这个定时系统的UML类图如下:
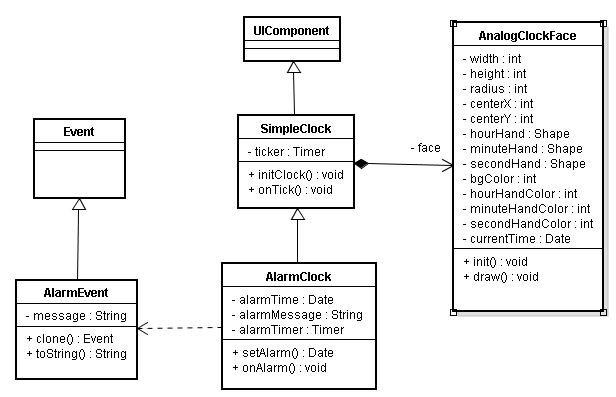
AnalogClockFace类


<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->packagecom.example.programmingas3.clock
{
importflash.display.Shape;
importflash.display.Sprite;
importflash.display.StageAlign;
importflash.display.StageScaleMode;
importflash.text.StaticText;
importflash.events.*;
importflash.text.TextField;
importflash.text.TextFormat;
importmx.core.UIComponent;
/**
*Displaysaroundclockfacewithanhourhand,aminutehand,andasecondhand.
*/
publicclassAnalogClockFaceextendsUIComponent
{
/**
*Thedesiredwidthofthiscomponent,asopposedtothe.width
*propertywhichrepresentsthaactualwidth.
*/
publicvarw:uint=200;
/**
*Thedesiredheightofthiscomponent,asopposedtothe.height
*propertywhichrepresentsthaactualheight.
*/
publicvarh:uint=200;
/**
*Theradiusfromthecenteroftheclocktothe
*outeredgeofthecircularfaceoutline.
*/
publicvarradius:uint;
/**
*Thecoordinatesofthecenteroftheface.
*/
publicvarcenterX:int;
publicvarcenterY:int;
/**
*Thethreehandsoftheclock.
*/
publicvarhourHand:Shape;
publicvarminuteHand:Shape;
publicvarsecondHand:Shape;
/**
*Thecolorsofthebackgroundandeachhand.
*Thesecouldbesetusingparametersor
*stylesinthefuture.
*/
publicvarbgColor:uint=0xEEEEFF;
publicvarhourHandColor:uint=0x003366;
publicvarminuteHandColor:uint=0x000099;
publicvarsecondHandColor:uint=0xCC0033;
/**
*Storesasnapshotofthecurrenttime,soit
*doesn'tchangewhileinthemiddleofdrawingthe
*threehands.
*/
publicvarcurrentTime:Date;
/**
*Contructsanewclockface.Thewidthand
*heightwillbeequal.
*/
publicfunctionAnalogClockFace(w:uint)
{
this.w=w;
this.h=w;
//Roundstothenearestpixel
this.radius=Math.round(this.w/2);
//Thefaceisalwayssquarenow,sothe
//distancetothecenteristhesame
//horizontallyandvertically
this.centerX=this.radius;
this.centerY=this.radius;
}
/**
*Createstheoutline,hourlabels,andclockhands.
*/
publicfunctioninit():void
{
//drawsthecircularclockoutline
drawBorder();
//drawsthehournumbers
drawLabels();
//createsthethreeclockhands
createHands();
}
/**
*Drawsacircularborder.
*/
publicfunctiondrawBorder():void
{
graphics.lineStyle(0.5,0x999999);
graphics.beginFill(bgColor);
graphics.drawCircle(centerX,centerY,radius);
graphics.endFill();
}
/**
*Putsnumericlabelsatthehourpoints.
*/
publicfunctiondrawLabels():void
{
for(vari:Number=1;i<=12;i++)
{
//CreatesanewTextFieldshowingthehournumber
varlabel:TextField=newTextField();
label.text=i.toString();
//Placeshourlabelsaroundtheclockface.
//Thesin()andcos()functionsbothoperateonanglesinradians.
varangleInRadians:Number=i*30*(Math.PI/180)
//Placethelabelusingthesin()andcos()functionstogetthex,ycoordinates.
//Themultipliervalueof0.9putsthelabelsinsidetheoutlineoftheclockface.
//Theintegervalueattheendoftheequationadjuststhelabelposition,
//sincethex,ycoordinateisintheupperleftcorner.
label.x=centerX+(0.9*radius*Math.sin(angleInRadians))-5;
label.y=centerY-(0.9*radius*Math.cos(angleInRadians))-9;
//Formatsthelabeltext.
vartf:TextFormat=newTextFormat();
tf.font="Arial";
tf.bold="true";
tf.size=12;
label.setTextFormat(tf);
//Addsthelabeltotheclockfacedisplaylist.
addChild(label);
}
}
/**
*Createshour,minute,andsecondhandsusingthe2DdrawingAPI.
*/
publicfunctioncreateHands():void
{
//UsesaShapesinceit'sthesimplestcomponentthatsupports
//the2DdrawingAPI.
varhourHandShape:Shape=newShape();
drawHand(hourHandShape,Math.round(radius*0.5),hourHandColor,3.0);
this.hourHand=Shape(addChild(hourHandShape));
this.hourHand.x=centerX;
this.hourHand.y=centerY;
varminuteHandShape:Shape=newShape();
drawHand(minuteHandShape,Math.round(radius*0.8),minuteHandColor,2.0);
this.minuteHand=Shape(addChild(minuteHandShape));
this.minuteHand.x=centerX;
this.minuteHand.y=centerY;
varsecondHandShape:Shape=newShape();
drawHand(secondHandShape,Math.round(radius*0.9),secondHandColor,0.5);
this.secondHand=Shape(addChild(secondHandShape));
this.secondHand.x=centerX;
this.secondHand.y=centerY;
}
/**
*Drawsaclockhandwithagivensize,color,andthickness.
*/
publicfunctiondrawHand(hand:Shape,distance:uint,color:uint,thickness:Number):void
{
hand.graphics.lineStyle(thickness,color);
hand.graphics.moveTo(0,distance);
hand.graphics.lineTo(0,0);
}
/**
*Calledbytheparentcontainerwhenthedisplayisbeingdrawn.
*/
publicfunctiondraw():void
{
//Storesthecurrentdateandtimeinaninstancevariable
currentTime=newDate();
showTime(currentTime);
}
/**
*DisplaysthegivenDate/Timeinthatgoodoldanalogclockstyle.
*/
publicfunctionshowTime(time:Date):void
{
//Getsthetimevalues
varseconds:uint=time.getSeconds();
varminutes:uint=time.getMinutes();
varhours:uint=time.getHours();
//Multipliesby6togetdegrees
this.secondHand.rotation=180+(seconds*6);
this.minuteHand.rotation=180+(minutes*6);
//Multipliesby30togetbasicdegrees,andthen
//addsupto29.5degrees(59*0.5)toaccount
//fortheminutes
this.hourHand.rotation=180+(hours*30)+(minutes*0.5);
}
}
}
SimpleClock类


