Java项目云笔记管理系统(java+uni-app+SpringBoot+Mybatis(MybatisPlus)+Maven+Mysql)
项目运行
环境配置:
Jdk1.8 + Tomcat(SpringBoot内置) + Mysql8 + HBuilderX(Webstorm也行)+IDEA(IntelliJ IDEA, Eclispe,MyEclispe,Sts都支持)。
项目技术:
JAVA + mybatis+spring + Maven + Vue 等等组成,B/S模式 + Maven管理等等。
环境需要
1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。
2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA;
3.tomcat环境:Tomcat 7.x,8.x,9.x版本均可
4.硬件环境:windows 7/8/10 1G内存以上;或者 Mac OS;
5.是否Maven项目: 是;
6.数据库:MySql 5.7/8.0等版本均可;
技术栈
1. 后端:JAVA mybatis SpringBoot SpringMVC
2. 前端:uni-app,css,html
使用说明
1. 使用Navicat或者其它工具,在mysql中创建对应名称的数据库,并导入项目的sql文件;
2. 使用IDEA/Eclipse/MyEclipse导入项目,修改配置,运行项目;
3.管理员账号:root 密码:123456
4.开发环境为Eclipse/idea,数据库为mysql 使用java语言开发。
5.运行NoteServiceApplication.java 即可打开首页
6.数据库连接src\main\resources\application.yml中修改
7.后台路径地址:localhost:8070
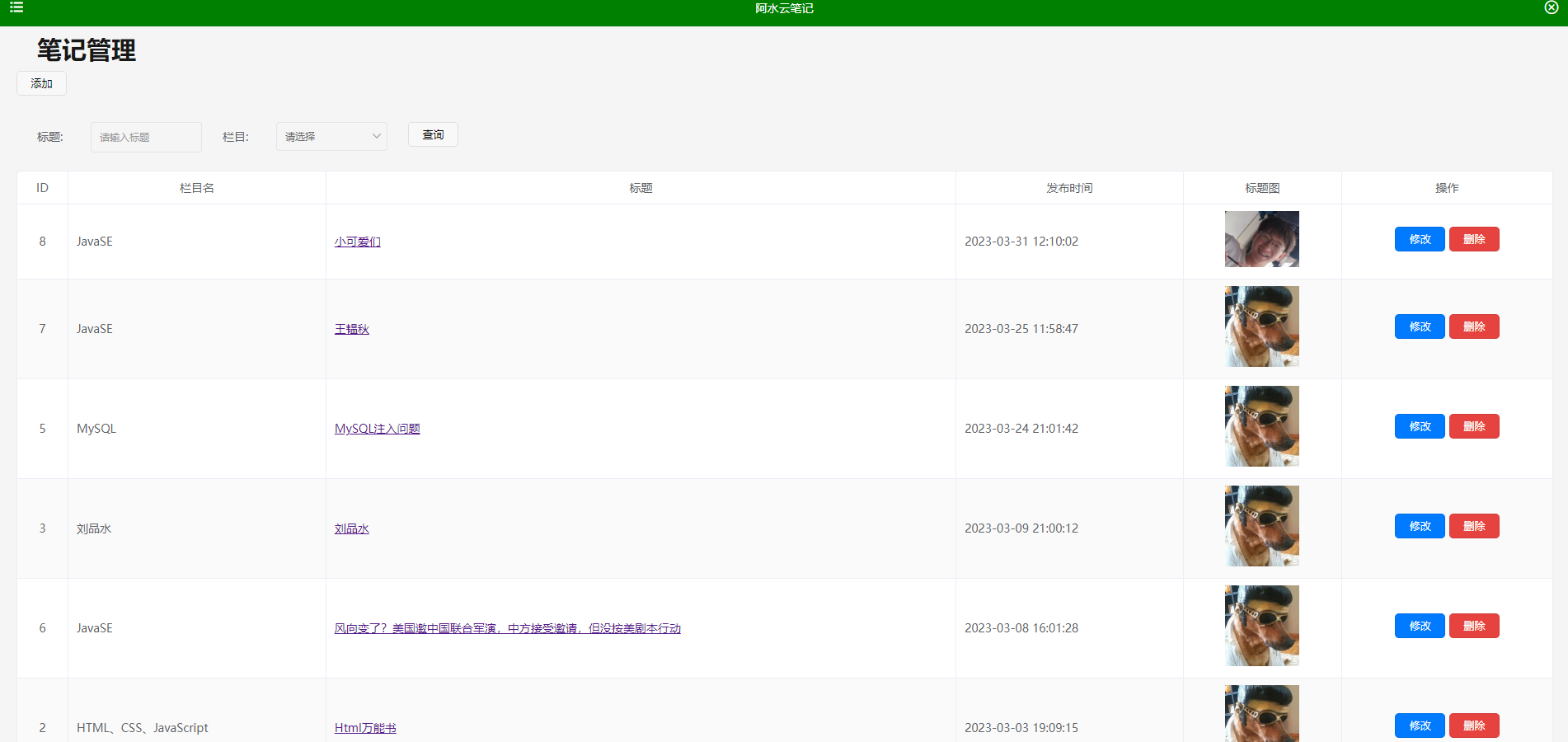
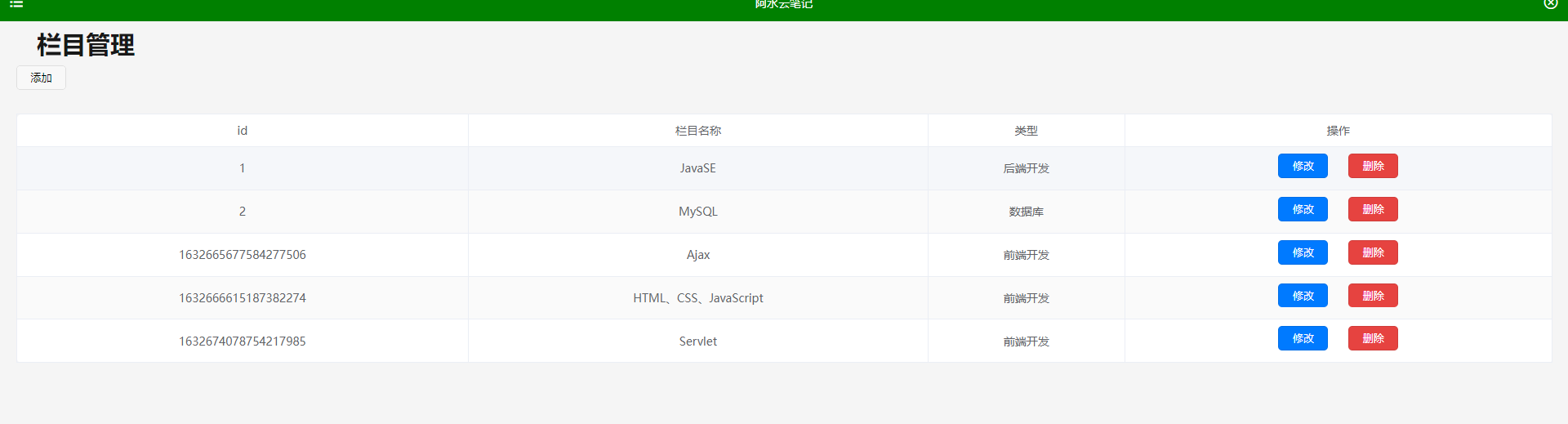
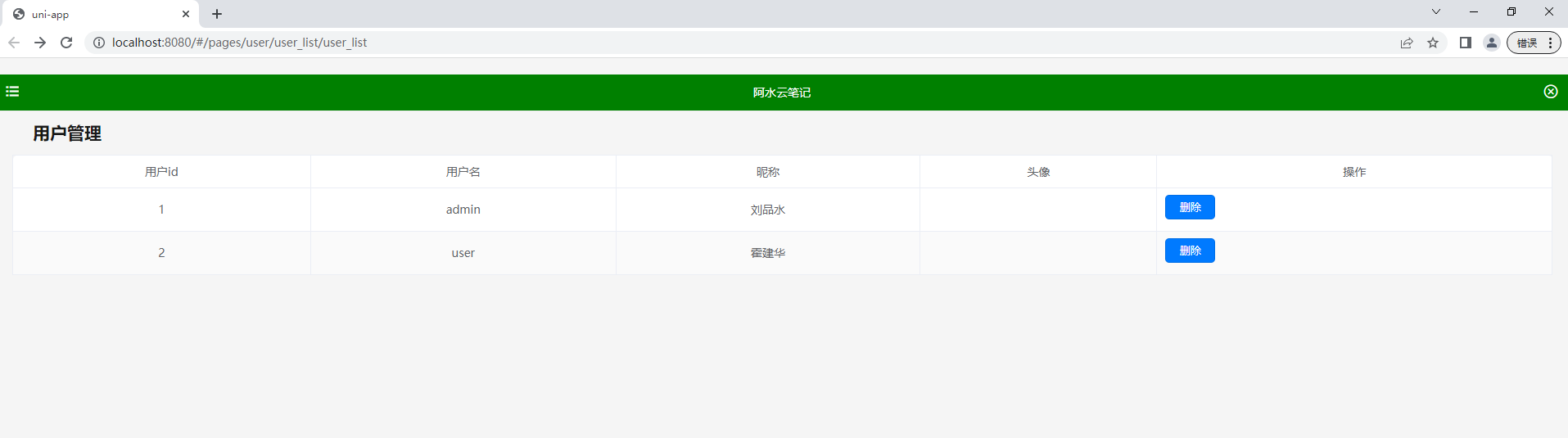

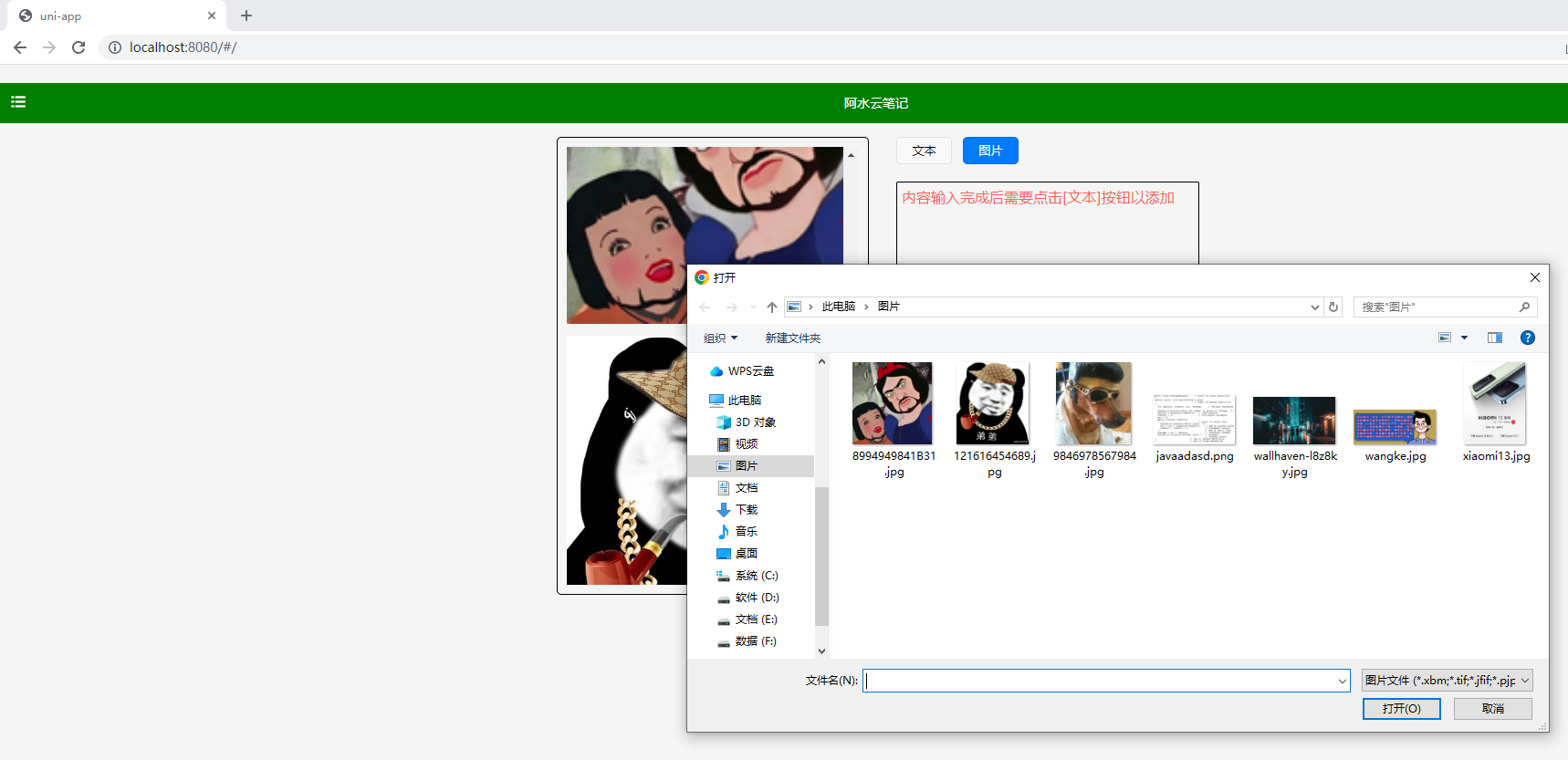
项目结构如下
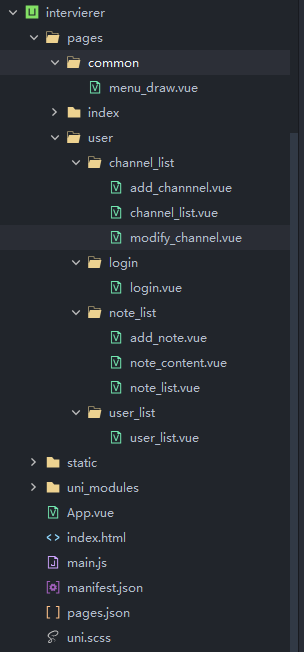
新建uni-app项目
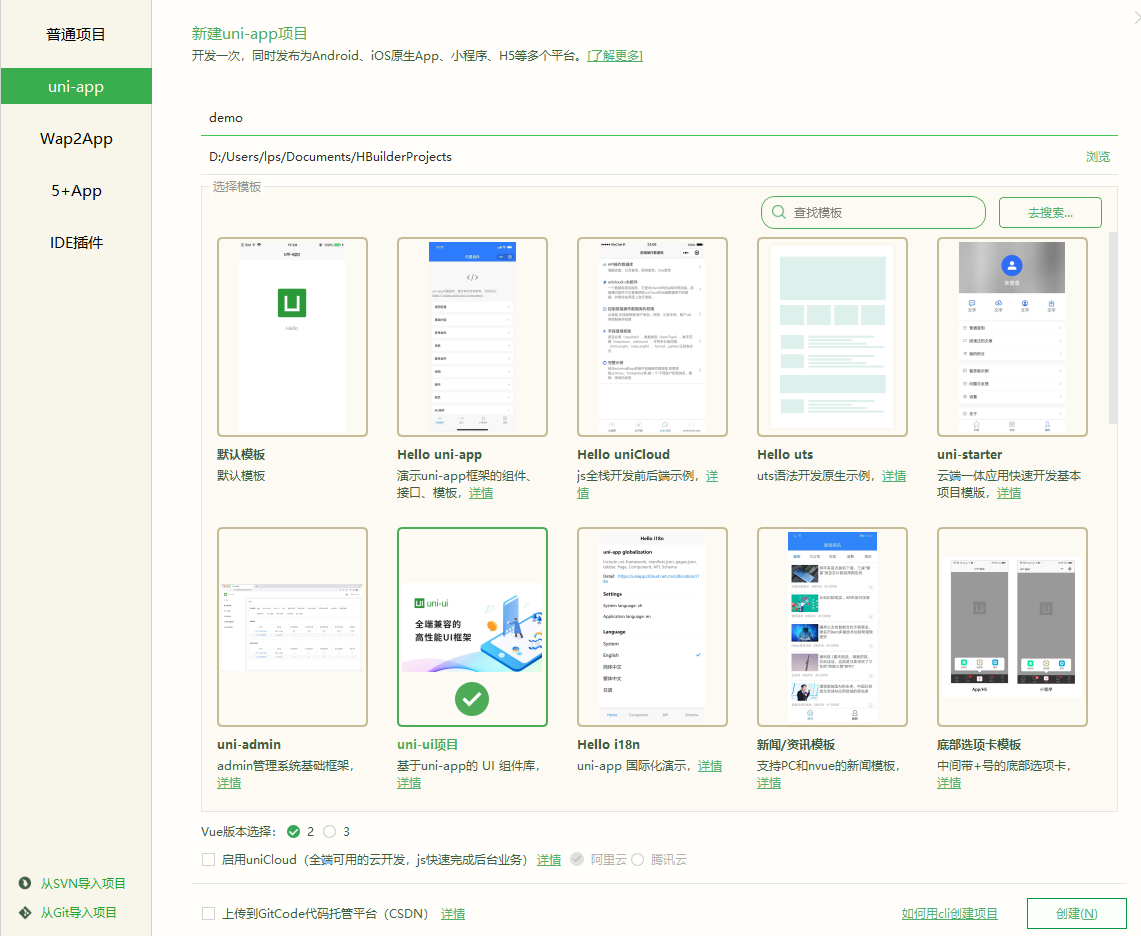
pages.json
{
"pages": [
{
"path": "pages/user/login/login",
"style": {
"navigationStyle": "custom"
}
},
{
"path": "pages/common/menu_draw",
"style": {
"navigationStyle": "custom"
}
},
{
"path": "pages/index/index",
"style": {
"navigationStyle": "custom"
}
} ,{
"path" : "pages/user/user_list/user_list",
"style" :
{
"navigationStyle": "custom"
}
}
,{
"path" : "pages/user/channel_list/channel_list",
"style" :
{
"navigationStyle": "custom"
}
},
{
"path" : "pages/user/channel_list/add_channnel",
"style" :
{
"navigationStyle": "custom"
}
},
{
"path" : "pages/user/channel_list/modify_channel",
"style" :
{
"navigationStyle": "custom"
}
},
{
"path" : "pages/user/note_list/note_list",
"style" :
{
"navigationStyle": "custom"
}
},
{
"path" : "pages/user/note_list/add_note",
"style" :
{
"navigationStyle": "custom"
}
},
{
"path" : "pages/user/note_list/note_content",
"style" :
{
"navigationStyle": "custom"
}
}
],
"globalStyle": {
"navigationBarTextStyle": "black",
"navigationBarTitleText": "uni-app",
"navigationBarBackgroundColor": "#F8F8F8",
"backgroundColor": "#F8F8F8",
"app-plus": {
"background": "#efeff4"
}
}
}
menu_draw.vue
<!--写页面的HTML代码-->
<template>
<view>
<!--导航栏-->
<uni-nav-bar left-icon="list" right-icon="close" title="阿水云笔记" backgroundColor="green" color="#ffffff" fixed="true" @clickLeft="doLeft" @clickRight="doRight" />
<!--抽屉:功能列表-->
<uni-drawer ref="showRight" mode="left" :mask-click="true">
<scroll-view style="height: 100%;" scroll-y="true">
<uni-list>
<uni-list-item :clickable="true" title="首页" @click="goIndex" :show-arrow="true"></uni-list-item>
<uni-list-item :clickable="true" title="用户管理" @click="goUser" :show-arrow="true"></uni-list-item>
<uni-list-item :clickable="true" title="栏目管理" @click="goChannel" :show-arrow="true"></uni-list-item>
<uni-list-item :clickable="true" title="笔记管理" @click="goArticle" :show-arrow="true"></uni-list-item>
</uni-list>
