IO流之字节输入输出流 字符输入输出流 转换流
字节流
字节输入输出流之文件的复制
字节输入流:
FileInputStream,是InputStream的子类;FileInputStrem从某个文件获取输入字节
字节输出流:
FileOutputStream,是OutputStream的子类;将数据写入File的字节流
字节不但可以写文本 还可以写图片 音频 视频等等
代码示例:文件复制
以字节方法读取写入
package com.lanou3g.p03;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class p05 {
public static void main(String[] args) {
copyDir(new File("/Users/lanou/Desktop/test/znb.txt"), new File("/Users/lanou/Desktop/test/utf.txt"));
}
public static void copyDir(File file1, File file2) {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
fis = new FileInputStream(file1);
fos = new FileOutputStream(file2);
int len = 0;
while((len = fis.read()) != -1){
fos.write(len);
}
} catch (FileNotFoundException e) {
throw new RuntimeException("文件连接失败");
} catch (IOException e) {
throw new RuntimeException("文件读取失败");
}finally {
try {
if(fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
if(fos != null) {
fos.close();
}
} catch (Exception e2) {
}
}
}
}
}
以字节数组方法读取写入
package com.lanou3g.p03;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class p05 {
public static void main(String[] args) {
copyDir(new File("/Users/lanou/Desktop/test/znb.txt"), new File("/Users/lanou/Desktop/test/utf8.txt"));
}
public static void copyDir(File file1, File file2) {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
fis = new FileInputStream(file1);
fos = new FileOutputStream(file2);
int len = 0;
byte[] c = new byte[1024];
while((len = fis.read(c)) != -1){
fos.write(c);
}
} catch (FileNotFoundException e) {
throw new RuntimeException("文件连接失败");
} catch (IOException e) {
throw new RuntimeException("文件读取失败");
}finally {
try {
if(fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
if(fos != null) {
fos.close();
}
} catch (Exception e2) {
}
}
}
}
}
IO部分类之间的关系
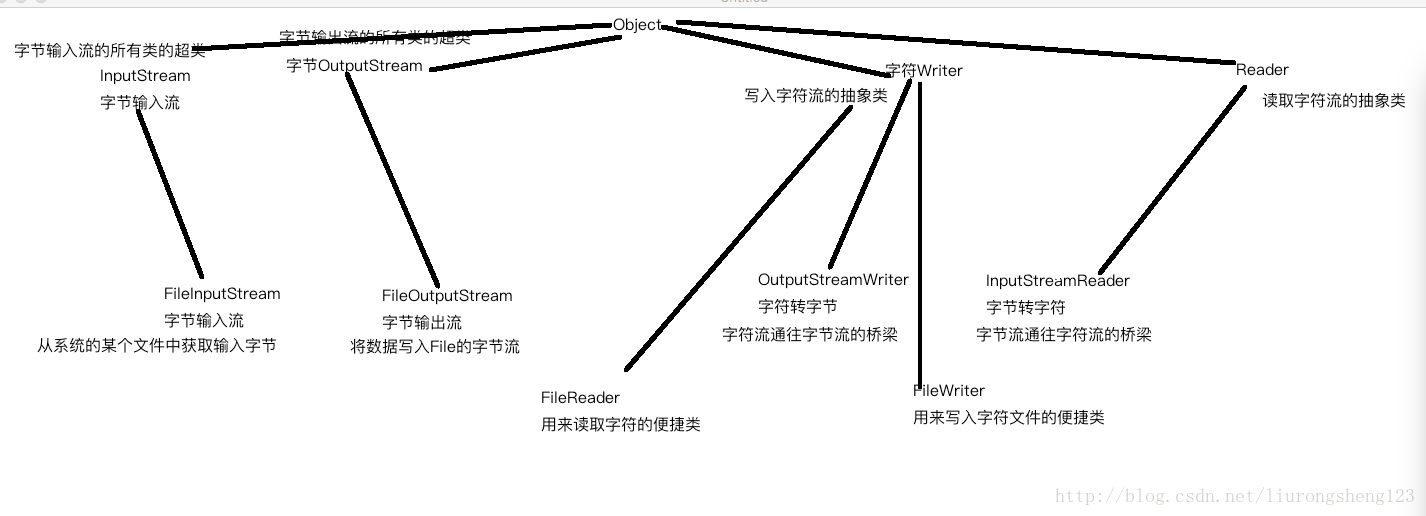
将一个文件夹 复制 到另一个文件夹下
public static void copyFileDirectory(File file1, File file2) {
FileInputStream dis= null;
FileOutputStream fos = null;
File file = new File(file2,file1.getName());
file.mkdir();
File[] listFiles = file1.listFiles();
