3.6编程练习
Demo30
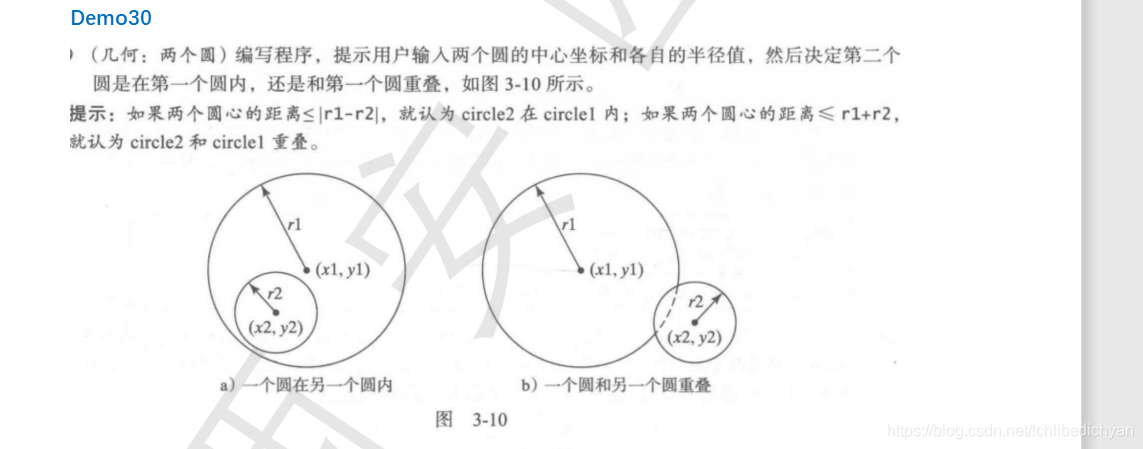
首先确定我们需要几个数据, 两个圆的圆心坐标 半径和两个圆的圆心距,当两个圆的圆心距小于等于两个圆半径的差说明小的圆在大的圆内,当两个圆的圆心距小于两个圆半径的和就说明两个圆相交,当两个圆的圆心距大于两个圆的半径之和,说明小的圆在大的圆外。
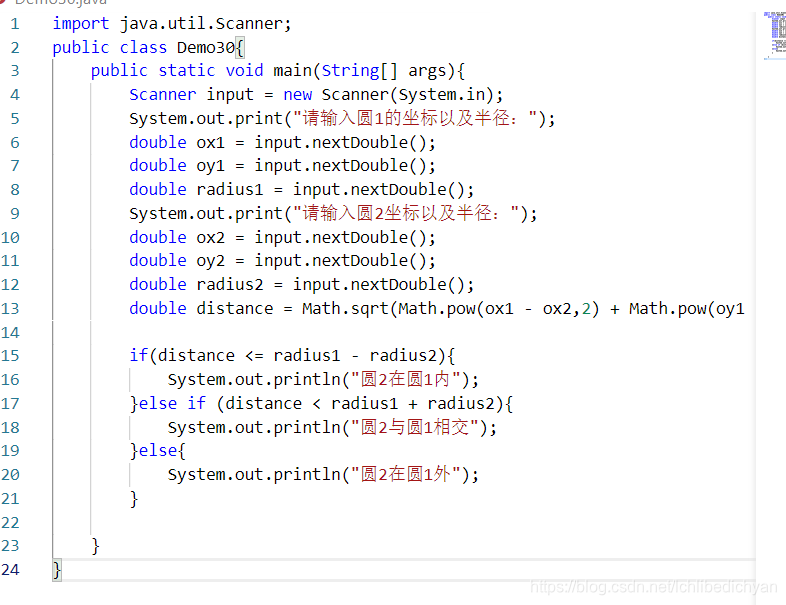
Demo32
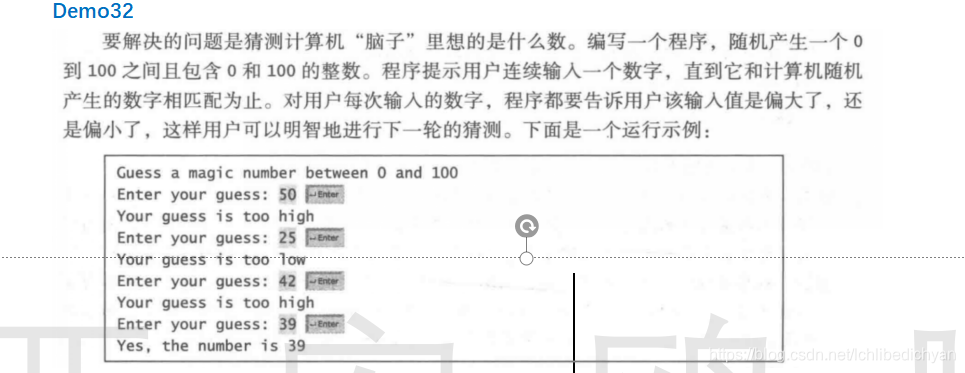
首先我们根据题目可得,我们在这题中需要有两个变量,一个是用户输入的变量,一个是电脑随机产生的变量,用户输入的变量可以多次输入,程序提示用户这个数对比电脑随机产生的数是大还是小,以此来猜出这个电脑随机出的数,我们在随机数上使用了random的方法来生成随机数,random本身的范围是[0,1),我们要使用一个在[a,b]范围内的整数可以使用[0,1) * (b - a + 1) + a ,来得到我们想要的范围,,通过对这两个数的比较,我们可以得到以下程序
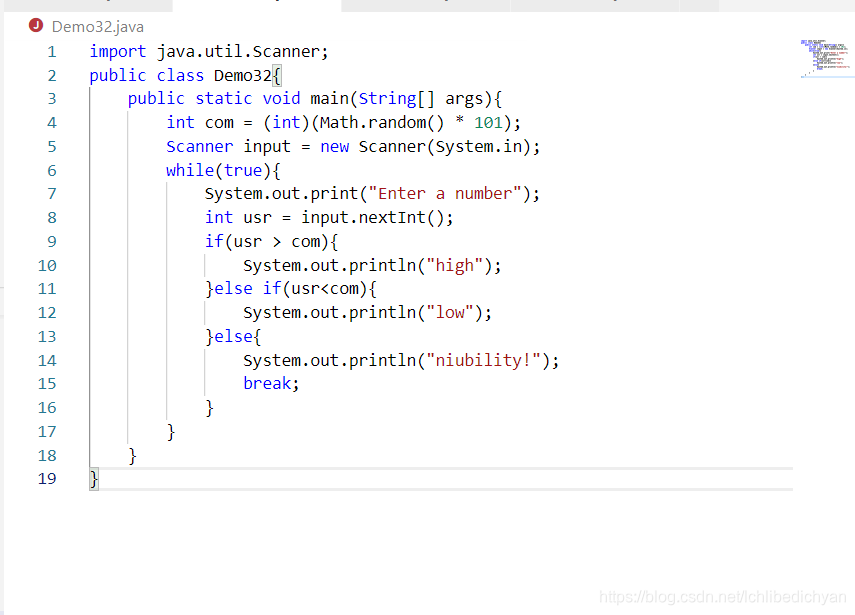
Demo33
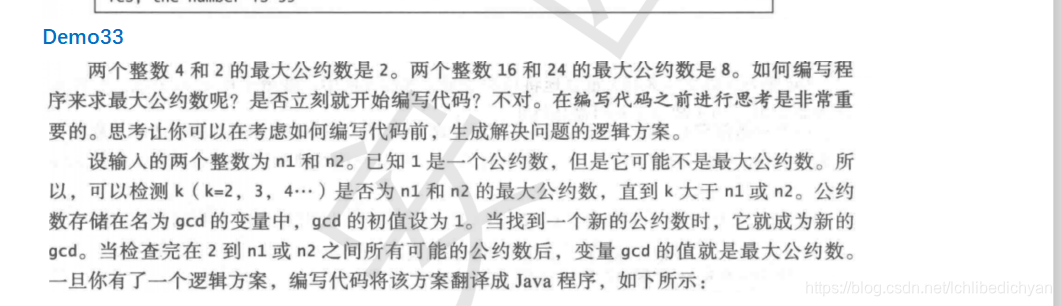
首先我们需要任意两个整数,我们首先可以去判断两个数的大小,而小的数就是我们循环的初始值,我们这个一小的数为初始值的循环就是我们能判断最大公约数的循环,我们对这个小的数进行递减,在设置判断条件能够同时整除这两个数的就是我们需要的最大公约数,以此我们可以得到如下程序
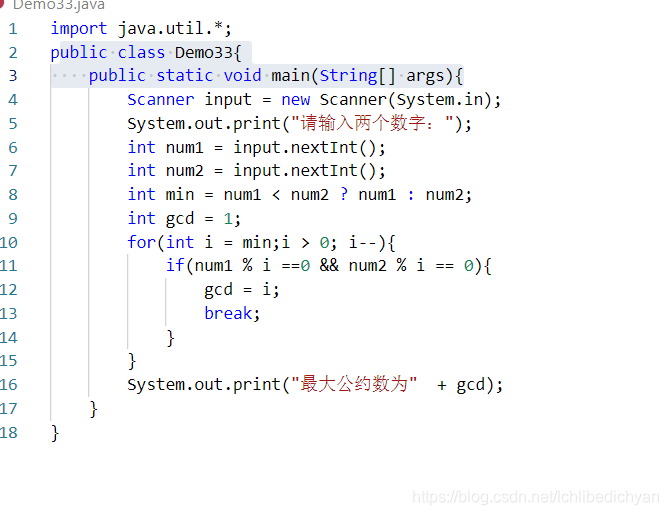
Demo35
