1,效果演示
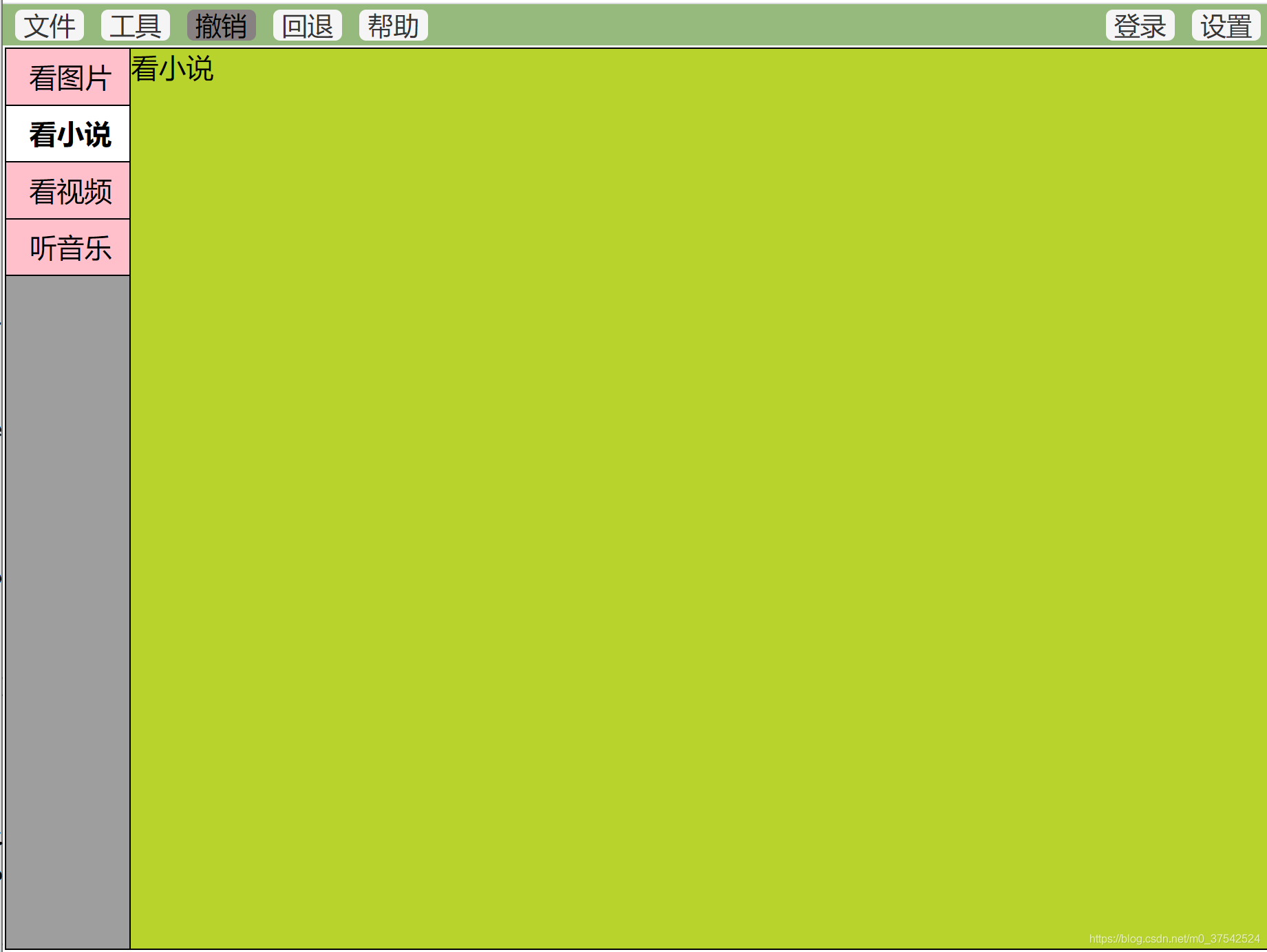
2,代码干货
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>button</title>
<style>
html,body{
width:100%;
height:100%;
margin:0;
padding:0;
font-family:"Microsoft YaHei", SimSun;
}
ul li{
list-style:none;
}
.button:hover{
color:white;
background-color:gray;
}
.button{
display:inline-block;
width:40px;
height:18px;
font-size:15px;
text-align:center;
line-height:18px;
margin:1px 5px 1px 5px;
border-radius:4px;
color:#353535;
background-color:#f5f5f5;
}
.button-span{
width:100%;
height:22px;
margin:0;
padding:1px;
background-color:#96b97d;;
}
.button-span .tool-bar{
display:inline-block;
float:left;
width:65%;
margin:0;
padding:1px;
}
.button-span .tool-bar .selected{
color:black;
background-color:#888181;
}
.button-span .tool-bar .button{
float:left;
}
.button-span .setting-bar .selected{
color:black;
background-color:#888181;
}
.button-span .setting-bar{
display:inline-block;
float:right;
width:30%;
margin:0;
padding:1px;
}
.button-span .setting-bar .button{
float:right;
}
.func-span{
width:100%;
height:30px;
background-color:yellow;
}
.main-area{
display:block;
width:100%;
height:80%;
margin:0;
padding:0;
}
.main-area .func-bar{
display:inline-block;
float:left;
width:10%;
height:100%;
}
.main-area .func-bar ul{
width:99%;
height:100%;
padding:0;
margin:1px 0px 1px 1px;
border:solid 1px black;
border-right:0;
background-color:#9e9e9e;
}
.main-area .func-bar ul .selected{
font-weight:bold;
background-color:white;
}
.main-area .func-bar ul li:hover{
background-color:#f5f5f5;
}
.main-area .func-bar ul li{
display:block;
float:left;
width:100%;
height:30px;
padding:1px;
border-bottom:solid 1px black;
text-align:center;
line-height:30px;
background-color:pink;
}
.main-area .show-area{
display:inline-block;
width:90%;
height:100%;
}
.main-area .show-area .process-area{
width:100%;
height:100%;
margin:1px 0 1px 0;
border:solid 1px black;
background-color:white;
}
</style>
</head>
<body>
<div class="button-span">
<ul id="id-tool-bar" class="tool-bar">
<li class="button">文件</li>
<li class="button">工具</li>
<li class="button">撤销</li>
<li class="button">回退</li>
<li class="button">帮助</li>
</ul>
<ul id="id-setting-bar" class="setting-bar">
<li class="button">设置</li>
<li class="button">登录</li>
</ul>
