人进来就要被拒绝策略接受了

8.6.2 源码分析
8.6.3 代码示例
package cn.guardwhy.BlockingQueue;
import java.util.concurrent.*;
/*
* 1.new ThreadPoolExecutor.CallerRunsPolicy(): 银行满了,还有客户进来。不处理这个客户,抛出异常
* 2.new ThreadPoolExecutor.AbortPolicy(): 队列满了丢任务,不抛出异常,哪来的去哪里。
* 3.new ThreadPoolExecutor.DiscardPolicy():队列满了,丢掉任务,不会抛出异常!!!!
* 4.new ThreadPoolExecutor.DiscardOldestPolicy():队列满了,尝试去和最早的竞争,但是不会抛出异常。
*
*/
public class ThreadPoolDemo07 {
public static void main(String[] args) {
ExecutorService threadPool = new ThreadPoolExecutor(2,
5
, 3,
TimeUnit.SECONDS,
new LinkedBlockingDeque<>(3),
Executors.defaultThreadFactory(),
new ThreadPoolExecutor.DiscardOldestPolicy());
try {
// 最大容量: Deque + maximumPoolSize
for (int i = 0; i < 9; i++) {
// 使用线程池来创建线程
threadPool.execute(()->{
System.out.println(Thread.currentThread().getName() + " 创建成功..");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭线程池,程序结束。
threadPool.shutdown();
}
}
}
8.6.4 线程池设置
最大的线程设置: IO密集型, CPU密集型 (调优)
package cn.guardwhy.BlockingQueue;
import java.util.concurrent.*;
/*
最大线程定义:
1.CPU 密集型,几核就是几,可以保持CPU的效率最高。cpu密集型的任务来说,线程数等于cpu数是最好的了。
2.IO 密集型 》= 判断程序中十分消耗的IO线程,线程数大于等于IO任务数是最佳的
*/
public class ThreadPoolDemo07 {
public static void main(String[] args) {
// 获取CPU的核数
System.out.println(Runtime.getRuntime().availableProcessors());
ExecutorService threadPool = new ThreadPoolExecutor(2,
Runtime.getRuntime().availableProcessors()
, 3,
TimeUnit.SECONDS,
new LinkedBlockingDeque<>(3),
Executors.defaultThreadFactory(),
new ThreadPoolExecutor.DiscardOldestPolicy());
try {
// 最大容量: Deque + maximumPoolSize
for (int i = 0; i < 9; i++) {
// 使用线程池来创建线程
threadPool.execute(()->{
System.out.println(Thread.currentThread().getName() + " 创建成功..");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭线程池,程序结束。
threadPool.shutdown();
}
}
}