vue-amap中文文档: https://www.wenjiangs.com/doc/mdxkhhtr
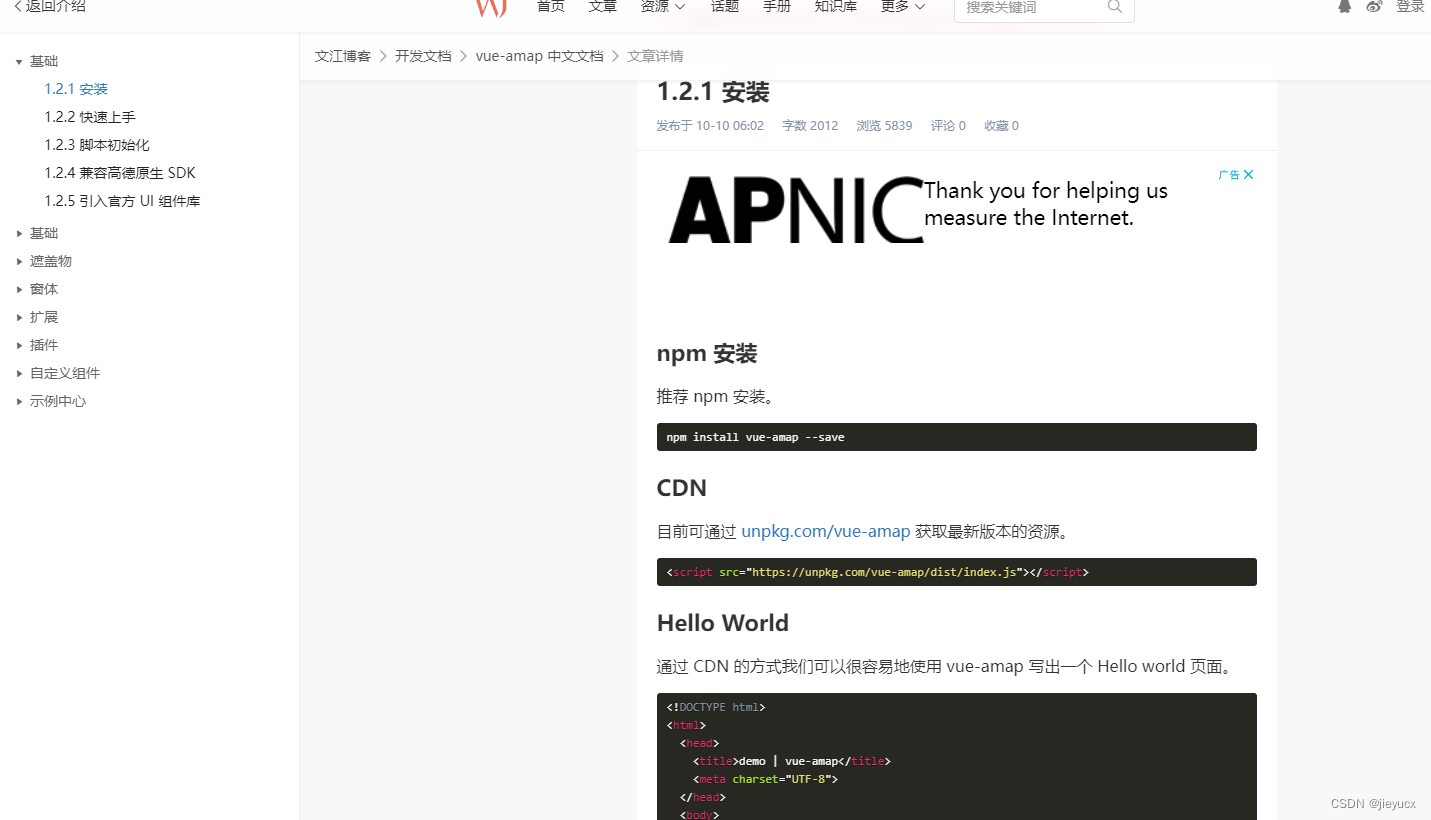
一、在vue项目中安装和基本使用
1. 安装
npm install vue-amap --save
2. 在vue项目中使用
1. 在main.js中引入
import VueAMap from 'vue-amap';
Vue.use(VueAMap);
VueAMap.initAMapApiLoader({
key: "你的key",
plugin: [
"AMap.Scale",
"AMap.OverView",
"AMap.ToolBar",
"AMap.MapType",
"AMap.DistrictSearch",
],
v: "1.4.4",
});
window._AMapSecurityConfig = {
securityJsCode: "你的安全密钥",
};
2. demo显示高德地图
<template>
<div>
<div class="amap-page-container">
<el-amap
ref="map"
vid="amapDemo"
:center="center"
:zoom="zoom"
:plugin="plugin"
:events="events"
class="amap-demo"
>
</el-amap>
</div>
</div>
</template>
<script>
export default {
data() {
return {
zoom: 12,
center: [121.59996, 31.197646],
events: {
init: (o) => {
console.log(o.getCenter());
console.log(this.$refs.map.$$getInstance());
o.getCity((result) => {
console.log(result);
});
},
moveend: () => {},
zoomchange: () => {},
click: (e) => {
alert("map clicked");
},
},
plugin: [
"ToolBar",
{
pName: "MapType",
defaultType: 0,
events: {
init(o) {
console.log(o);
},
},
},
],
};
},
};
</script>
<style lang="less" scoped>
.amap-page-container {
width: 100%;
height: 900px;
position: relative;
.amap-demo {
width: 100%;
height: 100%;
}
}
</style>
效果如图:
二、显示区域
效果如图:
代码如下:
<template>
<div>
<div class="amap-page-container">
<el-amap
ref="map"
vid="amapDemo"
:center="center"
:zoom="zoom"
:plugin="plugin"
:events="events"
class="amap-demo"
>
</el-amap>
</div>
</div>
</template>
<script>
export default {
data() {
return {
zoom: 12,
center: [121.59996, 31.197646],
events: {
init: this.initMap,
moveend: () => {},
zoomchange: () => {},
click: (e) => {
alert("map clicked");
},
},
plugin: [
"ToolBar",
{
pName: "MapType",
defaultType: 0,
events: {
init(o) {
console.log(o);
},
},
},
],
};
},
methods: {
initMap(map) {
setTimeout(() => {
this.drawBounds(map)
}, 200)
},
drawBounds(map) {
let opts = {
subdistrict: 0,
extensions: 'all',
level: 'city'
}
let district = new AMap.DistrictSearch(opts)
let polygons = []
district.setLevel('上海市')
district.search("上海市", (status, result) => {
map.remove(polygons)
polygons = [];
let bounds = result.districtList[0].boundaries;
if (bounds) {
for (let i = 0, l = bounds.length; i < l; i++) {
let polygon = new AMap.Polygon({
strokeWeight: 3,
path: bounds[i],
fillOpacity: 0.4,
fillColor: '#111e4b',
strokeColor: '#ffffff',
height: 100,
extrusionHeight: 100
});
polygons.push(polygon);