</div>
<div class="main-area">
<div class="func-bar">
<ul id="id-func-buttons">
<li>看图片</li>
<li>看小说</li>
<li>看视频</li>
<li>听音乐</li>
</ul>
</div>
<div class="show-area">
<div id="id-process-area" class="process-area">
main area
</div>
</div>
</div>
<script>
window.onload = function(){
EventUtils = {
addEventListener : function(element, eventType, func){
if(element.addEventListener) {
element.addEventListener(eventType, func);
}else if(element.attachEvent) {
element.attachEvent("on"+eventType, func);
}else{
element["on"+eventType] = func;
}
},
moveEventListener : function(element, eventType, func){
if(element.removeEventListener) {
element.removeEventListener(eventType, func);
}else if(element.detachEvent) {
element.detachEvent("on"+eventType, func);
}else{
element["on"+eventType] = undefined;
}
},
addEventForButtons : function(parentNode, eventType, func)
{
lis = parentNode.getElementsByTagName("li");
console.log(lis, eventType, func);
for(var i=0; i<lis.length; i++)
{
var node = lis.item(i);
console.log(node);
if(typeof(func) != "object")
{
EventUtils.addEventListener(node, eventType, func);
continue;
}
for(var j=0; j<func.length; j++){
fun = func[j];
EventUtils.addEventListener(node, eventType, fun);
}
}
}
}
addButtonsSelectEvent = function(event){
element = event.target;
lis = element.parentNode.children;
for(var i=0; i<lis.length; i++){
var node = lis[i];
node.index = i;
if(node == element && !node.classList.contains("selected")){
node.classList.add("selected");
}else if(node.classList.contains("selected")){
node.classList.remove("selected");
}
}
}
selectAreaByButton = function(event){
var areaColor = new Array('fed400','b8d32b','00a5cc','a61366','ed7419','e83331','ac4223','00a2e7','45b146');
var processArea = document.getElementById("id-process-area");
processArea.innerHTML = event.target.innerHTML;
processArea.style.background = "#"+areaColor[event.target.index];
}
EventUtils.addEventForButtons(document.getElementById("id-tool-bar"), "click", addButtonsSelectEvent);
EventUtils.addEventForButtons(document.getElementById("id-setting-bar"), "click", addButtonsSelectEvent);
EventUtils.addEventForButtons(document.getElementById("id-func-buttons"), "click", [addButtonsSelectEvent, selectAreaByButton]);
}
</script>
</body>
</html>