列队
- 队列是一个有序的列表,可以用数组或是链表来实现
- 遵循先进先出的原则。
思路
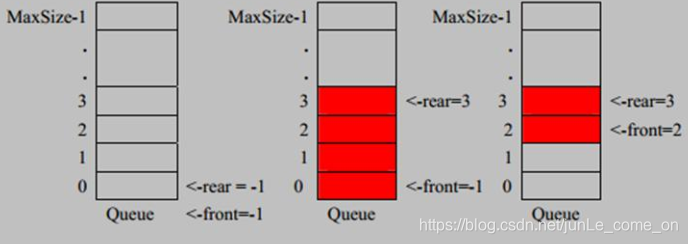
- front 表示列头的前一个位置,初始值为 front = -1
- rear 表示列尾, 初始值为 rear = -1
- 当front == rear 表示队列为空
- 当rear = maxSize - 1 表示队列已满
- maxSize 表示队列的容量,maxSize - 1 表示数组最大的索引
代码实现
import java.util.Scanner;
public class ArrayQueueTest {
public static void main(String[] args) {
ArrayQueue arrayQueue = new ArrayQueue(3);
char key = ' ';
Scanner scanner = new Scanner(System.in);
boolean loop = true;
while (loop) {
System.out.println("s(show): 显示队列数据");
System.out.println("e(exit): 退出该程序");
System.out.println("a(add): 添加数据到队列");
System.out.println("g(get): 从队列中取出数据");
System.out.println("p(peek): 偷瞄列头数据");
key = scanner.next().charAt(0);
switch (key) {
case 's':
arrayQueue.showQueue();
break;
case 'e':
scanner.close();
loop = false;
System.out.println("程序退出,exit(0)");
break;
case 'a':
System.out.println("请输入一个数据");
int data = scanner.nextInt();
arrayQueue.addQueue(data);
break;
case 'g':
System.out.printf("取出的数据为%d\n", arrayQueue.getQueue());
break;
case 'p':
System.out.printf("偷瞄的列头数据为%d\n", arrayQueue.peekQueue());
break;
}
}
}
}
class ArrayQueue {
private int MaxSize;
private int front;
private int rear;
private int[] queue;
public ArrayQueue(int maxSize) {
this.MaxSize = maxSize;
queue = new int[this.MaxSize];
front = -1;
rear = -1;
}
public boolean isFull() {
return rear == MaxSize - 1;
}
public boolean isEmpty() {
return front == rear;
}
public void addQueue(int data) {
if (isFull()) {
System.out.println("队列已满,无法添加数据");
return ;
}
rear++;
queue[rear] = data;
}
public int getQueue() {
if (isEmpty()) {
throw new RuntimeException("队列为空,无法取出数据");
}
front++;
return queue[front];
}
public void showQueue() {
if (isEmpty()) {
System.out.println("队列为空,没有数据");
return ;
}
for (int i = 0; i < queue.length; i++) {
System.out.printf("queue[%d] = %d \n", i, queue[i]);
}
}
public int peekQueue() {
if (isEmpty()) {
throw new RuntimeException("队列为空,列头无数据");
}
return queue[front + 1];
}
}
结论
简单数组模拟队列实现-代码地址