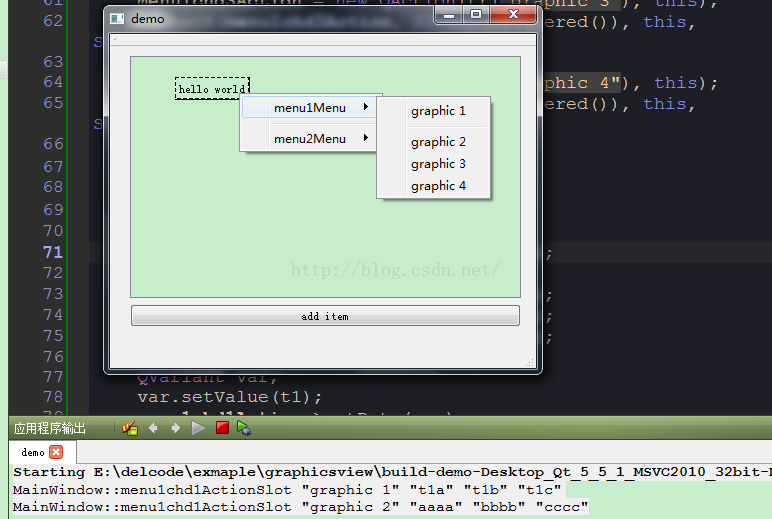
diagramtextitem.h:
/****************************************************************************
**
** Copyright (C) 2015 The Qt Company Ltd.
** Contact: http://www.qt.io/licensing/
**
** This file is part of the examples of the Qt Toolkit.
**
** $QT_BEGIN_LICENSE:BSD$
** You may use this file under the terms of the BSD license as follows:
**
** "Redistribution and use in source and binary forms, with or without
** modification, are permitted provided that the following conditions are
** met:
** * Redistributions of source code must retain the above copyright
** notice, this list of conditions and the following disclaimer.
** * Redistributions in binary form must reproduce the above copyright
** notice, this list of conditions and the following disclaimer in
** the documentation and/or other materials provided with the
** distribution.
** * Neither the name of The Qt Company Ltd nor the names of its
** contributors may be used to endorse or promote products derived
** from this software without specific prior written permission.
**
**
** THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
** "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
** LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
** A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
** OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
** SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
** LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
** DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
** THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
** (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
** OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE."
**
** $QT_END_LICENSE$
**
****************************************************************************/
#ifndef DIAGRAMTEXTITEM_H
#define DIAGRAMTEXTITEM_H
#include <QGraphicsTextItem>
#include <QPen>
QT_BEGIN_NAMESPACE
class QFocusEvent;
class QGraphicsItem;
class QGraphicsScene;
class QGraphicsSceneMouseEvent;
QT_END_NAMESPACE
struct testStruct
{
testStruct() : a("aaaa"), b("bbbb"), c("cccc") { }
~testStruct()
{
}
testStruct(const testStruct &other)
: a(other.a),
b(other.b),
c(other.c)
{ }
testStruct(const QString &aa,
const QString &bb,
const QString &cc)
: a(aa),
b(bb),
c(cc)
{
}
bool isNull() const { return (a.isEmpty())&&(b.isEmpty())&&(c.isEmpty()); }
bool operator== (const testStruct &other) const
{
return (a==other.a)&&(b==other.b)&&(c==other.c);
}
testStruct &operator =( const testStruct &other )
{
if (this == &other)
return *this;
a = other.a;
b = other.b;
c = other.c;
return *this;
}
QString a;
QString b;
QString c;
};
Q_DECLARE_METATYPE(testStruct)
class DiagramTextItem : public QGraphicsTextItem
{
Q_OBJECT
public:
enum { Type = UserType + 3 };
DiagramTextItem(QMenu *menu,QGraphicsItem *parent = 0);
int type() const Q_DECL_OVERRIDE { return Type; }
//signals:
// void lostFocus(DiagramTextItem *item);
// void selectedChange(QGraphicsItem *item);
protected:
//QVariant itemChange(GraphicsItemChange change, const QVariant &value) Q_DECL_OVERRIDE;
//void focusOutEvent(QFocusEvent *event) Q_DECL_OVERRIDE;
//void mouseDoubleClickEvent(QGraphicsSceneMouseEvent *event) Q_DECL_OVERRIDE;
void paint(QPainter * painter, const QStyleOptionGraphicsItem * option, QWidget * widget) Q_DECL_OVERRIDE;
void contextMenuEvent(QGraphicsSceneContextMenuEvent *event) Q_DECL_OVERRIDE;
private:
QMenu *myContextMenu;
};
//! [0]
#endif // DIAGRAMTEXTITEM_H
diagramtextitem.cpp:
