Kakuro Extension
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Submission(s): 2425 Accepted Submission(s): 844
Special Judge
Problem Description
If you solved problem like this, forget it.Because you need to use a completely different algorithm to solve the following one.
Kakuro puzzle is played on a grid of "black" and "white" cells. Apart from the top row and leftmost column which are entirely black, the grid has some amount of white cells which form "runs" and some amount of black cells. "Run" is a vertical or horizontal maximal one-lined block of adjacent white cells. Each row and column of the puzzle can contain more than one "run". Every white cell belongs to exactly two runs — one horizontal and one vertical run. Each horizontal "run" always has a number in the black half-cell to its immediate left, and each vertical "run" always has a number in the black half-cell immediately above it. These numbers are located in "black" cells and are called "clues".The rules of the puzzle are simple:
1.place a single digit from 1 to 9 in each "white" cell
2.for all runs, the sum of all digits in a "run" must match the clue associated with the "run"
Given the grid, your task is to find a solution for the puzzle.
Picture of the first sample input Picture of the first sample output
Kakuro puzzle is played on a grid of "black" and "white" cells. Apart from the top row and leftmost column which are entirely black, the grid has some amount of white cells which form "runs" and some amount of black cells. "Run" is a vertical or horizontal maximal one-lined block of adjacent white cells. Each row and column of the puzzle can contain more than one "run". Every white cell belongs to exactly two runs — one horizontal and one vertical run. Each horizontal "run" always has a number in the black half-cell to its immediate left, and each vertical "run" always has a number in the black half-cell immediately above it. These numbers are located in "black" cells and are called "clues".The rules of the puzzle are simple:
1.place a single digit from 1 to 9 in each "white" cell
2.for all runs, the sum of all digits in a "run" must match the clue associated with the "run"
Given the grid, your task is to find a solution for the puzzle.
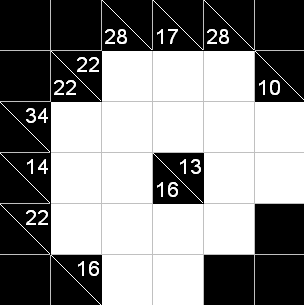
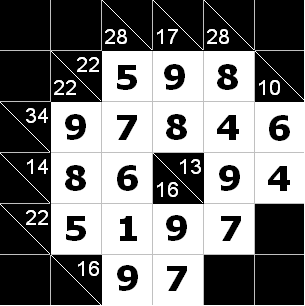
Picture of the first sample input Picture of the first sample output
Input
The first line of input contains two integers n and m (2 ≤ n,m ≤ 100) — the number of rows and columns correspondingly. Each of the next n lines contains descriptions of m cells. Each cell description is one of the following 7-character strings:
.......— "white" cell;
XXXXXXX— "black" cell with no clues;
AAA\BBB— "black" cell with one or two clues. AAA is either a 3-digit clue for the corresponding vertical run, or XXX if there is no associated vertical run. BBB is either a 3-digit clue for the corresponding horizontal run, or XXX if there is no associated horizontal run.
The first row and the first column of the grid will never have any white cells. The given grid will have at least one "white" cell.It is guaranteed that the given puzzle has at least one solution.
.......— "white" cell;
XXXXXXX— "black" cell with no clues;
AAA\BBB— "black" cell with one or two clues. AAA is either a 3-digit clue for the corresponding vertical run, or XXX if there is no associated vertical run. BBB is either a 3-digit clue for the corresponding horizontal run, or XXX if there is no associated horizontal run.
The first row and the first column of the grid will never have any white cells. The given grid will have at least one "white" cell.It is guaranteed that the given puzzle has at least one solution.
Output
Print n lines to the output with m cells in each line. For every "black" cell print '_' (underscore), for every "white" cell print the corresponding digit from the solution. Delimit cells with a single space, so that each row consists of 2m-1 characters.If there are many solutions, you may output any of them.
