之前我也用过mysql数据库,那个时候我还没有接触过qt,现在我们可以通过图形界面的形式对数据库进行操作。
一、简单的mysql的语法
每条语句都以分号结尾。
创建一个数据库
格式为:create database 数据库名;
例:create database test;
创建一个表
格式为:create table 表名(内容 类型);
例:create table student(number int , name char(32), score double);
向表中插入信息
格式为:insert into 表名 values(表中的内容);
例:insert into student values(1, 'xiaoming', 99);
从表格中删除信息
格式为:delete from 表名 where 列名 = 条件;
例:delete from student where name = 'xiaoming';
查询表中的内容
格式为:select 列名 from 表名 where 列名 = 条件;
例:select score from student where name = 'xiaoming';
我们可以用这样的语句查询所有的信息
select * from student;
修改表中的数据
格式为:update 表名 set 列名 = 新内容 where 列名 = 条件;
例:update student set score = 66 where name = 'xiaoming';
从数据库中删除一个表
格式为:drop table 表名;
例:drop table student;
二、使用qt创建图形界面对数据库进行操作
首先我们先用qt creator 创建一个项目,这里我们使用图形界面,所以要勾选创建图形界面,新建完成之后我们需要将其部好局,如下图所示。
我们如果要使用数据库就需要在pro 文件中加上这句话 QT += sql。
首先我们需要自己创建一个数据库,然后利用qt和数据库进行连接。
-
db = QSqlDatabase::addDatabase(
"QMYSQL");
//a
-
db.setHostName(
"127.0.0.1");
//b
-
db.setDatabaseName(
"test");
//c
-
db.setUserName(
"root");
//d
-
db.setPassword(
"123456");
//e
以上的过程就是和数据库进行连接,a表示我们需要使用的是哪种数据库
b是设置主机名,c是设置用户名,表示我们使用哪个数据库, d是设置用户名,e是设置密码,这个密码是和数据库登录时候的密码一致。如果不一致,我们就不能成功和数据库进行连接。
如果连接成功我们就可以通过以上的按钮对数据库进行操作
插入信息到数据库
右击插入转到槽,只要在这个槽函数中编写插入数据库的函数就行了。
-
QString namestr = ui->lineEditName->text();
-
int num = ui->lineEditNumber->text().toInt();
-
double score = ui->lineEditScore->text().toDouble();
-
QString str = QString(
"insert into student(num, name, score) values('%1', '%2', '%3')").arg(num).arg(namestr).arg(score);
-
QSqlQuery query;
-
query.exec(str);
-
ui->textEdit->setText(
"插入成功");
以上的代码首先就是获取三个行编辑框中的内容并且将它们转换为相应的数据类型。然后我们使用数据库语句,将这些信息存到数据库中,如果存入成功,下方的文本编辑框会显示插入成功。
删除数据库中的信息
我们首先需要从界面中获取需要删除的人名,然后将利用数据库的语句将这条信息从数据库中删除。右击删除转到槽,我们只要在这个槽函数中编辑以上所说的功能就行了。
-
QString name = ui->lineEditName->text();
//从行编辑框中获取需要删除的人名
-
QString str = QString(
"delete from student where name = '%1'").arg(name);
-
query.exec(str);
-
ui->textEdit->setText(
"删除成功");
这个就是从数据库中删除我们希望删除的信息,删除完成之后下方的文本编辑框就会提示相应的信息。
修改数据库中的内容
这个步骤和删除的步骤差不多,首先需要从界面中获取需要修改的信息,然后利用数据库语句根据条件修改信息
-
//从界面获取我们需要的信息
-
QString updatename = ui->lineEditName->text();
-
int number = ui->lineEditNumber->text().toInt();
-
double score = ui->lineEditScore->text().toDouble();
-
temp = QString(
"update student set num = '%1' , score = '%2' where name = '%3'").arg(number).arg(score).arg(updatename);
-
query.exec(temp);
//执行修改信息的操作
-
ui->textEdit->setText(
"修改成功");
和之前的一样,我们在修成完成之后,会在下方的文本编辑框中显示修改成功的字样。
根据条件查询数据库的信息
我这里写的就是根据一个人的名字来查询他的所有信息,包括名字,学号,成绩,然后让其显示在相应的行编辑中。
-
QString searchname = ui->lineEditName->text();
-
QString str = QString(
"select *from student where name = '%1'").arg(searchname);
-
QSqlQuery query;
-
query.exec(str);
-
QString name;
-
int number;
-
double score;
-
-
while (query.next())
-
{
-
number = query.value(
