1.建表
DROP TABLE IF EXISTS `account`;
CREATE TABLE `account` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`account` varchar(40) NOT NULL,
`pwd` varchar(40) NOT NULL,
`status` tinyint(1) NOT NULL DEFAULT '0' COMMENT '0不可用,1可用',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=utf8;
如图
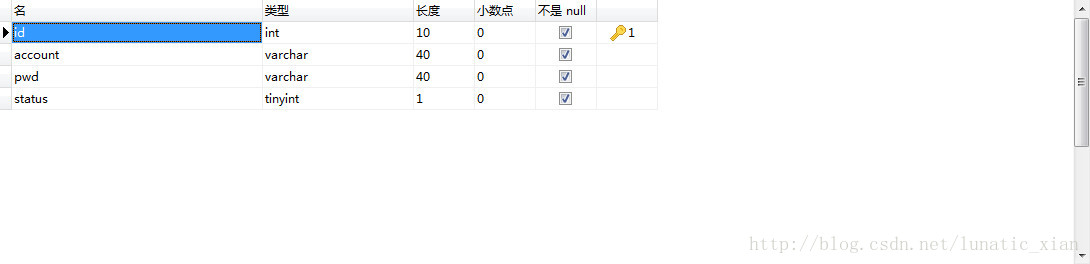
2.编写对应的枚举类
public enum AccountStatus {
// 账号不可用
DISABLE(1, "账号不可用"),
// 账号可用
AVALIABLE(2, "账号可用");
// 用一个map对象存储所有枚举对象
private static Map<Integer, AccountStatus> map = new HashMap<Integer, AccountStatus>();
static {
for (AccountStatus accountStatus : AccountStatus.values()) {
map.put(accountStatus.getValue(), accountStatus);
}
}
public static AccountStatus getEnumByValue(int value) {
return map.get(value);
}
private int value;
private String description;
private AccountStatus(int value, String description) {
this.value = value;
this.description = description;
}
//省略getter和setter方法
}
3.Handler
public class MyEnumHandler extends BaseTypeHandler<AccountStatus> {
/**
* 数据库类型转换为对应的Java类型
*/
@Override
public AccountStatus getNullableResult(ResultSet resultSet, String columnName) throws SQLException {
int value = resultSet.getInt(columnName);
AccountStatus accountStatus = null;
if (!resultSet.wasNull()) {
accountStatus = AccountStatus.getEnumByValue(value);
}
return accountStatus;
}
/**
* 数据库类型转换为对应的Java类型
*/
@Override
public AccountStatus getNullableResult(ResultSet resultSet, int columnIndex) throws SQLException {
int value = resultSet.getInt(columnIndex);
AccountStatus accountStatus = null;
if (!resultSet.wasNull()) {
accountStatus = AccountStatus.getEnumByValue(value);
}
return accountStatus;
}
/**
* 数据库类型转换为对应的Java类型
*/
@Override
public AccountStatus getNullableResult(CallableStatement callableStatement, int columnIndex) throws SQLException {
int value = callableStatement.getInt(columnIndex);
AccountStatus accountStatus = null;
if (!callableStatement.wasNull()) {
accountStatus = AccountStatus.getEnumByValue(value);
}
return accountStatus;
}
/**
* Java类型 转换为对应的数据库类型
*/
@Override
public void setNonNullParameter(PreparedStatement preparedStatement, int parameterIndex, AccountStatus accountStatus, JdbcType jdbcType)
throws SQLException {
preparedStatement.setInt(parameterIndex, accountStatus.getValue());
}
}
4.实体类
public class Account {
private int id;
private String account;
private String pwd;
private AccountStatus status;
//省略getter和setter方法
}
5.映射文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mapper.AccountMapper">
<insert id="insert" parameterType="po.Account">
insert into account (account, pwd, status)
values (#{account,jdbcType=VARCHAR}, #{pwd,jdbcType=VARCHAR},
#{status,typeHandler=util.MyEnumHandler})
</insert>
<resultMap type="po.Account" id="accountMap">
<id column="id" property="id"/>
<result column="account" property="account"/>
<result column="pwd" property="pwd"/>
<result column="status" property="status" typeHandler="util.MyEnumHandler"/>
</resultMap>
<select id="selectByPrimaryKey" resultMap="accountMap">
select id, account, pwd, status from account where id=#{id,jdbcType=INTEGER}
</select>
</mapper>
6.测试
public class Main {
@Test
public void testInsert() {
SqlSession session = MybatisUtil.getSession();
Account account = new Account();
account.setAccount("zidingyi1");
account.setPwd("zidingyi1");
account.setStatus(AccountStatus.AVALIABLE);
session.insert("mapper.AccountMapper.insert", account);
session.commit();
}
@Test
public void testSelect () {
SqlSession session = MybatisUtil.getSession();
Account account = session.selectOne("mapper.AccountMapper.selectByPrimaryKey", 2);
System.out.println(account.getStatus());
if (AccountStatus.AVALIABLE.equals(account.getStatus())) {
System.out.println("账号可用");
} else {
System.out.println("账号不可用");
}
System.out.println(account.getStatus().getDescription());
}
}
6.测试结果
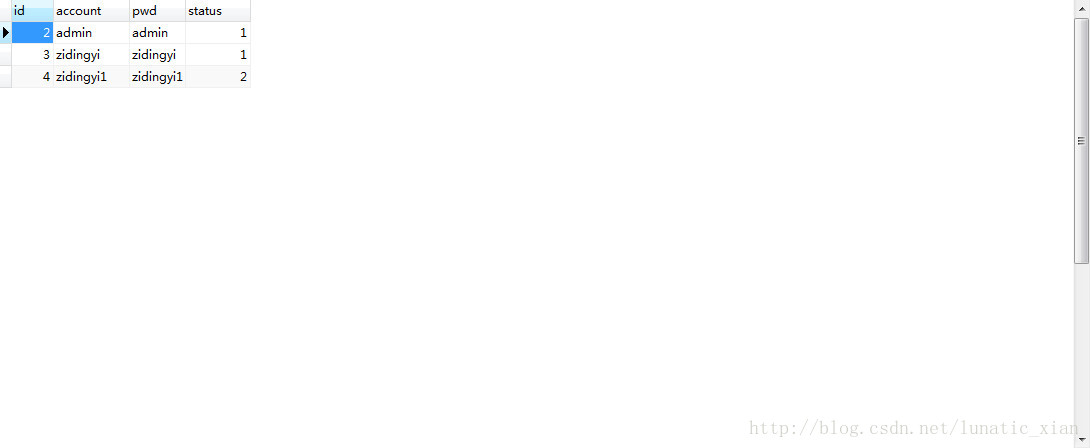