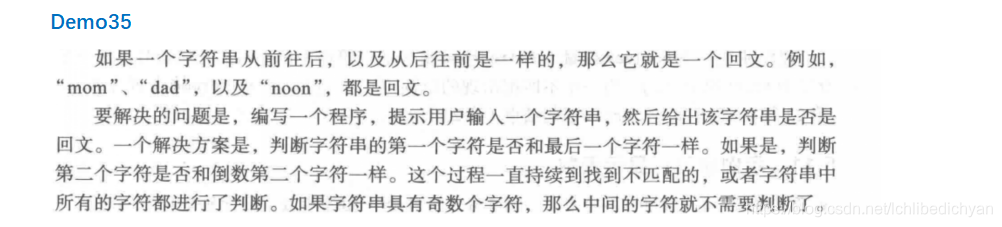
对于字符串的判断我们不能像对待数字那样进行简单的除和取余,我们通过str.charAt来获得每个字在字符串中的对应位置,我们可以通过定义两个变量,一个从零开始,一个从末尾开始,也就是str.length()-1,每次循环对零加一,对第二个减一就可以实现匹配,通过循环来匹配不同位置的字符是否相同,就可以得到该程序
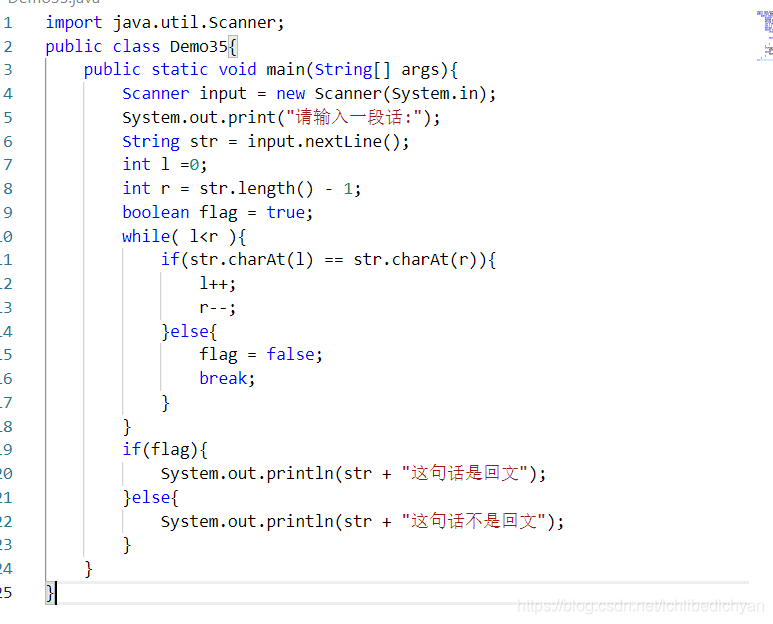
Demo36
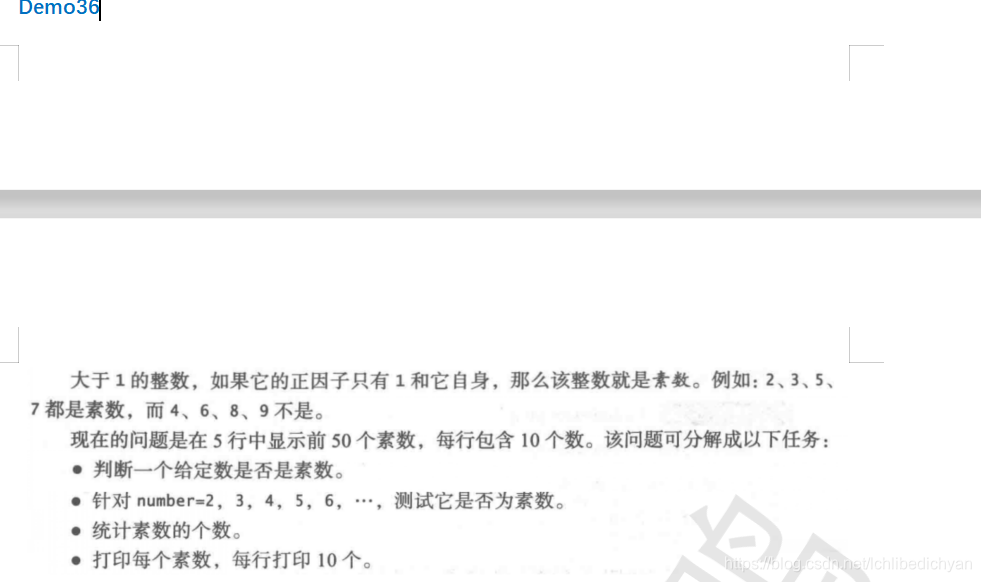
读完题,我们要把握住两个最主要的条件,一个是在5行中显示50个素数,一个就是对于一个数是否为素数的判断,而这两个问题都可以用循环完成,我们首先去实现50个素数,我们定义一个变量为零,每次循环加一就可以完成这个要求,第二个需求,我们需要判断一个数是否为素数,素数的定义是处理本身和一,其他数都不能对它进行整除,所以我们就需要写一个循环,把2到它本身-1的数都试一遍,同时我们可以发现,一旦除数到了这个数本身的1/2,就不可能发生整除了,所以我们只需要让它除到它的1/2就可以,根据以上我们就可以得到
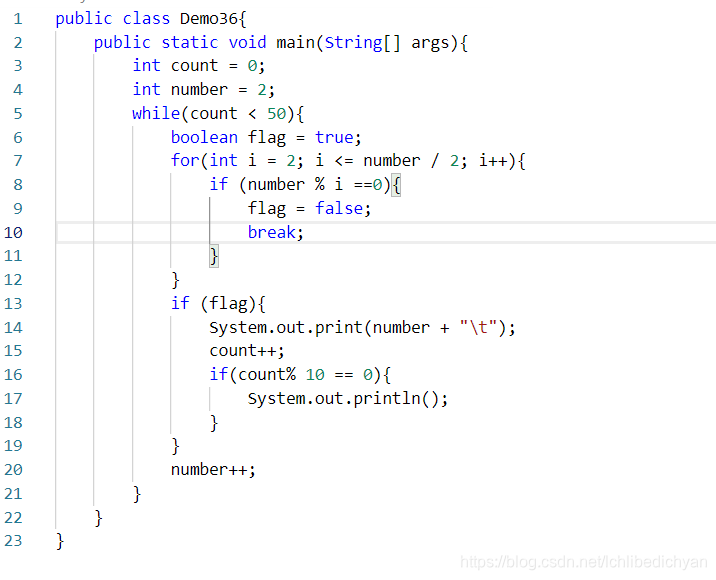
Demo49

该题是在前面一个问题的升级版,对于游戏本身我们可以直接使用上一题的代码,而我们只需要对用户和电脑赢的次数进行一个记录,在一方赢的次数达到2时停止就额可以,对于这个要求,我们可以先定义两个变量,另他们分别表示的人和电脑赢的次数,通过循环内嵌套判断,谁赢给谁加一,就可以完成程序
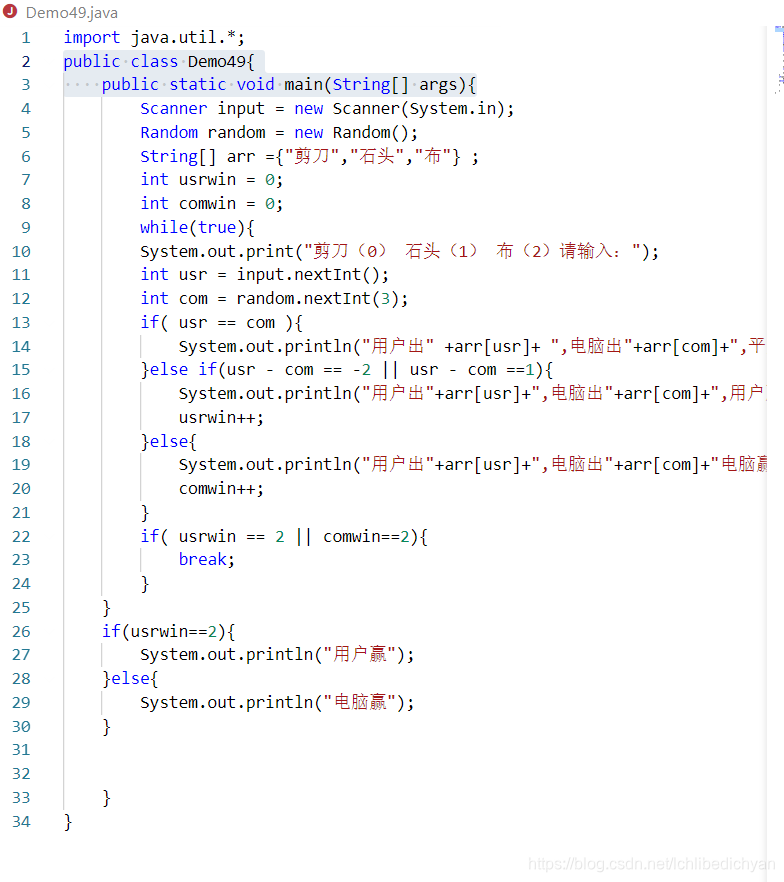
Demo50

