1.实现思路
- 创建一个xx.txt文件,存放于项目路径下
- 用文件流去读取文件内容并将读取的内容存放到页面的富文本编辑器框内
- 富文本编辑框内容改变后,保存时用文件流的方式保存到xx.txt文件中
提示:注意编码问题,否则容易出现中文乱码
2.页面展示
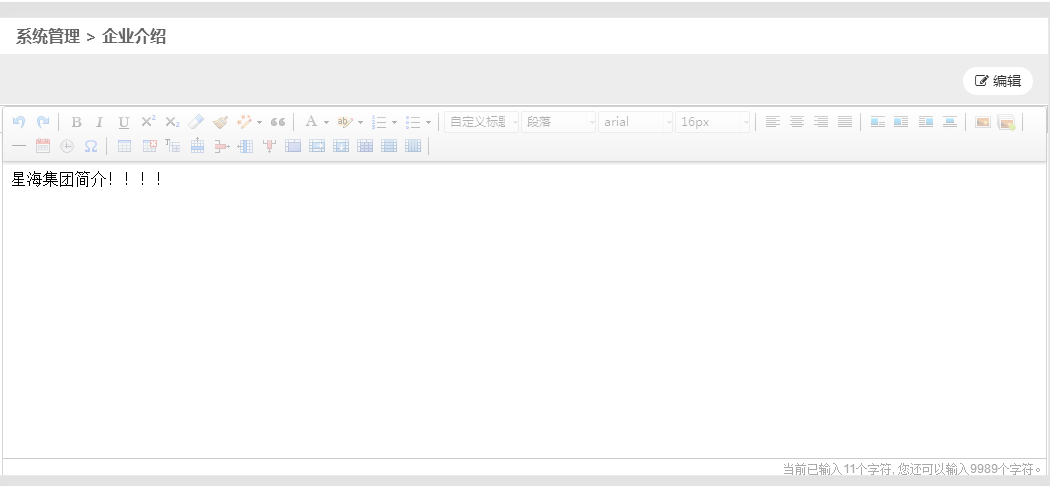
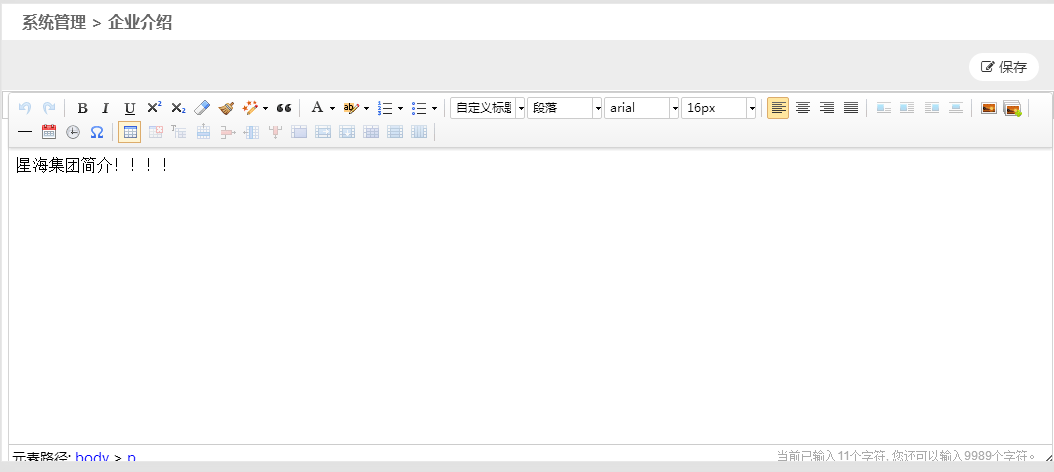
编辑器默认为禁止编辑状态,点击编辑按钮时可编辑内容,编辑完成后,点击保存按钮即可完成。
3.前端代码
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" charset="UTF-8" />
<title>企业介绍</title>
<!-- 引入公共资源 -->
<script th:replace="comm/comm::head"></script>
<link th:href="@{/boot/styles/learun-child.css}" rel="stylesheet" />
<script th:src="@{/boot/scripts/plugins/datepicker/DatePicker.js}"></script>
<script type="text/javascript"
th:src="@{/boot/scripts/plugins/UEditor/ueditor.config.js}"></script>
<script type="text/javascript"
th:src="@{/boot/scripts/plugins/UEditor/ueditor.all.js}"></script>
<script type="text/javascript"
th:src="@{/boot/scripts/plugins/UEditor/lang/zh-cn/zh-cn.js}"></script>
<link th:href="@{/boot/scripts/plugins/jquery-ui/bootstrap-select.css}"
rel="stylesheet" />
<script th:src="@{/boot/scripts/plugins/jquery-ui/bootstrap-select.js}"></script>
<style>
body {
height: 100%;
width: 100%;
margin-left: 20px;
}
</style>
</head>
<body>
<div class="ui-layout" id="vpapp" style="height: 100%; width: 100%;">
<div class="ui-layout-center">
<div id="div_right" class="ul_d_right"
style="width: 98%; overflow: hidden;">
<div class="show_tilte">系统管理 > 企业介绍</div>
<div class="toolsbutton">
<div class="childtitlepanel">
<div class="title-search">
<table>
<tr>
<td style="padding-left: 5px;">
<!-- <a id="btn_Search" class="btn btn-danger" v-on:click="serach" style="display:inline-block;"><i class="fa fa-search"></i> 查询</a> -->
</td>
</tr>
</table>
</div>
<div class="toolbar" id="edit">
<a id="item-edit" class="btn btn-default" onclick="item_edit()"><i
class="fa fa-pencil-square-o"></i> 编辑</a>
</div>
<div class="toolbar" id="save" style="display:none;">
<a id="item-save" class="btn btn-default" onclick="item_save()" ><i
class="fa fa-pencil-square-o" ></i> 保存</a>
</div>
</div>
</div>
<div class="gridPanel">
<table id="gridTable">
<tr>
<td class="formValue" colspan="3" style="height: 370px; vertical-align: top;">
<textarea class="form-control" id="coursecontent" style="width: 100%; height: 80%; resize: none;" th:utext="${words?:''}"></textarea>
</td>
</tr>
</table>
<div id="gridPager"></div>
</div>
</div>
</div>
</div>
<script th:src="@{/js/company/company_index.js}"></script>
</body>
</html>
js代码:
var editor;
$(function(){
editor = UE.getEditor('coursecontent',{
