#include <iostream>
#include <cstring>
using namespace std;
class Person
{
int age;
string &name;
public:
//构造函数
Person(int age,string &name):name(name)
{
cout<<"P的构造函数"<<endl;
this->age = age;
}
//析构函数
~Person()
{
cout<<"P的析构函数"<<endl;
}
//拷贝构造函数
Person (const Person &other):age(other.age),name(other.name)
{
cout<<"P的拷贝构造函数"<<endl;
}
//拷贝赋值函数
Person &operator = (const Person &other)
{
this->age = other.age;
this->name = other.name;
cout<<"P的拷贝赋值函数"<<endl;
return *this;
}
//算数运算符
Person operator+(const Person &other);
Person operator-(const Person &other);
Person operator*(const Person &other);
Person operator/(const Person &other);
Person operator%(const Person &other);
friend Person operator+(Person &p1,Person &p2);
friend Person operator-(Person &p1,Person &p2);
friend Person operator*(Person &p1,Person &p2);
friend Person operator/(Person &p1,Person &p2);
friend Person operator%(Person &p1,Person &p2);
/*------------------------------------*/
//条件运算符
bool operator>=(const Person &other);
bool operator<=(const Person &other);
bool operator==(const Person &other);
//逻辑
bool operator&&(const Person &other);
//自增/自减
Person operator--(int);//成员函数后--
friend Person operator++(Person &p);//全局前++
//插入/输出
friend ostream &operator<<(ostream &out,Person &p1);
friend istream &operator>>(istream &out,Person &p1);
void show();
};
void Person::show()
{
cout<<age<<endl;
}
/*--------------------类外定义------------------*/
Person Person::operator+(const Person &other)
{
string s = "a";
Person temp(1,s);
temp.age = this->age+other.age;
return temp;
}
Person Person::operator-(const Person &other)
{
string s = "a";
Person temp(1,s);
temp.age = this->age-other.age;
return temp;
}
Person Person::operator*(const Person &other)
{
string s = "a";
Person temp(1,s);
temp.age = this->age*other.age;
return temp;
}
Person Person::operator/(const Person &other)
{
string s = "a";
Person temp(1,s);
temp.age = this->age/other.age;
return temp;
}
Person Person::operator%(const Person &other)
{
string s = "a";
Person temp(1,s);
temp.age = this->age%other.age;
return temp;
}
bool Person::operator>=(const Person &other)
{
if(this->age >= other.age)
{
return this->age >= other.age;
}else
{
return this->age >= other.age;
}
}
bool Person::operator<=(const Person &other)
{
if(this->age <= other.age)
{
return this->age <= other.age;
}else
{
return this->age <= other.age;
}
}
bool Person::operator==(const Person &other)
{
return this->age==other.age;;
}
//逻辑
bool Person::operator&&(const Person &other)
{
return (this->age) && other.age;
}
//全局 前自增
Person operator++(Person &p)
{
++p.age;
return p;
}
//成员函数后自减
Person Person::operator--(int)
{
string s = "a";
Person temp(1,s);
temp.age = this->age--;
return temp;
}
//
ostream &operator<<(ostream &out,Person &p1)
{
out<<"age="<<p1.age;
return out;
}
istream &operator>>(istream &in,Person &p1)
{
in>>p1.age;
return in;
}
/*----------------全局运算符--------------------*/
Person operator+(Person &p1,Person &p2)
{
string s = "a";
Person temp(1,s);
temp.age = p1.age+p2.age;
return temp;
}
Person operator-(Person &p1,Person &p2)
{
string s = "a";
Person temp(1,s);
temp.age = p1.age-p2.age;
return temp;
}
Person operator*(Person &p1,Person &p2)
{
string s = "a";
Person temp(1,s);
temp.age = p1.age*p2.age;
return temp;
}
Person operator/(Person &p1,Person &p2)
{
string s = "a";
Person temp(1,s);
temp.age = p1.age/p2.age;
return temp;
}
Person operator%(Person &p1,Person &p2)
{
string s = "a";
Person temp(1,s);
temp.age = p1.age%p2.age;
return temp;
}
/*--------------------------------------------------------------*/
class Stu
{
double *score;
public:
//构造函数
Stu (double score):score(new double(score))
{
cout<<"Stu的构造函数"<<endl;
}
//析构函数
~Stu ()
{
delete score;
cout<<"S的析构函数"<<endl;
}
//拷贝构造函数
Stu (const Stu &other):score(other.score)
{
cout<<"S的构造函数"<<endl;
}
//拷贝赋值
Stu &operator =(const Stu &other)
{
*(this->score)=*(other.score);
cout<<"S的拷贝赋值"<<endl;
return *this;
}
void show();
};
void Stu::show()
{
cout<<*score<<endl;
}
int main()
{
string cc="lls";
string dd="sll";
Person p1(18,cc);
Person p2(12,dd);
cout<<p1<<"" <<p2<<"lllll"<<endl;
cin>>p1>>p2;
p1.show();
p2.show();
// Person p3 = operator*(p1,p2);
// p3 = p1.operator*(p2);
// cout<<p1.operator&&(p2)<<endl;
// Person p3(1,dd);
// p3 = p1.operator--(3);
// p3 = operator++(p1);
// p1.show();
// p3.show();
// p1.show();
// cout<<"---------------"<<endl;
// Person p2=p1;
// p2.show();
// cout<<"---------------"<<endl;
// Person p3(17,dd);
// p3.show();
// p3 = p2;
// p3.show();
// Stu s1(98.9);
// s1.show();
// cout<<"---------------"<<endl;
// Stu s2(s1);
// s2.show();
// cout<<"---------------"<<endl;
// Stu s3(60);
// s3.show();
// s3 = s2;
// s3.show();
return 0;
}
12-29
于 2024-01-02 09:01:56 首次发布
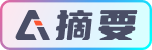