关于八大排序的算法思想我这里不再赘述,直接上代码。存在错误之处望指正,大家一起进步!
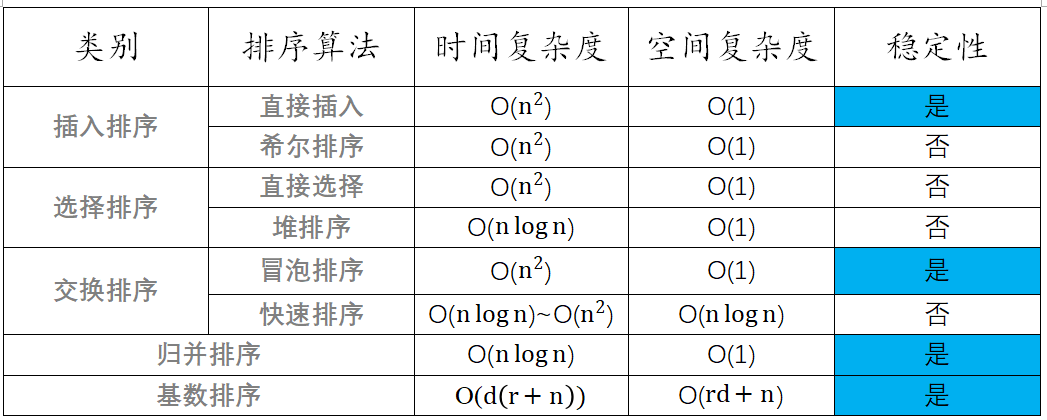
----------------------------------------------------------------------------------------------------------------------
一、直接插入(插入排序)
/**
* 直接插入(插入排序)
* */
public class
Insert_Sort_01 {
public static void
InsertSort(
int
[] array){
for
(
int
i=
1
; i<array.
length
; i++){
if
(array[i] < array[i-
1
]){
int
temp = array[i],j;
for
(j=i-
1
; j>-
1
&& temp <array[j]; j--){
array[j+
1
] = array[j];
}
array[j+
1
] = temp;
}
}
}
public static void
main(String[] args) {
int
[] a = {
3
,
2
,
5
,
8
,
4
,
7
,
6
,
9
};
InsertSort
(a);
for
(
int
i: a){
System.
out
.print(
" "
+i);
}
}
}
----------------------------------------------------------------------------------------------------------------------
二、希尔排序(插入排序)
/**
* 希尔排序(插入排序)
* */
public class
Shell_Sort_02 {
public static void
ShellSort(
int
[] array){
int
n = array.
length
;
int
h;
for
(h = n/
2
; h>
0
; h/=
2
){
for
(
int
i = h; i<n; i++){
for
(
int
j = i-h; j>=
0
; j-=h){
if
( array[j] > array[j+h] ){
int
temp = array[j];
array[j] = array[j+h];
array[j+h] = temp;
}
}
}
}
}
public static void
main(String[] args) {
int
[] a = {
3
,
2
,
5
,
8
,
4
,
7
,
6
,
9
};
ShellSort
(a);
for
(
int
i:a){
System.
out
.print(i+
" "
);
}
}
}
----------------------------------------------------------------------------------------------------------------------
三、直接选择(选择排序)
/**
* 直接选择(选择排序)
* */
public class
Select_Sort_03 {
public static void
SelectSort(
int
[] array) {
int
n = array.
length
;
for
(
int
i =
0
; i < n; i++) {
int
minIndex = i;
for
(
int
j = i +
1
; j < n; j++) {
if
(array[minIndex] > array[j]) {
minIndex = j;
}
}
if
(i != minIndex) {
int
temp = array[i];
array[i] = array[minIndex];
array[minIndex] = temp;
}
}
}
public static void
main(String[] args) {
int
[] a = {
3
,
2
,
5
,
8
,
4
,
7
,
6
,
9
};
SelectSort
(a);
for
(
int
i:a){
System.
