字符串:
字符串连接:使用加号 + 连接两个字符串。
>>> str1 = "Hello"
>>> str2 = "World"
>>> str1 + str2
'HelloWorld'
字符串重复:使用乘号 * 重复字符串。
>>> str1 = "Hello"
>>> str1 * 3
'HelloHelloHello'
字符串索引:使用方括号 [] 访问字符串中的单独字符。
>>> str1 = "Hello"
>>> str1[0]
'H'
数字类型:
数字运算:使用算术操作符(如加、减、乘、除等)对数字进行运算。
>>> x = 10
>>> y = 20
>>> x + y
30
>>> x * y
200
数字类型转换:使用内置函数 int()、float() 和 str() 将数字类型转换为其他类型。
>>> x = 10
>>> y = 20.0
>>> str(x)
'10'
>>> int(y)
20
>>> float(x)
10.0
列表:
列表元素添加:使用内置方法 append()、extend()、insert() 将元素添加到列表中。
>>> list1 = [1, 2, 3]
>>> list1.append(4)
>>> list1
[1, 2, 3, 4]
>>> list2 = [5, 6, 7]
>>> list1.extend(list2)
>>> list1
[1, 2, 3, 4, 5, 6, 7]
>>> list1.insert(0, 0)
>>> list1
[0, 1, 2, 3, 4, 5, 6, 7]
列表元素删除:使用内置方法 remove() 删除列表中的指定元素,使用 pop() 删除列表中的最后一个元素。
>>> list1 = [1, 2, 3, 4, 5]
>>> list1.remove(3)
>>> list1
[1, 2, 4, 5]
>>> list1.pop()
5
>>> list1
[1, 2, 4]
元组:
元组元素访问:使用方括号 [] 访问元组中的单独元素。
>>> tuple1 = (1, 2, 3)
>>> tuple1[0]
1
元组解包:将元组中的元素解包到多个变量中。
>>> tuple1 = (1, 2, 3)
>>> a, b, c = tuple1
>>> a
1
>>> b
2
>>> c
3
字典:
字典元素添加:使用方括号 [] 将元素添加到字典中。
>>> dict1 = {'name': 'John Doe', 'age': 30}
>>> dict1['gender'] = 'Male'
字典元素访问:使用方括号 [] 访问字典中的单独元素。
>>> dict1
{'name': 'John Doe', 'age': 30, 'gender': 'Male'}
>>> dict1 = {'name': 'John Doe', 'age': 30}
>>> dict1['name']
'John Doe'
字典元素删除:使用内置方法 pop() 删除字典中的指定元素。
>>> dict1 = {'name': 'John Doe', 'age': 30}
>>> dict1.pop('name')
'John Doe'
>>> dict1
{'age': 30}
控制语句:
if 语句:根据特定的条件执行代码。
>>> x = 10
>>> y = 20
>>> if x > y:
... print("x is greater than y")
... else:
... print("x is not greater than y")
...
'x is not greater than y'
for 循环:遍历列表、字符串、元组等序列中的所有元素。
>>> list1 = [1, 2, 3, 4, 5]
>>> for i in list1:
... print(i)
...
1
2
3
4
5
while 循环:当特定的条件为真时,重复执行代码。
>>> i = 1
>>> while i <= 5:
... print(i)
... i = i + 1
...
1
2
3
4
5
函数:
定义函数:使用关键字 def 定义函数。
>>> def add(x, y):
... return x + y
...
>>> add(10, 20)
30
调用函数:使用函数名和参数列表调用函数。
>>> def add(x, y):
... return x + y
...
>>> add(10, 20)
30
模块:
导入模块:使用关键字 import 导入模块。
>>> import math
>>> math.pi
3.141592653589793
从模块导入特定的函数:使用关键字 from 导入模块中的特定函数。
>>> from math import pi
>>> pi
3.141592653589793
文件 I/O:
读取文件:使用内置函数 open() 打开文件,使用方法 read() 读取文件内容。
>>> file = open("file.txt", "r")
>>> content = file.read()
>>> print(content)
'This is a text file.'
写入文件:使用内置函数 open() 打开文件,使用方法 write() 写入文件内容。
>>> file = open("file.txt", "w")
>>> file.write("This is a text file.")
>>> file.close()
不断总结,不断学习进步。
【开发云】年年都是折扣价,不用四处薅羊毛 https://dev.csdn.net/activity?utm_source=sale_source&sale_source=q4AnCOkys7
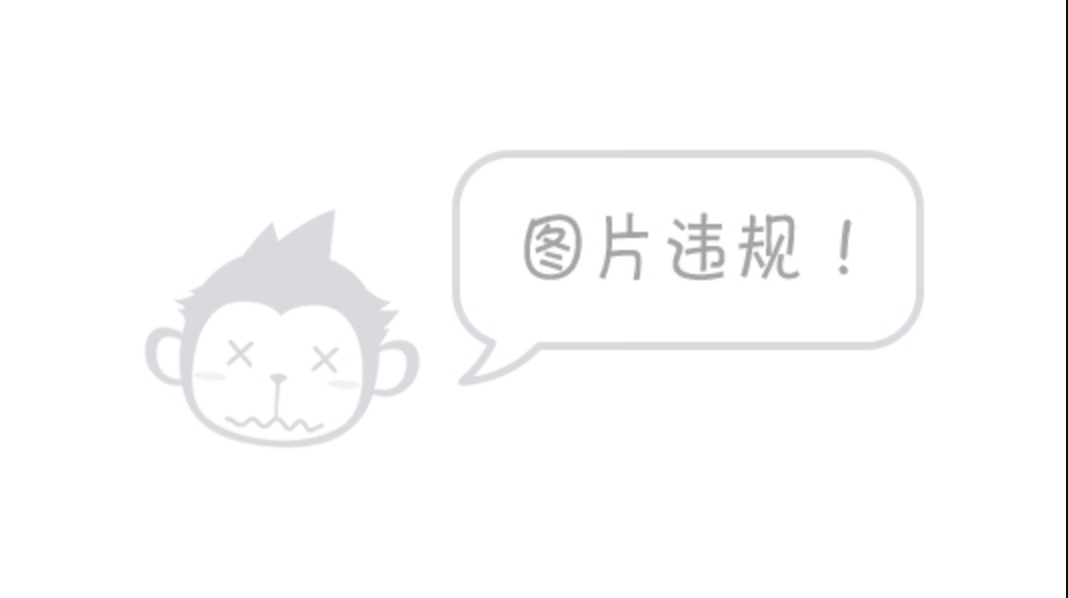