function Music() {
this.Audio = null;
this.GetAudio = function () {
if (this.Audio == null)
this.Audio = new Audio();
return this.Audio;
}
this.GetAudio();
this.CurrentMusic = { index: -1, url: '' }
this.CurrentIndex=-1;
this.MusicList = [this.CurrentMusic];
this.MusicList.length = 0;
this.LoopModel = { none: 0, single: 1, loop: 2, random: 3 }
this.Model = this.LoopModel.none;
}
function SetInterval(func, time) {
if (func()) return;
return setTimeout(function () {
SetInterval(func, time);
}, time);
}
Music.prototype.Play = function (index, callback) {
var Audio = this.Audio;
var $this=this;
this.CurrentIndex=index;
Audio.src = this.MusicList[index].url;
this.CurrentMusic = this.MusicList[index];
switch (this.Model) {
case this.LoopModel.none://默认播放一次
Audio.loop = false;
Audio.play();
if(callback){
callback($this.CurrentMusic)
}
break;
case this.LoopModel.single://单曲循环
Audio.loop = true;
Audio.play();
if(callback){
callback($this.CurrentMusic)
}
break;
case this.LoopModel.loop://列表循环
Audio.loop = false;
Audio.play();
if(callback){
callback($this.CurrentMusic)
}
var $length = $this.MusicList.length - 1;
SetInterval(function () {
if (Audio.ended) {
if ($this.CurrentIndex == $length)
$this.CurrentIndex = 0;
else
$this.CurrentIndex += 1;
$this.Play($this.CurrentIndex, callback);
return true;
}
},1000)
break;
case this.LoopModel.random://随机播放
Audio.loop = false;
Audio.play();
if(callback){
callback($this.CurrentMusic)
}
var $length = $this.MusicList.length - 1;
$this.CurrentIndex = Math.round(Math.random() * $length);
SetInterval(function () {
if (Audio.ended) {
$this.Play($this.CurrentIndex, callback);
return true;
}
}, 1000);
break;
}
}
Music.prototype.Pause = function (callback) {
this.Audio.pause();
if(callback){
callback(this.CurrentMusic)
}
}
Music.prototype.Next = function (callback) {
var l = this.CurrentMusic.index + 1;
this.Play(l,callback)
}
Music.prototype.Prev = function (callback) {
var l = this.CurrentMusic.index - 1;
this.Play(l,callback)
}
window.Music=new Music()
window.Music.Model = window.Music.LoopModel.loop;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>music~jay</title>
<script src="jquery-1.7.2.min.js" type="text/javascript"></script>
<style type="text/css">
body {
margin: 0;
padding: 0;
}
.container {
margin: auto auto auto auto;
height: 100%;
width: 375px;
}
.top {
line-height: 80px;
height: 80px;
width: 375px;
background-color: #ececec;
top: 0;
left: 0;
right: 0;
}
.top img {
margin-left: 10px;
margin-right: 10px;
border-radius: 50%;
width: 50px;
height: 50px;
vertical-align: middle;
}
.top span {
color: dimgray;
font-size: 18px;
}
.list {
height: 100%;
}
.list ul {
padding: 0;
margin: 0;
border: 1px solid #ececec;
height: 600px;
overflow: auto;
}
.list ul li {
list-style: none;
margin: 0;
padding: 0px 10px;
line-height: 40px;
height: 40px;
font-size: 12px;
display: flex;
justify-content: space-between;
}
.list ul li:hover {
cursor: pointer;
transition: .8s
}
.list ul li div {
display: inline-block;
}
.list ul li .bofang {
cursor: pointer;
}
.toopbar {
display: inline-block;
width: calc(100% - 110px);
vertical-align: middle;
}
.toopbar-container{
display: flex;
justify-content: flex-end;
}
.toopbar-container svg{
margin-right: 5px;
}
ul::-webkit-scrollbar {
width: 8px;
height: 1px;
}
ul::-webkit-scrollbar-thumb {
border-radius: 10px;
-webkit-box-shadow: inset 0 0 5px rgba(0,0,0,0.2);
