为请求创建了一个接收者对象的链。这种模式给予请求的类型,对请求的发送者和接收者进行解耦。这种类型的设计模式属于行为型模式。在这种模式中,通常每个接收者都包含对另一个接收者的引用。如果一个对象不能处理该请求,那么它会把相同的请求传给下一个接收者,依此类推。
优点:
1、降低耦合度。它将请求的发送者和接收者解耦。
2、简化了对象。使得对象不需要知道链的结构。
3、增强给对象指派职责的灵活性。通过改变链内的成员或者调动它们的次序,允许动态地新增或者删除责任。4、增加新的请求处理类很方便。
缺点:
1、不能保证请求一定被接收。
2、系统性能将受到一定影响,而且在进行代码调试时不太方便,可能会造成循环调用。
3、可能不容易观察运行时的特征,有碍于除错。
应用:
1、servlet 的 Filter。
2、系统日志记录,当任务出现error时,需要记录debug和info日志,任务正常时,只需记录info日志。
总结:
责任链设计模式使代码更加专业,专业的东西,往往意味着难以理解。使用强判断虽容易理解,但每次修改任务链时,需要重新编写方法,使得后期维护困难。
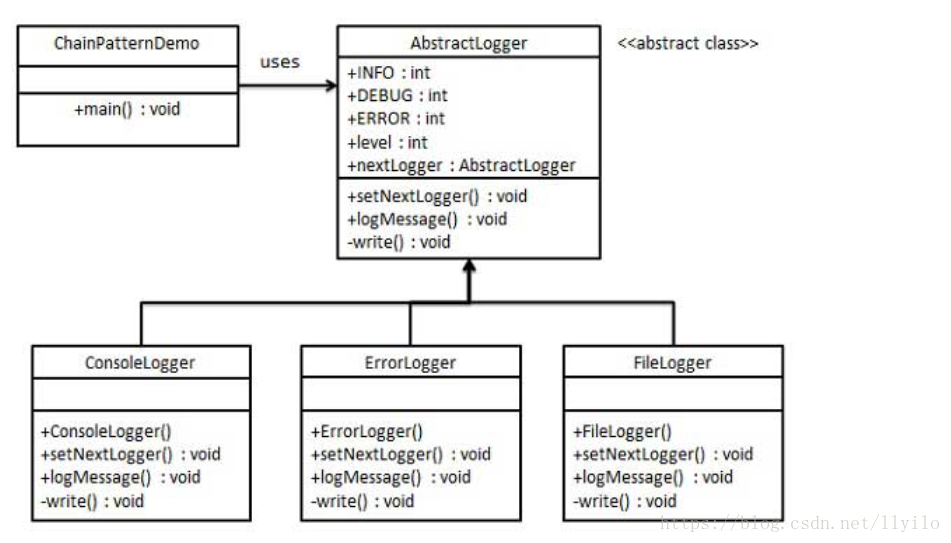
实现代码:
public abstract class AbstractLogger {
public static int INFO = 1;
public static int DEBUG = 2;
public static int ERROR = 3;
protected int level;
// 责任链中的下一个元素
protected AbstractLogger nextLogger;
public void setNextLogger(AbstractLogger nextLogger) {
this.nextLogger = nextLogger;
}
// 此处有点类似于递归的方式
public void logMessage(int level, String message) {
if (this.level <= level) {
write(message);
}
if (nextLogger != null) {
nextLogger.logMessage(level, message);
}
}
abstract protected void write(String message);
}
public class DebugLogger extends AbstractLogger {
public DebugLogger(int level) {
this.level = level;
}
@Override
protected void write(String message) {
System.out.println("File::Logger: " + message);
}
}
public class ErrorLogger extends AbstractLogger {
public ErrorLogger(int level) {
this.level = level;
}
@Override
protected void write(String message) {
System.out.println("Error Console::Logger: " + message);
}
}
public class InfoLogger extends AbstractLogger {
public InfoLogger(int level) {
this.level = level;
}
@Override
protected void write(String message) {
System.out.println("Standard Console::Logger: " + message);
}
}
public class Test {
private static AbstractLogger getChainOfLoggers() {
// 创建每个责任链的下一个责任链
AbstractLogger error = new ErrorLogger(AbstractLogger.ERROR);
AbstractLogger debug = new DebugLogger(AbstractLogger.DEBUG);
AbstractLogger info = new InfoLogger(AbstractLogger.INFO);
error.setNextLogger(debug);
debug.setNextLogger(info);
return error;
}
public static void main(String[] args) {
AbstractLogger loggerChain = getChainOfLoggers();
loggerChain.logMessage(AbstractLogger.INFO, "This is an information.");
}
}
对比代码:
public abstract class AbstractLogger {
public static int INFO = 1;
public static int DEBUG = 2;
public static int ERROR = 3;
protected int level;
abstract protected void write(String message);
}
public class DebugLogger extends AbstractLogger {
public DebugLogger(int level) {
this.level = level;
}
@Override
protected void write(String message) {
System.out.println("File::Logger: " + message);
}
}
public class ErrorLogger extends AbstractLogger {
public ErrorLogger(int level) {
this.level = level;
}
@Override
protected void write(String message) {
System.out.println("Error Console::Logger: " + message);
}
}
public class InfoLogger extends AbstractLogger {
public InfoLogger(int level) {
this.level = level;
}
@Override
protected void write(String message) {
System.out.println("Standard Console::Logger: " + message);
}
}
public class Test {
// 使用强判断进行链表
private static void getChainOfLoggers(int level) {
AbstractLogger error = new ErrorLogger(AbstractLogger.ERROR);
AbstractLogger debug = new DebugLogger(AbstractLogger.DEBUG);
AbstractLogger info = new InfoLogger(AbstractLogger.INFO);
if(level == AbstractLogger.ERROR){
error.write("This is an information.");
debug.write("This is an information.");
info.write("This is an information.");
} else if(level == AbstractLogger.DEBUG){
debug.write("This is an information.");
info.write("This is an information.");
} else {
info.write("This is an information.");
}
}
public static void main(String[] args) {
getChainOfLoggers(AbstractLogger.DEBUG);
}
}