最近公司项目有需要开发一个相机?要识别车牌号和VIN(车架号)要求如下:
1.要求扫描车牌号要竖屏显示。
2.要求扫描车架号要横屏显示。
并且在扫描的时候要遮挡区域只显示中间的矩形扫描框,拍照完成后只显示矩形框的图片,然后将图片上传上去进行识别返回车牌信息和VIN信息。这里项目做完了做了一个Dome希望可以帮到大家。先上两张图:
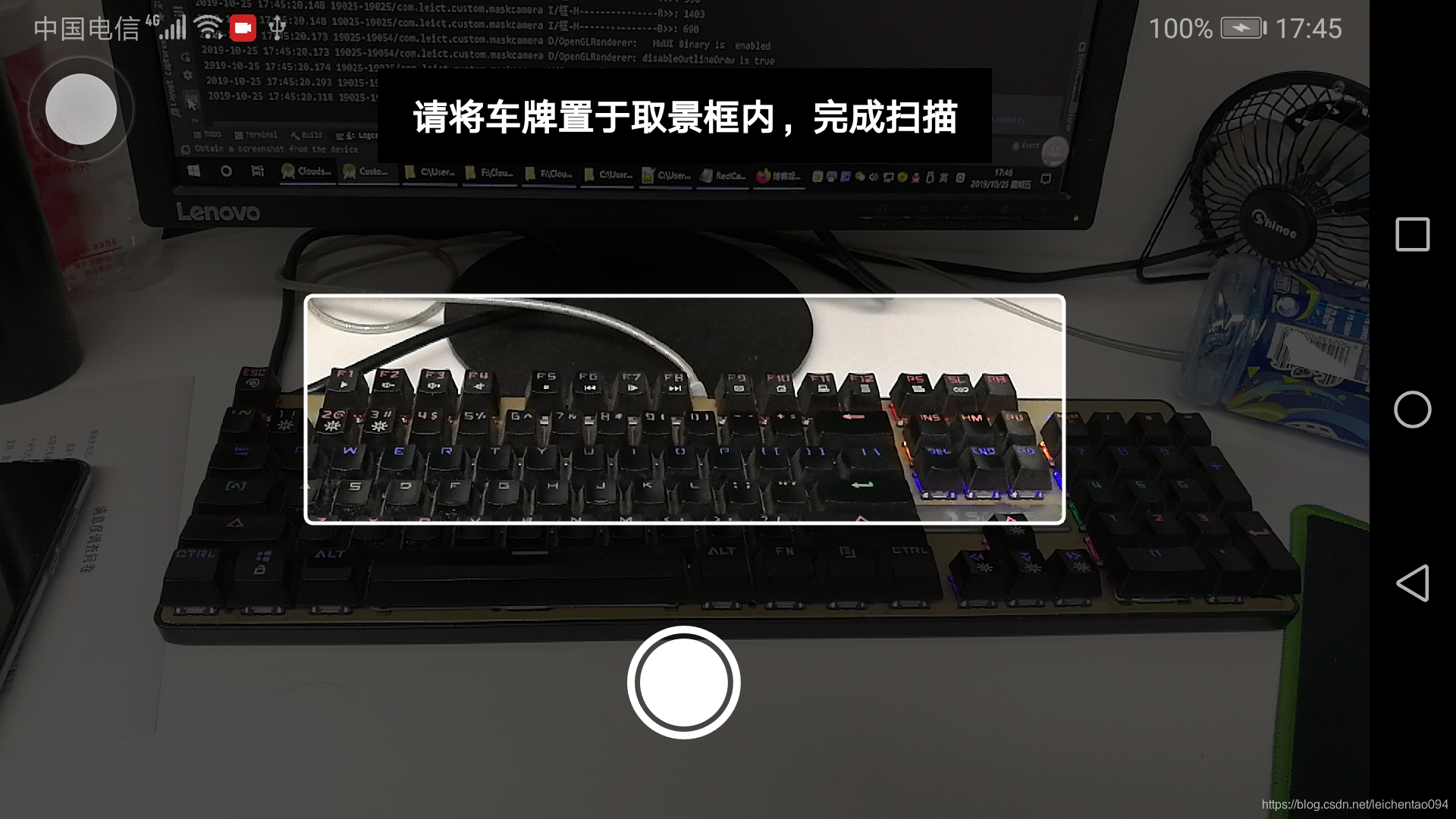
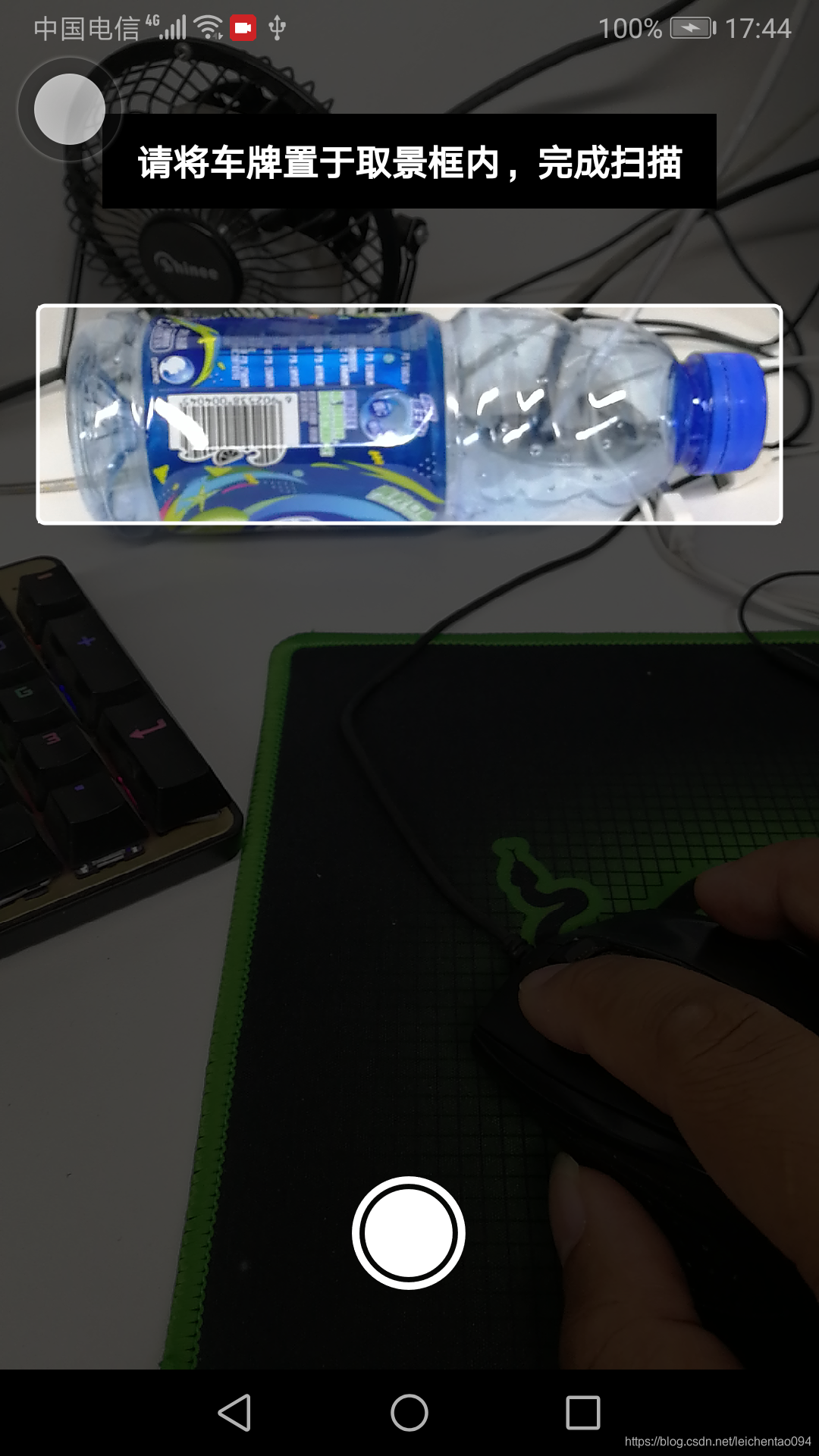
第一步:
package com.leict.custom.maskcamera.camera; import android.content.Context; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.ImageFormat; import android.graphics.Matrix; import android.hardware.Camera; import android.hardware.Camera.Parameters; import android.hardware.Camera.PictureCallback; import android.hardware.Camera.Size; import android.media.AudioManager; import android.media.ToneGenerator; import android.os.Environment; import android.util.Log; import android.view.SurfaceHolder; import com.leict.custom.maskcamera.utils.Utils; import com.leict.custom.maskcamera.views.PlateSurfaceView; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; import java.util.List; public class CameraPlateHelper { private final String TAG = CameraPlateHelper.class.getSimpleName(); private boolean isPreview; private static CameraPlateHelper helper; private Camera camera; private ToneGenerator tone; private PlateSurfaceView surfaceView; /** * 分辨率 */ private Size resolution; /** * 照片质量 */ private int picQuality = 100; /** * 照片尺寸 */ private Size pictureSize; /** * 上下文 */ private Context mContext; /** * 闪光灯模式(default:自动) */ private String flashStatus = Parameters.FLASH_MODE_AUTO; public enum Flashlight { AUTO, ON, OFF } private CameraPlateHelper(Context context) { this.mContext = context; } public static CameraPlateHelper getInstance(Context context) { if (helper == null) { synchronized (CameraPlateHelper.class) { if (helper == null) { helper = new