</scroll-view>
</uni-drawer>
</view>
</template>
<!--写业务代码,最常见的:发送网络请求-->
<script>
export default {
data() {
return {
}
},
methods: {
//导航栏左侧按钮
doLeft(){
this.showDrawer();
},
//导航栏右侧按钮
doRight(){
},
showDrawer() {
this.$refs.showRight.open();
},
closeDrawer() {
this.$refs.showRight.close();
},
//跳转到栏目列表页面
goChannel(){
uni.redirectTo({
url: '/pages/user/channel_list/channel_list'
})
},
//跳转到文章列表页面
goArticle(){
uni.redirectTo({
url: '/pages/user/note_list/note_list'
})
},
//跳转到首页
goIndex(){
uni.redirectTo({
url: '/pages/index/index'
})
},
//跳转到用户管理
goUser(){
uni.redirectTo({
url: '/pages/user/user_list/user_list'
})
}
}
}
</script>
<!--页面的效果-->
<style>
</style>
add_channel.vue
<!--写页面的HTML代码-->
<template>
<view>
<menu-draw></menu-draw>
<h2 style="margin-top: 30rpx;margin-bottom: 30rpx;padding-left: 50rpx;margin-left: auto;margin-right: auto;">
<!-- style="margin-top: 30rpx; margin-bottom: 30rpx; padding-left: 30rpx;" -->
栏目添加管理界面</h2>
<uni-forms ref="form" :model="formData" :rules="rules"
style="width: 600rpx; margin-left: auto; margin-right: auto;">
<uni-forms-item label="栏目名:" name="channelName">
<uni-easyinput v-model="formData.channelName" placeholder="请输入栏目名"></uni-easyinput>
</uni-forms-item>
<uni-forms-item label="类型:" name="channelType">
<uni-data-select :localdata="typeData" v-model="formData.channelType" @change="doChange"></uni-data-select>
</uni-forms-item>
<uni-forms-item>
<button type="primary" size="mini" @click="doAdd()" style="margin-left: 110rpx;">添加</button>
<button type="primary" size="mini" @click="doReturn()" style="margin-left: 110rpx;">返回</button>
</uni-forms-item>
</uni-forms>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm">
</uni-popup-dialog>
</uni-popup>
</view>
</template>
<!--写业务代码,最常见的:发送网络请求-->
<script>
import menuDraw from '../../common/menu_draw.vue'
export default {
//2、外部的组件
components: {
menuDraw
},
data() {
return {
msg: null,
formData: {
channelName: null,
channelType: null,
},
typeData: [{
value: "1",
text: '前端开发'
},
{
value: "2",
text: "后端开发"
},
{
value: "3",
text: "服务器开发"
},
{
value: "4",
text: "数据库开发"
}
],
rules: {
channelName: {
rules: [{
required: true,
errorMessage: '请输入栏目名',
}, ]
},
channelType: {
rules: [{
required: true,
errorMessage: '请选择类型',
}, ]
},
}
}
},
methods: {
doChange(e) {
console.log(e)
},
doLeft() {
this.msg = "点击了返回按钮"
this.open();
},
open() {
this.$refs.popup.open()
},
/**
* 点击取消按钮触发
*/
close() {
this.$refs.popup.close()
},
/**
* 点击确认按钮触发
*/
confirm(value) {
this.$refs.popup.close()
},
doAdd() {
this.$refs.form.validate().then(res => {
uni.request({
url: 'http://localhost:8070/channel/save',
method: 'POST',
data: {
//注意前面的是数据库的格式
channelName: this.formData.channelName,
type: this.formData.channelType
},
success: (res) => {
console.log(res.data);
if (res.data.code == 200) {
uni.redirectTo({
url: 'channel_list'
});
} else {
this.msg = res.data.msg;
this.open();
}
}
})
}).catch(err => {
console.log('表单错误信息:', err);
})
},
doReturn(){
uni.redirectTo({
url: "channel_list"