/****************************************************************************
**
** Copyright (C) 2015 The Qt Company Ltd.
** Contact: http://www.qt.io/licensing/
**
** This file is part of the examples of the Qt Toolkit.
**
** $QT_BEGIN_LICENSE:BSD$
** You may use this file under the terms of the BSD license as follows:
**
** "Redistribution and use in source and binary forms, with or without
** modification, are permitted provided that the following conditions are
** met:
** * Redistributions of source code must retain the above copyright
** notice, this list of conditions and the following disclaimer.
** * Redistributions in binary form must reproduce the above copyright
** notice, this list of conditions and the following disclaimer in
** the documentation and/or other materials provided with the
** distribution.
** * Neither the name of The Qt Company Ltd nor the names of its
** contributors may be used to endorse or promote products derived
** from this software without specific prior written permission.
**
**
** THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
** "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
** LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
** A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
** OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
** SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
** LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
** DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
** THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
** (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
** OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE."
**
** $QT_END_LICENSE$
**
****************************************************************************/
#include "diagramtextitem.h"
#include <QPainter>
#include <QGraphicsScene>
#include <QMenu>
#include <QGraphicsSceneContextMenuEvent>
//#include "diagramscene.h"
//! [0]
DiagramTextItem::DiagramTextItem(QMenu *menu, QGraphicsItem *parent)
: QGraphicsTextItem(parent)
{
myContextMenu=menu;
setPlainText("hello world");
setFlag(QGraphicsItem::ItemIsMovable);
setFlag(QGraphicsItem::ItemIsSelectable);
}
//! [0]
//! [1]
//QVariant DiagramTextItem::itemChange(GraphicsItemChange change,
// const QVariant &value)
//{
// if (change == QGraphicsItem::ItemSelectedHasChanged)
// emit selectedChange(this);
// return value;
//}
//! [1]
//! [2]
//void DiagramTextItem::focusOutEvent(QFocusEvent *event)
//{
// setTextInteractionFlags(Qt::NoTextInteraction);
// emit lostFocus(this);
// QGraphicsTextItem::focusOutEvent(event);
//}
//! [2]
//! [5]
//void DiagramTextItem::mouseDoubleClickEvent(QGraphicsSceneMouseEvent *event)
//{
// if (textInteractionFlags() == Qt::NoTextInteraction)
// setTextInteractionFlags(Qt::TextEditorInteraction);
// QGraphicsTextItem::mouseDoubleClickEvent(event);
//}
void DiagramTextItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
// QBrush cbrush(myColor);
// painter->setBrush(cbrush);
QRectF boundRect = boundingRect();
painter->drawRect(boundRect);
QGraphicsTextItem::paint(painter, option, widget);
//QGraphicsItem::paint(painter,option,widget);
}
void DiagramTextItem::contextMenuEvent(QGraphicsSceneContextMenuEvent *event)
{
scene()->clearSelection();
setSelected(true);
myContextMenu->exec(event->screenPos());
}
//! [5]
mainwindow.h:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
