动态添加表格数据(jQuery、Vue)
1、效果图
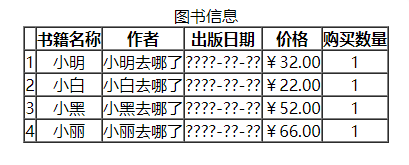
2、参考代码
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<table border="1" cellspacing="0" cellpadding="0" id="tb2" align="center" style="text-align: center;">
<caption>图书信息</caption>
<thead>
<tr>
<th></th>
<th>书籍名称</th>
<th>作者</th>
<th>出版日期</th>
<th>价格</th>
<th>购买数量</th>
</tr>
</thead>
<tbody></tbody>
</table>
</table>
<script src="../../qz/js/jquery-3.4.1.min.js"></script>
<script>
var books = [{
id: 1,
author: '小明',
name: '小明去哪了',
date: '????-??-??',
price: 32,
count: 1
}, {
id: 2,
author: '小白',
name: '小白去哪了',
date: '????-??-??',
price: 22,
count: 1
}, {
id: 3,
author: '小黑',
name: '小黑去哪了',
date: '????-??-??',
price: 52,
count: 1
}, {
id: 4,
author: '小丽',
name: '小丽去哪了',
date: '????-??-??',
price: 66,
count: 1
}]
var trLen = $("#tb2").find("tr").length;
var bLen = books.length;
while (true) {
if (bLen + 1 - trLen != 0 && bLen + 1 - trLen > 0) {
trLen++;
$("#tb2 tbody").append(
"<tr><td></td><td></td><td></td><td></td><td></td><td></td></tr>");
continue;
} else {
break;
}
}
var count = 0
$.each(books, function(i) {
count = 0;
$.each(books[i], function(j) {
$("#tb2 tr:nth(" + (i + 1) + ") td:nth(" + count + ")").text(books[i][j])
count++;
})
$("#tb2 tr:nth(" + (i + 1) + ") td:nth(4)").html("¥" + $("#tb2 tr:nth(" + (i + 1) + ") td:nth(4)").html() + ".00")
});
</script>
</body>
</html>
1、效果图
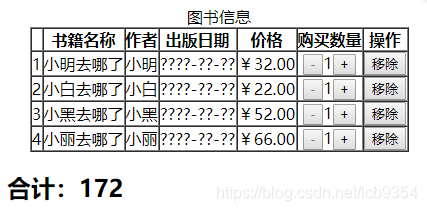
2、参考代码
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<div id="tb">
<table border="1" cellpadding="0" cellspacing="0" align="center" style="text-align: center;">
<caption>图书信息</caption>
<thead>
<tr>
<th></th>
<th>书籍名称</th>
<th>作者</th>
<th>出版日期</th>
<th>价格</th>
<th>购买数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<!-- 遍历books数组 -->
<tr v-for="(item,index) in books">
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{{item.author}}</td>
<td>{{item.date}}</td>
<td>{{item.price | showPrice}}</td>
<!-- :disabled="item.count<=1"==如果数目小于等于1,就不能再减少 -->
<td><button @click="reduceCount(index)" :disabled="item.count<=1">-</button>{{item.count}}<button @click="addCount(index)">+</button></td>
<td>
<button @click="removeRow(index)">移除</button>
</td>
</tr>
</tbody>
</table>
<h2>合计:{{totalPrice}}</h2>
</div>
<script src="../js/vue.js"></script>
<script>
const tb = new Vue({
el: '#tb',
data: {
books: [{
id: 1,
author: '小明',
name: '小明去哪了',
date: '????-??-??',
price: 32,
count: 1
}, {
id: 2,
author: '小白',
name: '小白去哪了',
date: '????-??-??',
price: 22,
count: 1
}, {
id: 3,
author: '小黑',
name: '小黑去哪了',
date: '????-??-??',
price: 52,
count: 1
}, {
id: 4,
author: '小丽',
name: '小丽去哪了',
date: '????-??-??',
price: 66,
count: 1
}]
},
methods: {
addCount(index) {
this.books[index].count++;
},
reduceCount(index) {
this.books[index].count--;
},
removeRow(index) {
this.books.splice(index, 1);
}
},
computed: {
totalPrice() {
let totalPrice = 0;
for (let i = 0; i < this.books.length; i++) {
totalPrice += this.books[i].count * this.books[i].price;
}
return totalPrice;
}
},
filters: {
showPrice(price) {
return "¥" + price.toFixed(2);
}
}
})
</script>
</body>
</html>