1. html代码
<table
id="grid"
style="width: 940px"
title="用户操作"
data-options="iconCls:'icon-view'">
</table>
2.显示
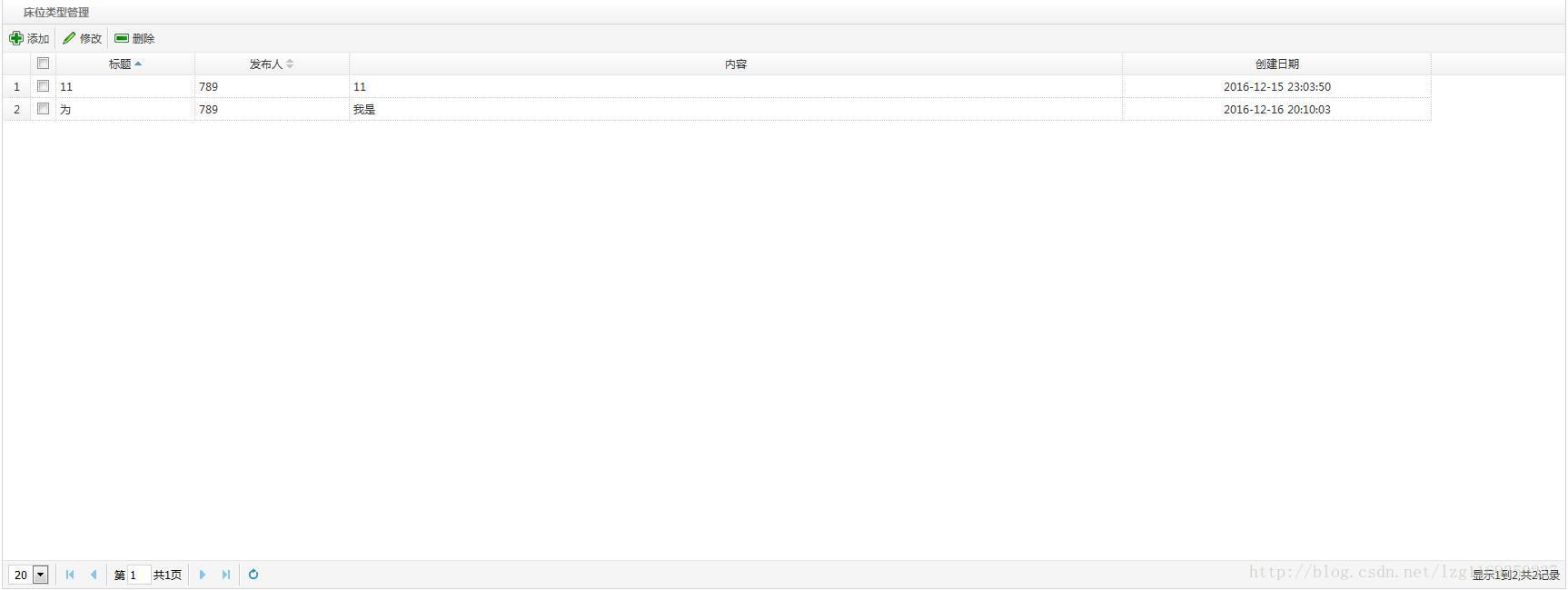
3.js代码
$(function() {
var queryData = {};
InitGrid(queryData);
});
function InitGrid(queryData) {
$('#grid').datagrid({
url : 'getNoticesByUserId',
title : '公告管理',
iconCls : 'icon-view',
height : 650,
width : function() {
return document.body.clientWidth
},
pagination : true,
rownumbers : true,
sortName : 'title',
pageSize : 20,
sortOrder : 'asc',
remoteSort : false,
idField : 'id',
queryParams : queryData,
columns : [ [ {
field : 'ck',
width : '1%',
checkbox : true
}, {
title : '标题',
field : 'title',
width : '9%',
sortable : true,
halign : 'center'
}, {
title : '发布人',
field : 'userName',
width : '10%',
sortable : true,
halign : 'center'
}, {
title : '内容',
field : 'content',
width : '50%',
sortable : true,
halign : 'center',
sortable : false
}, {
title : '创建日期',
field : 'createDate',
width : '20%',
sortable : true,
halign : 'center',
align : 'center',
sortable : false
} ] ],
toolbar : [ {
id : 'btnAdd',
text : '添加',
iconCls : 'icon-add',
handler : function() {
ShowAddDialog();
}
}, '-', {
id : 'btnEdit',
text : '修改',
iconCls : 'icon-edit',
handler : function() {
ShowEditDialog();
}
}, '-', {
id : 'btnDelete',
text : '删除',
iconCls : 'icon-remove',
handler : function() {
Delete();
}
} ]
});
};
4.Json数据
{
"total": 2,
"rows":[{
"content": "11",
"createDate": "2016-12-15 23:03:50",
"id": 1,
"title": "11",
"userName": "789"
}, {
"content": "我是",
"createDate": "2016-12-16 20:10:03",
"id": 4,
"title": "为",
"userName": "789"
}
]
}
5.Java后台封装
/********************1.action代码*******************/
private NoticeManager noticeManager;
private int page;
private int rows;
Map<String, Object> map = new HashMap<String, Object>();
public NoticeManager getNoticeManager() {
return noticeManager;
}
public void setNoticeManager(NoticeManager noticeManager) {
this.noticeManager = noticeManager;
}
public int getPage() {
return page;
}
public void setPage(int page) {
this.page = page;
}
public int getRows() {
return rows;
}
public void setRows(int rows) {