CameraPlateHelper(context.getApplicationContext()); } } } return helper; } /** * 设置闪光灯模式 * * @param status * @return */ public CameraPlateHelper setFlashState(Flashlight status) { switch (status) { case AUTO: this.flashStatus = Parameters.FLASH_MODE_AUTO; break; case ON: this.flashStatus = Parameters.FLASH_MODE_ON; break; case OFF: this.flashStatus = Parameters.FLASH_MODE_OFF; break; default: this.flashStatus = Parameters.FLASH_MODE_AUTO; } return helper; } public CameraPlateHelper setMaskSurfaceView(PlateSurfaceView surfaceView) { this.surfaceView = surfaceView; return helper; } /** * 获取Camera对象 * * @return */ private Camera getCamera() { Camera camera; try { camera = Camera.open(); } catch (Exception e) { camera = null; e.printStackTrace(); } return camera; } /** * 打开相机并开启预览 * * @param holder SurfaceHolder * @param format 图片格式 * @param width SurfaceView宽度 * @param height SurfaceView高度 * @param screenWidth 屏幕宽度 * @param screenHeight 屏幕高度 */ public void openCamera(SurfaceHolder holder, int format, int width, int height, int screenWidth, int screenHeight) { if (this.camera != null) { this.camera.release(); } this.camera = getCamera(); this.setParameter(holder, format, width, height, screenWidth, screenHeight); this.startPreview(); } /** * 照相 */ public void tackPicture(final TakePhotoCallback callback) { this.camera.autoFocus(new Camera.AutoFocusCallback() { @Override public void onAutoFocus(boolean flag, Camera camera) { camera.takePicture(new Camera.ShutterCallback() { @Override public void onShutter() { if (tone == null) { //发出提示用户的声音 tone = new ToneGenerator(AudioManager.STREAM_MUSIC, ToneGenerator.MAX_VOLUME); } tone.startTone(ToneGenerator.TONE_PROP_BEEP2); } }, null, new