一 ,工厂模式
工厂模式(Factory Pattern)是 Java 中最常用的设计模式之一。这种类型的设计模式属于创建型模式,它提供了一种创建对象的最佳方式。在工厂模式中,我们在创建对象时不会对客户端暴露创建逻辑,并且是通过使用一个共同的接口来指向新创建的对象。
由于工厂模式较为简单,就不进行详细的解释,文章中直接进行代码的演示举例,同学们有兴趣可以在这里看看:http://www.runoob.com/design-pattern/factory-pattern.html。
首先创建一个接口,以便规范后续的编码
public interface Animal { void draw(); } |
然后创建多个(本例创建两个)实现该接口规范的类
public class Cat implements Animal { public void draw() { // TODO Auto-generated method stub System.out.println("画一只猫"); } } |
public class Dog implements Animal { public void draw() { // TODO Auto-generated method stub System.out.println("画一只狗"); } } |
然后创建一个工厂类,用于根据用户需求生成指定的类的对象
public class AnimalFactory { public Animal getAnimal(String animal){ if(animal.equalsIgnoreCase("Dog")){ return new Dog(); } if(animal.equalsIgnoreCase("Cat")){ return new Cat(); } return null; } } |
public class Test1 { @Test public void test1(){ AnimalFactory aFactory=new AnimalFactory(); Animal cat=aFactory.getAnimal("Cat"); cat.draw(); Animal dog=aFactory.getAnimal("Dog"); dog.draw(); } } |
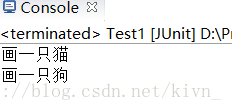
二,抽象工厂模式
抽象工厂模式(Abstract Factory Pattern)是围绕一个超级工厂创建其他工厂。该超级工厂又称为其他工厂的工厂。这种类型的设计模式属于创建型模式,它提供了一种创建对象的最佳方式。在抽象工厂模式中,接口是负责创建一个相关对象的工厂,不需要显式指定它们的类。每个生成的工厂都能按照工厂模式提供对象。
先创建一个画衣服的接口
public interface Clothes { void draw(); } |
再根据接口画出两种衣服
public class Shirt implements Clothes { public void draw() { // TODO Auto-generated method stub System.out.println("衬衣"); } } public class Coat implements Clothes { |
public interface Pants { void draw(); } |
public class Shorts implements Pants { public void draw() { // TODO Auto-generated method stub System.out.println("短裤"); } } public class Trousers implements Pants { |
创建抽象工厂的接口,用于规范抽象工厂的规范。
public interface AbstractFactory { public Clothes getClothes(String Clothes); public Pants getpants(String Pants); } |
public class FactoryProducer { public static AbstractFactory getFactory(String choice){ if(choice.equalsIgnoreCase("Pants")){ return new PantsFactory(); } if(choice.equalsIgnoreCase("Clothes")){ return new ClothesFactory(); } return null; } } |
public class ClothesFactory implements AbstractFactory { public Clothes getClothes(String Clothes) { if(Clothes.equalsIgnoreCase("Coat")){ return new Coat(); } if(Clothes.equalsIgnoreCase("Shirt")){ return new Shirt(); } return null; } public Pants getpants(String Pants) { return null; } } public class PantsFactory implements AbstractFactory { |
@Test public void test2(){ AbstractFactory aFactory= FactoryProducer.getFactory("Clothes"); Clothes c=aFactory.getClothes("Coat"); c.draw(); AbstractFactory bFactory=FactoryProducer.getFactory("Pants"); Pants pants=bFactory.getpants("Shorts"); pants.draw(); } |
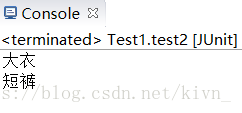