自定义事件选择器。
package app.goa.com.ydbgnew.utils;
import android.app.Dialog;
import android.content.Context;
import android.view.Display;
import android.view.LayoutInflater;
import android.view.View;
import android.view.WindowManager;
import android.widget.Button;
import android.widget.DatePicker;
import android.widget.FrameLayout;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.TimePicker;
import app.goa.com.ydbgnew.R;
/**
* Created by Administrator on 2018/8/8.
*/
public class TimeDialog {
private Context context;
private Dialog dialog;
public static DatePicker datePicker;
public static TimePicker timePicker;
public static TextView time_text;
public static TextView date_text;
private LinearLayout time_dialog_ll;
private Button queren;
private Button quxiao;
private TextView date_select_txt;
private TextView time_select_txt;
private Display display;
public TimeDialog(Context context) {
this.context = context;
WindowManager windowManager = (WindowManager) context
.getSystemService(Context.WINDOW_SERVICE);
display = windowManager.getDefaultDisplay();
}
public TimeDialog builder() {
// 获取Dialog布局
View view = LayoutInflater.from(context).inflate(
R.layout.datepicker_layout, null);
// 获取自定义Dialog布局中的控件
date_select_txt = (TextView) view.findViewById(R.id.date_select_txt);
time_select_txt = (TextView) view.findViewById(R.id.time_select_txt);
time_dialog_ll = (LinearLayout) view.findViewById(R.id.time_dialog_ll);
datePicker = (DatePicker) view.findViewById(R.id.datepicker);
timePicker = (TimePicker) view.findViewById(R.id.timepicker);
time_text = (TextView) view.findViewById(R.id.time_select_txt);
date_text = (TextView) view.findViewById(R.id.date_select_txt);
timePicker.setIs24HourView(true);
quxiao = (Button) view.findViewById(R.id.quxiao);
queren = (Button) view.findViewById(R.id.queren);
// 定义Dialog布局和参数
dialog = new Dialog(context, R.style.AlertDialogStyle);
dialog.setContentView(view);
// 调整dialog背景大小
time_dialog_ll.setLayoutParams(new FrameLayout.LayoutParams((int) (display
.getWidth() * 0.85), LinearLayout.LayoutParams.WRAP_CONTENT));
return this;
}
public void isshowTimePicker(boolean isshow) {
if (!isshow) {
timePicker.setVisibility(View.GONE);
time_select_txt.setVisibility(View.GONE);
}
}
public void isshowDatePicker(boolean isshow) {
if (!isshow) {
datePicker.setVisibility(View.GONE);
date_select_txt.setVisibility(View.GONE);
}
}
public void setdate(int year, int month, int day) {
datePicker.updateDate(year, month, day);
}
public TimeDialog setPositiveButton(String text, final View.OnClickListener listener) {
if ("".equals(text)) {
queren.setText("确定");
} else {
queren.setText(text);
}
queren.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.onClick(v);
dialog.dismiss();
}
});
return this;
}
public TimeDialog setNegativeButton(String text, final View.OnClickListener listener) {
if ("".equals(text)) {
quxiao.setText("取消");
} else {
quxiao.setText(text);
}
quxiao.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.onClick(v);
dialog.dismiss();
}
});
return this;
}
public void show() {
dialog.show();
}
}
对应的布局文件。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/time_dialog_ll"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/alert_bg"
android:orientation="vertical">
<TextView
android:id="@+id/date_select_txt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:gravity="center"
android:text="选择日期"
android:textColor="@color/colorPrimary"
android:textSize="18sp" />
<DatePicker
android:id="@+id/datepicker"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:calendarViewShown="false"
android:theme="@android:style/Theme.Holo.Light.NoActionBar"></DatePicker>
<TextView
android:id="@+id/time_select_txt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:gravity="center"
android:text="选择时间"
android:textColor="@color/actionsheet_blue"
android:textSize="18sp" />
<TimePicker
android:id="@+id/timepicker"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@android:style/Theme.Holo.Light.NoActionBar"></TimePicker>
<View
android:layout_width="match_parent"
android:layout_height="0.5dp"
android:background="@color/alertdialog_line" />
<LinearLayout
android:id="@+id/btns_ll"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/quxiao"
android:layout_width="match_parent"
android:layout_height="43dp"
android:layout_weight="1"
android:background="@drawable/alertdialog_left_selector"
android:gravity="center"
android:text="取消"
android:textColor="@color/actionsheet_blue"
android:textSize="16sp" />
<ImageView
android:id="@+id/img_line"
android:layout_width="0.5dp"
android:layout_height="43dp"
android:background="@color/alertdialog_line" />
<Button
android:id="@+id/queren"
android:layout_width="match_parent"
android:layout_height="43dp"
android:layout_weight="1"
android:background="@drawable/alertdialog_right_selector"
android:gravity="center"
android:text="确认"
android:textColor="@color/actionsheet_blue"
android:textSize="16sp" />
</LinearLayout>
</LinearLayout>
布局文件中用到的颜色。
<color name="colorPrimary">#4bb9ff</color>
<color name="actionsheet_blue">#037BFF</color>
<color name="alertdialog_line">#c6c6c6</color>
用到的背景图片alert_bg,是一张.9图片。

还用到两个样式的xml文件。
其中alertdialog_left_selector.xml内容如下:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/alert_btn_left_pressed" android:state_pressed="true" />
<item android:drawable="@drawable/trans_bg" />
</selector>
其中用到两张图片alert_btn_left_pressed为
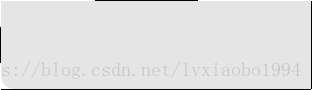
trans_bg为

。
另一个alertdialog_right_selector.xml内容为:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/alert_btn_right_pressed" android:state_pressed="true" />
<item android:drawable="@drawable/trans_bg" />
</selector>
其中alert_btn_right_pressed为
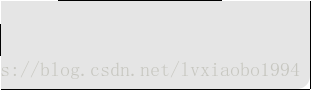
。
trans_bg同上。
然后就是调用了。
public static void showTimeDateAndTime(final View view, final Context context) {
TimeDialog dialog = new TimeDialog(context).builder();
// dialog.isshowTimePicker(false);//设置是否显示时和分
dialog.setPositiveButton("确认", new View.OnClickListener() {
@RequiresApi(api = Build.VERSION_CODES.M)
@Override
public void onClick(View v) {
int year = TimeDialog.datePicker.getYear();
int mouth = TimeDialog.datePicker.getMonth() + 1;
int day = TimeDialog.datePicker.getDayOfMonth();
int hour = TimeDialog.timePicker.getHour();
int minute = TimeDialog.timePicker.getMinute();
String mouth1 = changetime(mouth);
String day1 = changetime(day);
String hour1 = changetime(hour);
String minute1 = changetime(minute);
String time = year + "-" + mouth1 + "-" + day1 + " " + hour1 + ":" + minute1;
}
});
dialog.setNegativeButton("取消", new View.OnClickListener() {
@Override
public void onClick(View v) {
}
});
dialog.show();
}
private static String changetime(int time) {
String time1 = null;
if (time < 10) {
time1 = "0" + time;
} else {
time1 = String.valueOf(time);
}
return time1;
}