//#include <DiagramScene>
#include <QGraphicsScene>
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
public slots:
void menu1chd1ActionSlot();
private:
Ui::MainWindow *ui;
QGraphicsScene *scene;
//DiagramScene *scene;
QGraphicsView *view;
QGraphicsTextItem *item;
QPolygonF myPolygon;
private slots:
void addItem();
private:
void createMenu();
private:
QAction *menu1Action;
QAction *itemColorAction_test;
QAction *menu2Action;
QAction *menu1chd1Action;
QAction *menu1chd2Action;
QAction *menu1chd3Action;
QAction *menu1chd4Action;
QMenu *AllItemMenu;
QMenu *menu1Menu;
QMenu *menu2Menu;
};
#endif // MAINWINDOW_H
mainwindow.cpp:
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QGraphicsItem>
#include <QGraphicsScene>
#include <QGraphicsTextItem>
#include <QHBoxLayout>
#include <QGraphicsView>
#include "diagramtextitem.h"
#include <QDebug>
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
myPolygon << QPointF(-100, -100) << QPointF(100, -100)
<< QPointF(100, 100) << QPointF(-100, 100)
<< QPointF(-100, -100);
ui->setupUi(this);
scene = new QGraphicsScene(this);
scene->setSceneRect(QRectF(0, 0, 300, 200));
createMenu();
view = new QGraphicsView(scene);
ui->horizontalLayout->addWidget(view);
setWindowTitle(tr("demo"));
setUnifiedTitleAndToolBarOnMac(true);
connect(ui->pushButton,SIGNAL(clicked(bool)),this,SLOT(addItem()));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::addItem()
{
QString itemText = "hello world";
item =new DiagramTextItem(AllItemMenu);
item->setPlainText(itemText);
//item->setPolygon(myPolygon);
item->setFlag(QGraphicsItem::ItemIsMovable, true);
item->setFlag(QGraphicsItem::ItemIsSelectable, true);
item->setFlag(QGraphicsItem::ItemSendsGeometryChanges, true);
scene->addItem(item);
}
void MainWindow::createMenu()
{
//! 4 个图形添加 b
menu1chd1Action = new QAction(tr("graphic 1"), this);
connect(menu1chd1Action, SIGNAL(triggered()), this, SLOT(menu1chd1ActionSlot()));
menu1chd2Action = new QAction(tr("graphic 2"), this);
connect(menu1chd2Action, SIGNAL(triggered()), this, SLOT(menu1chd1ActionSlot()));
menu1chd3Action = new QAction(tr("graphic 3"), this);
connect(menu1chd3Action, SIGNAL(triggered()), this, SLOT(menu1chd1ActionSlot()));
menu1chd4Action = new QAction(tr("graphic 4"), this);
connect(menu1chd4Action, SIGNAL(triggered()), this, SLOT(menu1chd1ActionSlot()));
//! 4 个图形添加 e
//! menu1Menu添加 b
menu1Menu = new QMenu(this);
menu1Menu->setTitle("menu1Menu");
menu1Menu->addAction(menu1chd1Action);
menu1Menu->addSeparator();
menu1Menu->addAction(menu1chd2Action);
menu1Menu->addAction(menu1chd3Action);
menu1Menu->addAction(menu1chd4Action);
testStruct t1("t1a","t1b","t1c");
QVariant var;
var.setValue(t1);
menu1chd1Action->setData(var);
//! menu1Menu添加 e
//! menu2Menu添加 b
menu2Menu = new QMenu(this);
menu2Menu->setTitle("menu2Menu");
menu2Menu->addAction(menu1chd1Action);
menu2Menu->addSeparator();
menu2Menu->addAction(menu1chd2Action);
menu2Menu->addAction(menu1chd3Action);
menu2Menu->addAction(menu1chd4Action);
//! menu2Menu添加 e
//! AllItemMenu 添加 b
AllItemMenu = new QMenu(this);
AllItemMenu->addMenu(menu1Menu);
AllItemMenu->addSeparator();
AllItemMenu->addMenu(menu2Menu);
//! AllItemMenu 添加 e
}
void MainWindow::menu1chd1ActionSlot()
{
QObject * sobj = sender();
QAction *act = qobject_cast<QAction *>(sobj);
QVariant var = act->data();
testStruct s = var.value<testStruct>();
qDebug()<<"MainWindow::menu1chd1ActionSlot"<<act->text()<<s.a<<s.b<<s.c;
}