})
}
}
}
</script>
<!--页面的效果-->
<style>
</style>
channel_list.vue
<template>
<view class="container">
<menuDraw></menuDraw>
<h2 style="margin-top: 30rpx;margin-bottom: 30rpx;padding-left: 50rpx;margin-left: auto;margin-right: auto;">
栏目管理</h2>
<uni-forms-item>
<button type="default" size="mini" @click="doAdd()">添加</button>
</uni-forms-item>
<uni-table stripe border :loading="loading">
<uni-tr>
<uni-td align="center">id</uni-td>
<uni-td align="center">栏目名称</uni-td>
<uni-td align="center">类型</uni-td>
<uni-td align="center">操作</uni-td>
</uni-tr>
<uni-tr v-for="u in channelList ">
<uni-td align="center">{{u.id}}</uni-td>
<uni-td align="center">{{u.channelName}}</uni-td>
<uni-td align="center">
<!-- {{u.type==1?"前端开发":u.type==2?"后端开发":u.type==3?"服务器":"数据库"}} -->
<view v-if="u.type==1">
前端开发
</view>
<view v-if="u.type==2">
后端开发
</view>
<view v-if="u.type==3">
服务器
</view>
<view v-if="u.type==4">
数据库
</view>
</uni-td>
<uni-td align="center">
<button type="primary" size="mini" @click="doUpdate(u.id)">修改</button>
<button type="warn" size="mini" @click="doDelete(u.id)" style="margin-left: 50rpx;">删除</button>
</uni-td>
</uni-tr>
</uni-table>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm">
</uni-popup-dialog>
</uni-popup>
</view>
</template>
<script>
import menuDraw from '../../common/menu_draw.vue'
export default {
//2、外部的组件
components: {
menuDraw
},
data() {
return {
channelList: null,
msg: null,
loading: null,
}
},
methods: {
requestchannelList() {
this.loading = true
uni.request({
url: 'http://localhost:8070/channel/list',
method: 'GET',
success: (res) => {
console.log(res.data)
this.loading = false
this.channelList = res.data.data
}
})
},
doDelete(id) {
uni.request({
url: "http://localhost:8070/channel/remove?id=" + id,
method: 'GET',
success: (res) => {
console.log(res.data)
if (res.data.code == 200) {
// this.msg = "删除成功啦,宝贝!"
// this.open
this.requestchannelList()
} else {
this.msg = res.data.msg;
this.open
}
}
})
},
doAdd() {
uni.redirectTo({
url: "add_channnel"
})
},
doUpdate(id){
uni.redirectTo({
url: "modify_channel?id="+id
})
},
open() {
this.$refs.popup.open()
},
/**
* 点击取消按钮触发
*/
close() {
this.$refs.popup.close()
},
/**
* 点击确认按钮触发
*/
confirm(value) {
this.$refs.popup.close()
},
},
onLoad() {
uni.request({
url: 'http://localhost:8070/channel/list',
method: 'GET',
success: (res) => {
console.log(res.data)
this.channelList = res.data.data
}
})
}
}
</script>
<style>
.container {
padding: 20px;
font-size: 20px;
line-height: 24px;
}
</style>
modify_channel.vue
<!--写页面的HTML代码-->
<template>
<view>
<menu-draw></menu-draw>
<h2 style="margin-top: 30rpx;margin-bottom: 30rpx;padding-left: 50rpx;margin-left: auto;margin-right: auto;">
栏目修改管理界面</h2>
<uni-forms ref="form" :model="formData" :rules="rules"
style="width: 600rpx; margin-left: auto; margin-right: auto;">
<uni-forms-item label="栏目名:" name="channelName">
<uni-easyinput v-model="formData.channelName" placeholder="请输入栏目名"></uni-easyinput>
</uni-forms-item>