for (File subFile : listFiles) {
if(subFile.isFile()) {
try {
dis = new FileInputStream(subFile);
File newFile = new File(file,subFile.getName());
fos = new FileOutputStream(newFile);
int len = 0;
while((len = dis.read()) != -1) {
fos.write(len);
}
dis.close();
fos.close();
} catch (FileNotFoundException e) {
throw new RuntimeException("文件连接失败");
} catch (IOException e) {
throw new RuntimeException("文件读取失败");
}
}else {
copyFileDirectory(subFile, file2);
}
}
}
将一个文件夹下的所有txt文件 复制到目标文件夹下
public static void copyTxtFile(File file1,File file2) {
File[] listFiles = file1.listFiles(new MyFileFilteTxt());
FileInputStream fis = null;
FileOutputStream fos = null;
for (File file : listFiles) {
if(file.isFile()) {
try {
fis = new FileInputStream(file);
File newFile = new File(file2,file.getName());
fos = new FileOutputStream(newFile);
int length = 0;
byte[] c = new byte[1024];
while((length = fis.read(c))!= -1) {
fos.write(c,0,length);
}
} catch (FileNotFoundException e) {
throw new RuntimeException("文件连接失败");
} catch (IOException e) {
throw new RuntimeException("文件读取失败");
}finally {
try {
fis.close();
} catch (IOException e) {
throw new RuntimeException("fis关闭失败");
}finally {
try {
fos.close();
} catch (IOException e) {
throw new RuntimeException("fos关闭失败");
}
}
}
}
}
}
文件过滤器
class MyFilterByTxt implements FileFilter{
@Override
public boolean accept(File pathname) {
if(pathname.isDirectory()) {
return true;
}
return pathname.getName().endsWith("txt");
}
}
字符流
字符流:一个字符一个字符地读
注意:只能用来操作文本(不能写图片 音频 视频)
Writer(所有字符输出流的父类 抽象类)
FileWriter
构造方法(绑定写入的路径)
文件
字符串
mac系统下 3个字节 默认使用UTF-8的编码表
Windows 系统下 一个字符占两个字节
默认使用GBK编码表(简体中文)
注意:字符输出流 在写入文件的时候
需要调用刷新方法
建议:每次写入 最好都刷新一次
使用close()方法的时候 会默认刷新
字符输出流-代码示例
FileWiter fw = new FileWriter("/Users/lanou/Desktop/test/haha.txt")
fr.write(100)
fw.flash()
char[] c = {'a','b','c','d'}
fw.write(c,1,2)
fw.flush()
fw.write("窗前明月光\n")
fw.flush()
fw.close()
字符输入流
字符输入流
Reader(所有字符输入流的父类)
FileReader
写的时候可以直接写入字符串
读的时候不能 因为 字符串很难界定到哪里结束 不太容易判断一个字符串
代码示例
public static void fun(){
