AutoHotkey Style Guide() {
A mostly reasonable approach to AutoHotkey
为了达到代码自解释的目标,任何自定义编程元素在命名时,使用尽量完整的单词组合来表达。
采用 4 个空格缩进,禁止使用 tab 字符。 说明:如果使用 tab 缩进,必须设置 1 个 tab为 4 个空格。
变量与常量名Variables:
所有变量用驼峰命名或蛇形命名
All variables should be camelCase or snake_case
常量命名全部大写,单词间用下划线隔开,力求语义表达完整清楚,不要嫌名字长。
Constants should be in ALLCAPS
在常量与变量的命名时,表示类型的名词放在词尾,以提升辨识度。workQueue / nameList / TERMINATED_THREAD_COUNT
不建议任何魔法值(即未经预先定义的常量)直接出现在代码中。
对枚举类型(enum)中的变量,要求用枚举变量或其缩写做前缀。并且要求用大写
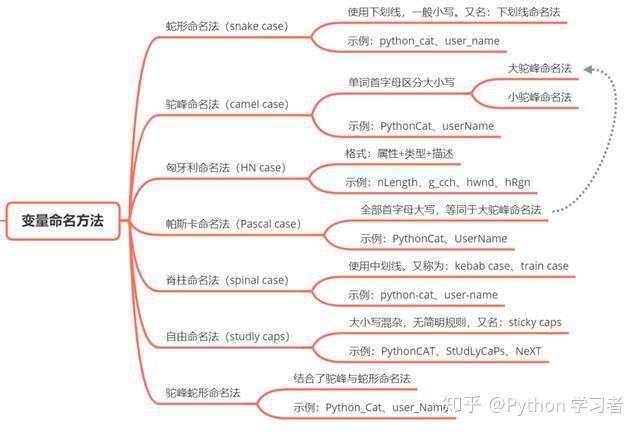
函数与方法名Functions and Methods:
方法名、参数名、成员变量、局部变量都统一使用驼峰式
Should be camelCase
IF语句 if statements:
Should be on own line
Should be proceeded by a space
Should be mostly enclosed in parenthesis:
if (var) if (func()) if (!var) if (!func()) if (var == 1) if (var != 1) if (var == 1 || var == 2) if !(var == 1 || var == 2)
括号Braces:
尽可能的使用花括号Should be used whenever possible.
1) 左大括号后换行。
2) 右大括号前换行
3) 右大括号后还有 else 等代码则可不换行;表示终止的右大括号后必须换行。
if (i > 0){
...
} else {
...
}
No-brace if statements are allowed, as long as the content is actually one line long:
; Good!
if (condition)
MsgBox, yes
; Bad!
if (condition1)
if (condition2)
MsgBox, no
左小括号和右边相邻字符之间不出现空格;右小括号和左边相邻字符之间也不出现空格
反例:if (空格 a == b 空格)
类Classes:
静态(类)变量:全部大写。
实例变量:内部私有的成员变量和成员函数建议用下划线开头;外部可访问的实例变量用驼峰命名或蛇形命名。
如果模块、接口、类、方法使用了设计模式,在命名时需体现出具体模式。 说明:将设计模式体现在名字中,有利于阅读者快速理解架构设计理念。class OrderFactory; class LoginProxy; class ResourceObserver;
建议参考下面的类模板should follow this template
class name {
; --- Static Variables ---
static CONSTANT := 1
; --- Instance Variables ---
external_use := 1
_internal_use := 1
; --- Properties ---
external[] {
get {
return this._internal_use
}
}
; --- Static Methods ---
method() {
return 1
}
; --- Constructor, Destructor, Meta-Functions ---
__New() {
}
__Delete() {
}
; --- Instance Methods ---
externalUseMethod() {
return 1
}
_internal_UseMethod() {
return 1
}
; --- Nested Classes ---
class nested1 {
}
class nested2 {
}
}
}
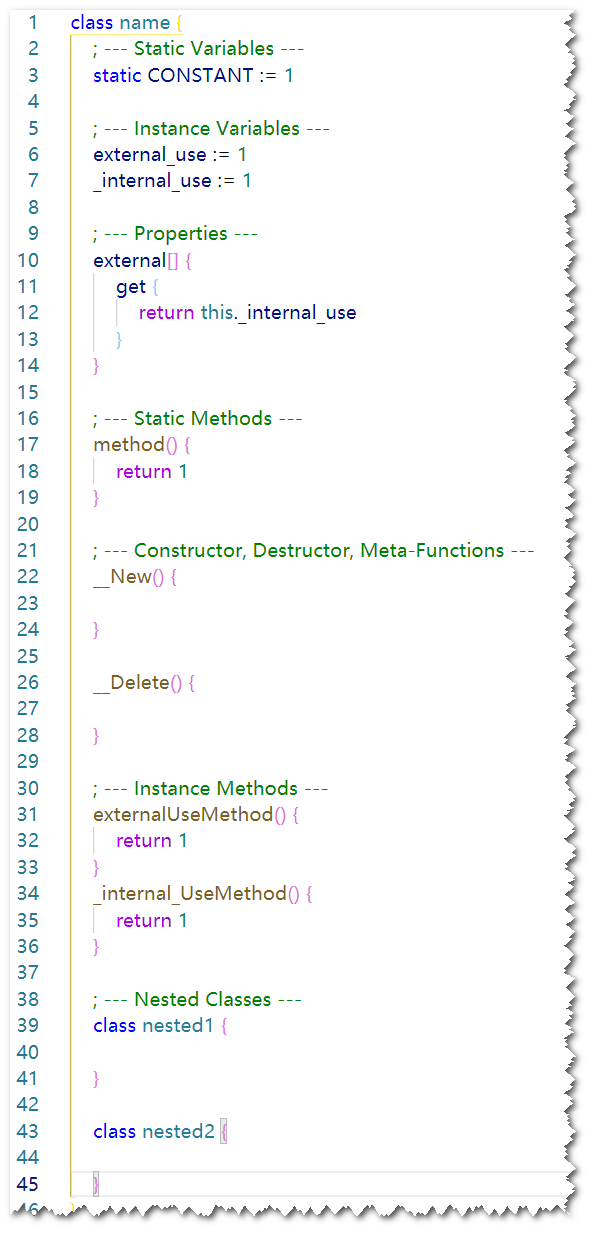