<uni-forms-item label="类型:" name="channelType">
<uni-data-select :localdata="typeData" v-model="formData.channelType" @change="doChange">
</uni-data-select>
</uni-forms-item>
<uni-forms-item>
<button type="primary" size="mini" @click="doAdd()" style="margin-left: 110rpx;">修改</button>
<button type="primary" size="mini" @click="doReturn()" style="margin-left: 110rpx;">返回</button>
</uni-forms-item>
</uni-forms>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm">
</uni-popup-dialog>
</uni-popup>
</view>
</template>
<!--写业务代码,最常见的:发送网络请求-->
<script>
import menuDraw from '../../common/menu_draw.vue'
export default {
//2、外部的组件
components: {
menuDraw
},
data() {
return {
msg: null,
id: null,
formData: {
channelName: null,
channelType: null,
},
typeData: [{
value: 1,
text: '前端开发'
},
{
value: 2,
text: "后端开发"
},
{
value: 3,
text: "服务器开发"
},
{
value: 4,
text: "数据库开发"
}
],
rules: {
channelName: {
rules: [{
required: true,
errorMessage: '请输入栏目名',
}, ]
},
channelType: {
rules: [{
required: true,
errorMessage: '请选择类型',
}, ]
},
}
}
},
methods: {
doChange(e) {
console.log(e)
},
doLeft() {
this.msg = "点击了返回按钮"
this.open();
},
open() {
this.$refs.popup.open()
},
/**
* 点击取消按钮触发
*/
close() {
this.$refs.popup.close()
},
/**
* 点击确认按钮触发
*/
confirm(value) {
this.$refs.popup.close()
},
doAdd() {
this.$refs.form.validate().then(res => {
uni.request({
url: 'http://localhost:8070/channel/modify',
method: 'POST',
data: {
//注意前面的是数据库的格式
channelName: this.formData.channelName,
type: this.formData.channelType,
id: this.id,
},
success: (res) => {
console.log(res.data);
if (res.data.code == 200) {
uni.redirectTo({
url: 'channel_list'
});
} else {
this.msg = res.data.msg;
this.open();
}
}
})
}).catch(err => {
console.log('表单错误信息:', err);
})
},
doReturn(){
uni.redirectTo({
url: "channel_list"
})
}
},
onLoad(options) {
console.log("传来的总体" + options)
console.log("传来的id是:" + options.id)
this.id = options.id;
uni.request({
url: 'http://localhost:8070/channel/get?id=' + this.id,
method: 'GET',
success: (res) => {
console.log(res.data)
this.formData.channelName = res.data.data.channelName
this.formData.channelType = res.data.data.type
}
})
}
}
</script>
<!--页面的效果-->
<style>
</style>
login.vue
<template>
<view>
<uni-forms ref="form" :model="formData" :rules="rules"
style="width: 600rpx; margin-top: 50rpx; margin-left: auto;margin-right: auto;">
<uni-forms-item style="margin-top: 100rpx; text-align: center;">
<h1>用户登录</h1>
</uni-forms-item>
<uni-forms-item label="用户名" name="userName">
<uni-easyinput v-model="formData.userName" placeholder="请输入账号"></uni-easyinput>
</uni-forms-item>
<uni-forms-item label="密码" name="passwd">
<uni-easyinput v-model="formData.passwd" type="password" placeholder="请输入密码"></uni-easyinput>
</uni-forms-item>
<uni-forms-item>
<button type="primary" size="mini" @click="doLogin">登录</button>
</uni-forms-item>
</uni-forms>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm">
</uni-popup-dialog>
</uni-popup>
</view>
</template>
<script>
export default {
