1,效果
2,代码
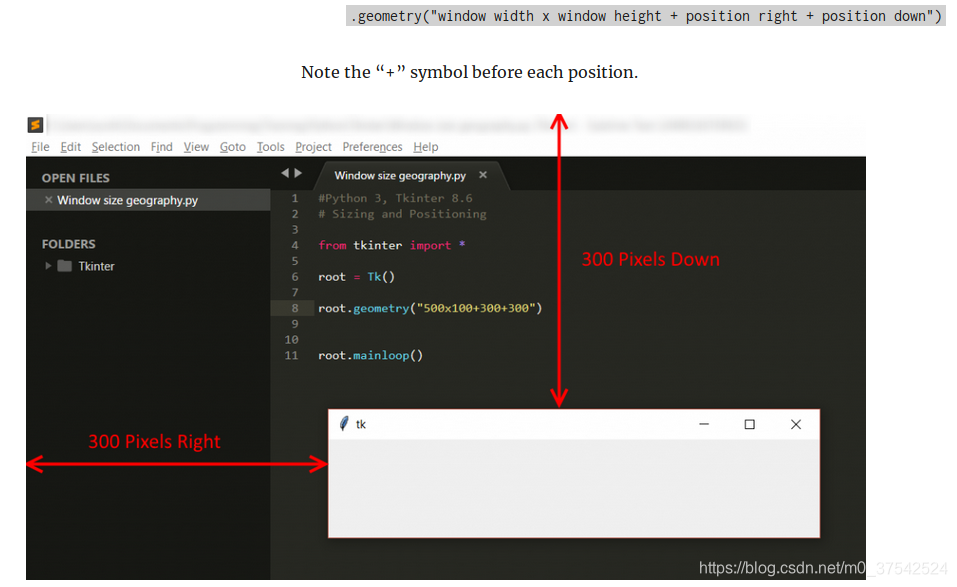
from tkinter import *
import time
class FloadWindow(object):
def __init__(self, shape, locate):
self.wnd = Tk()
s_shape = str(shape[0])+'x'+str(shape[1])
s_locate = '+' + str(locate[0]-shape[0]) + '+' + str(locate[1])
self.wnd.overrideredirect(True)
path = ['camera_1', 'camera_2', 'camera_3', 'camera_4', 'camera_5', 'camera_6']
for i in range(len(path)):
label = Label(self.wnd, text=path[i])
label.place(x=0,y=i*15+5)
for width in range(0, shape[0], int(shape[0]/20)):
time.sleep(0.01)
self.overturn((width, shape[1]), locate)
def overturn(self, shape, locate):
s_shape = str(shape[0])+'x'+str(shape[1])
s_locate = '+' + str(locate[0]-shape[0]) + '+' + str(locate[1])
self.wnd.geometry(s_shape + s_locate)
self.wnd.update()
def destroy(self, shape, locate):
for width in range(shape[0], 0, -int(shape[0]/20)):
time.sleep(0.01)
self.overturn((width, shape[1]), locate)
self.wnd.destroy()
class RootWindow(object):
def __init__(self, shape, locate):
self.wnd = Tk()
self.flag = False
s_shape = str(shape[0])+'x'+str(shape[1])
s_locate = '+' + str(locate[0]-shape[0]) + '+' + str(locate[1])
self.wnd.geometry(s_shape + s_locate)
self.close = False
button = Button(self.wnd, text='on/off', command=self.fold_on_off)
button.pack()
def fold_on_off(self):
if not self.flag:
self.flag = True
self.fload_window = FloadWindow((150, 150),(self.wnd.winfo_x(), self.wnd.winfo_y()))
else:
self.fload_window.destroy((150, 150),(self.wnd.winfo_x(), self.wnd.winfo_y()))
self.flag = False
def wnd_update(self):
self.wnd.update()
if self.flag:
self.fload_window.overturn((150, 150), (self.wnd.winfo_x(), self.wnd.winfo_y()))
if __name__ == '__main__':
root = RootWindow((300,200),(500,100))
while True:
root.wnd_update()
root.wnd.mainloop()
3,连续多次点击弹窗按钮,重复打开窗口问题
from tkinter import *
import time
from listdir2 import HbarFrame
import os
from queue import Queue
class FoldWindow(object):
def __init__(self, shape, locate):
self.wnd = Tk()
self.wnd.overrideredirect(True)
self.overturn((0, shape[1]), locate)
path = ['camera_1', 'camera_2', 'camera_3', 'camera_4', 'camera_5', 'camera_6']
for i in range(len(path)):
label = Label(self.wnd, text=path[i])
label.place(x=0,y=i*15+5)
self.create(shape, locate)
def overturn(self, shape, locate):
s_shape = str(shape[0])+'x'+str(shape[1])
s_locate = '+' + str(locate[0]-shape[0]) + '+' + str(locate[1])
self.wnd.geometry(s_shape + s_locate)
self.wnd.update()
def create(self, shape, locate):
for width in range(0, shape[0], int(shape[0]/20)):
self.overturn((width, shape[1]), locate)
time.sleep(0.01)
def destroy(self, shape, locate):
for width in range(shape[0], 0, -int(shape[0]/20)):
self.overturn((width, shape[1]), locate)
time.sleep(0.01)
self.wnd.destroy()
class RootWindow(object):
def __init__(self, shape, locate):
self.wnd = Tk()
self.flag = False
s_shape = str(shape[0])+'x'+str(shape[1])
s_locate = '+' + str(locate[0]-shape[0]) + '+' + str(locate[1])
self.wnd.geometry(s_shape + s_locate)
self.fold_window_shape = (150,200)
self.fold_window_list = []
button = Button(self.wnd, text='on/off', command=self.fold_on_off)
button.pack()
def fold_on_off(self):
if not self.flag:
if len(self.fold_window_list) == 0:
self.fold_window = FoldWindow(self.fold_window_shape,
(self.wnd.winfo_x(), self.wnd.winfo_y()))
self.fold_window_list.insert(0, self.fold_window)
while len(self.fold_window_list) > 1:
self.fold_window_list.pop().wnd.destroy()
self.flag = True
else:
if len(self.fold_window_list) > 0:
self.fold_window_list.pop().destroy(self.fold_window_shape,
(self.wnd.winfo_x(), self.wnd.winfo_y()))
self.flag = False
def wnd_update(self):
self.wnd.update()
if self.flag:
self.fold_window.overturn(self.fold_window_shape,
(self.wnd.winfo_x(), self.wnd.winfo_y()))
if __name__ == '__main__':
root = RootWindow((300,200),(500,100))
while True:
root.wnd_update()
root.wnd.mainloop()