package com.test;
import java.util.regex.Pattern;
public class TestRegex {
/**
* 正则表达式:验证单个手机号
*/
public static final String REGEX_MOBILE = "^((17[0-9])|(14[0-9])|(13[0-9])|(15[^4,\\D])|(18[0,5-9]))\\d{8}$";
/**
* 正则表达式:验证手机号,多个以英文逗号分隔
*/
public static final String REGEX_MOBILE_MULTI = "^1[3578][0-9]{9}(,1[3578][0-9]{9})*$";
/**
* 正则表达式:验证单个邮箱(允许英文大小写字母、数字、下划线、英文句号、以及中划线组成)
*/
public static final String REGEX_EMAIL = "^([a-z0-9A-Z]+[-|\\.]?)+[a-z0-9A-Z]@([a-z0-9A-Z]+(-[a-z0-9A-Z]+)?\\.)+[a-zA-Z]{2,}$";
/**
* 正则表达式:验证邮箱,多个以英文逗号分隔
*/
public static final String REGEX_EMAIL_MULTI = "^([a-z0-9A-Z]+[-|\\.]?)+[a-z0-9A-Z]@([a-z0-9A-Z]+(-[a-z0-9A-Z]+)?\\.)+[a-zA-Z]{2,}(,([a-z0-9A-Z]+[-|\\.]?)+[a-z0-9A-Z]@([a-z0-9A-Z]+(-[a-z0-9A-Z]+)?\\.)+[a-zA-Z]{2,})*$";
/**
* 校验手机号
*
* @param mobile
* @return 校验通过返回true,否则返回false
*/
public static boolean isMobile(String mobile) {
// return Pattern.matches(REGEX_MOBILE, mobile);
return Pattern.matches(REGEX_MOBILE_MULTI, mobile);
}
/**
* 校验邮箱
*
* @param email
* @return 校验通过返回true,否则返回false
*/
public static
验证手机号邮箱,多个以英文逗号分隔
最新推荐文章于 2023-05-08 16:02:10 发布
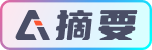