data() {
return {
msg: null,
formData: {
userName: null,
passwd: null,
},
rules: {
userName: {
rules: [{
required: true,
errorMessage: '请输入用户名',
}, ]
},
passwd: {
rules: [{
required: true,
errorMessage: '请输入密码',
}, ]
},
}
}
},
methods: {
doLogin() {
//进行表单校验
this.$refs.form.validate().then(res => {
uni.request({
url: "http://localhost:8070/user/login",
method: 'POST',
data: {
userName: this.formData.userName,
passwd: this.formData.passwd
},
success: (res) => {
console.log(res.data);
if (res.data.code == 200) {
uni.setStorageSync('user_info', res.data.data); //将用户信息保存到本地
//跳转到主页
uni.redirectTo({
url: "/pages/index/index",
})
} else {
this.msg = res.data.msg
this.open()
}
}
})
}).catch(err => {
console.log('表单错误信息:', err);
})
},
open() {
this.$refs.popup.open()
},
/**
* 点击取消按钮触发
*/
close() {
this.$refs.popup.close()
},
/**
* 点击确认按钮触发
*/
confirm(value) {
this.$refs.popup.close()
},
}
}
</script>
<style>
</style>
add_note.vue
<template>
<view>
<uni-forms ref="form" :model="formData" :rules="rules"
style="width: 600rpx; margin-top: 50rpx; margin-left: auto;margin-right: auto;">
<uni-forms-item style="margin-top: 100rpx; text-align: center;">
<h1>用户登录</h1>
</uni-forms-item>
<uni-forms-item label="用户名" name="userName">
<uni-easyinput v-model="formData.userName" placeholder="请输入账号"></uni-easyinput>
</uni-forms-item>
<uni-forms-item label="密码" name="passwd">
<uni-easyinput v-model="formData.passwd" type="password" placeholder="请输入密码"></uni-easyinput>
</uni-forms-item>
<uni-forms-item>
<button type="primary" size="mini" @click="doLogin">登录</button>
</uni-forms-item>
</uni-forms>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm">
</uni-popup-dialog>
</uni-popup>
</view>
</template>
<script>
export default {
data() {
return {
msg: null,
formData: {
userName: null,
passwd: null,
},
rules: {
userName: {
rules: [{
required: true,
errorMessage: '请输入用户名',
}, ]
},
passwd: {
rules: [{
required: true,
errorMessage: '请输入密码',
}, ]
},
}
}
},
methods: {
doLogin() {
//进行表单校验
this.$refs.form.validate().then(res => {
uni.request({
url: "http://localhost:8070/user/login",
method: 'POST',
data: {
userName: this.formData.userName,
passwd: this.formData.passwd
},
success: (res) => {
console.log(res.data);
if (res.data.code == 200) {
uni.setStorageSync('user_info', res.data.data); //将用户信息保存到本地
//跳转到主页
uni.redirectTo({
url: "/pages/index/index",
})
} else {
this.msg = res.data.msg
this.open()
}
}
})
}).catch(err => {
console.log('表单错误信息:', err);
})
},
open() {
this.$refs.popup.open()
},
/**
* 点击取消按钮触发
*/
close() {
this.$refs.popup.close()
},
/**
* 点击确认按钮触发
*/
confirm(value) {
this.$refs.popup.close()
},
}
}
</script>
<style>
</style>
note_content.vue
<template>
<view class="container">
<menuDraw></menuDraw>
<div style="margin-top: 30rpx; margin-left: auto; margin-right: auto; width: 1400rpx; height: 960rpx;">
<div
style="width: 640rpx; height: 960rpx; margin-right: 10rpx; padding: 20rpx; border: 1px solid #000000; border-radius: 10rpx; float: left;">