我们知道,一个十进制数要转换成二进制数,要对这个十进制数一直除二取余数,我们先定义一个字符变量为空,让后通过循环来不停的对输入的数进行除二,然后跟这个字符变量相加,最后就可以得到这个二进制数
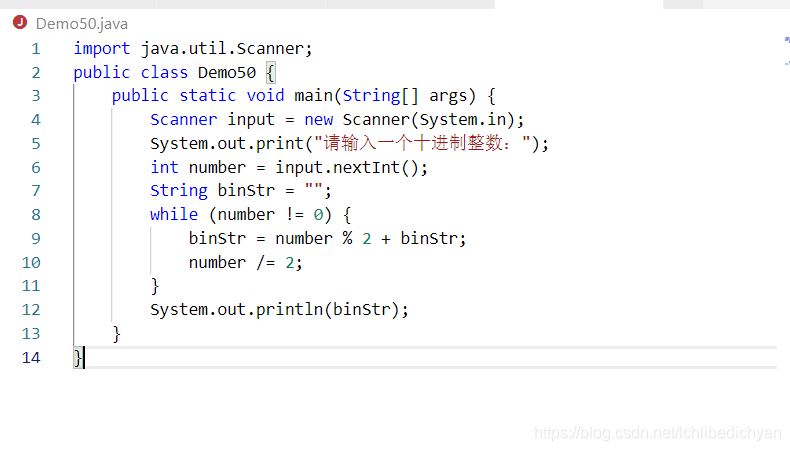
Demo52
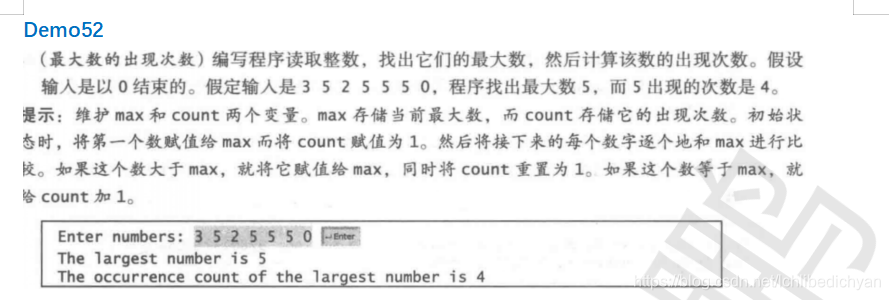
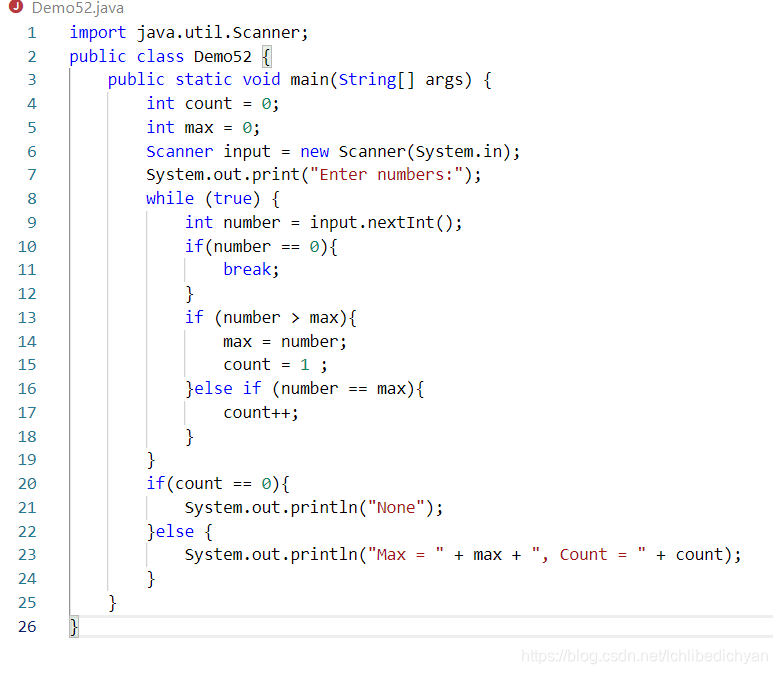
第四章常用类
4.1 Math类
Math类是用于数学计算的一个工具类 :对于工具类而言,里面的大部分成员都是静态的static
自带常量
static double E
: 自然对数
static double PI
: 圆周率
取整方法
static double ceil(double a)
: 向上取整
static double floor(double a)
:向下取整
static long round(double a)
: 四舍五入
三角函数
static double sin(double a)
:正弦函数 参数是弧度值
static double cos(double a)
:余弦函数
static double tan(double a)
:正切函数
static double toDegrees(double a)
:将弧度转角度
static double toRadians(double angles)
:将角度转弧度
static double asin(double a)
:反正弦函数
static double acos(double a)
:反余弦函数
static double atan(double a)
:反正切函数
指数函数
static double pow(double a, double b)
