简单的ajax评论完整代码
数据库结构CREATE TABLE `comments` (
`id` int(10) unsigned NOT NULL auto_increment,
`name` varchar(128) collate utf8_unicode_ci NOT NULL default '',
`url` varchar(255) collate utf8_unicode_ci NOT NULL default '',
`email` varchar(255) collate utf8_unicode_ci NOT NULL default '',
`body` text collate utf8_unicode_ci NOT NULL,
`dt` timestamp NOT NULL default CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci AUTO_INCREMENT=1;
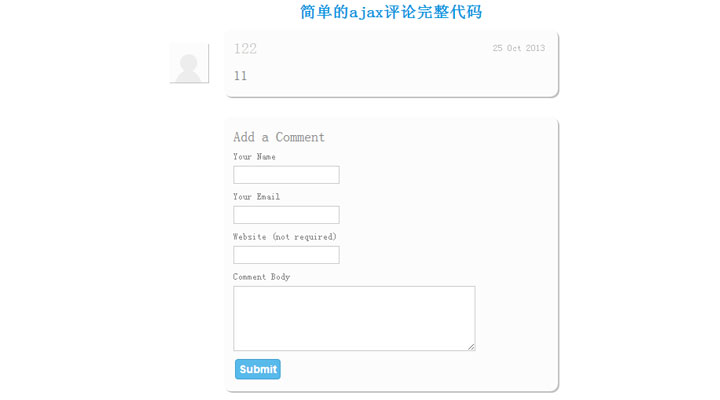
PHP Code
- <?php
- // Error reporting:
- error_reporting(E_ALL^E_NOTICE);
- include "conn.php";
- include "comment.class.php";
- /*
- / Select all the comments and populate the $comments array with objects
- */
- $comments = array();
- $result = mysql_query("SELECT * FROM comments ORDER BY id ASC");
- while($row = mysql_fetch_assoc($result))
- {
- $comments[] = new Comment($row);
- }
- ?>
PHP Code
- <div id="main">
- <?php
- /*
- / Output the comments one by one:
- */
- foreach($comments as $c){
- echo $c->markup();
- }
- ?>
- <div id="addCommentContainer">
- <p>Add a Comment</p>
- <form id="addCommentForm" method="post" action="">
- <div>
- <label for="name">Your Name</label>
- <input type="text" name="name" id="name" />
- <label for="email">Your Email</label>
- <input type="text" name="email" id="email" />
- <label for="url">Website (not required)</label>
- <input type="text" name="url" id="url" />
- <label for="body">Comment Body</label>
- <textarea name="body" id="body" cols="20" rows="5"></textarea>
- <input type="submit" id="submit" value="Submit" />
- </div>
- </form>
- </div>
- </div>
submit.php
PHP Code
- <?php
- // Error reporting:
- error_reporting(E_ALL^E_NOTICE);
- include "conn.php";
- include "comment.class.php";
- /*
- / This array is going to be populated with either
- / the data that was sent to the script, or the
- / error messages.
- /*/
- $arr = array();
- $validates = Comment::validate($arr);
- if($validates)
- {
- /* Everything is OK, insert to database: */
- mysql_query(" INSERT INTO comments(name,url,email,body)
- VALUES (
- '".$arr['name']."',
- '".$arr['url']."',
- '".$arr['email']."',
- '".$arr['body']."'
- )");
- $arr['dt'] = date('r',time());
- $arr['id'] = mysql_insert_id();
- /*
- / The data in $arr is escaped for the mysql query,
- / but we need the unescaped variables, so we apply,
- / stripslashes to all the elements in the array:
- /*/
- $arr = array_map('stripslashes',$arr);
- $insertedComment = new Comment($arr);
- /* Outputting the markup of the just-inserted comment: */
- echo json_encode(array('status'=>1,'html'=>$insertedComment->markup()));
- }
- else
- {
- /* Outputtng the error messages */
- echo '{"status":0,"errors":'.json_encode($arr).'}';
- }
- ?>
comment.class.php
PHP Code
- <?php
- class Comment
- {
- private $data = array();
- public function __construct($row)
- {
- /*
- / The constructor
- */
- $this->data = $row;
- }
- public function markup()
- {
- /*
- / This method outputs the XHTML markup of the comment
- */
- // Setting up an alias, so we don't have to write $this->data every time:
- $d = &$this->data;
- $link_open = '';
- $link_close = '';
- if($d['url']){
- // If the person has entered a URL when adding a comment,
- // define opening and closing hyperlink tags
- $link_open = '<a href="'.$d['url'].'">';
- $link_close = '</a>';
- }
- // Converting the time to a UNIX timestamp:
- $d['dt'] = strtotime($d['dt']);
- // Needed for the default gravatar image:
- $url = 'http://'.dirname($_SERVER['SERVER_NAME'].$_SERVER["REQUEST_URI"]).'/img/default_avatar.gif';
- return '
- <div class="comment">
- <div class="avatar">
- '.$link_open.'
- <img src="" />
- '.$link_close.'
- </div>
- <div class="name">'.$link_open.$d['name'].$link_close.'</div>
- <div class="date" title="Added at '.date('H:i \o\n d M Y',$d['dt']).'">'.date('d M Y',$d['dt']).'</div>
- <p>'.$d['body'].'</p>
- </div>
- ';
- }
- public static function validate(&$arr)
- {
- /*
- / This method is used to validate the data sent via AJAX.
- /
- / It return true/false depending on whether the data is valid, and populates
- / the $arr array passed as a paremter (notice the ampersand above) with
- / either the valid input data, or the error messages.
- */
- $errors = array();
- $data = array();
- // Using the filter_input function introduced in PHP 5.2.0
- if(!($data['email'] = filter_input(INPUT_POST,'email',FILTER_VALIDATE_EMAIL)))
- {
- $errors['email'] = 'Please enter a valid Email.';
- }
- if(!($data['url'] = filter_input(INPUT_POST,'url',FILTER_VALIDATE_URL)))
- {
- // If the URL field was not populated with a valid URL,
- // act as if no URL was entered at all:
- $url = '';
- }
- // Using the filter with a custom callback function:
- if(!($data['body'] = filter_input(INPUT_POST,'body',FILTER_CALLBACK,array('options'=>'Comment::validate_text'))))
- {
- $errors['body'] = 'Please enter a comment body.';
- }
- if(!($data['name'] = filter_input(INPUT_POST,'name',FILTER_CALLBACK,array('options'=>'Comment::validate_text'))))
- {
- $errors['name'] = 'Please enter a name.';
- }
- if(!empty($errors)){
- // If there are errors, copy the $errors array to $arr:
- $arr = $errors;
- return false;
- }
- // If the data is valid, sanitize all the data and copy it to $arr:
- foreach($data as $k=>$v){
- $arr[$k] = mysql_real_escape_string($v);
- }
- // Ensure that the email is lower case:
- $arr['email'] = strtolower(trim($arr['email']));
- return true;
- }
- private static function validate_text($str)
- {
- /*
- / This method is used internally as a FILTER_CALLBACK
- */
- if(mb_strlen($str,'utf8')<1)
- return false;
- // Encode all html special characters (<, >, ", & .. etc) and convert
- // the new line characters to <br> tags:
- $str = nl2br(htmlspecialchars($str));
- // Remove the new line characters that are left
- $str = str_replace(array(chr(10),chr(13)),'',$str);
- return $str;
- }
- }
- ?>