<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->packagecom.example.programmingas3.clock
{
importmx.core.UIComponent;
publicclassSimpleClockextendsUIComponent
{
importcom.example.programmingas3.clock.AnalogClockFace;
importflash.events.TimerEvent;
importflash.utils.Timer;
/**
*Thetimedisplaycomponent.
*/
publicvarface:AnalogClockFace;
/**
*TheTimerthatactslikeaheartbeatfortheapplication.
*/
publicvarticker:Timer;
publicstaticconstmillisecondsPerMinute:int=1000*60;
publicstaticconstmillisecondsPerHour:int=1000*60*60;
publicstaticconstmillisecondsPerDay:int=1000*60*60*24;
/**
*SetsupaSimpleClockinstance.
*/
publicfunctioninitClock(faceSize:Number=200):void
{
//setstheinvoicedatetotoday’sdate
varinvoiceDate:Date=newDate();
//adds30daystogettheduedate
varmillisecondsPerDay:int=1000*60*60*24;
vardueDate:Date=newDate(invoiceDate.getTime()+(30*millisecondsPerDay));
varoneHourFromNow:Date=newDate();//startsatthecurrenttime
oneHourFromNow.setTime(oneHourFromNow.getTime()+millisecondsPerHour);
//CreatestheclockfaceandaddsittotheDisplayList
face=newAnalogClockFace(Math.max(20,faceSize));
face.init();
addChild(face);
//Drawstheinitialclockdisplay
face.draw();
//CreatesaTimerthatfiresaneventoncepersecond.
ticker=newTimer(1000);
//DesignatestheonTick()methodtohandleTimerevents
ticker.addEventListener(TimerEvent.TIMER,onTick);
//Startstheclockticking
ticker.start();
}
/**
*CalledoncepersecondwhentheTimereventisreceived.
*/
publicfunctiononTick(evt:TimerEvent):void
{
//Updatestheclockdisplay.
face.draw();
}
}
}
AlarmClock类