this.rows = rows;
}
public Map<String, Object> getMap() {
return map;
}
public void setMap(Map<String, Object> map) {
this.map = map;
}
/**
* @Title: getNoticesByUserId
* @Description: TODO(获取首页显示的所有公告数据)
* @return??? 设定文件
* @return String??? 返回类型
* @throws
*/
public String getNoticesByUserId() {
List<ANotice> aNotices = new ArrayList<ANotice>();
User u = (User) getSession().get("LoginUser");
List<Notice> notices = noticeManager.GetNotices(page, rows, u.getId());
for (Notice notice : notices) {
ANotice aNotice = new ANotice();
aNotice.setId(notice.getId());
aNotice.setTitle(notice.getTitle());
aNotice.setCreateDate(notice.getCreateDate());
aNotice.setUserName(u.getUsername());
aNotice.setContent(notice.getContent());
aNotices.add(aNotice);
}
map.put("total", noticeManager.getTotal(page, rows, u.getId()));
map.put("rows", aNotices);
return SUCCESS;
}
map.put("total", noticeManager.getTotal(page, rows, u.getId()));
map.put("rows", aNotices);
/********************2.Manager代码*******************/
@Override
public List<Notice> GetNotices(int page, int rows, int userId) {
String hql="From Notice Where 1=1 and userId = ?";
return dao.find(hql, new Object[]{userId}, page, rows);
}
@Override
public Long getTotal(int page, int rows, int userId) {
String hql="select count(*) from Notice Where 1=1 and userId = ?";
return dao.count(hql, new Object[]{userId});
}
/********************3.dao代码*******************/
public List<T> find(String hql, Object[] param, Integer page, Integer rows) {
if (page == null || page < 1) {
page = 1;
}
if (rows == null || rows < 1) {
rows = 10;
}
Query q = this.getCurrentSession().createQuery(hql);
if (param != null && param.length > 0) {
for (int i = 0; i < param.length; i++) {
q.setParameter(i, param[i]);
}
}
return q.setFirstResult((page - 1) * rows).setMaxResults(rows).list();
}
6.struts配置文件
<package name="jsonPackage" extends="struts-default,json-default">
<action name="getNoticesByUserId" class="NoticeAction" method="getNoticesByUserId">
<result name="success" type="json">
<param name="root">map</param>
</result>
</action>
</package>