PictureCallback() { @Override public void onPictureTaken(byte[] data, Camera camera) { String filepath = savePicture(data); boolean success = false; if (filepath != null) { success = true; } stopPreview(); callback.onCapture(success, filepath); } }); } }); } /** * 裁剪并保存照片 * * @param data * @return */ private String savePicture(byte[] data) { String filePath = Environment.getExternalStorageDirectory() + "/cbx/vehicle.jpg"; Bitmap bitmap = cutPicture(data); try { int length = filePath.length(); String strPath = filePath.substring(0, filePath.indexOf("v")); String strName = filePath.substring(filePath.indexOf("v"), length); if (Utils.isExist(strPath, strName)) { File imgFile = new File(filePath); FileOutputStream fos = new FileOutputStream(imgFile); BufferedOutputStream bos = new BufferedOutputStream(fos); bitmap.compress(Bitmap.CompressFormat.JPEG, 100, bos); fos.close(); bos.flush(); bos.close(); } } catch (IOException e) { e.printStackTrace(); } return filePath; } /** * 初始化相机参数 * * @param holder SurfaceHolder * @param format 图片格式 * @param width SurfaceView宽度 * @param height SurfaceView高度 * @param screenWidth 屏幕宽度 * @param screenHeight 屏幕高度 */ private void setParameter(SurfaceHolder holder, int format, int width, int height, int screenWidth, int screenHeight) { try { Parameters p = this.camera.getParameters(); this.camera.setPreviewDisplay(holder); if (width > height) { //横屏 this.camera.setDisplayOrientation(0); } else { //竖屏 this.camera.setDisplayOrientation(90); } //照片质量 p.set("jpeg-quality", picQuality); //设置照片格式 p.setPictureFormat(ImageFormat.JPEG); //设置闪光灯 p.setFlashMode(flashStatus); //设置最佳预览尺寸 List<Size> previewSizes = p.getSupportedPreviewSizes(); //设置预览分辨率 if (this.resolution == null) {