<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->packagecom.example.programmingas3.clock
{
importmx.core.UIComponent;
publicclassAlarmClockextendsSimpleClock
{
importcom.example.programmingas3.clock.AnalogClockFace;
importflash.events.TimerEvent;
importflash.utils.Timer;
publicvaralarmTime:Date;
publicvaralarmMessage:String;
/**
*TheTimerthatwillbeusedforthealarm.
*/
publicvaralarmTimer:Timer;
publicstaticvarMILLISECONDS_PER_DAY:Number=1000*60*60*24;
/**
*InstantiatesanewAlarmClockofagivensize
*/
publicoverridefunctioninitClock(faceSize:Number=200):void
{
super.initClock(faceSize);
alarmTimer=newTimer(0,1);
alarmTimer.addEventListener(TimerEvent.TIMER,onAlarm);
}
/**
*Setsthetimeatwhichthealarmshouldgooff.
*@paramhourThehourportionofthealarmtime
*@paramminutesTheminutesportionofthealarmtime
*@parammessageThemessagetodisplaywhenthealarmgoesoff.
*@returnThetimeatwhichthealarmwillgooff.
*/
publicfunctionsetAlarm(hour:Number=0,minutes:Number=0,message:String="Alarm!"):Date
{
this.alarmMessage=message;
varnow:Date=newDate();
//createthistimeontoday'sdate
alarmTime=newDate(now.fullYear,now.month,now.date,hour,minutes);
//determineifthespecifiedtimehasalreadypassedtoday
if(alarmTime<=now)
{
alarmTime.setTime(alarmTime.time+MILLISECONDS_PER_DAY);
}
//resetthealarmtimerifit'scurrentlyset
alarmTimer.reset();
//calculatehowmanymillisecondsshouldpassbeforethealarmshould
//gooff(thedifferencebetweenthealarmtimeandnow)andsetthat
//valueasthedelayforthealarmtimer
alarmTimer.delay=Math.max(1000,alarmTime.time-now.time);
alarmTimer.start();
returnalarmTime;
}
/**
*CalledwhentheTimereventisreceived
*/
publicfunctiononAlarm(event:TimerEvent):void
{
trace("Alarm!");
varalarm:AlarmEvent=newAlarmEvent(this.alarmMessage);
this.dispatchEvent(alarm);
}
}
}
AlarmEvent类


<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->packagecom.example.programmingas3.clock
{
importflash.events.Event;
/**
*ThiscustomEventclassaddsamessagepropertytoabasicEvent.
*/
publicclassAlarmEventextendsEvent
{
/**
*ThenameofthenewAlarmEventtype.
*/
publicstaticconstALARM:String="alarm";
/**
*Atextmessagethatcanbepassedtoaneventhandler
*withthiseventobject.
*/
publicvarmessage:String;
/**
*Constructor.
*@parammessageThetexttodisplaywhenthealarmgoesoff.
*/
publicfunctionAlarmEvent(message:String="ALARM!")
{
super(ALARM);
this.message=message;
}
/**
*Createsandreturnsacopyofthecurrentinstance.
*@returnAcopyofthecurrentinstance.
*/
publicoverridefunctionclone():Event
{
returnnewAlarmEvent(message);
}
/**
*ReturnsaStringcontainingallthepropertiesofthecurrent
*instance.
*@returnAstringrepresentationofthecurrentinstance.
*/
publicoverridefunctiontoString():String
{
returnformatToString("AlarmEvent","type","bubbles","cancelable","eventPhase","message");
}
}
}
页面AS脚本


<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->importcom.example.programmingas3.clock.AlarmEvent;
publicfunctioninitApp():void
{
clock.addEventListener(AlarmEvent.ALARM,onAlarm);
//Adds60secondstothecurrenttimeandpreloadsthealarmfields
varalarmTime:Date=newDate();
alarmTime.setTime(alarmTime.time+60000);
hourNs.value=alarmTime.hours;
minuteNs.value=alarmTime.minutes;
}
publicfunctionsetAlarm():void
{
varalarmTime:Date=clock.setAlarm(hourNs.value,minuteNs.value,messageTxt.text);
alarmTimeTxt.text="AlarmTime:"+alarmTime.hours+":"+padZeroes(alarmTime.minutes.toString());
}
publicfunctiononAlarm(evt:AlarmEvent):void
{
alarmTimeTxt.text=evt.message;
}
publicfunctionpadZeroes(numStr:String,desiredLength:uint=2):String
{
if(numStr.length<desiredLength)
{
for(vari:uint=0;i<(desiredLength-numStr.length);i++)
{
numStr="0"+numStr;
}
}
returnnumStr;
}
运行结果:
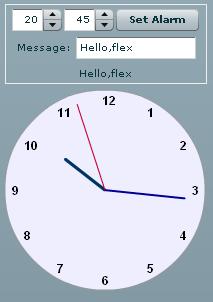