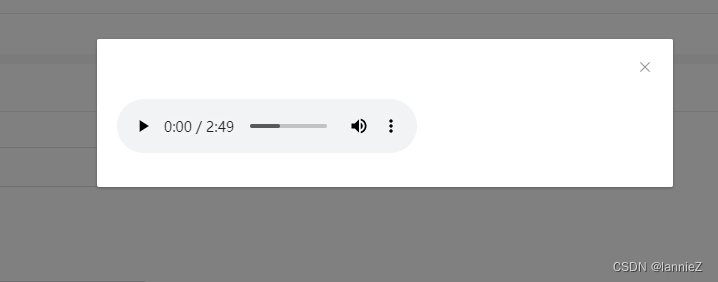
<audio ref="audio"
@pause="onPause"
@play="onPlay"
@timeupdate="onTimeupdate"
@loadedmetadata="onLoadedmetadata"
:src="list.voiceUrl"
controls="controls">
</audio>
<script>
function realFormatSecond(second) {
var secondType = typeof second
if (secondType === 'number' || secondType === 'string') {
second = parseInt(second)
var hours = Math.floor(second / 3600)
second = second - hours * 3600
var mimute = Math.floor(second / 60)
second = second - mimute * 60
return hours + ':' + ('0' + mimute).slice(-2) + ':' + ('0' + second).slice(-2)
} else {
return '0:00:00'
}
}
export default {
data() {
return {
audio: {
playing: false,
currentTime: 0,
maxTime: 0
}
}
},
filters: {
transPlayPause(value) {
return value ? '暂停' : '播放'
},
formatSecond(second = 0) {
return realFormatSecond(second)
}
},
methods: {
startPlayOrPause () {
return this.audio.playing ? this.pause() : this.play()
},
play () {
this.$refs.audio.play()
},
pause () {
this.$refs.audio.pause()
},
onPlay () {
this.audio.playing = true
},
onPause () {
this.audio.playing = false
},
onTimeupdate(res) {
console.log('timeupdate')
console.log(res)
this.audio.currentTime = res.target.currentTime
},
onLoadedmetadata(res) {
console.log('loadedmetadata')
console.log(res)
this.audio.maxTime = parseInt(res.target.duration)
}
},
};
</script>