0).toInt();
-
name = query.value(
1).toString();
-
score = query.value(
2).toDouble();
-
}
-
ui->lineEditName->setText(name);
-
ui->lineEditNumber->setText(QString().setNum(number));
-
ui->lineEditScore->setText(QString().setNum(score));
-
ui->textEdit->setText(
"查询成功");
对数据库查询的操作是这样的,数据库会根据数据库语句一行一行的查询,查询到复合条件的内容就会停止,我们只要将数据库中的查询出来的东西转为相应的数据类型就行了,然后将它们显示在相应的行编辑框中就行了,同样的如果查询成功就会在下方的文本编辑框中显示查询成功的字样。
三、以下是我的代码和界面
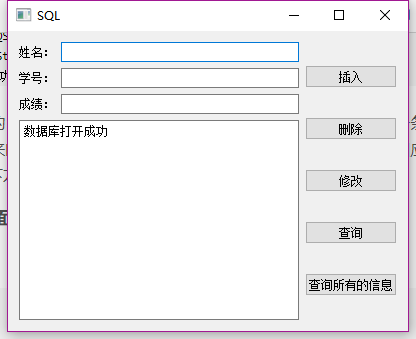
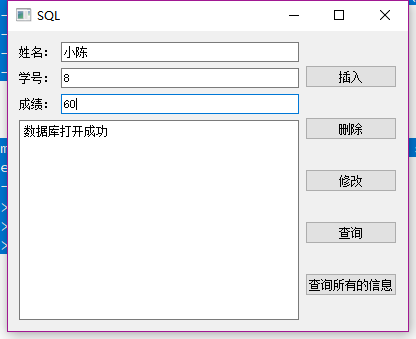
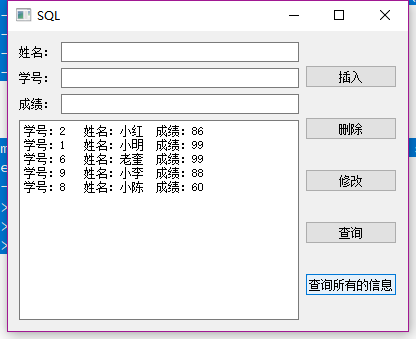
下面的代码我加了一些出错处理,以及提示信息。
-
#include "widget.h"
-
#include "ui_widget.h"
-
#include <QTextCodec>
-
#include <QMessageBox>
-
#include <QDebug>
-
-
-
Widget::Widget(QWidget *parent) :
-
QWidget(parent),
-
ui(
new Ui::Widget)
-
{
-
ui->setupUi(
this);
-
qDebug() << QSqlDatabase::drivers();
//打印qt支持的数据库类型
-
setWindowTitle(
"SQL");
//设置窗口的标题
-
-
QTextCodec::setCodecForLocale(QTextCodec::codecForLocale());
//设置显示中文
-
db = QSqlDatabase::addDatabase(
"QMYSQL");
-
db.setHostName(
"127.0.0.1");
-
db.setDatabaseName(
"test");
-
db.setUserName(
"root");
-
db.setPassword(
"123456");
-
-
if(!db.open())
//如果数据库打开失败,会弹出一个警告窗口
-
{
-
QMessageBox::warning(
this,
"警告",
"数据库打开失败");
-
}
-
else
-
{
-
ui->textEdit->setText(
"数据库打开成功");
-
}
-
-
// QString str = "create table student(num int, name varchar(32), score double);";
-
// QSqlQuery query;
-
// query.exec(str);
-
-
-
}
-
-
Widget::~Widget()
-
{
-
delete ui;
-
}
-
-
void Widget::on_pushButtonInsert_clicked()
-
{
-
QString namestr = ui->lineEditName->text();
-
int num = ui->lineEditNumber->text().toInt();
-
double score = ui->lineEditScore->text().toDouble();
-
if(namestr ==
NULL || num ==
0 || ui->lineEditScore ==
NULL)
//插入信息的时候需要输入完整的信息
-
{
-
ui->textEdit->setText(
"请输入完整的信息");
-
}
-
else
-
{
-
QString str = QString(
"insert into student(num, name, score) values('%1', '%2', '%3')").arg(num).arg(namestr).arg(score);
-
QSqlQuery query;
-
query.exec(str);
//执行插入操作
-
ui->lineEditName->clear();
-
ui->lineEditNumber->clear();
-
ui->lineEditScore->clear();
-
ui->textEdit->setText(
"插入成功");
-
}
-
-
}
-
-
void Widget::on_pushButtonDelete_clicked()
-
{
-
QString name = ui->lineEditName->text();
//从行编辑框中获取需要删除的人名
-
if(name ==
NULL)
-
{
-
-
ui->textEdit->setText(
"请输入需要删除的人的名字");
//删除的时候需要输入姓名
-
}
-
-
else
-
{
-
//从数据库中查询是否有这个人
-
QSqlQuery query;
-
QString temp = QString(
"select * from student where name = '%1'").arg(name);
-
query.exec(temp);
-
QString deletename;
-
while (query.next())
-
{
-
deletename = query.value(
1).toString();
-
}
-
if(deletename ==
NULL)
-
{
-
QString a = QString(
"没有叫%1的人,删除失败").arg(name);
-
ui->textEdit->setText(a);
-
ui->lineEditName->clear();
-
ui->lineEditNumber->clear();
-
ui->lineEditScore->clear();
-
}
-
else
-
{
-
QString str = QString(
