这段时间都是自主学习,有些无聊了,在我的强烈要求下我师父让我做一个类似于B站视频预览的效果,效果如下图,然后让我看video.js的文档来实现,现在做一个总结。
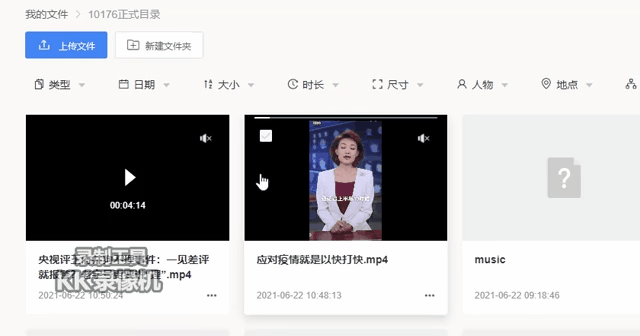
video.js安装及使用
npm install video.js
//在main.js中引入
import Video from 'video.js'
import 'video.js/dist/video-js.css'
Vue.prototype.$video = Video //引入Video播放器
//使用以及定义
<template>
<video ref="videoPlayer" class="video-js"></video>
</template>
data() {
return {
player: null,
loading: true,
videoOptions: {
// autoplay: "muted", //自动播放
autoplay: false,
controls: false, //用户可以与之交互的控件
loop: true, //视频一结束就重新开始
muted: true, //默认情况下将使所有音频静音
aspectRatio: '16:9', //显示比率
poster: '',
fullscreen: {
options: { navigationUI: 'hide' },
},
sources: '',
},
}
},
created() {
this.videoOptions.sources = this.url//定义视频来源
this.videoOptions.poster = this.poster //这个poster是父组件传过来的
},
mounted() {
let _this = this
//onPlayerReady方法是为了视频播放不了的时候不显示错误的信息
this.player = this.$video(this.$refs.videoPlayer, this.videoOptions, function onPlayerReady() {
this.on('error', function() {
this.errorDisplay.close_() //将错误信息不显示
})
this.on('canplaythrough', function() {
_this.loading = false
})
})
},
鼠标移入播放移出停止播放
handleMouseEnter() {
if (this.player) {
this.player.play()
}
this.$emit('videoTime', this.player.duration())
},
handleMouseLeave() {
if (this.player) {
this.player.pause()
}
},
根据鼠标移动位置播放对应帧及进度条显示
思路:根据鼠标在video中悬停的位置所占整个video宽度的百分比来显示当前视频的播放帧,进度条也一样的思路。
具体实现:
currentTime:当前播放的所在位置
duration:video的总时长
getBoundingClientRect:显示一个元素距离浏览器多少距离的集合
mouseMove(event) {//这个event是接收父组件传过来的鼠标位置
var videoleft=this.$refs.videoPlayer.getBoundingClientRect().left//video插件距离浏览器左边的距离
var clientx = event.clientX //鼠标距离浏览器左边的距离
var videoWidth = this.$refs.videoPlayer.clientWidth //div总宽度
var ratio = (clientx-videoleft) / videoWidth //当前鼠标移动的比率
var videoduration = this.player.duration() //总时长
this.player.currentTime(videoduration * ratio)
this.$emit('videoratio', ratio)
},
进度条实现
<!-- 进度条 -->
<span class="item-box-view-progress" v-show="item.Type == 1 && item.showPlay && item.showModal">
<el-row class="item-box-view-progress-ratio">
<el-col :span="videoplayerprogress"><div class="item-box-view-progress-ratio-arrive"></div></el-col>
<el-col :span="24 - videoplayerprogress"
><div class="item-box-view-progress-ratio-surplus"></div
></el-col>
</el-row>
</span>
<VideoPlayer
:poster="item.Mate ? item.Mate.picUrl : item.Thumbnail"
:url="item.PlayUrl"
:mute="item.mute"
:play="item.play"
:ref="'videoPlayer' + index"
@videoratio="videoratio"
></VideoPlayer>
videoplayerprogress: 0, //预览播放进度
videoratio(value) {
console.log(value)
this.videoplayerprogress = Math.round(24 * value)
},
PS:以上就完成了,这里的话是在父组件中通过ref调用子组件的mouseMove的方法,其实也可以都在子组件中去写,不需要用到父组件,因为是用了getBoundingClientRect这个方法,先获取到元素在浏览器中距离左侧的距离,然后获取鼠标在浏览器中距离左侧的距离,两个相减就是鼠标在元素中距离左侧的距离,然后除于div的宽度就可以了。这里一开始是因为video元素中还有2个div,鼠标经过的时候判断距离元素左侧是用的offsetx,这样就会判断成子元素的offsetx就不对了,后来才改用的getBoundingClientRect方法来做,通过父组件调用子组件也能跑就没去改了。