}
}
map.add(polygons)
map.setFitView(polygons);
})
},
}
};
</script>
<style lang="less" scoped>
.amap-page-container {
width: 100%;
height: 900px;
position: relative;
.amap-demo {
width: 100%;
height: 100%;
}
}
</style>
三、只显示某个区域
效果如图:
代码如下:
<template>
<div>
<div class="amap-page-container">
<el-amap
ref="map"
vid="amapDemo"
:center="center"
:zoom="zoom"
:plugin="plugin"
:events="events"
class="amap-demo"
>
</el-amap>
</div>
</div>
</template>
<script>
export default {
data() {
return {
zoom: 12,
center: [121.59996, 31.197646],
events: {
init: this.initMap,
moveend: () => {},
zoomchange: () => {},
click: (e) => {
alert("map clicked");
},
},
plugin: [
"ToolBar",
{
pName: "MapType",
defaultType: 0,
events: {
init(o) {
console.log(o);
},
},
},
],
};
},
methods: {
initMap(map) {
setTimeout(() => {
this.drawBounds(map)
}, 200)
},
drawBounds(map) {
let opts = {
subdistrict: 0,
extensions: 'all',
level: 'city'
}
let district = new AMap.DistrictSearch(opts)
let polygons = []
district.setLevel('上海市')
district.search("上海市", (status, result) => {
map.remove(polygons)
polygons = [];
let bounds = result.districtList[0].boundaries;
if (bounds) {
for (let i = 0, l = bounds.length; i < l; i++) {
let polygon = new AMap.Polygon({
strokeWeight: 3,
path: bounds[i],
fillOpacity: 0.4,
fillColor: '#ffffff',
strokeColor: '#ffffff',
});
polygons.push(polygon);
}
}
map.add(polygons)
map.setFitView(polygons);
let outer = [
new AMap.LngLat(-360, 90, true),
new AMap.LngLat(-360, -90, true),
new AMap.LngLat(360, -90, true),
new AMap.LngLat(360, 90, true),
]
let holes = result.districtList[0].boundaries
console.log('holes', holes)
let pathArray = [
outer
]
pathArray.push.apply(pathArray, holes)
let polygon = new AMap.Polygon({
pathL: pathArray,
strokeColor: "#ffffff",
strokeWeight: 3,
strokeOpacity: 1,
fillColor: '#031f52',
fillOpacity: 1,
});
polygon.setPath(pathArray)
map.add(polygon)
})
},
}
};
</script>
<style lang="less" scoped>
.amap-page-container {
width: 100%;
height: 900px;
position: relative;
.amap-demo {
width: 100%;
height: 100%;
}
}
</style>
四、3D棱柱区域
效果如图:
代码如下:
<template>
<div>
<div class="amap-page-container">
<el-amap
ref="map"
vid="amapDemo"
:center="center"
:zoom="zoom"
:plugin="plugin"
:events="events"
:pitch="pitch"
viewMode="3D"
class="amap-demo"
>
</el-amap>
</div>
</div>
</template>
<script>
export default {
data() {
return {
pitch: 60,
zoom: 12,
center: [121.59996, 31.197646],
events: {