out
.print(i+
" "
);
}
}
}
----------------------------------------------------------------------------------------------------------------------
四、堆排序(选择排序)
/**
*
堆排序(选择排序)
* */
public class
Heap_Sort_04 {
public static void
HeapSort(
int
[] array) {
//
创建最大堆:从最后一个节点的父节点开始
int
lastIndex = array.
length
-
1
;
int
startIndex = (lastIndex -
1
) /
2
;
for
(
int
i = startIndex; i >=
0
; i--) {
maxHeap
( array, array.
length
, i );
}
//
排序:末尾与头交换,逐一找出最大值,最终形成一个递增的有序序列
for
(
int
i = array.
length
-
1
; i >
0
; i--) {
int
temp = array[
0
];
array[
0
] = array[i];
array[i] = temp;
maxHeap
(array, i,
0
);
}
}
private static void
maxHeap(
int
[] data,
int
heapSize,
int
index) {
//
左子节点
int
leftChild =
2
* index +
1
;
//
右子节点
int
rightChild =
2
* index +
2
;
//
最大元素下标
int
largestIndex = index;
//
分别比较当前节点和左右子节点,找出最大值
if
( leftChild < heapSize && data[leftChild] > data[largestIndex] ) {
largestIndex = leftChild;
}
if
( rightChild < heapSize && data[rightChild] > data[largestIndex] ) {
largestIndex = rightChild;
}
//
如果最大值是子节点,则进行交换
if
( largestIndex != index ) {
int
temp = data[index];
data[index] = data[largestIndex];
data[largestIndex] = temp;
//
交换后,其子节点可能就不是最大堆了,需要对交换的子节点重新调整
maxHeap
(data, heapSize, largestIndex);
}
}
public static void
main(String[] args) {
int
[] array = {
3
,
2
,
5
,
8
,
4
,
7
,
6
,
9
};
HeapSort
(array);
for
(
int
i:array){
System.
out
.print(i+
" "
);
}
}
}
----------------------------------------------------------------------------------------------------------------------
五、冒泡排序(交换排序)
/**
* 冒泡排序(交换排序)
* */
public class
Bubble_Sort_05 {
public static void
BubbleSort_01(
int
[] array) {
int
len = array.
length
;
for
(
int
i =
0
; i < len; i++) {
for
(
int
j =
0
; j < len - i -
1
; j++) {
if
(array[j] > array[j +
1
]) {
int
temp = array[j +
1
];
array[j +
1
] = array[j];
array[j] = temp;
}
}
}
}
public static void
BubbleSort_02(
int
[] array){
int
len = array.
length
;
boolean
flag =
true
;
while
(flag){
flag =
false
;
for
(
int
i =
0
; i<len-
1
; i++){
if
(array[i] > array[i+
1
]){
int
temp = array[i+
1
];
array[i+
1
] = array[i];
array[i] = temp;
flag =
true
;
}
}
len--;
}
}
public static void
main(String[] args) {
int
[] array = {
3
,
2
,
5
,
8
,
4
,
7
,
6
,
9
};
BubbleSort_02
(array);
for
(
int
i:array){
System.
out
.print(i+
" "
);
}
}
}
----------------------------------------------------------------------------------------------------------------------
六、快速排序(交换排序)
/**
* 快速排序(交换排序)
* */
public class
Quick_Sort_06 {
public static void
QuickSort(
int
[] array,
int
low,
int
high){
int
t,i,j;
if
( high-low>
1
)
{
i=low+
1
;
j=high-
1
;
while
( i<=j )
{
while
( i<high && array[i]<array[low] )i++;
while
( j>low && array[j]>=array[low] )j--;
if
( i<j )
{
t=array[i];
array[i]=array[j];
array[j]=t;
}
}
if
( (j-low)!=
0
)
{
t=array[low];
array[low]=array[j];
array[j]=t;
QuickSort
(array,low,j);
QuickSort
(array,j+
1
,high);
}
}
}
public static void
main(String[] args) {
int
[] array = {
3
,
2
,
5
,
8
,
4
,
7
,
6
,
9
};
QuickSort
(array,
0
,array.
