一、安装依赖包Pillow
pip install Pillow
二、验证码一般是放在一个项目的工具方法中
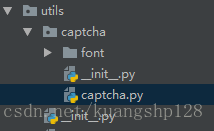
三、验证码的代码
from random import randint,choice
from PIL import Image,ImageDraw,ImageFont
from cStringIO import StringIO
from string import printable
def pillow_test():
font_path = "utils/captcha/font/Arial.ttf"
font_color = (randint(150, 200), randint(0, 150), randint(0, 150))
line_color = (randint(0, 150), randint(0, 150), randint(150, 200))
point_color = (randint(0, 150), randint(50, 150), randint(150, 200))
width, height = 100, 34
image = Image.new("RGB",(width, height),(200,200,200))
font = ImageFont.truetype(font_path,height - 10)
draw = ImageDraw.Draw(image)
text = "".join([choice(printable[:62]) for i in xrange(4)])
font_width, font_height = font.getsize(text)
draw.text((10, 10), text, font=font, fill=font_color)
for i in xrange(0, 5):
draw.line(((randint(0, width), randint(0, height)),
(randint(0, width), randint(0, height))),
fill=line_color, width=2)
for i in xrange(randint(100, 1000)):
draw.point((randint(0, width), randint(0, height)), fill=point_color)
out = StringIO()
image.save(out, format='jpeg')
content = out.getvalue()
out.close()
return text, content
四、创建一个视图返回验证码(基于tornado)
class TestHandler(BaseHandler):
def get(self):
text, img = pillow_test()
self.set_header("Content-Type", "image/jpg")
self.write(img)
五、直接在前端页面中的img
中使用这个视图