:求
a
的
b
次幂
static double sqrt(double a)
:求
a
的平方根
static double cbrt(double a)
:求
a
的立方根
其他方法
static double abs(double a)
:求
a
的绝对值
static double hypot(double deltX, double deltY)
:返回 两点间距离
static double max(a,b)
:返回
a
和
b
之间的最大值
static double min(a,b)
:返回
a
和
b
之间的最小值
static double random()
:返回
[0,1
)之间的随机小数
例:
public class
Sample
{
public static
void
main
(
String
[]
args
) {
System
.
out
.
println
(
Math
.
E
);
System
.
out
.
println
(
Math
.
PI
);
System
.
out
.
println
(
Math
.
ceil
(
3.1
));
System
.
out
.
println
(
Math
.
ceil
(
3.9
));
System
.
out
.
println
(
Math
.
ceil
(
-
3.1
));
System
.
out
.
println
(
Math
.
ceil
(
-
3.9
));
System
.
out
.
println
(
Math
.
floor
(
3.1
));
System
.
out
.
println
(
Math
.
floor
(
3.9
));
System
.
out
.
println
(
Math
.
floor
(
-
3.1
));
System
.
out
.
println
(
Math
.
floor
(
-
3.9
));
System
.
out
.
println
(
Math
.
round
(
3.1
));
System
.
out
.
println
(
Math
.
round
(
3.9
));
System
.
out
.
println
(
Math
.
round
(
-
3.1
));
System
.
out
.
println
(
Math
.
round
(
-
3.9
));
System
.
out
.
println
(
Math
.
sin
(
Math
.
PI
/
6
));
System
.
out
.
println
(
Math
.
cos
(
Math
.
PI
/
3
));
System
.
out
.
println
(
Math
.
tan
(
Math
.
PI
/
4
));
System
.
out
.
println
(
Math
.
toDegrees
(
Math
.
PI
/
2
));
System
.
out
.
println
(
Math
.
toRadians
(
90
));
System
.
out
.
println
(
Math
.
cbrt
(
8
));
System
.
out
.
println
(
Math
.
hypot
(
0
-
1
,
0
-
1
));
}
}
4.2 Scanner类
主要用于负责数据输入的类,底层是和
IO
流相关。
常用命令
String next()
:获取直到遇到空格为止的一个字符串
String nextLine()
:获取直到遇到回车为止的一个字符串
int nextInt()
:获取下一个整数
byte short long
double nextDouble()
boolean nextBoolean()
float nextFloat()
例:
import
java
.
util
.
Scanner
;
public class
Sample
{
public static
void
main
(
String
[]
args
) {
Scanner input
=
new
Scanner
(
System
.
in
);
System
.
out
.
print
(
"
输入一句话:
"
);
String
line
=
input
.
nextLine
();
System
.
out
.
println
(
line
);
System
.
out
.
print
(
"
输入三个单词:
"
);
String
word1
=
input
.
next
();
String
word2
=
input
.
next
();
String
word3
=
input
.
next
();
System
.
out
.
println
(
word1
);
System
.
out
.
println
(
word2
);
System
.
out
.
println
(
word3
);
}
}
4.3 Random类
主要用于产生随机数
boolean nextBoolean()
:随机产生布尔类型值
double nextDouble()
:随机生成
0.0~1.0
之间的小数
double nextInt()
:随机生成一个整数
0~2
32
的整数
double nextInt(n)
:随机生成一个整数
[0,n)
的整数
例:
import
java
.
util
.