readonly: true
});
// setTimeout(() => {
// editor.disable();
// }, 3000);
});
//编辑
function item_edit(){
$('#edit').attr("style","display:none;");
$("#save").attr("style","display:block;");
editor.enable();
}
//保存
function item_save(){
$.ajax({
url: basePath + "/company/company_save",
data:{
words: encodeURI(encodeURI(editor.getContent()))
},
type: 'post',
dataType: 'json',
success(result){
if(result.code == '1') {
dialogMsg('保存成功!', 0);
$('#edit').attr("style","display:block;");
$("#save").attr("style","display:none;");
editor.disable();
}else{
dialogMsg('保存失败!', 0);
}
}
});
}
4.后台代码
package io.renren.modules.company;
import java.io.*;
import java.net.URLDecoder;
import org.apache.commons.lang3.StringUtils;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import io.renren.common.utils.JsonResult;
@Controller
@RequestMapping("/company")
public class CompanyController {
private static String path = CompanyController.class.getClassLoader().getResource("").getPath();
@RequestMapping("/list")
public String list(Model model) {
String string = readWord();
if(StringUtils.isNotEmpty(string)) {
model.addAttribute("words", string);
}
return "company/company_index";
}
/**
* 读取文件内容
*/
public static String readWord () {
try (
FileInputStream fileInputStream = new FileInputStream(URLDecoder.decode(path, "UTF-8")+"static\\company\\企业介绍.txt");
InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream,"UTF-8");
BufferedReader br = new BufferedReader(inputStreamReader);
){
String line = null;
StringBuffer sBuffer = new StringBuffer();
while((line = br.readLine())!=null){
sBuffer.append(line);
}
return sBuffer.toString();
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException(e);
}
}
/**
* 书写文件内容
*/
public static void writeWord(String str) throws IOException {
try (
FileOutputStream fileOutputStream = new FileOutputStream(URLDecoder.decode(path, "UTF-8")+"static\\company\\企业介绍.txt");
OutputStreamWriter outputStreamWriter = new OutputStreamWriter(fileOutputStream,"UTF-8");
PrintWriter out = new PrintWriter(outputStreamWriter);
){
out.write(str);
out.flush();
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException(e);
}
}
/**
* 保存
*/
@RequestMapping("/company_save")
@ResponseBody
public JsonResult companySave(String words) {
try {
String content = URLDecoder.decode(URLDecoder.decode(words, "UTF-8"), "UTF-8");
writeWord(content);
return JsonResult.success();
} catch (IOException e) {
e.printStackTrace();
return JsonResult.error();
}
}
}