json文件:
{
"IsStrucuralCheacked":"False",
"IsMechanicalChecked":"True",
"IsOnlyVisibleChecked":"True",
"IsWholeModelSelected":"True",
"IsRangeSelected":"false",
"IsLevelSelected":"false",
"AnalysisScope":"3000",
"Limitation":"2500",
"MinHeight":"2300",
"RoundValue":"10"
}
1、根据json文件生成一个对象 ---- ConfigEntity
public class ConfigEntity
{
public bool IsStrucuralCheacked { get; set; }
public bool IsMechanicalChecked { get; set; }
public bool IsOnlyVisibleChecked { get; set; }
public bool IsWholeModelSelected { get; set; }
public bool IsRangeSelected { get; set; }
public bool IsLevelSelected { get; set; }
public string AnalysisScope { get; set; }
public string Limitation { get; set; }
public string MinHeight { get; set; }
public string RoundValue { get; set; }
}
2、读取jiso文件里面的内容
public T GetConfigValue<T>(Func<ConfigEntity, T> selector)
{
string filePath = Path.GetDirectoryName(Assembly.GetExecutingAssembly().Location) + @"\ConfigFiles\Config.json";
T result = default(T);
// 读取配置文件内容
using (StreamReader reader = new StreamReader(filePath))
{
string json = reader.ReadToEnd();
ConfigEntity config = JsonConvert.DeserializeObject<ConfigEntity>(json);
// 根据传入的 selector 函数获取需要的配置项
result = selector(config);
}
return result;
}
3、设置json文件里面的内容
/// <summary>
/// 根据value设置配置文件里面的参数
/// </summary>
/// <param name="value"></param>
public void SetConfigValue<T>(Action<ConfigEntity, T> modifier, T value)
{
string filePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "ConfigFiles", "Config.json");
// 读取配置文件内容
string json = File.ReadAllText(filePath);
// 反序列化配置文件内容为 ConfigEntity 对象
ConfigEntity config = JsonConvert.DeserializeObject<ConfigEntity>(json);
// 调用传入的 modifier 函数修改属性值
modifier(config, value);
// 将修改后的对象序列化为 JSON 字符串
string updatedJson = JsonConvert.SerializeObject(config);
// 将更新后的 JSON 字符串写入配置文件
File.WriteAllText(filePath, updatedJson);
}
4、具体使用
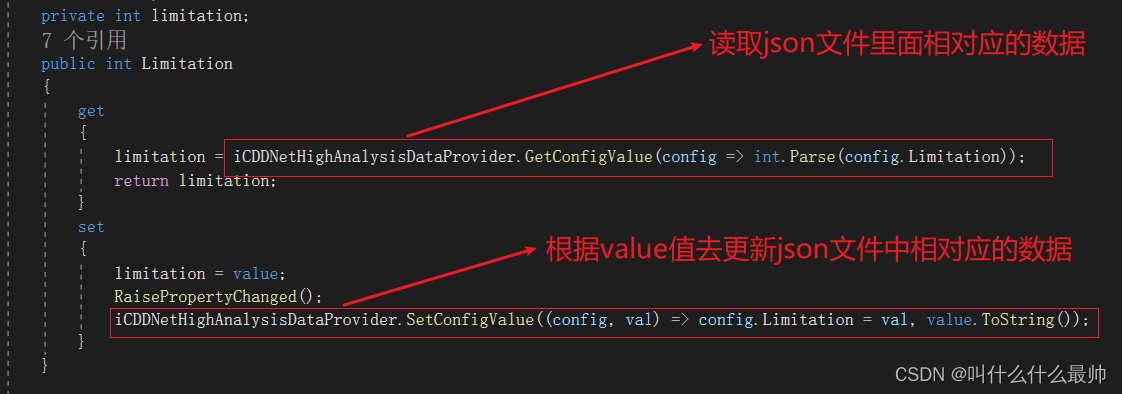