Random
;
public class
Sample
{
public static
void
main
(
String
[]
args
) {
Random random
=
new
Random
();
for
(
int
i
=
1
;
i
<=
10
;
i
++
) {
System
.
out
.
print
(
random
.
nextBoolean
()
+
" "
);
}
System
.
out
.
println
();
for
(
int
i
=
1
;
i
<=
10
;
i
++
) {
System
.
out
.
print
(
random
.
nextInt
(
2
)
+
" "
);
}
System
.
out
.
println
();
for
(
int
i
=
1
;
i
<=
10
;
i
++
) {
System
.
out
.
print
(
random
.
nextInt
()
+
" "
);
}
System
.
out
.
println
();
}
}
4.4 String类
String
是一个类,它描述的是字符串。在
Java
代码当中,所有字符串常量(字符串字面量)都是
String
类的一个实例对象。并且,字符串一旦创建,则不可修改!
不可修改其长度,不可修改其内容
。所
以将来对字符串内容的改变,不能在原地改,只能重新创建一个字符串。
获取相关
1.char charAt(int index)
:获取指定角标
index
处的字符
2.int indexOf(int ch)
:获取指定字符
(
编码
)
在字符串中第一次(从左到右)出现的地方返回的是
角标。如果是
-1
表示不存在
3.int lastIndexOf(int ch)
:获取指定字符
(
编码
)
在字符串中第一次(从右到做)出现的地方返回的
是角标
4.int indexOf(String str)
:获取指定字符串在本字符串中第一次(从左到右)出现的地方返回的是
角标
5.int lastIndexOf(String str)
:获取指定字符串在本字符串中第一次(从右到左)出现的地方返回
的是角标
6.int length()
:获取字符串的长度(字符的个数)
7.String[] split(String regex)
:将字符串按照
regex
的定义进行切割(
regex
指的是正则表达式)
8.String substring(int beginIndex)
:截取一段子字符串,从
beginIndex
开始到结尾
9.String substring(int beginIndex, int endIndex)
:截取一段子字符串,从
beginIndex
开始到
endIndex(
不包含
)
判断相关
- int compareTo(String anotherString):按照字典顺序比较两个字符串的大小
- boolean contains(String another):判断当前字符串中是否包含指定字符串another
- boolean equals(String another):比较当前字符串与指定字符串的内容是否相同
- boolean isEmpty():判断当前字符串是否为空,length() == 0
- boolean startsWith(String prefix):判断该字符串是否以prefix开头
- boolean endsWith(String suwix):判断该字符串是否已suwix结尾
修改相关
- String toLowerCase():将字符串中所有的英文字母全部变为小写
- String toUpperCase():将字符串中所有的英文字母全部变为大写
- String trim():将字符串中两端的多余空格进行删除
- String replace(char oldCh,char newCh):将字符串中oldCh字符替换成newCh字符
例:
import
java
.
util
.
Random
;
public class
Sample
{
public static
void
main
(
String
[]
args
) {
String
str
=
"banana orange apple watermelon"
;
System
.
out
.
println
(
str
.
charAt
(
1
));
//'a'
System
.
out
.
println
(
str
.
indexOf
(
'o'
));
//7
System
.
out
.
println
(
str
.
lastIndexOf
(
97
));
//21
System
.
out
.
println
(
str
.
length
());
System
.
out
.
println
(
str
.
substring
(
6
));
System
.
out
.
println
(
str
.
substring
(
9
,
13
));
String
[]
arr
=
str
.
split
(
" "
);
System
.
out
.
println
(
arr
[
2
]);
String
s1
=
"abc"
;
String
s2
=
"abe"
;
System
.
out
.
println
(
s1
.
compareTo
(
s2
));
//
结果
<0 s1
在
s2
之前
==0 s1
和
s2
一样
>0 s1
在
s2
之后
System
.
out
.
println
(
"hehe"
.
compareTo
(
"hehe"
));
System
.
out
.
println
(
"abcbc"
.
contains
(
"bcb"
));
System
.
out
.
println
(
"lala"
.
equals
(
"lala"
));
String
s3
=
"
大桥未久
.avi"
;
System
.
out
.
println
(
s3
.
endsWith
(
".avi"
));
System
.
out
.
println
(
s3
.
startsWith
(
"
大桥未久
"
));
String
s4
=
"Happy Boy Happy Girl"
;
System
.
out
.
println
(
s4
.
toLowerCase
());
System
.
out
.
println
(
s4
.
toUpperCase
());
System
.
out
.
println
(
s4
);
System
.
out
.
println
(
" 123123 321321 "
.
trim
());
String
s5
=
"
旺财是只狗,旺财咋叫?旺财来叫一个
~"
;
System
.
out
.
println
(
s5
.
replace
(
'
狗
'
,
'
猫
'
));
System
.
out
.
println
(
s5
.