length
);
for
(
int
i :array){
System.
out
.print(i+
" "
);
}
}
}
----------------------------------------------------------------------------------------------------------------------
七、归并排序
/**
*归并排序
* */
public class
Merge_Sort_07 {
public static void
MergeSort(
int
[] a,
int
low,
int
high){
int
mid,i,j,k;
int
[] c =
new int
[
100
];
if
(high-low>
1
)
{
mid=(low+high)/
2
;
MergeSort
(a,low,mid);
MergeSort
(a,mid,high);
k=i=low;j=mid;
while
(i<mid||j<high)
{
if
(j>=high||i<mid&&a[i]<a[j])
c[k++]=a[i++];
else
c[k++]=a[j++];
}
for
(k=low;k<high;k++)
a[k]=c[k];
}
}
public static void
main(String[] args){
int
N =
9
;
int
i;
int
[] a={
3
,
2
,
5
,
8
,
4
,
7
,
6
,
9
,
1
};
MergeSort
(a,
0
,N);
for
(i=
0
;i<N;i++)
System.
out
.print(
" "
+a[i]);
}
}
----------------------------------------------------------------------------------------------------------------------
八、基数排序
这里要说明一下的是基数排序,负数和正数应该分开处理。这里我的代码优化的不是很好,后面会持续更新!
/**
* 基数排序
*
@param
//array 待排序数组
*
@param
//d 表示最大的元素的位数
* */
public class
Radix_Sort_08 {
public static void
RadixSort(
int
[] array,
int
d){
int
n =
1
;
int
times =
1
;
// 排序次数,由位数最多的元素决定
int
[][] temp =
new int
[
10
][array.
length
];
//数组的第一维表示可能的余数0-9
int
[] order =
new int
[
10
];
//数组order用来表示该位是i的元素个数
while
(times <= d) {
for
(
int
i =
0
; i < array.
length
; i++) {
int
lsd = ((array[i] / n) %
10
);
temp[lsd][order[lsd]] = array[i];
order[lsd]++;
}
int
k =
0
;
for
(
int
i =
0
; i <
10
; i++) {
if
(order[i] !=
0
) {
for
(
int
j =
0
; j < order[i]; j++) {
array[k] = temp[i][j];
k++;
}
order[i] =
0
;
}
}
n *=
10
;
times++;
}
}
public static int
Maximum(
int
[] array){
//计算出数组中最大元素的位数
int
max = array[
0
];
for
(
int
i=
0
; i<array.
length
; i++){
if
( array[i]>max )
max = array[i];
}
int
digit =
0
;
while
( max>
0
){
max/=
10
;
digit++;
}
return
digit;
}
public static void
Group(
int
[] array){
int
negative_num =
0
;
for
(
int
i=
0
; i<array.
length
; i++){
if
( array[i]<
0
)
negative_num++;
}
int
[] negative =
new int
[negative_num] ;
int
[] positive =
new int
[array.
length
-negative_num];
int
n =
0
, p =
0
;
for
(
int
i=
0
; i<array.
length
; i++){
if
( array[i]<
0
)
negative[n++] = array[i]*(-
1
);
else
positive[p++] = array[i];
}
if
(negative.
length
>
0
){
RadixSort
( negative,
Maximum
(negative));
for
(
int
i = negative.
length
-
1
; i>-
1
; i--){
System.
out
.print(negative[i]*(-
1
)+
" "
);
}
}
if
(positive.
length
>
0
){
RadixSort
( positive,
Maximum
(positive));
for
(
int
i:positive){
System.
out
.print(i+
" "
);
}
}
}
public static void
main(String[] args) {
int
[] array = {-
3
,-
2
,
5
,-
8
,-
0
,
0
,
6
,
9
};
Group
(array);
}
}