01-购物车案例
1.1 代码实战
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>01-购物车案例</title>
<style>
table {
border: 1px solid #e9e9e9;
border-collapse: collapse;
border-spacing: 0;
}
th,td {
padding: 8px 16px;
border: 1px solid #e9e9e9;
text-align: left;
}
th {
background-color:#f7f7f7;
color: #5c6b77;
font-weight: 600;
}
</style>
</head>
<body>
<div id="app">
<div v-if="books.length">
<table>
<thead>
<tr>
<th></th>
<th>书籍名称</th>
<th>出版日期</th>
<th>价格</th>
<th>购买数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(book,index) in books">
<td>{{book.id}}</td>
<td>{{book.name}}</td>
<td>{{book.date}}</td>
<td>{{book.price | showPrice}}</td>
<td>
<button @click="decrement(index)" :disabled="book.count<=1">-</button>
{{book.count}}
<button @click="increment(index)">+</button>
</td>
<td>
<button @click="removeHandle(index)">移除</button>
</td>
</tr>
</tbody>
</table>
<h2>总价格:{{totalPrice | showPrice}}</h2>
</div>
<h1 v-else>购物车为空</h1>
</div>
<script type="text/javascript" src="../js/vue.js"></script>
<script type="text/javascript">
const vm = new Vue({
el: '#app',
data: {
message: 'hello world!',
books:[
{
id:1,
name:'《算法导论》',
date:'2006-9',
price:85.00,
count:1,
},
{
id:2,
name:'《Unix编程艺术》',
date:'2006-2',
price:59.00,
count:1,
},
{
id:3,
name:'《编程珠玑》',
date:'2008-10',
price:39.00,
count:1,
},
{
id:4,
name:'《代码大全》',
date:'2006-3',
price:128.00,
count:1,
},
]
},
methods: {
decrement(index){
this.books[index].count--;
},
increment(index){
this.books[index].count++;
},
removeHandle(index){
this.books.splice(index,1);
}
},
filters:{
showPrice(price){
return '¥' + price.toFixed(2);
}
},
computed: {
totalPrice(){
let totalPrice = 0;
for (let i = 0; i < this.books.length; i++) {
totalPrice += this.books[i].price * this.books[i].count;
}
return totalPrice;
}
}
})
</script>
</body>
</html>
运行结果
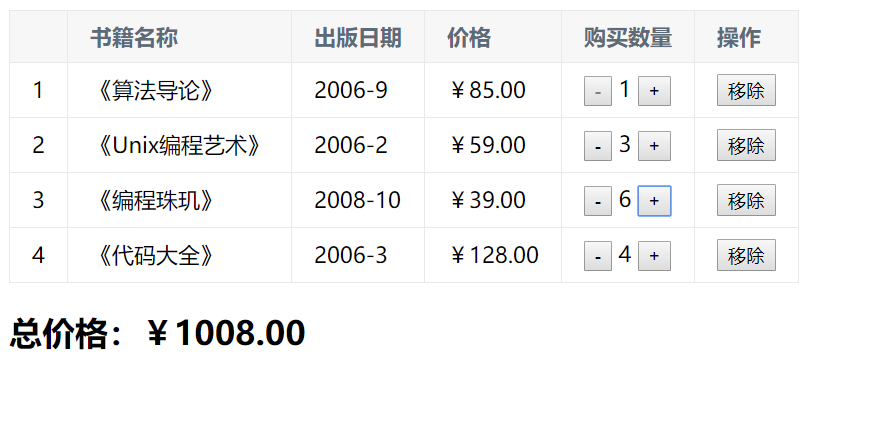
02-JS高阶函数——filter/map/reduce详解
2.1 代码实战
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>02-js高阶函数的使用</title>
</head>
<body>
<div id="app">
{{"原来的数组:"+ nums}}<br>
{{'过滤掉小于100之后的新数组:' + useFilter()}}<br>
{{'将过滤之后的数组,乘以2后的数组:' + useMap()}}<br>
{{'乘以2后的数组各元素的和:'+ useReduce()}}<br>
{{'三种函数,联用:' + useOnceCount()}}<br>
{{'一行代码搞定,以上三种需求:' + useOnceRowCount()}}<br>
</div>
<script type="text/javascript" src="../js/vue.js"></script>
<script type="text/javascript">
const vm = new Vue({
el: '#app',
data: {
nums: [10, 20, 111, 222, 333, 444, 40, 50]
},
methods: {
useFilter(){
let newNums = this.nums.filter(function (n) {
return n < 100;
})
return newNums;
},
useMap(){
let newNums2 = this.useFilter().map(function (n) {
return n * 2;
})
return newNums2;
},
useReduce(){
let total = this.useMap().reduce(function (preValue,n) {
return preValue+n
},0)
return total;
},
useOnceCount(){
let total = this.nums.filter(function (n) {
return n < 100
}).map(function (n) {
return n * 2
}).reduce(function (preValue,n) {
return preValue + n
},0)
return total
},
useOnceRowCount(){
let total = this.nums.filter(n => n < 100).map(n => n * 2).reduce(((previousValue,n) => previousValue + n),0);
return total;
}
}
})
</script>
</body>
</html>
2.2 运行结果
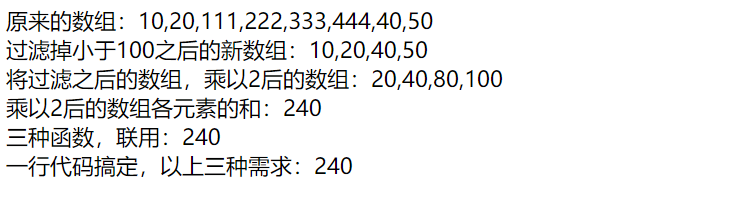