FileReader fr = new FileReader("/Users/lanou/Desktop/test/haha.txt");
int len = 0;
while((len = fr.read()) != -1){
System.out.println((char)len);
}
}
public static void fun(){
FileReader fr = new FileReader("/Users/lanou/Desktop/test/haha.txt");
int len = 0;
char[] a = new char[1024];
while((len = fr.read(a)) != -1){
System.out.println(new String(a,0,len));
}
}
字符流复制文件
public class Demo06 {
public static void main(String[] args) throws IOException {
fun(new File("/Users/lanou/Desktop/test/haha.txt"), new File("/Users/lanou/Desktop/XTest/znb.txt"));
}
public static void fun(File file1,File file2) throws IOException {
FileReader fReader = new FileReader(file1);
FileWriter fileWriter = new FileWriter(file2);
char[] ch = new char[1024];
int length;
while((length = fReader.read(ch)) != -1) {
fileWriter.write(ch);
fileWriter.flush();
}
fileWriter.close();
fReader.close();
}
}
转换流
OutputStreamWriter(字符流转向字节流)
作用不同编码格式写入
需要使用到FileOutpuStream类
OutputStream 字节流父类
Writer 字符流父类
InputStreamReader
作用:可以读取不同编码格式的文件
需要使用到FileInputStream
图形解释
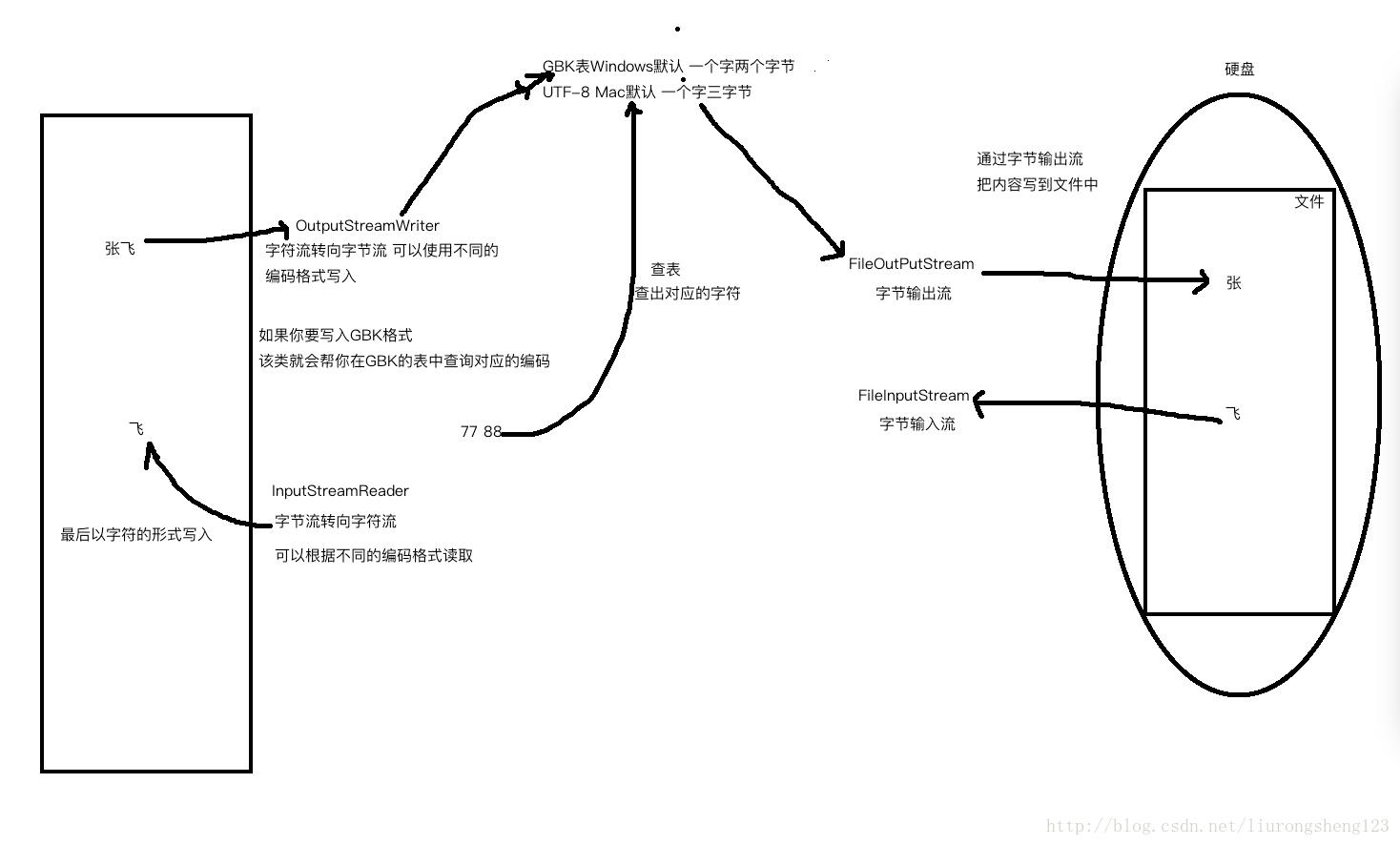
public class Demo07 {
public static void main(String[] args) throws IOException {
readGBK();
}
public static void readUTF8() throws IOException {
FileInputStream fls = new FileInputStream("/Users/lanou/Desktop/test/utf8.txt");
InputStreamReader isr = new InputStreamReader(fls);
char[] c = new char[1024];
int len;
while((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
public static void readGBK() throws IOException {
FileInputStream fis = new FileInputStream("/Users/lanou/Desktop/test/gbk.txt");
InputStreamReader isr = new InputStreamReader(fis, "GBK");
char[] c = new char[1024];
int len;
while((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
public static void getUTF8() throws IOException{
FileOutputStream fos = new FileOutputStream("/Users/lanou/Desktop/test/utf8.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos);
osw.write("窗前");
osw.close();
}
public static void getGBK() throws IOException {
