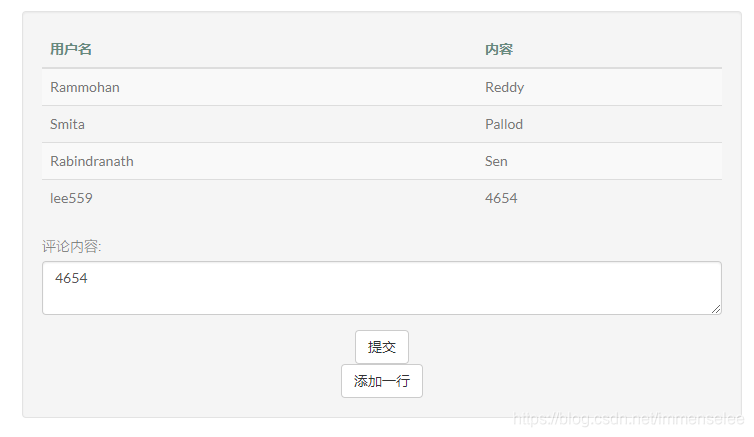
一、前端设计
<table class="table table-striped" id="tableOne">
<thead>
<tr >
<th>用户名</th>
<th>内容</th>
</tr>
</thead>
<tbody id="tbody">
<tr>
<td>Rammohan </td>
<td>Reddy</td>
</tr>
<tr>
<td>Smita</td>
<td>Pallod</td>
</tr>
<tr>
<td>Rabindranath</td>
<td>Sen</td>
</tr>
</tbody>
</table>
<form name="CommenForm" id="CommenForm">
<div class="form-group">
<label for="InputComment">评论内容:</label>
<textarea type="text" row="3" class="form-control" name="InputComment" id="InputComment" placeholder="评论内容" required></textarea>
</div>
<div class="form-group">
<input type=hidden name="aType" value="<%=request.getParameter("aType")%>">
</div>
<div class="form-group">
<input type=hidden name="Id" value="<%=request.getParameter("Id")%>">
</div>
<div class="form-group">
<input type=hidden name="Recent_URL" value="<%=request.getRequestURL()%>">
</div>
<button type="button" class="btn btn-default center-block" id="one" onclick="SubmitForm()">提交</button>
<button type="button" class="btn btn-default center-block" id="add" onclick="createRow()">添加一行</button>
</form>
二、 JavaScript设计
<script type="text/javascript">
//新建表格一行
function createRow(){
var CommentInput = document.CommenForm.InputComment.value;
var editTable=document.getElementById("tbody");
var tr=document.createElement("tr");
var td1=document.createElement("td");
td1.innerHTML="<%=aUser.getUsername()%>";
var td2=document.createElement("td");
td2.innerHTML=CommentInput;
tr.appendChild(td1);
tr.appendChild(td2);
editTable.appendChild(tr);
}
function SubmitForm() {
$.ajax({
//几个参数需要注意一下
type: "POST",//方法类型
dataType: "json",//预期服务器返回的数据类型
url: "PostComment.action" ,//url
data: $('#CommenForm').serialize(),
success: function (result) {
console.log(result);//打印服务端返回的数据(调试用)
var JsResult = JSON.parse(result);
console.log(typeof JsResult.resultCode);//打印服务端返回的数据(调试用)
console.log(JsResult.resultCode);//打印服务端返回的数据(调试用)
if (JsResult.resultCode==200) {
alert("请求成功");
createRow();
}
},
error : function() {
alert("异常!");
}
});
}
</script>
三、action设计
/**
*
*/
package struts2.action;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.apache.struts2.ServletActionContext;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.opensymphony.xwork2.ActionSupport;
import jiajiao.qiantai.Comment;
import jiajiao.qiantai.CommentHome;
import jiajiao.qiantai.User;
import net.sf.json.JSONObject;
/**
* @author Administrator
*
*/
public class PostComment extends ActionSupport{
/**
* @param args
*/
private String result;
public String getResult() {
return result;
}
public void setResult(String result) {
this.result = result;
}
public String execute() throws Exception {
try {
HttpServletRequest request = ServletActionContext.getRequest();
HttpSession session = request.getSession();
String Msg_Time = "";//Time
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd-HH:mm:ss");//设置日期格式
Msg_Time=df.format(new Date());
ApplicationContext ctx=new ClassPathXmlApplicationContext("applicationContext.xml");
Comment aComment=(Comment) ctx.getBean("Comment");
CommentHome aCommentHome=(CommentHome) ctx.getBean("CommentHome");
User aUser=(User) ctx.getBean("User");
//检测是否登录
Integer UserId=-1;
if(session.getAttribute("Iduser")!=null) {
UserId=(Integer)session.getAttribute("Iduser");
System.out.println("userid:"+UserId);
}else {
return "PostComment_Fail_NeedLogin";
}
if(session.getAttribute("aUser")!=null) {
aUser = (User) session.getAttribute("aUser");
}else {
return "PostComment_Fail_NeedLogin";
}
//获取提交的数据
String aType = request.getParameter("aType");
if(aType==null||aType.equals("")) {
System.out.println("aType:"+aType);
return "PostComment_NoInfo";
}
String InfoId = request.getParameter("Id");
if(InfoId==null||InfoId.equals("")) {
System.out.println("InfoId:"+InfoId);
return "PostComment_NoInfo";
}
String InputComment = request.getParameter("InputComment");
if(InputComment==null||InputComment.equals("")) {
System.out.println("InputComment:"+InputComment);
return "PostComment_NoInfo";
}
//开始持久化数据
aComment.setCommentContent(InputComment);
aComment.setCommentLikesNumber(0);
aComment.setCommentPublishTime(Msg_Time);
aComment.setCommentState("initial");
aComment.setTopicId(Integer.valueOf(InfoId));
aComment.setTopicType(aType);
aComment.setUserByFromUserid(aUser);
aCommentHome.attachDirty(aComment);
//将原地址放入Recent_URL
String Recent_URL="Default";
Recent_URL = request.getParameter("Recent_URL");
request.setAttribute("Recent_URL", Recent_URL);
//将数据存储在map里,再转换成json类型数据,也可以自己手动构造json类型数据
Map<String,Object> map = new HashMap<String,Object>();
map.put("resultCode",200);
JSONObject json = JSONObject.fromObject(map);//将map对象转换成json类型数据
result = json.toString();//给result赋值,传递给页面
return "PostComment_Success";
}catch(Exception e) {
// System.out.println("Exception");
e.printStackTrace();
throw e;
//return "post1_fail";
}
}
}
四、struts.xml设计
<action name="PostComment" class="struts2.action.PostComment">
<result name="PostComment_Fail_NeedLogin">/login.jsp</result>
<result name="PostComment_NoInfo">/error.jsp</result>
<result name="PostComment_Success" type="json">
<param name="root">result<!-- result是action中设置的变量名,也是页面需要返回的数据,该变量必须有setter和getter方法 --></param>
</result>
</action>
参考:https://www.cnblogs.com/lraa/p/3249990.html