Sample Input
6 6 XXXXXXX XXXXXXX 028\XXX 017\XXX 028\XXX XXXXXXX XXXXXXX 022\022 ....... ....... ....... 010\XXX XXX\034 ....... ....... ....... ....... ....... XXX\014 ....... ....... 016\013 ....... ....... XXX\022 ....... ....... ....... ....... XXXXXXX XXXXXXX XXX\016 ....... ....... XXXXXXX XXXXXXX 5 8 XXXXXXX 001\XXX 020\XXX 027\XXX 021\XXX 028\XXX 014\XXX 024\XXX XXX\035 ....... ....... ....... ....... ....... ....... ....... XXXXXXX 007\034 ....... ....... ....... ....... ....... ....... XXX\043 ....... ....... ....... ....... ....... ....... ....... XXX\030 ....... ....... ....... ....... ....... ....... XXXXXXX
Sample Output
_ _ _ _ _ _ _ _ 5 8 9 _ _ 7 6 9 8 4 _ 6 8 _ 7 6 _ 9 2 7 4 _ _ _ 7 9 _ _ _ _ _ _ _ _ _ _ _ 1 9 9 1 1 8 6 _ _ 1 7 7 9 1 9 _ 1 3 9 9 9 3 9 _ 6 7 2 4 9 2 _
一个白块需要填数的大小同时被两个黑块限制,这让我们联想到最大流问题
关键是如何建边
首先对题目进行分析,每个黑块的左边数字,等于它下方的行和,每个黑块右边的数字等于他右边的列和
那么每一个白块一定在一个黑块的下方,也一定在另一个黑块的右方,而所有白块组成所有黑块的左边和右边,于是所有黑块的左边之和等于所有黑块的右边之和
有了这个结论,建边的时候就可以限制流量了
1.源点连黑块左边,容量为黑块左边数字 - 下方白块数量
2.黑块右边连汇点,容量为黑块右边数字 - 右方白块数量
3.黑块左边与它下方每一个白块相连,容量为8
4.白块与每一个它左边的黑块相连,容量为8
这样就可以限制流量使得行之和等于列之和
关于容量为8解释一下,因为每个白块能填的数字是[1,9],这就变成了有上下界的网络流问题,可以将问题简化,将原来需要填的值-1,此时值域就变为了[0,8],这样就不用管上下界的问题了,因为所有白块都减去了1,所以源点与左黑块相连时,流量也应减去它所相连的白块数量,右黑块与汇点相连同理
最后输出答案时,再把1加上就行了
#include<stdio.h>
#include<string.h>
#include<algorithm>
#include<queue>
#define INF 0x3f3f3f3f
#define mem(a,b) memset(a,b,sizeof(a))
using namespace std;
const int maxn=20000+50;
const int maxm=2e7+50;
struct node
{
node(){};
node(int tu,int tv,int tw,int tnext){from=tu,v=tv,w=tw,next=tnext;};
int v,w,next,from;
}e[maxm];
int first[maxn],vis[maxn],dis[maxn],tot;
void add_edge(int u,int v,int w)
{
e[tot]=node(u,v,w,first[u]);
first[u]=tot++;
e[tot]=node(v,u,0,first[v]);
first[v]=tot++;
}
int bfs(int s,int t)
{
mem(vis,0);
mem(dis,0);
queue<int>q;
q.push(s);
vis[s]=1;
while(!q.empty())
{
int u=q.front();
q.pop();
for(int i=first[u];~i;i=e[i].next)
{
if(!vis[e[i].v]&&e[i].w>0)
{
vis[e[i].v]=1;
dis[e[i].v]=dis[u]+1;
q.push(e[i].v);
}
}
}
return dis[t];
}
int dfs(int u,int t,int flow)
{
if(u==t)return flow;
for(int i=first[u];~i;i=e[i].next)
{
if(dis[e[i].v]==dis[u]+1&&e[i].w>0)
{
int dd=dfs(e[i].v,t,min(e[i].w,flow));
if(dd)
{
e[i].w-=dd;
e[i^1].w+=dd;
return dd;
}
}
}
dis[u]=0;
return 0;
}
int Dinic(int s,int t)
{
int ans=0,flow;
while(bfs(s,t))
{
while(flow=dfs(s,t,INF))
ans+=flow;
}
return ans;
}
void init()
{
mem(first,-1);
tot=0;
}
char s[105][105][10],ans[105][105];
int main()
{
int n,m;
while(~scanf("%d%d",&n,&m))
{
init();
mem(ans,'_');
for(int i=1; i<=n; i++)
for(int j=1; j<=m; j++)
scanf("%s",s[i][j]+1);
for(int i=1; i<=n; i++)
{
for(int j=1; j<=m; j++)
{
if(s[i][j][4]=='X')continue;
if(s[i][j][4]=='\\')
{
int l=0,r=0,u=(i-1)*m+j,sumx=0,sumy=0;
if(s[i][j][1]!='X') l=(s[i][j][1]-'0')*100+(s[i][j][2]-'0')*10+(s[i][j][3]-'0');
if(s[i][j][5]!='X')r=(s[i][j][5]-'0')*100+(s[i][j][6]-'0')*10+(s[i][j][7]-'0');
for(int k=i+1;l&&k<=n; k++)
{
if(s[k][j][1]=='.')
sumx++;
else
break;
}
l-=sumx;
for(int k=j+1;r&&k<=m; k++)
{
if(s[i][k][1]=='.')
sumy++;
else
break;
}
r-=sumy;
for(int k=i+1;l&&k<=n; k++)
{
if(s[k][j][1]=='.')
{
int v=(k-1)*m+j;
add_edge(u,v,8);
}
else
break;
}
for(int k=j+1;r&&k<=m; k++)
{
if(s[i][k][1]=='.')
{
int v=(i-1)*m+k;
add_edge(v+n*m,u+n*m,8);
}
else
break;
}
add_edge(0,u,l);
add_edge(u+n*m,2*n*m+1,r);
}
else
{
int u=(i-1)*m+j;
int v=u+n*m;
add_edge(u,v,8);
}
}
}
Dinic(0,2*n*m+1);
for(int i=0;i<tot;i++)
{
if(e[i].v+n*m==e[i].from)
{
int x=e[i].v%m?e[i].v/m+1:e[i].v/m;
int y=e[i].v-(x-1)*m;
ans[x][y]=e[i].w+1+'0';
}
}
for(int i=1;i<=n;i++)
{
for(int j=1;j<=m;j++)
{
if(j-1)printf(" ");
printf("%c",ans[i][j]);
}
puts("");
}
}
return 0;
}