<scroll-view scroll-y="true" style="height: 960rpx;">
<div style="text-align: center; margin-bottom: 30rpx;"><b>{{title}}</b></div>
<div v-for="c in contentList" style="margin-bottom: 12rpx;">
<div v-if="c.type=='txt'">{{c.content}}</div>
<div v-if="c.type=='img'">
<image :src="serverUrl+c.content" style="640rpx;" mode="widthFix"></image>
</div>
</div>
</scroll-view>
</div>
<div style="width: 640rpx; height: 960rpx; margin-left: 50rpx; float: left;">
<div style="margin-bottom: 25rpx;">
<button @click="doTxt" class="mini-btn" type="default" size="mini"
style="margin-right: 25rpx;">文本</button>
<button @click="doImg" class="mini-btn" type="primary" size="mini"
style="margin-right: 25rpx;">图片</button>
</div>
<textarea placeholder-style="color:#F76260" placeholder="内容输入完成后需要点击[文本]按钮以添加" v-model="articleValue"
style="border: 1px solid #000000; border-radius: 5rpx; height: 300rpx; width: 640rpx; padding: 10rpx; margin-bottom: 35rpx;" />
</div>
</div>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm"></uni-popup-dialog>
</uni-popup>
</view>
</template>
<script>
import menuDraw from '../../common/menu_draw.vue'
var _self;
export default {
components: {
menuDraw
},
data() {
return {
serverUrl: 'http://localhost:8060', //文件服务器地址
title: null,
nid: null,
msg: null,
title: null,
articleValue: null,
contentList: null
}
},
methods: {
requestContent() {
uni.request({
url: 'http://localhost:8070/content/list?nid=' + this.nid,
method: 'GET',
success: (res) => {
console.log(res.data)
this.contentList = res.data.data
}
})
},
open() {
this.$refs.popup.open()
},
close() {
this.$refs.popup.close()
},
confirm(value) {
this.$refs.popup.close()
},
doTxt() {
uni.request({
url: 'http://localhost:8070/content/save',
method: 'POST',
data: {
nid: this.nid,
type: 'txt',
content: this.articleValue
},
success: (res) => {
console.log(res.data)
if (res.data.code == 200) {
//刷新列表
this.requestContent()
this.articleValue = null
} else {
this.msg = res.data.msg
this.open()
}
}
})
},
doImg() {
//选择图片
_self = this;
//打开手机权限 选择上传的文件
uni.chooseImage({
count:1,
sizeType: ['original', 'compressed'], //可以指定是原图还是压缩图,默认二者都有
sourceType: ['album'], //从相册选择
success: (res) => {
const tempFilePaths=res.tempFilePaths;
//上传图片
uni.uploadFile({
url:'http://localhost:8070/upload/file',
filePath:tempFilePaths[0],
name: 'fileName',
success: (res) => {
console.log(res.data)
uni.request({
url:'http://localhost:8070/content/save',
method:'POST',
data:{
nid:_self.nid,
type:'img',
content:res.data
},
success: (res) => {
console.log(res.data)
if(res.data.code==200){
//刷新列表
_self.requestContent()
_self.articleValue=null
}else{
_self.msg=res.data.msg
_self.open()
}
}
})
}
})
}
})
}
},
onLoad(options) {
this.nid = options.nid
this.title = options.title
this.requestContent()
}
}
</script>
<style>
.container {
padding: 20px;
font-size: 14px;
line-height: 24px;
}
</style>
note_list.vue
<template>
<view class="container">
<menuDraw></menuDraw>
<h2 style="margin-top: 30rpx;margin-bottom: 30rpx;padding-left: 50rpx;margin-left: auto;margin-right: auto;">