init: this.initMap,
moveend: () => {},
zoomchange: () => {},
click: (e) => {
alert("map clicked");
},
},
plugin: [
"ToolBar",
{
pName: "MapType",
defaultType: 0,
events: {
init(o) {
console.log(o);
},
},
},
],
};
},
methods: {
initMap(map) {
setTimeout(() => {
this.drawBounds(map);
}, 200);
},
drawBounds(map) {
map.AmbientLight = new AMap.Lights.AmbientLight([1, 1, 1], 0.5);
map.DirectionLight = new AMap.Lights.DirectionLight(
[0, 0, 1],
[1, 1, 1],
1
);
let object3Dlayer = new AMap.Object3DLayer();
map.add(object3Dlayer);
let opts = {
subdistrict: 0,
extensions: "all",
level: "city",
};
let district = new AMap.DistrictSearch(opts);
let polygons = [];
district.setLevel("上海市");
district.search("上海市", (status, result) => {
map.remove(polygons);
polygons = [];
let bounds = result.districtList[0].boundaries;
let height = 50000;
let color = "#0088ffcc"; // #0088ffcc; #111e4bcc
let prism = new AMap.Object3D.Prism({
path: bounds,
height: height,
color: color,
});
prism.transparent = true;
object3Dlayer.add(prism);
if (bounds) {
for (let i = 0, l = bounds.length; i < l; i++) {
let polygon = new AMap.Polygon({
strokeWeight: 3,
path: bounds[i],
fillOpacity: 0.4,
fillColor: "#ffffff",
strokeColor: "#ffffff",
});
polygons.push(polygon);
}
}
map.add(polygons);
map.setFitView(polygons);
});
},
},
};
</script>
<style lang="less" scoped>
.amap-page-container {
width: 100%;
height: 900px;
position: relative;
.amap-demo {
width: 100%;
height: 100%;
}
}
</style>
五、添加卫星图层
效果如图:
代码如下:
<template>
<div>
<div class="amap-page-container">
<el-amap
ref="map"
vid="amapDemo"
:center="center"
:zoom="zoom"
:plugin="plugin"
:events="events"
:pitch="pitch"
viewMode="3D"
class="amap-demo"
>
</el-amap>
</div>
</div>
</template>
<script>
export default {
data() {
return {
pitch: 0,
zoom: 6,
center: [121.59996, 31.197646],
events: {
init: this.initMap,
moveend: () => {},
zoomchange: () => {},
click: (e) => {
alert("map clicked");
},
},
plugin: [
"ToolBar",
{
pName: "MapType",
defaultType: 0,
events: {
init(o) {
console.log(o);
},
},
},
],
};
},
methods: {
initMap(map) {
setTimeout(() => {
this.setSatelliteLayer();
}, 200);
},
setSatelliteLayer() {
const tileLayer = new AMap.TileLayer.Satellite({
map: this.$refs.map.$$getInstance(),
});
tileLayer.show();
},
},
};
</script>
<style lang="less" scoped>
.amap-page-container {
width: 100%;
height: 900px;
position: relative;
.amap-demo {
width: 100%;
height: 100%;
}
}
</style>