background: #bfbfbf;
}
ul::-webkit-scrollbar-track {
-webkit-box-shadow: inset 0 0 5px rgba(0,0,0,0.2);
border-radius: 10px;
background: #dfdfdf;
}
.toopbar-container svg:hover{
cursor: pointer;
}
</style>
</head>
<body>
<div class="container">
<div class="top">
<img src="img/1.png" />
<span>jay</span>
<div class="toopbar">
<div class="toopbar-container">
<svg id="sys" t="1604458924569" class="icon" viewBox="0 0 1024 1024" version="1.1" xmlns="http://www.w3.org/2000/svg" p-id="905" width="32" height="32"><path d="M552.24 511.68l85.31 107.86 43.76 55.5V348.76l-43.76 55.5-85.31 107.42z m-201.25 0l85.29 107.86 43.76 55.5V348.76l-43.76 55.5-85.29 107.42z m35.63 147.12L285.99 532.2c-9.04-11.51-9.47-27.98 0-39.7L386.62 365l98.85-124.57c5.41-9.01 15.34-14.45 26.62-14.45 17.61 0 31.59 14.24 31.59 31.59v163.38L587.9 365l98.39-124.57c5.87-9.01 15.8-14.45 26.64-14.45 17.58 0 32.03 14.24 32.03 31.59V766.2c0 9.47-4.51 18.74-12.18 25.05-13.54 10.84-33.85 8.36-44.69-5.41L587.9 658.8l-44.23-55.52V766.2c0 9.47-4.04 18.74-12.18 25.05-13.54 10.84-33.62 8.36-44.69-5.41L386.62 658.8z" fill="#717071" p-id="906"></path></svg>
<svg id="zt" t="1604458969916" class="icon" viewBox="0 0 1024 1024" version="1.1" xmlns="http://www.w3.org/2000/svg" p-id="1050" width="32" height="32"><path d="M244.83 225.99h203.31c17.84 0 31.82 14.01 31.82 31.59v508.61c0 17.61-13.98 31.82-31.82 31.82H244.83c-17.58 0-31.59-14.21-31.59-31.82V257.58c0.01-17.58 14.01-31.59 31.59-31.59z m330.82 0h203.31c17.81 0 31.8 14.01 31.8 31.59v508.61c0 17.61-13.98 31.82-31.8 31.82H575.65c-17.61 0-32.05-14.21-32.05-31.82V257.58c0-17.58 14.44-31.59 32.05-31.59z m171.49 63.2H607.23v445.42h139.91V289.19z m-330.81 0H276.42v445.42h139.91V289.19z" fill="#717071" p-id="1051"></path></svg>
<svg id='play' style="display: none;" t="1604462184816" class="icon" viewBox="0 0 1024 1024" version="1.1" xmlns="http://www.w3.org/2000/svg" p-id="1632" width="32" height="32"><path d="M748.23 539.66L528 666.47 307.78 793.74c-14.9 8.78-34.52 3.6-43.32-11.74-2.93-4.97-4.07-10.37-4.07-15.8h-0.46V257.58c0-17.58 14.44-31.59 32.05-31.59 6.33 0 12.41 1.81 17.61 5.43L528 357.33 748.23 484.6c15.34 8.57 20.31 28.42 11.51 43.32-2.94 4.97-6.54 8.81-11.51 11.74z m-251.81 71.97l172.4-99.95-172.4-99.52-172.86-99.52v398.72l172.86-99.73z" fill="#717071" p-id="1633"></path></svg>
<svg id="xys" t="1604458990338" class="icon" viewBox="0 0 1024 1024" version="1.1" xmlns="http://www.w3.org/2000/svg" p-id="1195" width="32" height="32"><path d="M480.33 603.28l-43.99 55.52-100.43 127.04c-10.84 13.77-30.69 16.24-44.69 5.41-7.67-6.31-11.74-15.57-11.74-25.05h-0.44V257.58c0-17.35 14.44-31.59 32.05-31.59 11.04 0 20.98 5.43 26.62 14.45L436.33 365l43.99 55.96V257.58c0-17.35 14.21-31.59 31.82-31.59 11.51 0 21.21 5.43 26.85 14.45L637.82 365l100.42 127.5c9.24 11.71 8.8 28.19-0.46 39.7l-99.96 126.6-100.63 127.04c-10.84 13.77-30.92 16.24-44.69 5.41-7.9-6.31-11.74-15.57-11.74-25.05h-0.44V603.28h0.01z m107.63 16.26l85.08-107.86-85.08-107.42-43.99-55.5v326.28l43.99-55.5z m-201.28 0l85.08-107.86-85.08-107.42-43.76-55.5v326.28l43.76-55.5z" fill="#717071" p-id="1196"></path></svg>
</div>
</div>
</div>
<div class="list">
<ul class="musiclist"></ul>
</div>
</div>
</body>
</html>
<script type="text/template" id="list">
<li title="点击播放" data-url="@index">
<div>@name</div>
<div class="bofang"></div>
</li>
</script>
<script type="text/template" id="bf">