笔记管理</h2>
<uni-forms-item>
<button type="default" size="mini" @click="doAdd()">添加</button>
</uni-forms-item>
<uni-forms v-model="queryParam" style="margin-top: 50rpx; margin-left: 50rpx;">
<uni-forms-item label="标题:" style="width: 400rpx; float: left; margin-right: 50rpx;">
<uni-easyinput v-model="queryParam.title" placeholder="请输入标题"></uni-easyinput>
</uni-forms-item>
<uni-forms-item label="栏目:" style="width: 400rpx; float: left; margin-right: 50rpx;">
<uni-data-select v-model="queryParam.channelName" :localdata="range" @change="change"></uni-data-select>
</uni-forms-item>
<uni-forms-item>
<button type="default" size="mini" style="margin-right: 15rpx;" @click="doSearch">查询</button>
</uni-forms-item>
</uni-forms>
<uni-table stripe border :loading="loading">
<uni-tr>
<uni-td align="center">ID</uni-td>
<uni-td align="center">栏目名</uni-td>
<uni-td align="center">标题</uni-td>
<uni-td align="center">发布时间</uni-td>
<uni-td align="center">标题图</uni-td>
<uni-td align="center">操作</uni-td>
</uni-tr>
<uni-tr v-for="n in noteList">
<uni-td align="center">{{n.id}}</uni-td>
<uni-td>{{n.channel.channelName}}</uni-td>
<uni-td><a href="#" @click="goContent(n.id,n.title)">{{n.title}}</a></uni-td>
<uni-td>{{n.publishTime}}</uni-td>
<uni-td align="center">
<image :src="serverUrl+n.logo" style="width: 180rpx" mode="widthFix"></image>
</uni-td>
<uni-td align="center">
<button type="primary" size="mini" @click="doUpdate(n.id)" style="margin-right: 10rpx;">修改</button>
<button type="warn" size="mini" @click="doDelete(n.id)">删除</button>
</uni-td>
</uni-tr>
</uni-table>
<uni-pagination style="margin-top: 30rpx;" :current="pageIndex" :page-size="pageSize" :total="pageTotle"
@change="pageChanged"></uni-pagination>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm">
</uni-popup-dialog>
</uni-popup>
</view>
</template>
<script>
import menuDraw from '../../common/menu_draw.vue'
export default {
//2、外部的组件
components: {
menuDraw
},
data() {
return {
serverUrl: 'http://localhost:8060', //文件服务器地址
//分页相关
pageIndex: 1,
pageSize: 10,
pageTotle: 0,
range: [],
queryParam: {
title: null,
channelName: null
},
loading: false,
msg: null,
noteList: null
}
},
methods: {
requestnoteList() {
this.loading = true
if (this.queryParam.channelName == null) {
this.queryParam.channelName = 0;
}
uni.request({
url: 'http://localhost:8070/note/list?title=' + this.queryParam.title + "&cid=" + this
.queryParam.channelName + "&pageIndex=" + this.pageIndex + "&pageSize=" + this.pageSize,
method: 'GET',
success: (res) => {
console.log(res.data)
this.loading = false
this.noteList = res.data.data
this.pageTotle = res.data.total
}
})
},
doDelete(id) {
uni.request({
url: "http://localhost:8070/note/remove?id=" + id,
method: 'GET',
success: (res) => {
console.log(res.data)
if (res.data.code == 200) {
this.requestnoteList()
} else {
this.msg = res.data.msg;
this.open
}
}
})
},
doAdd() {
uni.redirectTo({
url: '/pages/user/note_list/add_note'
})
},