FileOutputStream fos = new FileOutputStream("/Users/lanou/Desktop/test/gbk.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, "GBK");
osw.write("明月");
osw.close();
}
}
/*
* "Success is the constant experience of failure and always keeping the initial enthusiasm"
把上列字符串以下列形式写入文件
Success=1
is=1
the=2
.....
*/
public class Demo08 {
public static void main(String[] args) throws IOException {
String string = "Success is the constant experience of failure and always keeping the initial enthusiasm";
HashMap< String, Integer> hashMap = new HashMap<>();
String[] strings = string.split(" ");
FileOutputStream fos = new FileOutputStream("/Users/lanou/Desktop/test/znb.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos);
for(int i = 0; i < strings.length; i++) {
if(hashMap.containsKey(strings[i])) {
System.out.println("++++++");
Integer i1 = hashMap.get(strings[i]);
i1++;
hashMap.put(strings[i],i1);
}else {
System.out.println("-------");
hashMap.put(strings[i], 1);
}
}
for (String string2 : hashMap.keySet()) {
String string3 = string2 + "=" + hashMap.get(string2) + "\n";
osw.write(string3);
osw.flush();
}
osw.close();
}
}
将一个文件夹下的所有txt文件 复制 到另一个文件夹下 并且把txt改成java
public class Demo09 {
public static void main(String[] args) throws IOException {
File file1 = new File("/Users/lanou/Desktop/test");
File file2 = new File("/Users/lanou/Desktop/test3");
copyDirToAnotherFile(file1, file2);
}
public static void copyDirToAnotherFile(File src,File dest) throws IOException {
File[] files = src.listFiles(new MyFileFilter());
for (File subFile : files) {
if(subFile.isFile()) {
String name = subFile.getName();
int lastIndexOf = name.lastIndexOf(".");
String substring = name.substring(0, lastIndexOf);
String string = substring + ".java";
File file = new File(dest,string);
file.createNewFile();
}else {
copyDirToAnotherFile(subFile, dest);
}
}
}
}
class MyFileFilter implements FileFilter{
@Override
public boolean accept(File pathname) {
if(pathname.isDirectory()) {
return true;
}
return pathname.getName().endsWith("txt");
}
}