<svg t="1604460113980" class="icon" viewBox="0 0 1024 1024" version="1.1" xmlns="http://www.w3.org/2000/svg" p-id="1485" width="16" height="16"><path d="M325.06 359.52v304.61l1.57 1.59 213.22 163.36V194.57L325.06 359.52z m385.85-122.76c-15.13-8.8-19.87-28.42-11.07-43.56 9.27-14.88 28.67-19.85 43.56-10.81 56.86 33.85 104.49 82.58 136.97 140.35 31.82 55.96 50.33 120.5 50.33 189.1 0 68.58-18.51 133.12-50.33 189.08-32.49 57.77-80.11 106.52-136.97 140.58-14.88 9.26-34.29 4.3-43.56-11.04-8.8-14.9-4.07-34.75 11.07-43.32 47.83-28.44 87.09-69.06 114.63-117.36 26.18-46.47 41.52-100.17 41.52-157.93 0-57.33-15.34-111.49-41.52-157.75-27.54-48.27-66.8-89.12-114.63-117.34zM286.68 366.07H157.17V657.6h129.51V366.07z m8.36-63.64l255.65-196.08c5.87-4.74 13.1-7.47 20.98-7.47 17.84 0 31.82 14.01 31.82 31.61v210.75c29.78 12.18 55.06 31.59 74.47 56.4 24.82 31.59 39.72 71.08 39.72 114.19 0 43.09-14.9 82.81-39.72 114.17-18.95 24.82-44.69 44.23-74.47 56.4v210.75c0 7.23-2.24 13.77-6.54 19.64-10.84 13.77-30.69 16.47-44.46 5.64l-257.45-197.2H125.12c-17.58 0-31.82-14.21-31.82-31.59V334.25c0-17.61 14.23-31.82 31.82-31.82h169.92z m308.45 80.78v257.24c17.61-9.47 32.51-22.57 44.69-37.92 19.64-24.82 31.36-56.4 31.36-90.69 0-33.85-11.95-65.67-31.36-90.48-12.18-15.57-27.08-28.45-44.69-38.15z" fill="#717071" p-id="1486"></path></svg>
</script>
<script type="text/javascript" src="music.js"></script>
<script type="text/javascript">
(function() {
$.getJSON("http://172.18.11.138:5000/api/music/getlist", function(data) {
if (data && data.data) {
window.Music.MusicList = data.data
var musicdata = data.data
for (var i = 0; i < musicdata.length; ++i) {
var item = $($("#list").html().replace("@name", musicdata[i].name).replace("@index", musicdata[i].index))
$('.musiclist').append(item)
}
window.Music.Play(0, function(currentmusic) {
$(".musiclist li .bofang").each(function() {
$(this).html('')
})
$('.musiclist').children().eq(currentmusic.index).children().eq(1).html($('#bf').html())
})
}
})
$('body').on('click', ".musiclist li", function() {
var $this = $(this)
$(".musiclist li .bofang").each(function() {
$(this).html('')
})
window.Music.Play($this.attr('data-url'), function() {
$this.children().eq(1).html($('#bf').html())
})
})
$("#sys").click(function(){
window.Music.Prev(function(currentmusic) {
$(".musiclist li .bofang").each(function() {
$(this).html('')
})
var $this=$("[data-url|='"+currentmusic.index+"']")
$this.children().eq(1).html($('#bf').html())
})
})
$("#xys").click(function(){
window.Music.Next(function(currentmusic) {
$(".musiclist li .bofang").each(function() {
$(this).html('')
})
var $this=$("[data-url|='"+currentmusic.index+"']")
$this.children().eq(1).html($('#bf').html())
})
})
$("#zt").click(function(){
window.Music.Pause()
$(this).hide()
$("#play").show()
})
$("#play").click(function(){
$(this).hide()
$("#zt").show()
window.Music.Audio.play()
})
})()
</script>
<script type="text/javascript" charset="utf-8" src="https://files.cnblogs.com/files/liuzhou1/L2Dwidget.0.min.js"></script>
<script type="text/javascript" charset="utf-8" src="https://files.cnblogs.com/files/liuzhou1/L2Dwidget.min.js"></script>
<script type="text/javascript">
L2Dwidget.init({"display": {
"superSample":2,
"width": 200,
"height": 400,
"position": "right",
"hOffset": 100,
"vOffset": 100
}
});
</script>
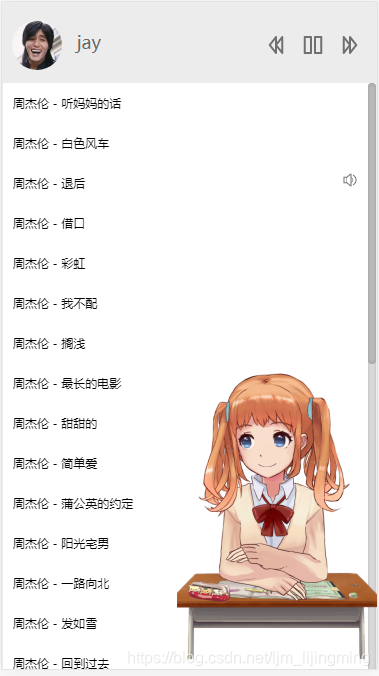
附件:HTML5音乐播放器配套后台服务器