"delete from student where name = '%1'").arg(name);
-
-
query.exec(str);
//删除信息
-
ui->lineEditName->clear();
-
ui->lineEditNumber->clear();
-
ui->lineEditScore->clear();
-
ui->textEdit->setText(
"删除成功");
-
}
-
}
-
-
-
}
-
-
void Widget::on_pushButtonSearch_clicked()
-
{
-
QString searchname = ui->lineEditName->text();
-
-
if(searchname ==
NULL)
-
{
-
ui->textEdit->setText(
"请输入需要查询的人名");
-
}
-
else
-
{
-
//从数据库中查询是否有这么一个人
-
QString str = QString(
"select *from student where name = '%1'").arg(searchname);
-
QSqlQuery query;
-
query.exec(str);
-
QString name;
-
int number;
-
double score;
-
-
while (query.next())
-
{
-
number = query.value(
0).toInt();
-
name = query.value(
1).toString();
-
score = query.value(
2).toDouble();
-
}
-
-
if(name ==
NULL)
-
{
-
QString a = QString(
"没有叫%1的人,请重新输入人名").arg(searchname);
-
ui->textEdit->setText(a);
-
ui->lineEditName->clear();
-
ui->lineEditNumber->clear();
-
ui->lineEditScore->clear();
-
}
-
else
-
{
-
ui->lineEditName->setText(name);
-
ui->lineEditNumber->setText(QString().setNum(number));
-
ui->lineEditScore->setText(QString().setNum(score));
-
ui->textEdit->setText(
"查询成功");
-
}
-
-
}
-
-
}
-
-
void Widget::on_pushButtonSearchAll_clicked()
-
{
-
QString name[
100];
//用来存储从数据库中找出来的信息
-
int number[
100];
-
double score[
100];
-
int i =
0;
-
QSqlQuery query;
-
query.exec(
"select * from student");
//查询所有的信息
-
while(query.next())
-
{
-
number[i] = query.value(
0).toInt();
-
name[i] = query.value(
1).toString();
-
score[i] = query.value(
2).toDouble();
-
i++;
-
}
-
ui->textEdit->clear();
-
int j =
0;
-
for(j =
0; j < i; j++)
//将这些信息都显示在下方的文本编辑框中
-
{
-
QString str = QString(
"学号:%1 姓名:%2 成绩:%3").arg(number[j]).arg(name[j]).arg(score[j]);
-
ui->textEdit->append(str);
-
}
-
}
-
-
void Widget::on_pushButtonUpdate_clicked()
-
{
-
//从界面获取我们需要的信息
-
QString updatename = ui->lineEditName->text();
-
int number = ui->lineEditNumber->text().toInt();
-
double score = ui->lineEditScore->text().toDouble();
-
if(updatename ==
NULL || number ==
0 || ui->lineEditScore->text() ==
NULL)
-
{
-
ui->textEdit->setText(
"请输入需要修改的人的学号,姓名以及成绩");
-
}
-
else
-
{
-
QString temp = QString(
"select * from student where name = '%1'").arg(updatename);
-
-
QSqlQuery query;
-
query.exec(temp);
// 查询信息
-
QString a;
-
while (query.next())
-
{
-
a = query.value(
1).toString();
-
}
-
-
if(a ==
NULL)
-
{
-
QString b = QString(
"没有名叫%1的人,修改失败").arg(updatename);
-
ui->textEdit->setText(b);
-
ui->lineEditName->clear();
-
ui->lineEditNumber->clear();
-
ui->lineEditScore->clear();
-
}
-
else
-
{
-
temp = QString(
"update student set num = '%1' , score = '%2' where name = '%3'").arg(number).arg(score).arg(updatename);
-
query.exec(temp);
-
ui->textEdit->setText(
"修改成功");
-
ui->lineEditName->clear();
-
ui->lineEditNumber->clear();
-
ui->lineEditScore->clear();
-
}
-
}
-
}