replace
(
"
旺财
"
,
"
小强
"
));
}
}
4.5 Character类
Character它是char基本数据类型的包装类 ,有这么几个静态方法我可目前可以使用到的
- static boolean isDigit(char ch):判断字符是否是数字
- static boolean isLetter(char ch):判断字符是否是字母
- static boolean isLetterOrDigit(char ch):判断字符是否是数字或字母
- static boolean isLowerCase(char ch):判断是否是小写字母
- static boolean isUpperCase(char ch):判断是否是大写字母
- static boolean isSpaceChar(char ch):判断是否空白字母(空格 制表符 回车)
例题
如何删除字符串中左右两端出现的空格
public class
Sample
{
public static
void
main
(
String
[]
args
) {
String
str
=
" 123123123 12123 "
;
//
去空格后
"123123123 12123"
int
l
=
0
;
int
r
=
str
.
length
()
-
1
;
while
(
str
.
charAt
(
l
)
==
' '
) {
l
++
;
}
while
(
str
.
charAt
(
r
)
==
' '
) {
r
--
;
}
System
.
out
.
println
(
"["
+
str
.
substring
(
l
,
r
+
1
)
+
"]"
);
}
}
在字符串
"abcbcbcbcbcbc"
中统计
"bcb"
出现的次数
public class
Sample
{
public static
void
main
(
String
[]
args
) {
//
最好的算法
KMP
算法
String
s1
=
"abcbcbcbcbcbc"
;
String
s2
=
"bcb"
;
//
贪心算法
5
个
int
count
=
0
;
for
(
int
i
=
0
;
i
<
s1
.
length
()
-
s2
.
length
()
+
1
;
i
++
) {
if
(
s1
.
charAt
(
i
)
==
s2
.
charAt
(
0
)) {
if
(
s2
.
equals
(
s1
.
substring
(
i
,
i
+
s2
.
length
()))) {
count
++
;
}
}
}
System
.
out
.
println
(
count
);
//
非贪心算法
3
个
/*
String temp = s1;
int count = 0;
while (true) {
int index = temp.indexOf(s2);
if (index == -1) {
break;
}
count++;
temp = temp.substring(index + s2.length());
}
System.out.println(count);
*/
}
}
注:贪心算法匹配不切割,布贪心算法匹配切割
查找两个字符串中最长的公共子串
public class
Sample
{
public static
void
main
(
String
[]
args
) {
String
s1
=
"c is a program but is diffcult"
;
String
s2
=
"Java is a program but is slow"
;
for
(
int
len
=
s2
.
length
();
len
>
0
;
len
--
) {
for
(
int
l
=
0
,
r
=
len
-
1
;
r
<
s2
.
length
();
l
++
,
r
++
) {
String
temp
=
s2
.
substring
(
l
,
r
+
1
);
if
(
s1
.
contains
(
temp
)) {
System
.
out
.
println
(
temp
);
return
;
//
直接结束程序
}
}
}
}
}
注:return和break的区别,break跳出当前循环或判断,return直接结束程序
将十六进制数字转为十进制
import
java
.
util
.
Scanner
;
public class
Sample
{
public static
void
main
(
String
[]
args
) {
Scanner input
=
new
Scanner
(
System
.
in
);
System
.
out
.
print
(
"
请输入一个十六进制的数字:
"
);
//0~9 A~F
String
hex
=
input
.
nextLine
().
toUpperCase
();
if
(
hex
.
length
()
==
0
) {
System
.
out
.
println
(
"
没有任何输入!
"
);
return
;
//
程序直接结束
}
for
(
int
i
=
0
;
i
<
hex
.
length
();
i
++
) {
char
ch
=
hex
.
charAt
(
i
);
if
(
Character
.
isDigit
(
ch
)
||
Character
.
isLetter
(
ch
)) {
if
(
Character
.
isLetter
(
ch
)
&&
(
ch
<
'A'
||
ch
>
'F'
)) {
System
.
out
.
println
(
"
非法字符
"
+
ch
);
return
;
}
}
else
{
System
.
out
.
println
(
"
非法字符
"
+
ch
);
return
;
}
}
int
sum
=
0
;
for
(
int
i
=
hex
.
length
()
-
1
;
i
>=
0
;
i
--
) {
char
ch
=
hex
.
charAt
(
i
);
if
(
Character
.
isDigit
(
ch
)) {
//'1' ? 1
sum
=
sum
+
(
int
)
Math
.
pow
(
16
,
hex
.
length
()
-
i
-
1
)
*
(
ch
-
48
);
}
else
{
//'A' - 65 + 10 -> 10
sum
=
sum
+
(
int
)
Math
.
pow
(
16
,
hex
.
length
()
-
i
-
1
)
*
(
ch
-
55
);
}
}
System
.
out
.
println
(
sum
);
}
}