doUpdate(id) {
uni.redirectTo({
url: '/pages/channel/channel_update/channel_update?id=' + id
})
},
open() {
this.$refs.popup.open()
},
/**
* 点击取消按钮触发
*/
close() {
this.$refs.popup.close()
},
/**
* 点击确认按钮触发
*/
confirm(value) {
this.$refs.popup.close()
},
change(e) {
console.log(e);
},
pageChanged(p) {
this.pageIndex = p.current; //设置被点击的
this.requestnoteList();
},
doSearch() {
this.requestnoteList();
},
goContent(id,title){
uni.redirectTo({
url: 'note_content?nid='+id+"&title="+title
})
}
},
onLoad() {
this.requestnoteList()
uni.request({
url: 'http://localhost:8070/channel/list',
method: 'GET',
success: (res) => {
console.log(res.data)
var channelList = res.data.data;
for (var i = 0; i < channelList.length; i++) {
var temp = {
value: channelList[i].id,
text: channelList[i].channelName
}
this.range.push(temp)
}
console.log(this.range)
}
})
}
}
</script>
<style>
.container {
padding: 20px;
font-size: 20px;
line-height: 24px;
}
</style>
user_list.vue
<template>
<view class="container">
<menuDraw></menuDraw>
<h2 style="margin-top: 30rpx;margin-bottom: 30rpx;padding-left: 50rpx;">用户管理</h2>
<uni-table stripe border :loading="loading">
<uni-tr>
<uni-td align="center">用户id</uni-td>
<uni-td align="center">用户名</uni-td>
<uni-td align="center">昵称</uni-td>
<uni-td align="center">头像</uni-td>
<uni-td align="center">操作</uni-td>
</uni-tr>
<uni-tr v-for="u in userList ">
<uni-td align="center">{{u.id}}</uni-td>
<uni-td align="center">{{u.userName}}</uni-td>
<uni-td align="center">{{u.nickName}}</uni-td>
<uni-td align="center">{{u.imgUrl}}</uni-td>
<uni-td>
<button type="primary" size="mini" @click="doDelete(u.id)">删除</button>
</uni-td>
</uni-tr>
</uni-table>
<!-- 提示框 -->
<uni-popup ref="popup" type="dialog">
<uni-popup-dialog mode="base" title="通知" :content="msg" :duration="2000" :before-close="true" @close="close"
@confirm="confirm">
</uni-popup-dialog>
</uni-popup>
</view>
</template>
<script>
import menuDraw from '../../common/menu_draw.vue'
export default {
//2、外部的组件
components: {
menuDraw
},
data() {
return {
userList: null,
msg: null,
loading:null,
}
},
methods: {
requestUserList(){
this.loading=true
uni.request({
url: 'http://localhost:8070/user/list',
method: 'GET',
success: (res) => {
console.log(res.data)
this.loading=false
this.userList = res.data.data
}
})
},
doDelete(id) {
uni.request({
url: "http://localhost:8070/user/remove?id=" + id,
method: 'GET',
success: (res) => {
console.log(res.data)
if (res.data.code == 200) {
// this.msg = "删除成功啦,宝贝!"
// this.open
this.requestUserList()
} else {
this.msg = res.data.msg;
this.open
}
}
})
},
open() {
this.$refs.popup.open()
},
/**
* 点击取消按钮触发
*/
close() {
this.$refs.popup.close()
},
/**
* 点击确认按钮触发
*/
confirm(value) {
this.$refs.popup.close()
},
},
onLoad() {
uni.request({
url: 'http://localhost:8070/user/list',
method: 'GET',
success: (res) => {
console.log(res.data)
this.userList = res.data.data
}
})
}
}
</script>
<style>
.container {
padding: 20px;
font-size: 14px;
line-height: 24px;
}
</style>