1.知识点

2.源程序
#include <iostream>
#include <string>
#include <tchar.h>
#include <map>
using namespace std;
int _tmain(int argc, _TCHAR* argv[])
{
cout << "1.map对象的默认构造" << endl;
map<int, char> map1;
map<string, float>map2;
cout << "\n\n";
cout << "2.map的插入与迭代器" << endl;
map<int, string> mapStu;
cout << "2.1 通过pair的方式插入对象" << endl;
mapStu.insert(pair<int, string>(3, "小张"));
cout << "2.2 通过value_type的方式插入对象" << endl;
mapStu.insert(map<int,string>::value_type(1,"小李"));
cout << "2.3 通过数组的方式插入值" << endl;
mapStu[4] = "小刘";
mapStu[5] = "小王";
string strName = mapStu[2];
cout << "遍历:法一 采用迭代器" << endl;
for (map<int, string>::iterator it = mapStu.begin(); it != mapStu.end(); ++it)
{
pair<int, string> pr = *it;
int iKey = pr.first;
string strValue = pr.second;
cout << "mapStu的关键字key:" << pr.first << " " << "mapStu的关键字的值value:" << pr.second << endl;
}
cout << "\n\n";
cout << "遍历:法二 采用数组的方式" << endl;
for (int it = 1; it < 6; ++it)
{
cout << "mapStu的关键字key:" << it << " " << "mapStu的关键字的值value:" << mapStu[it] << endl;
}
cout << "\n\n";
cout << "3.map的查找" << endl;
map<int, string> mapA;
mapA.insert(pair<int, string>(3, "小张"));
mapA.insert(pair<int, string>(1, "小杨"));
mapA.insert(pair<int, string>(7, "小赵"));
mapA.insert(pair<int, string>(5, "小王"));
cout << "3.1 map.find(key)" << endl;
map<int, string>::iterator itFind = mapA.find(3);
if (itFind == mapA.end())
{
cout << "没有找到" << endl;
}
else
{
pair<int, string>pairMapA = *itFind;
int iID = itFind->first;
string strName = itFind->second;
cout << "mapA的关键字key:" << iID << " " << "mapA的关键字的值value:" << strName << endl;
}
cout << "\n\n";
cout << "3.2 map.lower_bound(keyElem);返回第一个key>=keyElem元素的迭代器。" << endl;
map<int, string>::iterator itLower_bound = mapA.lower_bound(3);
cout << "mapA的关键字key:" << itLower_bound->first << " " << "mapA的关键字的值value:" << itLower_bound->second << endl << endl;
cout << "3.3 map.upper_bound(keyElem);返回第一个key>keyElem元素的迭代器。" << endl;
map<int, string>::iterator itUpper_bound = mapA.upper_bound(3);
pair<int, string>pairItUpper_bound = *itUpper_bound;
cout << "mapA的关键字key:" << pairItUpper_bound.first << " " << "mapA的关键字的值value:" << pairItUpper_bound.second << endl << endl;
cout << "3.4 map.equal_range(keyElem); " << endl;
pair<map<int, string>::iterator, map<int, string>::iterator> pairEqual_range = mapA.equal_range(3);
cout << "mapA的第一个关键字key:" << (pairEqual_range.first)->first << " " << "mapA的第一个关键字的值value:" << (pairEqual_range.first)->second << endl;
cout << "mapA的第二个关键字key:" << (pairEqual_range.second)->first << " " << "mapA的第二个关键字的值value:" << (pairEqual_range.second)->second << endl << endl;
return 0;
}
3.运行结果
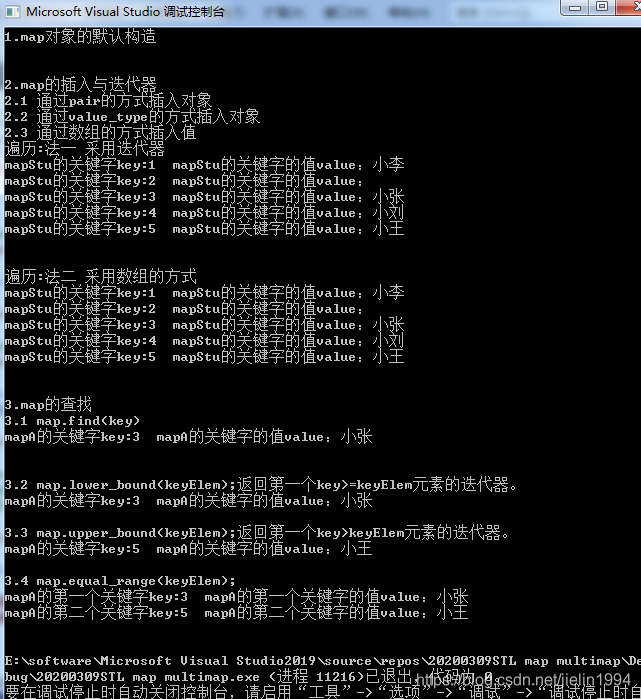